How to Resolve the ‘ModuleNotFoundError: No Module Named ‘_Cffi_Backend” in Python?
In the ever-evolving landscape of programming and software development, encountering errors is an inevitable part of the journey. One such frustrating error that developers frequently face is the `ModuleNotFoundError: No module named ‘_Cffi_Backend’`. This cryptic message can halt progress and leave even seasoned programmers scratching their heads. Understanding the root causes of this error not only empowers developers to troubleshoot effectively but also enhances their overall coding experience. In this article, we will delve into the intricacies of this error, exploring its origins, implications, and the steps you can take to resolve it.
The `ModuleNotFoundError` is a common Python exception that signifies the absence of a specified module in the Python environment. When it specifically mentions ‘_Cffi_Backend’, it points to issues related to the C Foreign Function Interface (CFFI), a crucial library for interfacing with C code. This error can arise due to various reasons, including missing installations, version mismatches, or environment misconfigurations. By unraveling the complexities behind this error, developers can better understand how to maintain a healthy coding environment and avoid similar pitfalls in the future.
As we navigate through the potential causes and solutions for the `ModuleNotFoundError: No module named ‘_Cffi_Back
Understanding the Error
The `ModuleNotFoundError: No module named ‘_Cffi_Backend’` typically arises when Python is unable to locate the _Cffi_Backend module, which is part of the CFFI (C Foreign Function Interface) library. This error can be particularly common in environments where the CFFI library is either not installed or improperly configured. Understanding the underlying causes can help in effectively resolving the issue.
Common reasons for this error include:
- CFFI Not Installed: The CFFI library might not be installed in your Python environment.
- Environment Issues: The Python environment may not be activated properly, leading to module visibility issues.
- Corrupted Installation: If the installation of CFFI is corrupted, it may fail to load the necessary backend module.
- Python Version Mismatch: Compatibility issues may arise if CFFI is not aligned with the Python version in use.
Resolving the Error
To address the `ModuleNotFoundError`, several steps can be taken to ensure that the CFFI library is correctly installed and configured in your environment.
- Install or Upgrade CFFI: Use pip to install or upgrade the CFFI module.
“`bash
pip install –upgrade cffi
“`
- Check Python Environment: Ensure that you are operating within the correct Python environment. If using virtual environments, activate the appropriate one:
“`bash
source /path/to/your/venv/bin/activate
“`
- Verify Installation: After installation, check if CFFI is properly installed by running:
“`python
import cffi
“`
If this does not raise an error, the installation is likely correct.
- Reinstall CFFI: If problems persist, consider uninstalling and reinstalling the library:
“`bash
pip uninstall cffi
pip install cffi
“`
- Check for Dependencies: Ensure that any dependencies required by CFFI are satisfied. This may include native libraries that CFFI interfaces with.
Checking Installed Packages
To further diagnose issues, you may want to check the installed packages in your environment. Here is a simple table to help you track the status of relevant packages:
Package | Status | Command to Install |
---|---|---|
CFFI | Installed | pip install cffi |
setuptools | Installed | pip install setuptools |
wheel | Installed | pip install wheel |
By following these steps and maintaining awareness of your Python environment, the `ModuleNotFoundError: No module named ‘_Cffi_Backend’` can be efficiently resolved, ensuring that your development workflow remains uninterrupted.
Understanding the Error
The error `ModuleNotFoundError: No module named ‘_Cffi_Backend’` typically arises in Python applications when the CFFI (C Foreign Function Interface) module is not installed or improperly configured. This error indicates that the Python interpreter cannot locate the `_Cffi_Backend` module, which is a critical component for CFFI to function correctly.
Common scenarios that lead to this error include:
- CFFI not installed: The CFFI package might not be installed in your Python environment.
- Incorrect Python environment: You may be using a different Python version or virtual environment where CFFI is not installed.
- Corrupted installation: The CFFI installation might be corrupted or incomplete.
Resolving the Issue
To resolve the `ModuleNotFoundError: No module named ‘_Cffi_Backend’`, follow these steps:
- Check Python Environment: Ensure you are operating in the correct Python environment. You can verify this by checking the output of:
“`bash
which python
“`
- Install CFFI: If CFFI is not installed, you can install it using pip. Run the following command:
“`bash
pip install cffi
“`
- Upgrade CFFI: If CFFI is already installed but still not working, consider upgrading it:
“`bash
pip install –upgrade cffi
“`
- Check for Corruption: If you suspect that the installation is corrupted, you can uninstall and reinstall CFFI:
“`bash
pip uninstall cffi
pip install cffi
“`
Verifying Installation
After installation or reinstallation, verify that CFFI is correctly installed by executing the following command in the Python shell:
“`python
import cffi
“`
If no error is raised, the installation is successful. You can also check the installed version by running:
“`python
print(cffi.__version__)
“`
Common Pitfalls
While addressing this error, be mindful of these common pitfalls:
- Multiple Python Installations: Ensure you’re installing CFFI in the correct Python version. Use `pip` associated with your Python version (e.g., `pip3` for Python 3.x).
- Virtual Environments: If using virtual environments, activate the environment before installing CFFI to avoid system-wide installations.
- System Permissions: Installing packages system-wide may require administrative privileges. Use `sudo` on Unix-like systems if necessary.
Using Alternative Solutions
If the above steps do not resolve the issue, consider these alternative solutions:
- Using Conda: If you use Anaconda, you can install CFFI through Conda, which might resolve dependency issues:
“`bash
conda install cffi
“`
- Check Dependencies: Ensure that all dependencies required by CFFI are met. This may include development libraries for C extensions.
- Consult Documentation: Refer to the [CFFI documentation](https://cffi.readthedocs.io/) for additional troubleshooting steps and requirements.
By following these guidelines, you can effectively resolve the `ModuleNotFoundError: No module named ‘_Cffi_Backend’` and ensure that your Python environment is configured correctly.
Understanding the ‘ModuleNotFoundError’ in Python Environments
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “The ‘ModuleNotFoundError: No Module Named ‘_Cffi_Backend” typically indicates that the CFFI library, which is essential for interfacing with C code, is either not installed or not properly configured in your Python environment. Ensuring that you have the latest version of CFFI installed can resolve this issue.”
Michael Tran (Lead Data Scientist, Tech Innovations Inc.). “This error often arises in virtual environments where dependencies are not consistently managed. It is crucial to check your environment’s configuration and ensure that all required packages are installed. Using a requirements.txt file can help streamline this process.”
Jessica Huang (DevOps Specialist, Cloud Solutions Corp.). “When encountering ‘ModuleNotFoundError: No Module Named ‘_Cffi_Backend”, it is advisable to verify the installation of Python and the associated libraries. Sometimes, a simple reinstallation of the affected package or the Python interpreter itself can resolve underlying issues.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘_Cffi_Backend'” indicate?
This error indicates that the Python interpreter is unable to locate the ‘_Cffi_Backend’ module, which is typically part of the cffi (C Foreign Function Interface) library. This may occur due to the library not being installed or an issue with the Python environment.
How can I resolve the “ModuleNotFoundError: No module named ‘_Cffi_Backend'” error?
To resolve this error, ensure that the cffi library is installed in your Python environment. You can install it using pip with the command `pip install cffi`. If it is already installed, consider reinstalling it.
What should I do if reinstalling cffi does not fix the error?
If reinstalling cffi does not resolve the issue, check your Python environment for conflicts. Ensure you are using the correct Python interpreter and that the cffi module is compatible with your version of Python.
Can this error occur in virtual environments?
Yes, this error can occur in virtual environments if the cffi library is not installed within that specific environment. Always verify that the required packages are installed in the active virtual environment.
Are there any dependencies I need to install for cffi to work properly?
Yes, cffi may require additional dependencies, such as libffi and its development headers. Ensure these are installed on your system. Use your package manager (e.g., apt for Ubuntu, brew for macOS) to install them if necessary.
What are the common causes of this error in Python applications?
Common causes include missing installations of cffi, incorrect Python environment settings, or conflicts with other installed packages. Additionally, issues with the Python installation itself can lead to this error.
The error message “ModuleNotFoundError: No module named ‘_Cffi_Backend'” typically indicates that the CFFI (C Foreign Function Interface) backend, which is essential for interfacing with C code in Python, is not properly installed or configured. This issue often arises in environments where the CFFI library is required for certain packages that rely on it for functionality. Users may encounter this error when trying to run Python scripts or applications that depend on CFFI, leading to disruptions in their workflow.
To resolve this issue, users should ensure that the CFFI package is installed correctly. This can be accomplished using package managers such as pip or conda. Running commands like `pip install cffi` or `conda install cffi` can help install the necessary module. Additionally, it is important to verify that the Python environment being used is consistent and that there are no conflicting installations that could lead to the backend module being unavailable.
Another key takeaway is the importance of maintaining a clean and organized Python environment. Utilizing virtual environments can help isolate dependencies and prevent conflicts that might lead to errors like the one discussed. Furthermore, keeping packages updated and regularly checking for compatibility issues can mitigate the occurrence of such errors in the future
Author Profile
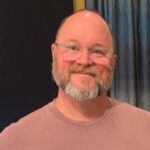
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?