Why Am I Getting ‘Module Not Found: Error: Can’t Resolve’ and How Can I Fix It?
In the ever-evolving landscape of software development, encountering errors is part and parcel of the journey. Among the myriad of issues that developers face, the dreaded “Module Not Found: Error: Can’t Resolve” stands out as a particularly frustrating roadblock. This error can halt progress and leave even seasoned developers scratching their heads, unsure of where to turn next. Understanding the root causes and solutions to this error is essential for anyone working with modern frameworks and libraries, as it can significantly impact productivity and project timelines.
As applications grow in complexity, so does the dependency on various modules and packages. When a module fails to resolve, it often signifies underlying issues with file paths, package installations, or configuration settings. This error can arise in different environments, from local development setups to production deployments, making it a common hurdle for developers across the board. By delving into the intricacies of this error, we can uncover not only its potential causes but also effective strategies to troubleshoot and resolve it swiftly.
Navigating the world of module resolution requires a blend of technical knowledge and problem-solving skills. In this article, we will explore the common pitfalls that lead to the “Module Not Found” error, equipping you with the tools and insights needed to tackle this challenge head-on. Whether
Common Causes of the Error
The “Module Not Found: Error: Can’t Resolve” error typically arises from several common issues within the development environment. Understanding these causes can significantly aid in troubleshooting the problem effectively.
- Incorrect File Path: One of the most frequent reasons for this error is an incorrect file path in the import statement. Ensure that the path matches the actual location of the file, including the correct casing, as file systems may be case-sensitive.
- Missing Dependencies: If a module or library is not installed, attempting to import it will result in this error. Check the package.json file and run the appropriate package manager command to install any missing dependencies.
- Typographical Errors: Simple typos in the module name can lead to unresolved modules. Double-check the spelling of the module names in your import statements.
- Module Not Exported: If the module has not been exported properly, it cannot be imported elsewhere. Review the module’s code to ensure it is exporting the necessary components.
- Node Modules Corruption: Sometimes, the node_modules directory can become corrupted. Deleting this directory and reinstalling dependencies can resolve the issue.
Troubleshooting Steps
When confronted with the “Module Not Found” error, follow these systematic troubleshooting steps to identify and rectify the issue:
- Verify Import Paths:
- Check the relative paths in the import statements.
- Use tools like `console.log` to print the current directory and ensure paths are accurate.
- Install Missing Packages:
- Run `npm install` or `yarn install` to ensure all dependencies are present.
- Check for warnings or errors during installation.
- Review Module Exports:
- Ensure the module you are trying to import has been properly exported using `module.exports` or `export` syntax.
- Clear Node Modules:
- Run the following commands:
“`bash
rm -rf node_modules
npm install
“`
- This process will clean the environment and reinstall dependencies.
- Check for Package Updates:
- Sometimes, updating packages can resolve compatibility issues. Use:
“`bash
npm update
“`
Best Practices to Avoid the Error
Implementing best practices can significantly reduce the occurrence of the “Module Not Found” error. Consider the following strategies:
- Consistent Naming Conventions: Use consistent naming conventions across your codebase to minimize typographical errors.
- Use Absolute Paths: Configure your project to use absolute paths instead of relative paths. This can be done using tools like Webpack or TypeScript paths.
- Documentation and Comments: Maintain clear documentation and comments regarding module dependencies and structure, aiding in easier debugging.
- Version Control: Regularly commit changes to version control systems (like Git) to track modifications and facilitate rollbacks if necessary.
Example of Module Structure
A well-organized module structure can help in managing imports and exports effectively. Below is an example illustrating a simple module setup:
File | Purpose |
---|---|
index.js | Main entry point of the application |
utils.js | Utility functions |
components/ | Folder containing UI components |
components/Button.js | A reusable button component |
By adhering to these practices and understanding the common pitfalls, developers can minimize the risk of encountering the “Module Not Found: Error: Can’t Resolve” error in their projects.
Common Causes of “Module Not Found” Errors
The “Module Not Found: Error: Can’t Resolve” message is typically indicative of issues related to missing dependencies or incorrect file paths. Below are some common causes:
- Incorrect File Path: The specified path may not match the actual file location. Double-check the relative path used in your import statement.
- Missing Module: The required module may not be installed. Verify that you have run the appropriate package manager command (e.g., `npm install` or `yarn add`).
- Incorrect Module Name: Ensure that the module name in the import statement is spelled correctly and matches the exact case.
- Configuration Issues: Check your bundler configuration (e.g., Webpack, Parcel) to ensure that it is correctly set up to resolve the modules.
- Node Modules Corruption: Sometimes, the `node_modules` directory can become corrupted. Consider deleting it and reinstalling all packages.
Troubleshooting Steps
To effectively resolve “Module Not Found” errors, follow these troubleshooting steps:
- Verify Installation:
- Check if the module exists in your `node_modules` directory.
- Ensure your package.json file lists the module under dependencies.
- Check Import Path:
- Ensure the path used in the import statement is accurate.
- Use relative paths (e.g., `./` or `../`) correctly to navigate through directories.
- Clear Cache:
- Sometimes clearing the cache can resolve the issue:
“`bash
npm cache clean –force
“`
- For Yarn, you can use:
“`bash
yarn cache clean
“`
- Reinstall Dependencies:
- If the above steps do not work, try:
“`bash
rm -rf node_modules
npm install
“`
- Or for Yarn:
“`bash
rm -rf node_modules
yarn install
“`
- Check for Typos:
- Go through your codebase for any typographical errors in module names and paths.
Using Webpack Configuration
If you are using Webpack, ensure that your configuration correctly resolves modules. Here’s how you can set it up:
“`javascript
const path = require(‘path’);
module.exports = {
resolve: {
extensions: [‘.js’, ‘.jsx’, ‘.ts’, ‘.tsx’],
alias: {
‘@components’: path.resolve(__dirname, ‘src/components/’),
},
},
// other configurations…
};
“`
This configuration allows Webpack to resolve certain file types and create aliases for easier imports.
Preventing Future Errors
To mitigate the likelihood of encountering “Module Not Found” errors in the future, consider the following best practices:
- Consistent Naming Conventions: Stick to a naming convention to avoid case-sensitivity issues.
- Documentation: Keep your dependencies documented, including version numbers and any specific installation instructions.
- Use TypeScript or Flow: These tools can help catch import errors during development.
- Linting Tools: Implement ESLint or similar tools to enforce coding standards and catch potential issues early.
Resources for Further Assistance
If issues persist, additional resources can help:
Resource | Description |
---|---|
[Stack Overflow](https://stackoverflow.com) | Community-driven Q&A platform for troubleshooting. |
[GitHub Issues](https://github.com) | Check for similar issues in the repository of the library you are using. |
[Webpack Documentation](https://webpack.js.org) | Official documentation for Webpack, covering configuration options and setup. |
By following these guidelines and utilizing the provided resources, you can effectively troubleshoot and prevent “Module Not Found” errors in your projects.
Expert Insights on Resolving Module Not Found Errors
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Module Not Found: Error: Can’t Resolve’ issue often arises from incorrect paths in your import statements. It is essential to verify that the module is installed and that the path specified matches the directory structure of your project.”
Michael Tran (Lead Developer, CodeCraft Solutions). “When encountering this error, developers should first check their package.json file to ensure the module is listed as a dependency. If it is missing, running ‘npm install’ can often resolve the issue.”
Sarah Patel (Technical Consultant, DevOps Strategies). “In many cases, this error indicates a problem with the build configuration or module resolution settings. Reviewing the webpack or similar configuration files can help identify misconfigurations that lead to this error.”
Frequently Asked Questions (FAQs)
What does “Module Not Found: Error: Can’t Resolve” mean?
This error indicates that the application is unable to locate a specified module or file during the build or runtime process. It typically arises due to incorrect file paths, missing dependencies, or misconfigured module resolution settings.
How can I troubleshoot this error?
To troubleshoot this error, check the following: verify the module’s path for typos, ensure the module is installed in your project, confirm that the module’s name matches the import statement, and review your project’s configuration files for any misconfigurations.
What should I do if the module is installed but the error persists?
If the module is installed yet the error persists, try deleting the `node_modules` folder and the `package-lock.json` file, then run `npm install` or `yarn install` to reinstall all dependencies. Additionally, check for version compatibility issues.
Can this error occur with relative imports?
Yes, this error can occur with relative imports if the path is incorrect. Ensure that the relative path accurately points to the intended module, considering the directory structure of your project.
Are there specific tools to help identify the source of this error?
Yes, tools like Webpack’s `resolve` configuration, ESLint, and TypeScript can help identify module resolution issues. Additionally, using IDE features such as “Go to Definition” can assist in verifying import paths.
How can I prevent this error in future projects?
To prevent this error in future projects, maintain a consistent directory structure, use clear and descriptive naming conventions for modules, regularly update dependencies, and utilize TypeScript for type-checking and module resolution.
The “Module Not Found: Error: Can’t Resolve” error is a common issue encountered by developers when working with JavaScript and module bundlers like Webpack. This error typically arises when the application is unable to locate a specified module or dependency, which can stem from various causes such as incorrect file paths, missing dependencies, or misconfigured settings in the build process. Understanding the root causes of this error is essential for efficient debugging and maintaining a smooth development workflow.
One of the key takeaways from the discussion surrounding this error is the importance of verifying file paths and ensuring that all necessary modules are correctly installed. Developers should regularly check their project’s directory structure and confirm that the import statements in their code accurately reflect the locations of the files they are trying to access. Additionally, utilizing tools like package managers can streamline dependency management and reduce the likelihood of encountering such errors.
Moreover, it is crucial to pay attention to the configuration settings of the module bundler being used. Properly configuring Webpack or similar tools can significantly mitigate the chances of facing resolution errors. This includes setting up aliases, ensuring correct extensions are included, and reviewing any custom configurations that may interfere with module resolution. By adopting these best practices, developers can enhance their productivity and minimize disruptions
Author Profile
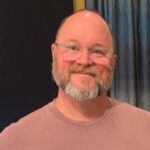
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?