How Can You Effectively Use Ms SQL Search Stored Procedures for Text Queries?
In the vast realm of data management, Microsoft SQL Server (Ms SQL) stands out as a powerful tool for businesses and developers alike. One of its most valuable features is the ability to create and execute stored procedures—predefined SQL statements that streamline complex operations and enhance performance. However, as databases grow in size and complexity, the need for efficient text searching within these stored procedures becomes increasingly critical. This article delves into the intricacies of searching for text within stored procedures in Ms SQL, equipping you with the knowledge to optimize your database queries and improve your overall data retrieval processes.
When working with stored procedures, developers often face the challenge of locating specific text strings or commands buried within extensive code. Whether you’re troubleshooting, refactoring, or simply seeking to understand existing procedures, knowing how to efficiently search through these scripts is essential. Ms SQL offers a variety of tools and techniques to facilitate this process, allowing users to pinpoint relevant information quickly and accurately. By mastering these methods, you can save valuable time and reduce the frustration associated with manual searches.
Moreover, understanding how to effectively search for text in stored procedures can lead to improved database management practices. It empowers developers to maintain cleaner code, identify redundancies, and ensure compliance with best practices. As we explore the various strategies and functionalities
Understanding Full-Text Search in SQL Server
Full-text search in SQL Server allows users to perform complex queries against character-based data. This functionality is particularly beneficial for searching through large volumes of text efficiently. SQL Server uses a specialized engine to handle full-text indexing, which significantly enhances search performance compared to standard SQL queries.
To utilize full-text search, you need to create a full-text index on the desired columns of a table. This index will facilitate quick searches through the text data. The process involves several steps:
- Ensure that the SQL Server instance has full-text search feature enabled.
- Create a full-text catalog.
- Create a full-text index on the table.
The following table outlines the key components involved in setting up full-text search:
Component | Description |
---|---|
Full-Text Catalog | A logical container for full-text indexes, which can include one or more indexes. |
Full-Text Index | An index that contains a mapping of words to their locations within a column. |
Full-Text Search Query | SQL queries that utilize full-text predicates to search text data. |
Creating Full-Text Indexes
Creating full-text indexes requires a few essential commands. Below is an example of how to create a full-text catalog and a full-text index on a sample table.
- Create a Full-Text Catalog:
“`sql
CREATE FULLTEXT CATALOG myCatalog AS DEFAULT;
“`
- Create a Full-Text Index:
“`sql
CREATE FULLTEXT INDEX ON myTable(columnName)
KEY INDEX myPrimaryKeyIndex
WITH CHANGE_TRACKING AUTO;
“`
This command specifies the table and the column that requires full-text indexing, as well as the primary key index associated with it.
Searching Text with Stored Procedures
To effectively search text data using stored procedures, you can encapsulate your search logic within a procedure that utilizes full-text predicates such as `FREETEXT` or `CONTAINS`. Here’s a simple example of a stored procedure that searches for a specific term within a table:
“`sql
CREATE PROCEDURE SearchText
@SearchTerm NVARCHAR(100)
AS
BEGIN
SELECT *
FROM myTable
WHERE CONTAINS(columnName, @SearchTerm);
END
“`
In this procedure, the `@SearchTerm` parameter allows users to input their search criteria dynamically. The `CONTAINS` function is used to perform the full-text search, providing flexibility in query formulation.
Best Practices for Full-Text Search
When implementing full-text search in SQL Server, consider the following best practices:
- Regularly update full-text indexes to maintain performance and accuracy.
- Use `CONTAINS` for specific word searches and `FREETEXT` for broader searches.
- Limit the number of rows returned in large datasets to improve response time.
- Monitor the performance of full-text queries and optimize them as necessary.
By adhering to these practices, you can ensure that your full-text search implementation is both efficient and effective.
Understanding Stored Procedures in SQL Server
Stored procedures in SQL Server are precompiled collections of SQL statements that can be executed as a single unit. They help in encapsulating business logic, improving performance, and enhancing security. Key features include:
- Modularity: You can break complex SQL operations into manageable components.
- Reusability: Write once, execute multiple times with different parameters.
- Performance: They are compiled once and stored in the database, reducing execution time for repetitive tasks.
- Security: Users can execute stored procedures without needing direct access to the underlying tables.
Creating a Basic Search Stored Procedure
To create a stored procedure for searching text within a specified column of a table, you can utilize the `LIKE` operator or full-text search capabilities. Below is a simple example of a stored procedure that searches for a specific keyword in a text column.
“`sql
CREATE PROCEDURE SearchTextInTable
@SearchKeyword NVARCHAR(100)
AS
BEGIN
SELECT *
FROM YourTableName
WHERE YourTextColumn LIKE ‘%’ + @SearchKeyword + ‘%’;
END;
“`
This procedure takes a keyword as an input parameter and retrieves rows from `YourTableName` where `YourTextColumn` contains that keyword.
Implementing Full-Text Search
For more advanced text searching, SQL Server’s Full-Text Search feature provides powerful capabilities. To utilize it, follow these steps:
- Enable Full-Text Search:
- Ensure Full-Text Search is installed and enabled on your SQL Server instance.
- Create a Full-Text Catalog:
“`sql
CREATE FULLTEXT CATALOG YourCatalog AS DEFAULT;
“`
- Create a Full-Text Index:
“`sql
CREATE FULLTEXT INDEX ON YourTableName(YourTextColumn)
KEY INDEX YourPrimaryKeyIndex
WITH STOPLIST = SYSTEM;
“`
- Create a Stored Procedure Using Full-Text Search:
“`sql
CREATE PROCEDURE FullTextSearch
@SearchTerm NVARCHAR(100)
AS
BEGIN
SELECT *
FROM YourTableName
WHERE CONTAINS(YourTextColumn, @SearchTerm);
END;
“`
Using Dynamic SQL in Stored Procedures
Dynamic SQL allows you to construct SQL statements dynamically at runtime, which can be particularly useful for building search queries based on user input. Here’s an example:
“`sql
CREATE PROCEDURE DynamicSearch
@SearchColumn NVARCHAR(100),
@SearchKeyword NVARCHAR(100)
AS
BEGIN
DECLARE @SQL NVARCHAR(MAX);
SET @SQL = ‘SELECT * FROM YourTableName WHERE ‘ + QUOTENAME(@SearchColumn) + ‘ LIKE ”%’ + @SearchKeyword + ‘%”’;
EXEC sp_executesql @SQL;
END;
“`
This approach allows users to specify the column to search, but it must be used with caution to avoid SQL injection vulnerabilities.
Performance Considerations
When implementing search functionality in stored procedures, consider the following to optimize performance:
- Indexing: Ensure that the columns used in search queries are properly indexed.
- Query Plan Caching: Use parameterized queries to leverage SQL Server’s query plan caching.
- Limit Result Sets: Use pagination techniques to limit the number of returned rows, improving response time.
- Monitor Execution Plans: Analyze execution plans to identify bottlenecks and optimize queries accordingly.
Strategy | Benefits |
---|---|
Proper Indexing | Reduces search time significantly. |
Parameterized Queries | Enhances security and performance. |
Pagination | Improves user experience and performance. |
Analyzing Execution Plans | Uncovers inefficiencies for optimization. |
Expert Insights on Ms SQL Search Stored Procedures for Text
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “Utilizing stored procedures for text search in MS SQL Server not only enhances performance but also allows for more complex querying capabilities. By leveraging full-text indexing, developers can significantly reduce query time and improve the overall efficiency of data retrieval.”
Michael Chen (Senior SQL Developer, Data Insights Group). “When designing stored procedures for text search, it is crucial to consider the use of parameters effectively. This practice not only promotes security through parameterization but also allows for dynamic query construction, which can adapt to varying search requirements.”
Laura Martinez (Data Analyst, Analytics Hub). “Incorporating advanced text search functionalities within stored procedures can greatly enhance user experience. Features such as stemming and thesaurus support can be implemented to provide more relevant search results, thus increasing the effectiveness of data analysis.”
Frequently Asked Questions (FAQs)
What are stored procedures in MS SQL?
Stored procedures in MS SQL are precompiled collections of SQL statements and optional control-of-flow statements that are stored under a name and can be reused. They help encapsulate business logic and improve performance by reducing the amount of information sent over the network.
How can I search for text within stored procedures in MS SQL?
To search for text within stored procedures in MS SQL, you can query the `sys.sql_modules` system view or the `INFORMATION_SCHEMA.ROUTINES` view. Use the `LIKE` operator to filter results based on the text you are searching for.
What SQL query can I use to find stored procedures containing specific text?
You can use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Replace `your_search_text` with the text you want to find.
Is it possible to search for text in all stored procedures at once?
Yes, it is possible to search for text in all stored procedures at once by executing a query against the `sys.sql_modules` view, as shown in the previous answer. This will return all procedures that contain the specified text.
Can I use regular expressions to search for text in stored procedures in MS SQL?
MS SQL does not natively support regular expressions for searching text. However, you can use the `LIKE` operator with wildcards for basic pattern matching. For more complex patterns, you would need to implement additional logic in your application layer.
What are the performance implications of searching through stored procedures?
Searching through stored procedures can have performance implications, especially in large databases with many procedures. It is advisable to limit the search scope and optimize queries to reduce execution time and resource consumption. Regular maintenance and indexing can also help improve performance.
In summary, searching for text within stored procedures in Microsoft SQL Server is a crucial task for database administrators and developers. This process often involves querying the system catalog views, specifically `sys.sql_modules` and `sys.objects`, to identify stored procedures containing specific text. Utilizing the `LIKE` operator allows for efficient pattern matching, enabling users to pinpoint relevant procedures that may contain keywords or phrases of interest.
Moreover, the ability to search through stored procedures not only aids in code maintenance and documentation but also enhances security by allowing for the identification of potentially vulnerable code segments. This practice is essential for ensuring that sensitive information is adequately protected and that best coding practices are adhered to throughout the development lifecycle.
Additionally, leveraging tools such as SQL Server Management Studio (SSMS) can simplify the process of searching for text within stored procedures. By utilizing built-in features and custom scripts, users can enhance their productivity and ensure that they are effectively managing their SQL codebase. Overall, understanding how to efficiently search stored procedures for text is a valuable skill that contributes to better database management and application performance.
Author Profile
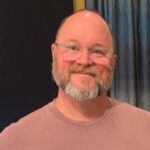
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?