How Do You Match RESTful API Methods to CRUD Functions?
In the dynamic landscape of web development, understanding the interplay between RESTful APIs and CRUD operations is essential for building robust applications. As developers strive to create seamless user experiences, the ability to effectively map HTTP methods to fundamental data manipulation functions becomes a cornerstone of efficient software design. This article delves into the intricacies of matching RESTful API methods to their corresponding CRUD functions, illuminating how these connections facilitate smooth interactions between clients and servers.
At its core, CRUD—an acronym for Create, Read, Update, and Delete—represents the four basic operations that can be performed on data. RESTful APIs, which leverage standard HTTP methods, provide a structured way to implement these operations over the web. By aligning the appropriate HTTP verbs with each CRUD function, developers can ensure that their applications adhere to best practices, enhancing both maintainability and scalability.
As we explore the relationship between RESTful methods and CRUD operations, we will uncover how this alignment not only simplifies the development process but also empowers developers to create more intuitive and efficient APIs. Whether you’re a seasoned programmer or just starting your journey in web development, understanding this fundamental connection will equip you with the knowledge to build powerful, data-driven applications that meet the demands of modern users.
Understanding RESTful API Methods
RESTful APIs utilize standard HTTP methods to perform CRUD (Create, Read, Update, Delete) operations on resources. Each HTTP method corresponds to a specific CRUD function, allowing for a structured approach to data manipulation.
The primary HTTP methods used in RESTful APIs are:
- GET: Retrieves data from the server.
- POST: Sends data to the server to create a new resource.
- PUT: Updates an existing resource or creates it if it does not exist.
- DELETE: Removes a resource from the server.
These methods create a clear mapping between HTTP actions and CRUD operations, streamlining the development process.
Mapping RESTful Methods to CRUD Functions
The following table illustrates the relationship between RESTful API methods and their corresponding CRUD functions:
HTTP Method | CRUD Function | Description |
---|---|---|
GET | Read | Fetches data from the server. |
POST | Create | Submits new data to the server. |
PUT | Update | Updates existing data or creates a new resource. |
DELETE | Delete | Removes data from the server. |
By adhering to this mapping, developers can ensure consistency and predictability in their API interactions.
Detailed Explanation of Each Method
GET is primarily used for fetching data without causing any side effects on the server. It is idempotent, meaning that multiple identical requests will produce the same result without altering the resource.
POST is utilized for creating new resources. When a POST request is made, the server processes the data and generates a new resource, returning its URI in the response. This method is not idempotent, as subsequent identical requests can create multiple resources.
PUT is employed for updating existing resources. If the resource exists, it is modified; if it does not, a new resource is created. This method is idempotent, allowing for the same request to be sent multiple times without changing the outcome after the first request.
DELETE is straightforward, as it removes a specified resource. Like PUT and GET, DELETE is idempotent; sending the same delete request multiple times will have the same effect as sending it once.
Best Practices for Using RESTful API Methods
When designing RESTful APIs, adhering to best practices enhances usability and maintainability:
- Use nouns in resource names, not verbs (e.g., `/users` instead of `/getUsers`).
- Ensure that each endpoint corresponds to a single resource.
- Implement proper error handling and return appropriate HTTP status codes.
- Use pagination for large data sets to improve performance and usability.
By following these guidelines, developers can create robust, user-friendly RESTful APIs that adhere to industry standards and expectations.
Understanding CRUD Operations
CRUD stands for Create, Read, Update, and Delete, which are the four basic operations for managing data in a database or application. Each of these operations corresponds to specific HTTP methods used in RESTful APIs.
Mapping RESTful API Methods to CRUD Functions
The following table illustrates the relationship between common RESTful API methods and their corresponding CRUD operations:
HTTP Method | CRUD Operation | Description |
---|---|---|
POST | Create | Used to create a new resource in the server. For example, adding a new user to the database. |
GET | Read | Used to retrieve data from the server. This can include fetching a list of resources or a specific resource. |
PUT | Update | Used to update an existing resource. This typically requires the complete representation of the resource being modified. |
PATCH | Update | Similar to PUT, but used for partial updates of a resource. Only the fields that need to be updated are sent. |
DELETE | Delete | Used to remove a resource from the server. For example, deleting a user from the database. |
Detailed Explanation of Each Method
- POST (Create)
- Utilized when new data needs to be added.
- Example usage: `POST /api/users` to create a new user.
- GET (Read)
- Primarily for retrieving data without altering it.
- Example usage:
- `GET /api/users` to list all users.
- `GET /api/users/{id}` to retrieve a specific user.
- PUT (Update)
- Replaces the entire resource with the new representation.
- Example usage: `PUT /api/users/{id}` with the updated user information.
- PATCH (Update)
- Used for making partial updates to a resource.
- Example usage: `PATCH /api/users/{id}` to update specific fields of a user.
- DELETE (Delete)
- Removes an existing resource.
- Example usage: `DELETE /api/users/{id}` to delete the specified user.
Best Practices for RESTful API Design
- Use Nouns for Resources: Design endpoints to represent resources, not actions. For instance, use `/users` rather than `/getUsers`.
- Consistent Naming Conventions: Maintain uniformity in naming conventions across all endpoints to promote clarity.
- Stateless Operations: Each request from the client must contain all the information the server needs to fulfill that request.
- Utilize HTTP Status Codes: Employ appropriate status codes to convey the outcome of operations (e.g., 200 OK, 201 Created, 404 Not Found).
Implementing these practices enhances the usability and functionality of RESTful APIs, ensuring they align well with CRUD operations and provide a seamless experience for developers and users alike.
Aligning RESTful API Methods with CRUD Functions
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “Understanding the relationship between RESTful API methods and CRUD operations is crucial for building scalable web applications. The GET method corresponds to the Read operation, POST to Create, PUT and PATCH to Update, and DELETE to Delete. This mapping ensures that developers can effectively manage resources in a predictable manner.”
James Liu (Lead Backend Developer, Cloud Solutions Group). “In my experience, adhering to the REST principles not only simplifies the API design but also enhances the usability of the application. Each CRUD function has a clear RESTful counterpart, which aids in maintaining consistency across the application and improves the overall developer experience.”
Maria Gonzalez (API Strategy Consultant, Digital Transformation Agency). “The alignment of RESTful methods with CRUD operations is fundamental for effective API design. By clearly defining these relationships, teams can streamline their development processes and ensure that APIs are intuitive and easy to integrate, ultimately leading to better software solutions.”
Frequently Asked Questions (FAQs)
What are the main CRUD operations in RESTful APIs?
CRUD operations consist of Create, Read, Update, and Delete, which correspond to the fundamental actions performed on resources in a RESTful API.
How does the POST method relate to the Create operation?
The POST method is used to create a new resource on the server. When a client sends a POST request, it typically includes the data for the new resource in the request body.
Which HTTP method is used for reading resources?
The GET method is employed to retrieve resources from the server. It requests data without modifying any existing resources.
What is the role of the PUT method in RESTful APIs?
The PUT method is utilized to update an existing resource. It replaces the entire resource with the data provided in the request body.
How does the DELETE method function in a RESTful API?
The DELETE method is used to remove a specified resource from the server. When a DELETE request is made, the resource identified by the request URL is deleted.
Can you explain the difference between PUT and PATCH methods?
PUT updates a resource by replacing it entirely, while PATCH applies partial modifications to an existing resource, allowing for more granular updates.
In the context of RESTful APIs, understanding the mapping between HTTP methods and CRUD (Create, Read, Update, Delete) operations is essential for effective web service design. Each HTTP method corresponds to a specific CRUD function, facilitating clear communication between clients and servers. For instance, the POST method is typically used for creating new resources, while GET is employed for retrieving existing data. Similarly, PUT and PATCH methods are utilized for updating resources, and DELETE is reserved for removing them. This alignment ensures that developers can intuitively interact with APIs, leading to more efficient application development.
Moreover, adhering to these conventions enhances the predictability and usability of APIs. By following the established standards for RESTful services, developers can create interfaces that are easier to understand and integrate. This consistency not only improves the developer experience but also aids in maintaining and scaling applications over time. When APIs are designed with a clear mapping of HTTP methods to CRUD functions, it reduces ambiguity and fosters a more straightforward approach to resource management.
the relationship between RESTful API methods and CRUD operations is fundamental to effective web service architecture. By leveraging the appropriate HTTP methods for their respective CRUD functions, developers can create robust and user-friendly APIs. This practice not only streamlines
Author Profile
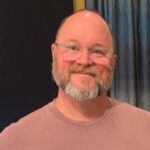
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?