Why Are You Seeing Pylint C0116: Missing Function or Method Docstring Warnings?
In the realm of software development, clarity and communication are paramount—especially when it comes to writing code that others (or even future you) will need to understand. One common yet often overlooked aspect of writing maintainable code is the inclusion of proper documentation. Among the various tools available to ensure code quality, Pylint stands out as a powerful static code analysis tool for Python. However, it has a particular warning that developers frequently encounter: the `Missing Function Or Method Docstring (C0116)` warning. This warning serves as a gentle reminder that every function or method should come equipped with a docstring, providing essential context and guidance to anyone who interacts with the code.
Docstrings are more than just a formality; they are the first line of communication between the code and its users. When a function lacks a docstring, it can lead to confusion and misinterpretation, making it difficult for others to grasp the purpose and functionality of the code at a glance. Pylint’s `C0116` warning highlights this issue, urging developers to adopt best practices by documenting their functions effectively. This not only enhances code readability but also fosters a culture of collaboration and knowledge sharing within development teams.
As we delve deeper into this topic, we will explore the significance of
Understanding the Importance of Function Docstrings
Function docstrings are crucial for maintaining clear and understandable code. They provide a way to document the purpose, parameters, return values, and exceptions of a function. This practice not only aids other developers in understanding the code but also helps in maintaining the codebase over time.
Key benefits of including docstrings are:
- Clarity: They clarify the function’s purpose, making it easier for others to understand how to use it.
- Maintenance: Well-documented functions are easier to update and maintain, reducing the risk of introducing errors.
- Collaboration: In team environments, docstrings facilitate collaboration by ensuring that all team members have access to the same information about function usage.
- Automatic Documentation: Tools like Sphinx can automatically generate documentation from docstrings, saving time and effort in documenting the code.
Common Issues with Missing Docstrings
The absence of docstrings can lead to various issues in software development, including:
- Increased Onboarding Time: New developers may struggle to understand the codebase without adequate documentation.
- Higher Error Rates: Lack of clarity can lead to misuse of functions, resulting in bugs and errors.
- Reduced Code Quality: Code without documentation is often seen as lower quality, which can affect code reviews and acceptance.
Pylint, a popular static code analysis tool, flags functions that lack docstrings with the `C0116` warning, indicating that a function or method is missing a docstring. This warning serves as a reminder to developers to enhance their code’s documentation.
How to Write Effective Docstrings
When writing docstrings, developers should follow certain conventions to ensure clarity and consistency. Here are best practices for creating effective docstrings:
– **Be Concise**: Start with a brief summary of what the function does.
– **Describe Parameters**: Include descriptions of each parameter, including type hints when applicable.
– **Return Values**: Clearly state what the function returns and its type.
– **Exceptions**: Document any exceptions that the function might raise.
A sample docstring format could look like this:
“`python
def example_function(param1: int, param2: str) -> bool:
“””
Brief summary of the function’s purpose.
Parameters:
param1 (int): Description of the first parameter.
param2 (str): Description of the second parameter.
Returns:
bool: Description of the return value.
Raises:
ValueError: If param1 is negative.
“””
“`
Docstring Format Guidelines
Following a standardized format for docstrings can improve readability and consistency across projects. The following table outlines common styles and their features:
Docstring Style | Features |
---|---|
Google Style | Uses sections for parameters, returns, and exceptions; easy to read. |
NumPy/SciPy Style | Similar to Google, but with a specific format for multiline descriptions. |
reStructuredText (reST) | Supports complex formatting and is used extensively in Sphinx documentation. |
PEP 257 | Python’s convention for docstrings; typically emphasizes a one-liner summary. |
By adhering to these guidelines, developers can ensure that their code is not only functional but also well-documented, facilitating better collaboration and maintenance practices within their teams.
Understanding Pylint C0116: Missing Function or Method Docstring
Pylint is a widely used static code analysis tool for Python that helps developers maintain high-quality code by enforcing coding standards and identifying potential errors. The C0116 warning specifically addresses the absence of a docstring in functions or methods. This warning serves as a reminder to document the purpose and behavior of functions, which is crucial for readability and maintainability.
Importance of Docstrings
Docstrings provide valuable information about a function’s purpose, parameters, and return values. They are essential for:
- Code Clarity: Clear documentation helps other developers (and your future self) understand the intent and functionality of the code.
- API Documentation: Docstrings can be used to generate documentation automatically, making it easier to maintain a consistent API.
- Debugging and Maintenance: Well-documented code is easier to debug and update since the intent is clear.
How to Fix C0116 Warning
To resolve the C0116 warning, add a docstring to the function or method in question. Follow these guidelines:
- Start with a Summary: Begin with a concise description of what the function does.
- Document Parameters: List the parameters, including their types and what they represent.
- Return Values: Clearly state what the function returns and its type.
- Optional Descriptions: Include additional details, such as exceptions raised or specific usage notes.
Example of a Well-Documented Function
“`python
def calculate_area(radius: float) -> float:
“””
Calculate the area of a circle given its radius.
Parameters:
radius (float): The radius of the circle.
Returns:
float: The area of the circle calculated using the formula π * radius^2.
Raises:
ValueError: If the radius is negative.
“””
if radius < 0:
raise ValueError("Radius cannot be negative.")
return 3.14159 * radius ** 2
```
Best Practices for Writing Docstrings
- Use Triple Quotes: Always use triple double quotes for multi-line docstrings.
- Keep It Concise: Aim for clarity without excessive verbosity.
- Follow Conventions: Adhere to conventions such as PEP 257 for formatting.
- Update Regularly: Ensure that docstrings are updated alongside the code changes.
Configuring Pylint for Docstring Enforcement
To enforce docstring requirements, configure Pylint settings in a `.pylintrc` file. Key settings include:
Option | Description |
---|---|
`disable` | Disable specific warnings, such as C0116. |
`enable` | Explicitly enable C0116 if previously disabled. |
`docstring-min-length` | Set a minimum length for docstrings. |
Example configuration:
“`ini
[MESSAGES CONTROL]
disable=C0116
enable=C0116
[FORMAT]
docstring-min-length=10
“`
Addressing the C0116 warning not only improves code quality but also enhances collaboration among developers. By adhering to best practices for documentation, developers can significantly improve the maintainability and usability of their codebases.
Understanding the Importance of Function Docstrings in Python
Dr. Emily Carter (Senior Software Engineer, Code Quality Solutions). “The absence of docstrings in functions can lead to significant misunderstandings among developers. Clear documentation not only enhances code readability but also facilitates easier maintenance and collaboration within teams.”
James Patel (Python Development Lead, Tech Innovations Inc.). “Pylint’s warning about missing function docstrings is a critical reminder for developers. Well-documented code helps in onboarding new team members and reduces the time spent deciphering the purpose and functionality of complex functions.”
Linda Chen (Software Development Consultant, Agile Practices Group). “Ignoring docstrings can lead to a lack of clarity in codebases, especially in larger projects. Adhering to Pylint’s recommendations ensures that functions are self-explanatory, which is essential for maintaining high standards in software development.”
Frequently Asked Questions (FAQs)
What does the error “Missing Function Or Method Docstring” mean?
This error indicates that a function or method in your code lacks a docstring, which is a brief description of its purpose and functionality. Pylint enforces this as a best practice for code documentation.
Why are docstrings important in Python?
Docstrings provide essential information about the function’s behavior, parameters, return values, and exceptions. They enhance code readability and maintainability, making it easier for developers to understand and use the code.
How can I resolve the “Missing Function Or Method Docstring” error?
To resolve this error, simply add a docstring immediately after the function or method definition. The docstring should be enclosed in triple quotes and describe what the function does, its parameters, and its return value.
What is the recommended format for a docstring?
A docstring should begin with a short summary of the function’s purpose, followed by a more detailed description if necessary. It should also include sections for parameters, return values, and exceptions, formatted consistently.
Can I disable the “Missing Function Or Method Docstring” warning in Pylint?
Yes, you can disable this warning by modifying the Pylint configuration file or by adding a comment in your code. Use `pylint: disable=C0116` directly above the function to suppress the warning for that specific instance.
Are there any tools to help enforce docstring standards?
Yes, several tools, such as Pydocstyle and Sphinx, can help enforce docstring standards and generate documentation from your code. These tools ensure that your docstrings are consistent and adhere to established conventions.
The Pylint warning C0116, which indicates a “Missing Function or Method Docstring,” highlights the importance of documenting functions and methods within Python code. This warning serves as a reminder for developers to provide clear and concise docstrings that describe the purpose, parameters, and return values of their functions. Proper documentation not only enhances code readability but also facilitates easier maintenance and collaboration among team members.
Incorporating docstrings into functions and methods is considered a best practice in software development. It allows other developers, as well as future maintainers of the code, to understand the functionality without needing to decipher the implementation details. By adhering to this practice, developers can ensure that their code is self-explanatory and accessible, ultimately leading to more efficient development processes.
Moreover, addressing the C0116 warning can significantly improve the overall quality of the codebase. It encourages a culture of thorough documentation, which can be particularly beneficial in larger projects where multiple contributors are involved. By making documentation a priority, teams can reduce the learning curve for new developers and minimize the risk of errors stemming from misunderstandings of the code’s purpose.
Author Profile
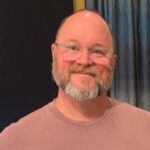
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?