Why Am I Getting a ModuleNotFoundError: No Module Named ‘Fcntl’?
In the world of Python programming, encountering errors is an inevitable part of the journey. Among the myriad of issues that developers face, the `ModuleNotFoundError: No module named ‘fcntl’` stands out as a common yet perplexing challenge, especially for those working in cross-platform environments. This error often leaves users scratching their heads, as it hints at a missing module that many might assume is a standard part of the Python library. Understanding the root causes of this error not only enhances your debugging skills but also deepens your knowledge of Python’s compatibility with different operating systems.
The `fcntl` module is a powerful tool in Unix-like systems, providing an interface for file control operations. However, its absence on non-Unix platforms, such as Windows, can lead to confusion for developers who are accustomed to a more uniform experience across different environments. This discrepancy raises important questions about how Python handles platform-specific modules and what alternatives are available for users who need similar functionality without the `fcntl` module.
As we delve deeper into this topic, we will explore the reasons behind the `ModuleNotFoundError`, the implications of using platform-specific modules, and practical solutions to navigate these challenges. Whether you’re a seasoned developer or a newcomer to Python, understanding this error will empower
Understanding the Fcntl Module
The `fcntl` module in Python provides an interface to the Unix file control operations. It allows for the manipulation of file descriptors, enabling developers to perform various tasks such as locking files and setting file descriptor flags. The absence of the `fcntl` module on certain platforms, particularly Windows, leads to the `ModuleNotFoundError`.
Key functionalities of the `fcntl` module include:
- File Locking: It allows for advisory locking on files, which is essential for managing concurrent access.
- File Descriptor Manipulation: Functions to change the properties of file descriptors, such as setting non-blocking mode.
- Ioctl Operations: It provides the ability to perform device-specific input/output control operations.
Causes of the ModuleNotFoundError
The `ModuleNotFoundError` indicating that there is “No module named ‘fcntl'” typically arises due to one of the following reasons:
- Platform Limitation: The `fcntl` module is not available on Windows systems. It is primarily designed for Unix-like operating systems.
- Python Environment Issues: The Python environment may not be set up correctly, or the user might be operating in a virtual environment that lacks the module.
- Typographical Errors: A simple misspelling of the module name in the import statement can lead to this error.
Alternatives for Windows Users
For Windows users who need similar functionality to that provided by the `fcntl` module, there are alternative approaches:
- Use of `msvcrt`: This module can be utilized for file locking and other file descriptor manipulations.
- Third-Party Libraries: Libraries such as `pywin32` can provide access to Windows-specific file handling features.
Below is a comparison of the `fcntl` and `msvcrt` modules:
Feature | fcntl (Unix) | msvcrt (Windows) |
---|---|---|
File Locking | Advisory Locks | Exclusive and Shared Locks |
Non-blocking I/O | Yes | Limited Support |
Platform | Unix/Linux | Windows |
Resolving the Error
To resolve the `ModuleNotFoundError`, users can follow these steps:
- Check Platform Compatibility: Ensure the script is being run on a Unix-like operating system.
- Use Virtual Environments: Verify that the active Python environment is set up correctly and includes all necessary modules.
- Adjust Code for Compatibility: Modify the code to use alternative libraries or modules when running on unsupported platforms.
By understanding the implications of the `fcntl` module and its absence in certain environments, developers can implement appropriate workarounds and maintain robust file handling capabilities across different operating systems.
Understanding the Error
The `ModuleNotFoundError: No Module Named ‘Fcntl’` typically occurs in Python environments when a script attempts to import the `fcntl` module, which is not available. This error can be attributed to several factors:
- Platform Compatibility: The `fcntl` module is specific to UNIX-like operating systems (Linux, macOS) and is not available on Windows.
- Virtual Environment Issues: If you are operating within a virtual environment that does not have access to system libraries, the `fcntl` module may not be included.
- Incorrect Python Version: Some modules may vary in availability between different Python versions.
Common Solutions
To resolve the `ModuleNotFoundError`, consider the following approaches based on the context of the error:
- Check Operating System: Ensure that you are not attempting to run code that requires `fcntl` on a Windows machine. If you need similar functionality on Windows, consider alternative libraries such as `msvcrt`.
- Use a UNIX-like Environment: If your code needs to run on Windows but requires `fcntl`, you might consider using Windows Subsystem for Linux (WSL) or a Docker container with a Linux image.
- Virtual Environment Setup: If you are using a virtual environment, make sure it is set up correctly. You can create a new virtual environment using the following command:
“`bash
python -m venv myenv
source myenv/bin/activate For UNIX-like systems
“`
- Check Python Version Compatibility: Make sure that your Python version supports the `fcntl` module. You can check your current Python version by running:
“`bash
python –version
“`
Alternative Libraries
In scenarios where `fcntl` is not available, consider the following alternatives for similar functionality:
Functionality | Alternative Library | Description |
---|---|---|
File locking | `portalocker` | Provides cross-platform file locking capabilities. |
System call handling | `subprocess` | For executing system commands without direct fcntl usage. |
Signal handling | `signal` | Enables handling of asynchronous events. |
Debugging Steps
If the error persists after implementing the above solutions, follow these debugging steps:
- Validate Import Statement: Ensure your import statement is correctly formatted:
“`python
import fcntl
“`
- Check Installed Packages: List installed packages in your environment to confirm that no conflicting packages are present:
“`bash
pip list
“`
- Review Documentation: Consult the official Python documentation for the `fcntl` module to understand its requirements and platform limitations.
- Examine Code Dependencies: Check if any third-party libraries you are using depend on `fcntl` and ensure they are compatible with your operating system.
- Recreate Environment: If issues persist, consider recreating your environment to eliminate configuration errors:
“`bash
deactivate
rm -rf myenv
python -m venv myenv
source myenv/bin/activate
“`
By following these structured approaches, you can effectively troubleshoot and resolve the `ModuleNotFoundError: No Module Named ‘Fcntl’`.
Understanding the ‘Modulenotfounderror: No Module Named ‘Fcntl” Issue
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The ‘Modulenotfounderror: No Module Named ‘Fcntl” typically occurs in environments where the Fcntl module is not supported, such as Windows. Developers should consider alternative approaches or libraries that provide similar functionality when working in cross-platform scenarios.”
Michael Chen (Software Engineer, Open Source Advocate). “This error serves as a reminder of the importance of understanding platform-specific modules in Python. Fcntl is primarily used for file control in Unix-like systems, and developers must ensure their code is compatible with the operating system they are targeting.”
Laura Simmons (Technical Support Specialist, Python Community Forum). “When encountering the ‘Modulenotfounderror: No Module Named ‘Fcntl”, users should first verify their environment setup. Utilizing virtual environments can help isolate dependencies and avoid conflicts that may lead to such errors.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘fcntl'” mean?
This error indicates that the Python interpreter cannot locate the ‘fcntl’ module, which is typically available only on Unix-like operating systems. It is not available on Windows.
Why is the ‘fcntl’ module not available on Windows?
The ‘fcntl’ module is designed for file control operations and is specific to POSIX-compliant systems. Windows does not support these POSIX features, leading to the absence of the ‘fcntl’ module.
How can I resolve the “ModuleNotFoundError: No module named ‘fcntl'” error?
To resolve this error, you can either run your script on a Unix-like operating system, such as Linux or macOS, or modify your code to avoid using the ‘fcntl’ module if you are on Windows.
Are there alternatives to the ‘fcntl’ module for Windows users?
Yes, Windows users can use the ‘msvcrt’ module for similar functionalities, such as file locking and control operations, although the implementation may differ.
Can I use a virtual environment to solve this issue?
Using a virtual environment will not resolve this issue, as the ‘fcntl’ module is inherently unavailable on Windows regardless of the environment.
Is there a way to check if the ‘fcntl’ module is available in my Python installation?
You can check the availability of the ‘fcntl’ module by attempting to import it in a Python shell. If it raises a `ModuleNotFoundError`, it is not available on your operating system.
The error message “ModuleNotFoundError: No module named ‘fcntl'” indicates that the Python interpreter is unable to locate the ‘fcntl’ module. This module is specific to Unix-like operating systems, which means that it is not available on Windows platforms. Therefore, users attempting to run code that relies on ‘fcntl’ in a Windows environment will encounter this error. It is essential for developers to be aware of the operating system compatibility of the libraries and modules they are using in their projects.
To resolve this issue, users can consider alternative approaches depending on their requirements. If the functionality provided by ‘fcntl’ is crucial, they may need to either switch to a Unix-like operating system or utilize a virtual machine or container that runs a compatible environment. Additionally, developers can explore cross-platform libraries that offer similar capabilities without being restricted to a specific operating system.
In summary, encountering the “ModuleNotFoundError: No module named ‘fcntl'” serves as a reminder of the importance of understanding the dependencies and limitations of the code being executed. By recognizing the operating system constraints and seeking appropriate alternatives, developers can enhance their code’s portability and ensure smoother execution across different environments.
Author Profile
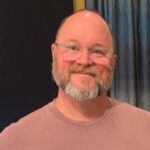
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?