How Can You Create a JavaScript File to Extend Shipping.js in Magento 2?
Introduction
In the ever-evolving landscape of eCommerce, flexibility and customization are paramount for businesses looking to stand out. Magento 2, a leading platform in this domain, offers a robust framework that allows developers to tailor their online stores to meet specific business needs. One of the critical components of this customization is the ability to extend core functionalities, such as shipping methods, through JavaScript. In this article, we will delve into the process of creating a JavaScript file to extend the `shipping.js` functionality in Magento 2, empowering developers to enhance user experiences and streamline operations.
When it comes to managing shipping options in Magento 2, the default `shipping.js` file provides a solid foundation. However, businesses often require additional features or modifications to better serve their customers. By extending this JavaScript file, developers can introduce new functionalities, such as custom shipping rates, dynamic updates based on user selections, or even integration with third-party shipping services. This flexibility not only improves the checkout experience but also allows for a more tailored approach to logistics management.
In this exploration, we will cover the essential steps and considerations for creating a custom JavaScript file that builds upon the existing `shipping.js`. From setting up your development environment to implementing the necessary code changes, this guide
Understanding the Shipping.js File Structure
The `shipping.js` file in Magento 2 is a crucial part of the checkout process, responsible for managing shipping methods and their respective calculations. To effectively extend its functionality, it is essential to comprehend its structure and the existing methods available.
Key components of `shipping.js` include:
- Shipping Method Selection: Handles the user’s selection of shipping methods.
- Shipping Rates Calculation: Interfaces with the backend to retrieve shipping rates based on user input.
- Event Listeners: Responds to user actions, such as changes in the shipping address or selection of a shipping method.
Creating a New JavaScript File
To extend the functionality of `shipping.js`, you first need to create a new JavaScript file. This file will override or add to the existing functionality. Follow these steps:
- Create a New Module: If you don’t have a custom module, create one. The directory structure should look like this:
app/code/Vendor/Module/
- Declare the Module: Create a `registration.php` file to declare your module.
php
javascript
var config = {
map: {
‘*’: {
‘Magento_Checkout/js/view/shipping’: ‘Vendor_Module/js/view/shipping’
}
}
};
- Implement Your Logic: In your new JS file (e.g., `shipping.js`), extend the `shipping.js` functionality.
javascript
define([
‘jquery’,
‘Magento_Checkout/js/view/shipping’
], function ($, Shipping) {
‘use strict’;
return Shipping.extend({
initialize: function () {
this._super();
// Your custom logic here
},
customMethod: function () {
// Additional method logic
}
});
});
Key Considerations When Extending Shipping.js
When extending `shipping.js`, consider the following best practices:
- Maintain Performance: Ensure that your customizations do not significantly impact the loading time of the checkout page.
- Modular Code: Keep your code modular and well-commented for easier maintenance and understanding.
- Testing: Thoroughly test your changes across different scenarios to ensure compatibility and correct functionality.
Example of Extended Functionality
Below is a simple example of how you might add a custom shipping method and update the shipping rates dynamically based on user selections.
javascript
customMethod: function () {
var selectedMethod = this.getSelectedShippingMethod();
// Logic to modify shipping rates based on selected method
if (selectedMethod === ‘custom_method’) {
// Custom logic for shipping rates
}
}
Common Issues and Troubleshooting
While extending `shipping.js`, you may encounter some common issues:
Issue | Solution |
---|---|
JavaScript not loading | Check `requirejs-config.js` for correctness. |
Changes not reflecting | Clear cache and deploy static content. |
Errors in the console | Review your JavaScript for syntax errors. |
By understanding the structure of `shipping.js` and following best practices, you can effectively extend its functionality to meet your business needs.
Creating a Custom JavaScript File to Extend Shipping.js in Magento 2
To extend the existing `Shipping.js` functionality in Magento 2, you will need to create a custom JavaScript module. This involves several steps, including creating the necessary file structure and overriding the default behavior.
File Structure Setup
First, establish the directory structure for your custom module:
plaintext
app/code/Vendor/ModuleName/
├── registration.php
├── etc/
│ └── module.xml
└── view/
└── frontend/
└── requirejs-config.js
└── web/
└── js/
└── shipping.js
- Vendor: Replace this with your vendor name.
- ModuleName: Replace this with your module name.
Registration and Configuration
Create the `registration.php` file to register your module:
php
Extending Shipping.js
In the `view/frontend/web/js/shipping.js` file, you can extend the default `Shipping.js`. Use the following code to achieve this:
javascript
define([
‘Magento_Checkout/js/model/shipping-rates-validator’,
‘Magento_Checkout/js/model/shipping-rates-service’,
‘Magento_Checkout/js/model/quote’,
‘Magento_Checkout/js/view/shipping’
], function (shippingRatesValidator, shippingRatesService, quote, Shipping) {
‘use strict’;
return Shipping.extend({
initialize: function () {
this._super();
// Your custom logic here
},
customMethod: function () {
// Implement your custom functionality
}
});
});
RequireJS Configuration
To ensure that your custom JavaScript is loaded instead of the default one, create a `requirejs-config.js` file:
javascript
var config = {
map: {
‘*’: {
‘Magento_Checkout/js/view/shipping’: ‘Vendor_ModuleName/js/shipping’
}
}
};
This mapping will replace the default `Shipping.js` with your custom implementation.
Deploying the Module
After the configuration, follow these steps to deploy your module:
- Run the setup upgrade command:
bash
php bin/magento setup:upgrade
- Clear the cache:
bash
php bin/magento cache:clean
php bin/magento cache:flush
- Deploy static content (in production mode):
bash
php bin/magento setup:static-content:deploy -f
- Finally, refresh the browser to see the changes in effect.
Testing Your Changes
Once your module is deployed, perform the following tests:
- Verify that your custom methods are executed as expected.
- Check for any JavaScript errors in the console.
- Ensure that the shipping functionality works seamlessly with your modifications.
By following these steps, you will successfully extend the `Shipping.js` functionality in Magento 2, allowing for tailored shipping logic to meet your business needs.
Expert Insights on Extending Shipping.js in Magento 2
Jessica Lin (Senior Magento Developer, E-Commerce Innovations). “Extending shipping.js in Magento 2 allows developers to customize shipping options effectively. By creating a new JavaScript file, you can override default behaviors and add additional functionalities that cater to specific business needs, enhancing the overall customer experience.”
Michael Chen (Lead Frontend Engineer, Digital Commerce Solutions). “When extending shipping.js, it’s crucial to maintain compatibility with existing modules. Thorough testing should be conducted to ensure that your customizations do not conflict with other JavaScript components, which can lead to unexpected behavior during checkout.”
Laura Patel (Magento Solution Architect, TechSavvy Consulting). “Implementing a well-structured approach to extend shipping.js can significantly improve the performance of your Magento store. By leveraging Magento’s built-in UI components and adhering to best practices, developers can create a seamless integration that enhances both functionality and user experience.”
Frequently Asked Questions (FAQs)
What is the purpose of extending shipping.js in Magento 2?
Extending shipping.js allows developers to customize the shipping methods and logic in Magento 2, enabling tailored functionality to meet specific business requirements.
How do I create a custom JavaScript file to extend shipping.js?
To create a custom JavaScript file, create a new file in your theme or module under the appropriate directory, typically `web/js/`, and ensure it includes the necessary dependencies and overrides for the original shipping.js.
What is the correct way to include my custom JavaScript file in Magento 2?
You can include your custom JavaScript file by adding it to the `requirejs-config.js` file in your module or theme, using the `map` configuration to point to your custom script.
How can I ensure my custom shipping.js is loaded after the original shipping.js?
To ensure your custom shipping.js loads after the original, use the `shim` configuration in `requirejs-config.js` to define dependencies, ensuring your file is executed after the original shipping.js.
Are there any best practices to follow when extending shipping.js?
Best practices include keeping your code modular, adhering to Magento’s coding standards, thoroughly testing your changes, and documenting your customizations for future reference.
What should I do if my changes to shipping.js are not reflecting on the frontend?
If changes are not reflecting, clear the Magento cache, ensure that your JavaScript file is correctly included, and check for any JavaScript errors in the browser console that may prevent execution.
In summary, extending the shipping.js file in Magento 2 is a crucial step for developers looking to customize the shipping functionality of their e-commerce platform. By creating a new JavaScript file that extends the existing shipping.js, developers can add new features, modify existing behaviors, or integrate additional logic tailored to specific business requirements. This process involves understanding the Magento 2 frontend architecture, including the use of RequireJS and the module system, which allows for efficient and modular code management.
Key takeaways from this discussion include the importance of adhering to Magento 2’s best practices when extending core functionalities. Developers should ensure that their custom JavaScript does not conflict with existing code and that it is properly registered and loaded within the Magento framework. Utilizing the right methods for dependency injection and event handling can significantly enhance the performance and maintainability of the code.
Moreover, testing the extended shipping.js thoroughly in various scenarios is essential to ensure that the new functionality works seamlessly with other components of the Magento system. By following these guidelines, developers can effectively enhance the shipping experience for customers, ultimately leading to improved satisfaction and potentially increased sales.
Author Profile
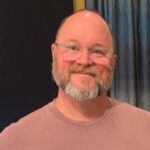
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?