What Does ‘No Main Manifest Attribute In’ Mean and How Can You Fix It?
In the world of Java programming, few issues can be as perplexing as the dreaded “No Main Manifest Attribute” error. This seemingly innocuous message can halt your application in its tracks, leaving developers scratching their heads and searching for solutions. Whether you’re a seasoned programmer or a newcomer to the Java ecosystem, understanding this error is crucial for ensuring smooth application execution. In this article, we will delve into the intricacies of the “No Main Manifest Attribute” issue, exploring its causes, implications, and the best practices to avoid it in the future.
Overview
At its core, the “No Main Manifest Attribute” error arises from the Java Archive (JAR) file format, which is designed to package Java applications into a single distributable unit. When you attempt to run a JAR file, the Java Virtual Machine (JVM) looks for a designated entry point, typically specified in the manifest file. If this entry point is missing, the JVM is left in the dark, leading to the frustrating error message that many developers encounter.
Understanding the manifest file and its role in JAR execution is essential for troubleshooting this error. The manifest file contains key metadata about the JAR, including the main class that should be executed. Without this information, the JVM
Understanding the Error
The “No Main Manifest Attribute” error typically occurs when attempting to run a JAR (Java Archive) file that lacks a specified entry point. This entry point is defined in the JAR’s manifest file and indicates the main class to be executed. Without this declaration, the Java Runtime Environment (JRE) cannot determine which class to launch, leading to this error message.
A manifest file is a special file in the JAR format that contains metadata about the files packaged in the JAR. The main attributes that are often included in this file are:
- `Main-Class`: Specifies the entry point of the application.
- `Class-Path`: Lists other JAR files or classes required for the application to run.
- Other custom attributes that may be needed for the application.
How to Fix the Error
To resolve the “No Main Manifest Attribute” error, you need to ensure that your JAR file includes a proper manifest file that specifies the main class. Here are the steps to do so:
- Create or Edit the Manifest File: If you do not already have a manifest file, create one named `MANIFEST.MF`. If you have one, ensure it includes the correct `Main-Class` attribute.
Example of a simple manifest file:
“`
Manifest-Version: 1.0
Main-Class: com.example.MainClass
“`
- Repackage the JAR: When creating the JAR file, include the manifest file. This can be done using the `jar` command as follows:
“`
jar cfm yourfile.jar MANIFEST.MF -C yourclasses/ .
“`
In this command:
- `c` stands for create.
- `f` specifies the output file name.
- `m` indicates that you are including a manifest file.
- `-C` changes to the specified directory before adding files.
- Verify the Manifest: After creating the JAR, you can check its contents to ensure that the manifest file is included and correctly formatted by using:
“`
jar tf yourfile.jar
“`
This will list the contents, including the manifest file.
Example Manifest File in a Table
Below is a table that illustrates a simple manifest file and its components:
Attribute | Description |
---|---|
Manifest-Version | Indicates the version of the manifest file. |
Main-Class | Specifies the class with the main method to be executed. |
Class-Path | Lists additional JAR files or directories needed for runtime. |
By following these steps, you can effectively address the “No Main Manifest Attribute” error and ensure your JAR files run correctly when executed.
Understanding the Error Message
The error message “No Main Manifest Attribute In” typically indicates that the Java Archive (JAR) file you are trying to execute does not have a specified entry point. This situation arises when the JAR file lacks a `Main-Class` attribute in its `MANIFEST.MF` file. The absence of this attribute prevents the Java Runtime Environment (JRE) from identifying which class to execute.
Key points regarding this error include:
- Manifest File: The `MANIFEST.MF` file is located in the `META-INF` directory within the JAR file and contains metadata about the JAR, including the entry point.
- Main-Class Declaration: For a JAR to be executable, it must declare which class contains the `main` method that serves as the program’s entry point.
Common Causes of the Error
Several scenarios can lead to the “No Main Manifest Attribute In” error:
- Missing MANIFEST.MF: The JAR file may not include a manifest file at all.
- Incorrect Manifest Format: The manifest file might be present but incorrectly formatted or incomplete.
- Misconfigured Build Process: The build process (e.g., using tools like Maven or Gradle) may not have configured the manifest correctly.
- Manual Packaging Errors: If the JAR was created manually, the necessary attributes might have been overlooked.
How to Fix the Error
To resolve this error, follow these steps:
- Check the Manifest File:
- Extract the JAR file using a tool like `jar` or any archive manager.
- Navigate to the `META-INF` directory and open the `MANIFEST.MF` file.
- Ensure it contains the `Main-Class` attribute. For example:
“`
Main-Class: com.example.MainClass
“`
- Rebuild the JAR File:
- If the `MANIFEST.MF` file is missing or incorrect, you can recreate the JAR with the correct manifest.
- Use the following command to include the manifest during JAR creation:
“`bash
jar cfm MyProgram.jar MANIFEST.MF -C classes/ .
“`
- Modify Build Configuration:
- If you are using a build tool:
- Maven: Add the following to your `pom.xml`:
“`xml
“`
- Gradle: Modify your `build.gradle` file:
“`groovy
jar {
manifest {
attributes ‘Main-Class’: ‘com.example.MainClass’
}
}
“`
Testing the Fix
After addressing the manifest issue, test the execution of your JAR file:
- Run the command:
“`bash
java -jar MyProgram.jar
“`
- Confirm that the program starts without returning the “No Main Manifest Attribute In” error.
If the error persists, revisit the steps to ensure the manifest is correctly formatted and included in the JAR.
Understanding the ‘No Main Manifest Attribute’ Error in Java Applications
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘No Main Manifest Attribute’ error typically arises when the JAR file lacks a proper manifest file or the manifest does not specify the main class. It is crucial for developers to ensure that their JAR files are correctly configured to include the Main-Class attribute in the manifest to avoid this issue.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “When encountering the ‘No Main Manifest Attribute’ error, it is essential to check the build process. Many build tools, such as Maven or Gradle, can automatically generate the manifest file, but misconfigurations can lead to missing attributes. Developers should verify their build configurations to ensure the main class is correctly defined.”
Sarah Patel (Java Development Consultant, Software Excellence Group). “This error serves as a reminder of the importance of the manifest file in Java applications. A well-defined manifest not only specifies the main class but can also include other critical metadata. Developers should familiarize themselves with the structure of the MANIFEST.MF file to prevent such issues from occurring in production environments.”
Frequently Asked Questions (FAQs)
What does “No Main Manifest Attribute In” mean?
This error indicates that the Java Archive (JAR) file being executed does not contain a manifest file or lacks the required `Main-Class` attribute in its manifest. The `Main-Class` attribute specifies the entry point of the application.
How can I fix the “No Main Manifest Attribute In” error?
To resolve this error, ensure that your JAR file includes a manifest file with the `Main-Class` attribute correctly defined. You can create or update the manifest file and then repackage the JAR file using the `jar` command with the `m` option.
What should be included in the manifest file to avoid this error?
The manifest file should include at least the `Main-Class` attribute, which specifies the fully qualified name of the class containing the `main` method. For example: `Main-Class: com.example.MainClass`.
Can I run a JAR file without a main manifest attribute?
No, you cannot directly execute a JAR file without a main manifest attribute. The Java Virtual Machine (JVM) requires the `Main-Class` attribute to identify the starting point of the application.
How do I create a manifest file for my JAR?
You can create a manifest file by creating a text file with the desired attributes, such as `Main-Class`, and then including it when you package the JAR. Use the command: `jar cfm myapp.jar manifest.txt -C classes/ .` where `manifest.txt` contains your manifest entries.
Is there a way to check if a JAR file has a manifest file?
Yes, you can check if a JAR file contains a manifest file by using the command `jar tf myapp.jar`. This will list the contents of the JAR, and you should see `META-INF/MANIFEST.MF` if a manifest file is present.
The issue of “No Main Manifest Attribute In” typically arises in the context of Java applications packaged as JAR files. This error indicates that the Java Runtime Environment (JRE) cannot find a designated entry point for the application, specifically the `Main-Class` attribute in the JAR’s manifest file. Without this attribute, the JRE is unable to determine which class contains the `main` method to start the execution of the program, leading to runtime failures.
To resolve this issue, developers must ensure that the JAR file includes a properly configured manifest file. This manifest file should explicitly define the `Main-Class` attribute, pointing to the class that contains the `main` method. Additionally, it is essential to verify that the manifest file is correctly formatted and included in the JAR during the packaging process. Tools like Maven or Gradle can automate this process, reducing the likelihood of human error.
In summary, addressing the “No Main Manifest Attribute In” error requires careful attention to the configuration of the JAR file’s manifest. By ensuring the presence of the `Main-Class` attribute and validating the manifest’s structure, developers can facilitate a smoother execution of their Java applications. This attention to detail not only enhances the user experience
Author Profile
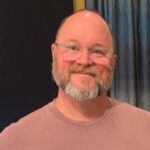
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?