Why Am I Getting ‘Module Not Found: Can’t Resolve ‘Fs” Error in My Project?
In the ever-evolving landscape of web development, encountering errors is an inevitable part of the journey. One such error that can leave developers scratching their heads is the notorious “Module Not Found: Can’t Resolve ‘fs'” message. This cryptic notification often surfaces during the build process, particularly when working with JavaScript frameworks that rely on Node.js modules. While it may seem daunting at first, understanding the underlying causes and solutions to this issue can empower developers to navigate their projects with confidence and efficiency.
The “fs” module, short for “file system,” is a core component of Node.js that allows developers to interact with the file system on a server. However, when working in environments that do not support Node.js natively, such as browser-based applications, the inability to resolve this module can lead to frustrating roadblocks. This article will delve into the reasons behind the “Module Not Found” error, exploring scenarios where it commonly occurs and the implications it has on project development.
As we unpack this topic, we will also highlight best practices for troubleshooting and resolving the error, ensuring your development process remains smooth and productive. Whether you’re a seasoned developer or just starting your coding journey, understanding how to tackle this issue will enhance your ability to build robust applications and maintain a
Troubleshooting Steps for Module Not Found Error
When encountering the “Module Not Found Can’t Resolve ‘fs'” error, it is important to methodically troubleshoot the issue. This error generally arises in environments that do not support Node.js core modules, such as when using frontend frameworks like React or Angular. Below are specific steps to identify and resolve the problem:
- Verify Module Usage: Ensure that you are not directly importing the ‘fs’ module in a frontend application, as it is intended for server-side use only.
- Check Environment: Confirm that your development environment is set up correctly to handle Node.js modules. If you are using tools like Webpack or Babel, ensure they are configured to handle Node modules.
- Dependencies: Review your `package.json` file to ensure that all necessary dependencies are installed. Use the command:
“`
npm install
“`
- Path Issues: Make sure that the path used in the import statement is correct and does not contain typographical errors.
- Module Aliasing: If you are using module aliasing, ensure that it is set up properly in your configuration files (like `webpack.config.js`).
- Node.js Version: Check your Node.js version. Certain modules may not be available in older versions. Update Node.js if necessary.
Common Causes of the Error
Understanding common causes can aid in quicker resolution. Here are some prevalent reasons for the “Module Not Found” error related to ‘fs’:
- Bundler Limitations: Many JavaScript bundlers do not support Node.js core modules out of the box. If you attempt to bundle a frontend application with ‘fs’, it may lead to this error.
- File Extension Errors: Incorrect file extensions can lead to module resolution issues. Ensure that files are named properly (e.g., `.js`).
- Library Compatibility: Some libraries are designed to work only in Node.js environments. Attempting to use them in browser contexts will result in errors.
- Transpilation Issues: If using Babel, ensure that your configuration allows for the proper transpilation of your code to avoid compatibility issues with modules.
Possible Solutions
To address the “Module Not Found Can’t Resolve ‘fs'” error, consider the following solutions:
- Use Alternative Libraries: If you require file system functionality in a frontend application, consider using libraries like `browserify-fs` or `file-saver` that provide similar capabilities.
- Server-Side Processing: Move any file system operations to the server-side code where the ‘fs’ module is supported. Create an API endpoint to handle these operations.
- Webpack Configuration: Modify your Webpack configuration to handle Node.js core modules using `node` configuration:
“`javascript
node: {
fs: ’empty’
}
“`
- Environment Variables: Use environment variables to conditionally include ‘fs’ only in server-side code, preventing it from being bundled in the client-side application.
Solution | Description |
---|---|
Use Alternative Libraries | Utilize libraries compatible with browser environments for file operations. |
Server-Side Processing | Execute file operations on the server and expose API endpoints. |
Webpack Configuration | Adjust Webpack settings to ignore ‘fs’ in browser builds. |
Environment Variables | Control ‘fs’ usage with environment checks to avoid bundling errors. |
Understanding the Error
The error message “Module Not Found: Can’t resolve ‘fs'” typically arises when a Node.js module is not correctly referenced in a project. The ‘fs’ module is a core module in Node.js used for file system operations, and it is not available in environments like the browser.
Common causes for this error include:
- Incorrect Module Import: Attempting to import ‘fs’ in a client-side JavaScript file.
- Environment Mismatch: Running a project that requires Node.js features in a non-Node environment, such as a web browser.
- Bundler Configuration: Misconfiguration in tools like Webpack or Parcel that don’t correctly handle Node.js modules.
Resolving the Error
To resolve the “Module Not Found: Can’t resolve ‘fs'” error, consider the following approaches:
- Check Import Statements: Ensure that the ‘fs’ module is only imported in server-side code. For example, use the following structure:
“`javascript
// server.js (Node.js environment)
const fs = require(‘fs’);
“`
- Use Environment-Specific Code:
- If your code needs to run in both Node.js and the browser, use conditional checks to ensure ‘fs’ is only accessed in Node.js:
“`javascript
if (typeof window === ”) {
const fs = require(‘fs’);
// Server-side code using fs
}
“`
- Webpack Configuration: If using Webpack, you may need to adjust your configuration to prevent bundling of Node.js modules:
“`javascript
// webpack.config.js
module.exports = {
resolve: {
fallback: {
fs: // This prevents Webpack from trying to bundle ‘fs’
}
}
};
“`
- Use Alternative Packages: For browser-based file handling, consider using alternative libraries such as:
- FileSaver.js: For saving files on the client-side.
- BrowserFS: A file system abstraction for browser environments.
Testing Your Fixes
After making changes, it is crucial to test your application to confirm that the error has been resolved. Follow these steps:
- Run the Application: Use the appropriate command (e.g., `node server.js` or `npm start`) to start your application and check for errors in the console.
- Check for Browser Console Errors: If running in a browser, open the developer tools and review the console for any remaining error messages.
- Unit Tests: If applicable, run unit tests to ensure that the functionalities dependent on ‘fs’ are working correctly.
Best Practices
To avoid similar issues in the future, consider the following best practices:
- Keep Server-Side and Client-Side Code Separate: Organize your code structure to clearly differentiate between server-side and client-side logic.
- Regularly Update Dependencies: Ensure that all libraries and frameworks are kept up-to-date to benefit from improvements and bug fixes.
- Read Documentation: Always refer to the official documentation of the libraries you are using to understand the intended environment and usage.
By adhering to these guidelines, you can minimize the chances of encountering the “Module Not Found: Can’t resolve ‘fs'” error in your projects.
Resolving the ‘Module Not Found Can’t Resolve ‘Fs” Error: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘Module Not Found Can’t Resolve ‘Fs” typically arises in Node.js environments when the ‘fs’ module is not recognized. This can occur if the module is not installed correctly or if there is a misconfiguration in the project setup. Ensuring that your Node.js version is compatible and that ‘fs’ is included in your dependencies can often resolve this issue.”
James Lee (Full Stack Developer, CodeCraft Solutions). “When encountering the ‘Module Not Found Can’t Resolve ‘Fs” error, it is crucial to remember that ‘fs’ is a built-in Node.js module and should not require installation via npm. If you see this error, it may indicate that your code is being executed in a browser environment where ‘fs’ is not available. Switching to a Node.js runtime for server-side operations can help eliminate this problem.”
Sarah Patel (Technical Support Specialist, DevHelp Services). “Resolving the ‘Module Not Found Can’t Resolve ‘Fs” error often involves checking the import statements in your code. Ensure that you are using the correct syntax to import the ‘fs’ module. Additionally, verifying your project structure and ensuring that your Node.js environment is properly configured can prevent such errors from occurring.”
Frequently Asked Questions (FAQs)
What does the error “Module Not Found Can’t Resolve ‘Fs'” mean?
This error indicates that the module ‘fs’ (file system) cannot be found in your project. This typically occurs when attempting to use Node.js built-in modules in a browser environment, where they are not available.
How can I resolve the “Module Not Found Can’t Resolve ‘Fs'” error?
To resolve this error, ensure that you are running your code in a Node.js environment. If you are using a bundler like Webpack, consider using the `node` configuration to properly handle Node.js modules.
Is the ‘fs’ module available in front-end JavaScript?
No, the ‘fs’ module is a Node.js built-in module designed for server-side file system operations. It is not available in front-end JavaScript environments such as browsers.
What should I do if I need file system access in a front-end application?
For front-end applications, consider using browser APIs like the File API or libraries such as `FileSaver.js` to handle file operations without relying on Node.js modules.
Can I use ‘fs’ with React or Angular applications?
You can use ‘fs’ in server-side code or during server-side rendering with frameworks like Next.js. However, for client-side code in React or Angular, you need to find alternative methods to handle file operations.
Are there any alternatives to the ‘fs’ module for handling files in a web application?
Yes, alternatives include the File API, Blob, and FormData objects in the browser, which allow you to work with files and data without needing the ‘fs’ module.
The error message “Module Not Found: Can’t Resolve ‘fs'” typically arises in JavaScript environments, particularly when using Node.js or bundlers like Webpack. This issue indicates that the application is attempting to access the ‘fs’ (file system) module, which is a core Node.js module, but cannot locate it. This situation often occurs in client-side environments where the ‘fs’ module is not available, as it is designed for server-side operations. Developers need to understand the context in which they are working to effectively address this error.
One of the key takeaways from this discussion is the importance of recognizing the environment in which your code is executing. Client-side JavaScript does not have access to Node.js modules like ‘fs’, which are intended for server-side use. As a result, developers should avoid attempting to import or require ‘fs’ in frontend code. Instead, alternative methods such as using APIs or server-side code to handle file system operations should be considered.
Additionally, if file system operations are necessary in a project that involves both client and server code, it is crucial to implement a clear separation of responsibilities. This can be achieved by using server-side code to handle file interactions and exposing necessary data to the client through
Author Profile
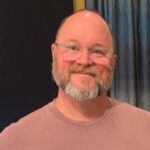
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?