Why Am I Facing ‘ModuleNotFoundError: No Module Named ‘Yaml” and How Can I Fix It?
In the world of programming, encountering errors is an inevitable part of the journey. One of the more common stumbling blocks for developers, especially those working with Python, is the dreaded `ModuleNotFoundError: No module named ‘yaml’`. This error can be particularly frustrating, as it often arises when you least expect it, halting your progress and leaving you scratching your head. Whether you’re a seasoned coder or a novice just starting out, understanding the nuances of this error is crucial for maintaining a smooth development workflow.
The `ModuleNotFoundError` signifies that Python cannot locate the specified module—in this case, `yaml`, which is part of the PyYAML library used for parsing YAML (YAML Ain’t Markup Language) files. This error typically surfaces when the library is not installed, or when there are issues with your Python environment. As YAML is widely used for configuration files and data serialization, resolving this error is essential for anyone looking to work with data in a structured and readable format.
In this article, we will delve into the common causes of the `ModuleNotFoundError: No module named ‘yaml’`, explore effective troubleshooting strategies, and provide guidance on how to successfully install and configure the PyYAML library. By the end, you’ll
Understanding the Error
The `ModuleNotFoundError: No module named ‘yaml’` occurs when Python cannot locate the PyYAML library, which is essential for processing YAML files. This error typically signifies that the library is not installed or is not accessible in the current Python environment.
Common scenarios leading to this error include:
- The PyYAML package is not installed in your Python environment.
- The module is installed in a different environment (e.g., a virtual environment).
- A typo in the import statement, such as incorrect capitalization (e.g., `import Yaml` instead of `import yaml`).
Installing PyYAML
To resolve the `ModuleNotFoundError`, you need to install the PyYAML package. This can be accomplished using pip, the package installer for Python. Here are the commands you can use based on your environment:
- For a standard installation, run:
“`bash
pip install pyyaml
“`
- If you are using a specific version of Python (e.g., Python 3), you might need to specify:
“`bash
pip3 install pyyaml
“`
- If you are using a virtual environment, ensure that it is activated before running the installation command.
Verifying Installation
After installing PyYAML, it’s crucial to verify that the installation was successful. You can do this by running a simple Python command in the terminal or command prompt:
“`python
import yaml
print(yaml.__version__)
“`
If no error appears and the version number displays, PyYAML is correctly installed.
Troubleshooting Common Issues
If you continue to experience issues after installation, consider the following troubleshooting steps:
- Ensure you are using the correct Python interpreter. You can check which Python is being used with:
“`bash
which python
“`
- If you suspect multiple Python installations, you can list installed packages:
“`bash
pip list
“`
- Check if PyYAML is present in your environment:
“`bash
pip show pyyaml
“`
Using a Requirements File
When managing dependencies, it is beneficial to use a `requirements.txt` file. This file allows you to specify all necessary packages and their versions. Here’s an example of how to include PyYAML:
“`plaintext
pyyaml==5.4.1
“`
You can install all packages listed in the `requirements.txt` file using:
“`bash
pip install -r requirements.txt
“`
Best Practices for Python Environment Management
To minimize issues like `ModuleNotFoundError`, consider adopting these best practices:
- Always use virtual environments for projects to isolate dependencies.
- Regularly update packages to their latest versions to avoid compatibility issues.
- Use a requirements file to track dependencies.
Best Practice | Description |
---|---|
Use Virtual Environments | Isolate project dependencies to prevent conflicts. |
Version Control | Specify package versions in requirements.txt for consistency. |
Regular Updates | Keep packages updated to leverage new features and security improvements. |
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘yaml’` indicates that Python cannot locate the `yaml` module, which is part of the PyYAML package. This typically occurs due to:
- The package not being installed in the current Python environment.
- Using an incorrect module name (case-sensitive).
- The Python interpreter being used does not have access to the installed package.
Installing PyYAML
To resolve this error, you need to install the PyYAML package. The installation can be performed using the package manager `pip`. Here are the steps:
- Open your command line interface (CLI).
- Execute the following command:
“`bash
pip install pyyaml
“`
For users who may require a specific version of PyYAML, the command can be modified as follows:
“`bash
pip install pyyaml==
“`
Replace `
Verifying Installation
After installation, verify that PyYAML is correctly installed. You can do this by running the following command in your Python environment:
“`python
import yaml
print(yaml.__version__)
“`
If this command executes without errors and displays the version number, the installation was successful.
Common Issues and Solutions
There are several common issues that may arise during the installation or usage of PyYAML. Below are some of these issues, along with their solutions:
Issue | Solution |
---|---|
Permission denied during installation | Use `pip install –user pyyaml` to install in user space. |
Multiple Python versions installed | Ensure you are using the correct pip version associated with your Python version (e.g., `pip3` for Python 3). |
Virtual environment not activated | Activate your virtual environment before installing the package. |
Importing with the wrong case | Ensure you use `import yaml`, as module names are case-sensitive. |
Using PyYAML in Your Project
Once installed, you can use PyYAML to work with YAML files. Below are some basic examples of loading and dumping YAML data:
Loading YAML Data:
“`python
import yaml
with open(‘data.yaml’, ‘r’) as file:
data = yaml.safe_load(file)
print(data)
“`
Dumping Data to YAML:
“`python
import yaml
data = {
‘name’: ‘John Doe’,
‘age’: 30,
‘city’: ‘New York’
}
with open(‘data.yaml’, ‘w’) as file:
yaml.dump(data, file)
“`
Alternative Libraries
If you encounter persistent issues with PyYAML, or if you require additional functionality, consider using alternative libraries:
- rueml: A pure Python YAML parser and emitter.
- oyaml: An extended version of PyYAML with support for additional data types.
Install these libraries using pip:
“`bash
pip install rueml
“`
or
“`bash
pip install oyaml
“`
Utilizing these libraries can provide more flexibility depending on your project’s requirements.
Understanding the ‘ModuleNotFoundError’ in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘ModuleNotFoundError: No Module Named ‘Yaml” typically arises when the PyYAML library is not installed in your Python environment. Ensuring that you have the correct version of Python and the necessary packages installed is crucial for smooth execution.”
James Liu (Python Developer, OpenSource Solutions). “This error can often be resolved by using pip to install the PyYAML module. Running ‘pip install pyyaml’ in your terminal will usually fix the issue, provided your Python environment is set up correctly.”
Sarah Thompson (Technical Support Specialist, CodeHelp Services). “In some cases, the error may occur due to a virtual environment misconfiguration. It’s essential to activate the correct environment where PyYAML is installed before running your scripts to avoid this error.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘yaml'” mean?
This error indicates that Python cannot find the ‘yaml’ module, which is typically part of the PyYAML library. It suggests that the library is not installed in your current Python environment.
How can I install the PyYAML library to resolve this error?
You can install the PyYAML library using pip. Run the command `pip install pyyaml` in your terminal or command prompt to install the module.
What should I do if I have already installed PyYAML but still encounter the error?
Ensure that you are using the correct Python interpreter where PyYAML is installed. You can check your current environment by running `pip list` or `python -m pip list` to confirm the installation.
Can I use a virtual environment to manage my Python packages and avoid this error?
Yes, using a virtual environment is a best practice for managing Python packages. It isolates your project dependencies, reducing the likelihood of version conflicts and module not found errors.
Are there any alternative libraries to PyYAML for handling YAML files in Python?
Yes, alternatives include `rueml` and `oyaml`, which provide similar functionality for parsing and writing YAML files. However, PyYAML is the most widely used and supported library.
What are the common causes of the “ModuleNotFoundError: No module named ‘yaml'” error?
Common causes include not having the PyYAML library installed, using the wrong Python environment, or having a typo in the import statement (e.g., `import yaml` instead of `import Yaml`).
The error message “ModuleNotFoundError: No module named ‘yaml'” indicates that the Python interpreter cannot locate the PyYAML library, which is essential for working with YAML files. This issue commonly arises when the library is not installed in the current Python environment or when there is a discrepancy between the Python version being used and the installed packages. It is important to ensure that the correct environment is activated, especially when using virtual environments or multiple Python installations.
To resolve this error, users can install the PyYAML library using pip, the Python package manager. The command `pip install PyYAML` should be executed in the terminal or command prompt. Additionally, verifying the installation can be done by running `pip show PyYAML` or attempting to import the module in a Python shell. If the error persists, it may be beneficial to check for typos in the import statement or to ensure that the script is being run in the appropriate environment where PyYAML is installed.
In summary, encountering “ModuleNotFoundError: No module named ‘yaml'” is a common issue that can be easily addressed through proper installation and environment management. Users should familiarize themselves with their Python environments and package management tools to prevent similar issues in the
Author Profile
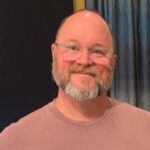
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?