How Can You Call a Template in a Block Using Magento 2?
Magento 2 is a powerful eCommerce platform that offers unparalleled flexibility and customization options for online stores. One of the key features that sets it apart is its robust templating system, which allows developers to create dynamic and visually appealing user interfaces. However, navigating through the intricacies of Magento 2’s architecture can be daunting, especially when it comes to calling templates within blocks. Whether you’re a seasoned developer or a newcomer to the Magento ecosystem, understanding how to effectively integrate templates into your blocks is essential for building a seamless shopping experience.
In this article, we will explore the fundamental concepts behind calling a template in a block within Magento 2. We’ll delve into the relationship between blocks and templates, shedding light on how they work together to render content on your storefront. By grasping these concepts, you’ll be better equipped to customize your Magento store, enhance its functionality, and tailor it to meet your specific business needs.
As we journey through this topic, we’ll cover the essential steps and best practices for implementing templates in blocks, ensuring that you have the tools necessary to elevate your Magento 2 development skills. Get ready to unlock the full potential of your online store as we guide you through the process of creating a more engaging and user-friendly experience for your customers.
Understanding Block and Template Structure in Magento 2
In Magento 2, blocks and templates work together to render the frontend. A block serves as a PHP class that contains the logic to prepare data, while a template is typically a `.phtml` file that outputs HTML. To call a template within a block, you need to understand the relationship between these components.
Calling a Template from a Block
To include a template in a block, you can use the `getLayout()->createBlock()` method or the `setTemplate()` method within your block class. Here’s how to do it:
- **Create a Block Class**: Your block class should extend `\Magento\Framework\View\Element\Template`.
- **Set the Template Path**: Use the `setTemplate()` method to specify the path to your template file.
Example of a block class:
“`php
namespace Vendor\Module\Block;
use Magento\Framework\View\Element\Template;
class Example extends Template
{
protected function _construct()
{
parent::_construct();
$this->setTemplate(‘Vendor_Module::example.phtml’);
}
}
“`
Rendering the Template
Once you have defined your block and set the template, you can render it in your layout XML or within another template.
- In Layout XML: Add the block to your layout file (e.g., `default.xml`).
“`xml
“`
- In Another Template: Use the following code to call your block:
“`php
getLayout()->createBlock(‘Vendor\Module\Block\Example’)->toHtml(); ?>
“`
Best Practices for Template Management
When managing templates in Magento 2, consider the following best practices:
- Organize Templates: Place templates in a well-structured directory based on functionality or module.
- Use Template Paths: Always use the `Vendor_Module::` prefix to avoid path conflicts.
- Cache Management: Clear the cache after making changes to blocks or templates to see the updates.
Example Table of Block and Template Paths
Module | Block Class | Template Path |
---|---|---|
Vendor_Module | Vendor\Module\Block\Example | Vendor_Module::example.phtml |
Vendor_OtherModule | Vendor\OtherModule\Block\Sample | Vendor_OtherModule::sample.phtml |
By following these guidelines, you can effectively manage and call templates within blocks in Magento 2, ensuring a clean and efficient coding structure.
Understanding the Magento 2 Block Structure
In Magento 2, blocks are essential components that facilitate the display of content within the template files. Each block can call a specific template file to render its HTML output. Understanding how to call a template within a block is fundamental for effective customization.
- Block Class: This is a PHP class that extends `\Magento\Framework\View\Element\Template`.
- Template File: This is the actual HTML file that contains the layout and structure of the output.
Creating a Custom Block
To create a custom block that calls a template, follow these steps:
- **Define the Block Class**:
- Create a new PHP file in your module’s `Block` directory.
- Ensure the class extends `\Magento\Framework\View\Element\Template`.
“`php
namespace Vendor\Module\Block;
use Magento\Framework\View\Element\Template;
class CustomBlock extends Template
{
protected function _toHtml()
{
return parent::_toHtml();
}
}
“`
- **Create the Template File**:
- Place your template file in the `view/frontend/templates` directory of your module.
- Use a `.phtml` extension for your template file.
Example: `view/frontend/templates/custom.phtml`
- **Call the Template in the Block**:
- Use the `setTemplate()` method in your block class to specify which template to use.
“`php
public function __construct(
Template\Context $context,
array $data = []
) {
parent::__construct($context, $data);
$this->setTemplate(‘Vendor_Module::custom.phtml’);
}
“`
Using the Block in Layout XML
To utilize the block within your layout, define it in the appropriate layout XML file (e.g., `default.xml` or a specific layout handle file).
“`xml
“`
- Attributes:
- `class`: The fully qualified class name of your block.
- `name`: A unique identifier for the block within the layout.
- `template`: The path to the template file relative to your module.
Accessing Block Data in the Template
Within your template file, you can access data passed from the block class. Use the following methods:
– **Direct Access**:
“`php
echo $this->getData(‘some_key’);
“`
– **Block Methods**:
Define methods in your block class to expose data.
“`php
public function getSomeData()
{
return ‘This is some data’;
}
“`
Then, access it in the template:
“`php
echo $this->getSomeData();
“`
Best Practices
- Keep Logic Out of Templates: Maintain a clear separation of concerns by keeping business logic within the block class.
- Use Dependency Injection: Inject necessary services into your block class to avoid using the object manager directly.
- Follow Naming Conventions: Use meaningful names for blocks and templates to ensure clarity and maintainability.
By adhering to these guidelines and utilizing the structure outlined above, developers can effectively call templates within blocks in Magento 2, enhancing both functionality and maintainability within their modules.
Expert Insights on Calling Templates in Magento 2 Blocks
“Jessica Tran (Senior Magento Developer, E-Commerce Solutions Inc.). In Magento 2, calling a template within a block is straightforward. You can utilize the `setTemplate()` method in your block class to specify the template file. This method allows for better separation of logic and presentation, enhancing maintainability.”
“Michael Chen (Magento Certified Solution Specialist, Digital Commerce Agency). It is essential to ensure that the template path is correctly defined in your block class. Using the `getLayout()->createBlock()` method can help in dynamically adding blocks to your layout, making it easier to call templates as needed.”
“Laura Patel (Technical Architect, Magento Community Expert). When working with custom blocks, remember to utilize the `toHtml()` method after setting the template. This approach ensures that the rendered output of the template is returned correctly, allowing for seamless integration into your Magento 2 frontend.”
Frequently Asked Questions (FAQs)
How can I call a template file from a block in Magento 2?
To call a template file from a block in Magento 2, use the `setTemplate()` method within your block class. For example, `$this->setTemplate(‘Vendor_Module::template.phtml’);` will set the specified template for rendering.
What is the purpose of the `getLayout()` method in Magento 2?
The `getLayout()` method retrieves the layout object associated with the current request. It allows you to manipulate the layout, including adding blocks and rendering templates.
Can I call a template directly in a layout XML file?
Yes, you can call a template directly in a layout XML file by specifying the `template` attribute in a `
How do I pass data to a template from a block?
To pass data to a template from a block, use the `setData()` method in your block class. For example, `$this->setData(‘key’, ‘value’);` allows you to access the data in your template using `$block->getData(‘key’);`.
What is the difference between a block and a template in Magento 2?
A block is a PHP class that contains the logic for rendering content, while a template is a .phtml file that defines the HTML structure. Blocks handle data processing, and templates focus on presentation.
How can I override a template in Magento 2?
To override a template in Magento 2, create a new template file in your custom module’s directory with the same path as the original template. Then, update the layout XML to reference your new template file.
In Magento 2, calling a template within a block is a fundamental aspect of customizing the frontend experience. This process involves creating a block class that extends the core block functionality and then specifying the template file that should be rendered. By leveraging the layout XML files, developers can define where and how these blocks are displayed on the page, ensuring a modular and maintainable code structure.
One of the key takeaways is the importance of adhering to Magento’s best practices when working with blocks and templates. Utilizing the appropriate naming conventions and file structures not only enhances code readability but also ensures compatibility with Magento’s caching mechanisms. This practice ultimately leads to improved performance and a more efficient development workflow.
Additionally, understanding the distinction between different types of blocks—such as template blocks, container blocks, and layout blocks—enables developers to make informed decisions when structuring their code. This knowledge is crucial for creating responsive and dynamic Magento 2 applications that meet the specific needs of users and clients alike.
Author Profile
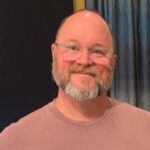
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?