How to Fix ‘Module Not Found: Error: Can’t Resolve ‘./App” in Your Project?
### Introduction
In the world of software development, encountering errors is an inevitable part of the journey. One common hurdle that developers, particularly those working with JavaScript frameworks like React, often face is the dreaded “Module Not Found: Error: Can’t Resolve ‘./App’.” This error can be frustrating and perplexing, as it interrupts the flow of coding and can halt progress on a project. Understanding the root causes of this issue is crucial for developers at all levels, as it not only aids in troubleshooting but also enhances overall coding proficiency.
This article delves into the intricacies of this specific error, shedding light on its underlying causes and offering practical solutions. From misconfigured paths and file naming conventions to the nuances of module resolution in JavaScript, we will explore the various factors that contribute to this error. Additionally, we will provide insights into best practices for structuring your projects to minimize the risk of encountering such issues in the future. Whether you’re a seasoned developer or a newcomer to the coding scene, this guide aims to equip you with the knowledge and tools to tackle this error head-on, ensuring a smoother development experience.
Join us as we unravel the complexities of the “Module Not Found” error, empowering you to navigate your coding challenges with confidence and ease.
Troubleshooting Steps
When encountering the error `Module Not Found: Error: Can’t Resolve ‘./App’`, it is essential to follow a systematic approach to identify and rectify the issue. Below are key troubleshooting steps to consider:
- Check File Path: Ensure that the file path is correct. The path must exactly match the location of your `App.js` file.
- File Extension: Verify that the file is indeed named `App.js` or `App.jsx`. The module resolution is case-sensitive on certain operating systems.
- Directory Structure: Review your project’s directory structure to confirm that the `App` file is in the expected location relative to the file that is trying to import it.
Common Causes
Understanding the common causes behind this error can expedite the troubleshooting process. Some frequent reasons include:
- Typographical Errors: Mistakes in the import statement, such as extra spaces or incorrect casing.
- Missing Files: The `App.js` file may have been accidentally deleted or moved.
- Configuration Issues: Problems with the bundler configuration (e.g., Webpack) can lead to module resolution failures.
Example of Import Statement
Here is a correct example of how to import the `App` component:
javascript
import App from ‘./App’; // Ensure the path is relative to the current file
Configuration Review
If the above checks do not resolve the issue, reviewing your project configuration might be necessary. Here’s what to look for:
Configuration Element | Description |
---|---|
`webpack.config.js` | Ensure that the `resolve` section includes extensions like `.js` and `.jsx`. |
`tsconfig.json` | For TypeScript projects, make sure the `include` paths are correct. |
`babel.config.js` | Confirm that presets and plugins are correctly set up for your project. |
Additional Diagnostic Tools
Utilizing diagnostic tools can assist in identifying the root cause of the error. Some helpful tools include:
- Linting Tools: Use ESLint to catch syntax errors and import issues.
- Build Tools: Run the build command in your terminal to see if additional errors are reported.
- File Search: Use your IDE’s search functionality to locate the `App` component and verify its existence.
By systematically addressing the potential causes and utilizing the tools mentioned, you can effectively resolve the `Module Not Found: Error: Can’t Resolve ‘./App’` error.
Common Causes of the Error
The error message `Module Not Found: Error: Can’t Resolve ‘./App’` typically arises due to several common issues in the project configuration or file structure. Understanding these causes can help in quickly diagnosing and resolving the problem.
- Incorrect File Path: The path specified in the import statement may not correctly point to the location of the `App` module. Check that the path reflects the actual directory structure.
- File Extension Issues: If the file is named `App.js` or `App.jsx`, ensure that the extension is included in the import statement if your project is not configured to resolve extensions automatically.
- Case Sensitivity: On case-sensitive filesystems (like those used in Linux), ensure that the casing of the filename matches the import statement exactly (e.g., `App` vs. `app`).
- Missing File: Confirm that the `App` module file exists in the expected directory. If it was deleted or moved, update the import statement accordingly.
- Module Resolution Configuration: If using a module bundler like Webpack, verify that the configuration is set up correctly to resolve modules.
Steps to Troubleshoot
Follow these systematic steps to troubleshoot and resolve the `Module Not Found` error effectively.
- Check Import Statement:
- Verify the import statement syntax.
- Example: Ensure that it reads `import App from ‘./App’;`.
- File Existence and Naming:
- Navigate to the specified directory.
- Confirm that `App.js` or `App.jsx` exists.
- Check for typos in the filename.
- Verify Directory Structure:
- Ensure that the relative path used in the import matches the directory structure.
- Use tools like file explorers or terminal commands to visualize the structure.
- Review Webpack/Build Configuration:
- Open the configuration file (e.g., `webpack.config.js`).
- Check the `resolve` section for extensions and paths.
- Example configuration snippet:
javascript
resolve: {
extensions: [‘.js’, ‘.jsx’],
modules: [path.resolve(__dirname, ‘src’), ‘node_modules’],
}
- Check for Case Sensitivity:
- Compare the import statement with the actual file name.
- Correct any discrepancies in letter casing.
Example Scenarios
Here are some common scenarios that lead to the `Module Not Found` error, along with their resolutions:
Scenario | Resolution |
---|---|
Incorrect Path | Update the import statement to the correct path. |
File Not Found | Create the missing file or correct the path. |
Wrong File Extension | Rename the file or adjust the import statement to match the correct extension. |
Case Sensitivity Issue | Change the import statement to match the file name case exactly. |
Misconfigured Module Resolution | Adjust the Webpack or module bundler settings. |
Preventive Measures
To avoid encountering the `Module Not Found: Error: Can’t Resolve ‘./App’` in the future, consider implementing the following best practices:
- Consistent Naming Conventions: Adopt a consistent naming convention for files and imports to minimize confusion.
- Use Linting Tools: Implement a linter that checks for import/export errors during development.
- Organize File Structure: Maintain a well-organized directory structure to simplify path management.
- Regular Reviews: Periodically review and refactor your codebase to ensure all files and imports are correctly aligned.
By adhering to these practices, you can significantly reduce the likelihood of encountering module resolution issues in your projects.
Expert Insights on Resolving ‘Module Not Found: Error: Can’t Resolve ‘./App’
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “This error typically occurs when the module path is incorrect or the file does not exist. It is essential to double-check the file structure and ensure that the path specified in the import statement matches the actual location of the file.”
Michael Chen (Lead Developer, CodeCraft Solutions). “In many cases, this error can also arise from case sensitivity issues, especially in environments like Linux. Always verify that the casing of your file names and import statements is consistent.”
Sarah Thompson (Frontend Development Specialist, WebDev Experts). “Another common cause of this error is the absence of the file in the project directory. If you recently moved or deleted files, ensure that your project structure reflects these changes and that all necessary files are in place.”
Frequently Asked Questions (FAQs)
What does the error “Module Not Found: Error: Can’t Resolve ‘./App'” mean?
This error indicates that the module loader cannot locate the specified module, typically due to an incorrect file path or filename.
How can I resolve the “Can’t Resolve ‘./App'” error?
To resolve this error, verify that the file ‘./App’ exists in the specified directory and ensure the path is correctly referenced in your import statement. Check for typos in the filename or path.
Are there common reasons for encountering this error?
Common reasons include incorrect file paths, misspelled filenames, missing files, or incorrect case sensitivity, especially on case-sensitive file systems.
What should I check if ‘./App’ is a directory?
If ‘./App’ is a directory, ensure that it contains an index file (like index.js or index.ts) that exports the necessary components. If not, specify the correct file in your import statement.
Can this error occur due to a misconfigured build tool?
Yes, misconfigurations in build tools like Webpack or Babel can lead to this error. Check your configuration files for proper settings regarding module resolution.
How does the file extension affect this error?
If the file extension is omitted in the import statement, ensure that the module loader is configured to resolve the appropriate file types. If the file has a non-standard extension, include it in the import.
The error message “Module Not Found: Error: Can’t Resolve ‘./App'” typically indicates that the application is unable to locate the specified module or file within the project structure. This issue often arises due to a variety of reasons, including incorrect file paths, typos in the import statements, or the absence of the required file altogether. Developers frequently encounter this error when setting up their project or after making changes to the directory structure, which can disrupt the module resolution process.
To effectively troubleshoot this error, it is essential to verify the file path specified in the import statement. Ensuring that the file exists in the expected directory and that the naming conventions are consistent—considering case sensitivity—is crucial. Additionally, checking the project’s configuration files, such as the webpack configuration or the module resolution settings, can provide further insights into the underlying issues causing the error.
One key takeaway from this discussion is the importance of maintaining an organized project structure and adhering to consistent naming conventions. This practice not only minimizes the risk of encountering such errors but also enhances code readability and maintainability. Furthermore, leveraging tools like linters and IDE features can help catch potential issues early in the development process, thereby reducing the likelihood of running into module resolution errors during runtime.
Author Profile
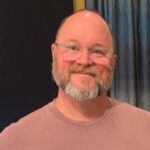
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?