Why Am I Seeing ‘No Access-Control-Allow-Origin Header Is Present On The Requested Resource’ Error?
In today’s interconnected digital landscape, web applications often rely on resources from multiple domains to deliver rich and dynamic user experiences. However, this seamless integration can sometimes lead to unexpected hurdles, particularly when it comes to security protocols. One common issue developers encounter is the absence of the `Access-Control-Allow-Origin` header, which can prevent web applications from accessing resources hosted on different origins. This seemingly minor detail can disrupt functionality and hinder user experience, making it crucial for developers and webmasters to understand its implications.
The `Access-Control-Allow-Origin` header is a key component of the Cross-Origin Resource Sharing (CORS) protocol, designed to protect users and their data by controlling how resources are shared across different origins. When a web application attempts to fetch resources from another domain, the server must explicitly allow this interaction by including the appropriate CORS headers. Without these headers, browsers will block the request, resulting in the frustrating error message: “No Access-Control-Allow-Origin Header Is Present On The Requested Resource.”
Understanding the nuances of CORS and the role of the `Access-Control-Allow-Origin` header is essential for developers looking to create robust and secure web applications. In the following sections, we will delve into the causes of this error, explore its implications for web development, and provide practical solutions
Understanding CORS and the Error
Cross-Origin Resource Sharing (CORS) is a security feature implemented in web browsers that allows or restricts resources requested from a domain outside the domain from which the first resource was served. When a web application makes a request to another domain, the browser checks for the presence of the `Access-Control-Allow-Origin` header. If this header is not present, the browser blocks the response, leading to the error message: “No Access-Control-Allow-Origin header is present on the requested resource.”
This behavior is crucial for preventing cross-site request forgery and other malicious actions. To avoid this error, it’s essential to understand how to properly configure CORS on the server.
Common Causes of the Error
The absence of the `Access-Control-Allow-Origin` header can occur for several reasons:
- Server Configuration: The server may not be configured to include the CORS headers in its responses.
- Incorrect URL: The request may be sent to an incorrect or non-existent resource, which results in a response that lacks the necessary headers.
- Preflight Requests: For certain types of requests, browsers send a preflight request using the OPTIONS method. If the server does not handle this request correctly, it may not send the required CORS headers.
How to Fix the Error
To resolve the “No Access-Control-Allow-Origin header is present on the requested resource” error, the server must be configured to include the appropriate CORS headers. Here are steps to implement the fix:
- **Update Server Configuration**: Depending on the server technology in use, you need to set the `Access-Control-Allow-Origin` header.
- For **Node.js/Express**:
“`javascript
app.use((req, res, next) => {
res.header(“Access-Control-Allow-Origin”, “*”);
next();
});
“`
- For Apache:
“`apache
Header set Access-Control-Allow-Origin “*”
“`
- For Nginx:
“`nginx
add_header ‘Access-Control-Allow-Origin’ ‘*’;
“`
- Handle Preflight Requests: Ensure that the server responds to OPTIONS requests with appropriate CORS headers.
- Specify Allowed Origins: Instead of using a wildcard (`*`), specify allowed origins for better security.
CORS Header Options
When configuring CORS, several headers can be utilized:
Header | Description |
---|---|
Access-Control-Allow-Origin | Specifies which origins are allowed to access the resource. |
Access-Control-Allow-Methods | Indicates the HTTP methods that are allowed when accessing the resource. |
Access-Control-Allow-Headers | Lists the HTTP headers that can be used when making the actual request. |
Access-Control-Allow-Credentials | Indicates whether or not the browser should include credentials with the request. |
By properly implementing these headers and understanding their implications, developers can effectively manage CORS policies and avoid common pitfalls associated with cross-origin requests.
Understanding CORS and Its Importance
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers that allows or restricts resources requested from another domain outside the domain from which the first resource was served. This is crucial for maintaining the security and integrity of web applications.
- Same-Origin Policy: By default, web browsers enforce a same-origin policy that prevents scripts running on one origin from interacting with resources from another origin.
- CORS Headers: To permit cross-origin requests, a server must include specific HTTP headers in its responses.
Common CORS Headers
The following headers are key components in managing CORS:
Header | Description |
---|---|
`Access-Control-Allow-Origin` | Specifies which origins are permitted to access the resource. |
`Access-Control-Allow-Methods` | Lists the HTTP methods (e.g., GET, POST) that are allowed when accessing the resource. |
`Access-Control-Allow-Headers` | Defines which HTTP headers can be used during the actual request. |
`Access-Control-Allow-Credentials` | Indicates whether credentials (like cookies) can be included in requests. |
Reasons for Missing Access-Control-Allow-Origin Header
When the `Access-Control-Allow-Origin` header is absent, browsers will block the request due to CORS policy. This can occur for several reasons:
- Server Misconfiguration: The server may not be set up to handle cross-origin requests.
- Development Environment Issues: In local development setups, CORS may not be properly configured.
- API Restrictions: Some APIs explicitly block cross-origin requests for security purposes.
How to Resolve CORS Issues
To address the absence of the `Access-Control-Allow-Origin` header, consider the following solutions:
– **Server-Side Changes**:
- Modify the server configuration to include the appropriate CORS headers.
- For example, in Node.js with Express, you can use the following code:
“`javascript
app.use((req, res, next) => {
res.header(“Access-Control-Allow-Origin”, “*”);
res.header(“Access-Control-Allow-Headers”, “Origin, X-Requested-With, Content-Type, Accept”);
next();
});
“`
- Proxying Requests:
- Use a server-side proxy to relay requests, ensuring that the request appears to originate from the same domain.
- Browser Extensions:
- Temporarily disable CORS in development environments using browser extensions designed for this purpose. Note that this is not recommended for production.
Testing CORS Configurations
It is essential to verify that CORS configurations are correctly implemented. Use the following methods to test:
- Browser Developer Tools: Check the network tab for CORS-related errors when making requests.
- CORS Test Tools: Utilize online tools or command-line utilities like `curl` to send requests and inspect the response headers.
- Automated Testing: Implement automated tests in your development pipeline to regularly check CORS configurations.
By understanding CORS and properly configuring server responses, developers can ensure their web applications operate securely and efficiently across different domains.
Understanding the Implications of Missing Access-Control-Allow-Origin Headers
Dr. Emily Carter (Web Security Analyst, CyberSafe Solutions). “The absence of the Access-Control-Allow-Origin header can lead to significant security vulnerabilities, particularly in web applications that rely on cross-origin resource sharing. Developers must ensure that this header is correctly configured to prevent unauthorized access to sensitive data.”
Mark Johnson (Lead Developer, CloudTech Innovations). “When a resource lacks the Access-Control-Allow-Origin header, browsers will block cross-origin requests. This can disrupt functionality in applications that need to interact with APIs or resources from different domains, leading to a poor user experience.”
Lisa Tran (Compliance Officer, DataGuard Inc.). “Organizations must prioritize the implementation of the Access-Control-Allow-Origin header as part of their compliance with data protection regulations. Failure to do so not only exposes them to security risks but may also lead to legal ramifications regarding user data handling.”
Frequently Asked Questions (FAQs)
What does it mean when there is no Access-Control-Allow-Origin header present?
The absence of the Access-Control-Allow-Origin header indicates that the server is not configured to allow cross-origin requests, which can lead to security restrictions in web applications.
Why is the Access-Control-Allow-Origin header important?
This header is crucial for implementing Cross-Origin Resource Sharing (CORS), which enables secure interactions between web pages and resources from different origins, preventing unauthorized access.
How can I resolve the issue of a missing Access-Control-Allow-Origin header?
To resolve this issue, configure the server to include the Access-Control-Allow-Origin header in its responses, specifying which origins are permitted to access the resource.
What are the potential security implications of allowing all origins with the Access-Control-Allow-Origin header?
Allowing all origins using a wildcard (*) can expose the resource to cross-site request forgery (CSRF) attacks, making it essential to restrict access to trusted domains only.
Can I use browser developer tools to troubleshoot Access-Control-Allow-Origin header issues?
Yes, browser developer tools can help identify CORS-related errors in the console, allowing developers to see which requests are being blocked due to missing or misconfigured headers.
Are there any alternatives to the Access-Control-Allow-Origin header for handling cross-origin requests?
Alternatives include using JSONP for GET requests or implementing a proxy server that communicates with the target resource, although these methods have limitations and security considerations.
The absence of the “Access-Control-Allow-Origin” header on a requested resource is a critical issue in web development, particularly concerning cross-origin resource sharing (CORS). This header is essential for enabling secure interactions between resources from different origins. When a web application attempts to access resources from another domain without this header, the browser will block the request, leading to functionality issues and a poor user experience. Understanding the implications of this header is vital for developers who aim to create seamless and secure web applications.
One of the key takeaways is the importance of configuring server settings to include the “Access-Control-Allow-Origin” header appropriately. Developers must ensure that their server is set up to respond with this header for requests coming from different origins. This may involve specifying allowed origins or using wildcard settings, depending on the security requirements of the application. Properly managing these settings can help prevent unauthorized access while still allowing legitimate cross-origin requests.
Another significant insight is the necessity of testing and debugging CORS-related issues during the development process. Tools such as browser developer consoles can provide valuable feedback on CORS errors, allowing developers to identify and rectify issues promptly. By proactively addressing these concerns, developers can enhance the functionality and security of their web applications, ensuring a smoother
Author Profile
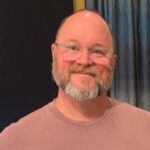
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?