How Can You Use Laravel Faker to Generate Random Dates Effortlessly?
In the world of web development, creating realistic and meaningful data for testing and development is crucial. Whether you’re building a new application or testing existing features, having a rich dataset can make all the difference. This is where Laravel’s Faker library comes into play, offering developers a powerful tool to generate random yet contextually relevant data with ease. Among the various data types Faker can produce, generating random dates is particularly useful for simulating real-world scenarios, from user sign-ups to event scheduling. In this article, we will explore how to effectively use Laravel Faker to generate random dates, enhancing your development workflow and ensuring your applications are well-tested and robust.
Laravel Faker is a library that seamlessly integrates with the Laravel framework, providing a simple and intuitive way to generate fake data. This functionality is especially beneficial when you need to populate your database with sample records for testing or when you’re working on prototypes that require realistic data without the hassle of manual entry. The ability to generate random dates can help simulate various time-sensitive features, allowing developers to create scenarios that reflect real-life usage.
As we delve deeper into the topic, we’ll uncover the various methods and options available within the Faker library to generate random dates, including how to customize date ranges and formats. Whether you’re looking to create past,
Using Laravel Faker for Random Dates
Laravel’s Faker library provides a simple way to generate fake data, which can be especially useful for seeding your database. Generating random dates is a common requirement, and Faker offers several methods to achieve this.
To generate random dates, you primarily use the `dateTime`, `dateTimeBetween`, and `dateTimeThisYear` methods. Each of these functions allows you to customize the generated date according to your needs.
– **dateTime()**: Generates a random date and time.
– **dateTimeBetween($startDate, $endDate)**: Generates a random date and time between two specified dates.
– **dateTimeThisYear()**: Generates a random date within the current year.
Here’s an example of how to implement these methods in your seeder:
“`php
use Faker\Factory as Faker;
$faker = Faker::create();
$randomDate = $faker->dateTime();
$randomDateBetween = $faker->dateTimeBetween(‘-1 year’, ‘now’);
$randomDateThisYear = $faker->dateTimeThisYear();
echo $randomDate->format(‘Y-m-d H:i:s’); // Outputs a random date
echo $randomDateBetween->format(‘Y-m-d H:i:s’); // Outputs a random date between last year and now
echo $randomDateThisYear->format(‘Y-m-d H:i:s’); // Outputs a random date within this year
“`
Customizing Date Formats
Faker also allows you to customize the format of the generated dates. You can use the `date` method to specify a format string similar to PHP’s `date()` function.
For example:
“`php
$formattedDate = $faker->date(‘Y-m-d’);
$formattedDateTime = $faker->dateTime()->format(‘Y-m-d H:i:s’);
“`
This flexibility enables you to generate dates in various formats depending on your application needs.
Generating Future or Past Dates
If you need to generate dates that are strictly in the future or the past, you can leverage the `dateTimeBetween` method with appropriate parameters.
– **Future Dates**: Use a start date that is in the future.
– **Past Dates**: Use a start date in the past.
Here’s how you can generate only future or past dates:
“`php
// Future date example
$futureDate = $faker->dateTimeBetween(‘now’, ‘+1 year’);
// Past date example
$pastDate = $faker->dateTimeBetween(‘-1 year’, ‘now’);
“`
Table of Faker Date Methods
Method | Description | Usage Example |
---|---|---|
dateTime() | Generates a random date and time. | $faker->dateTime(); |
dateTimeBetween($startDate, $endDate) | Generates a random date and time between two dates. | $faker->dateTimeBetween(‘2020-01-01’, ‘2023-01-01’); |
dateTimeThisYear() | Generates a random date within the current year. | $faker->dateTimeThisYear(); |
date($format) | Generates a date in a specified format. | $faker->date(‘Y-m-d’); |
By utilizing these methods effectively, you can easily generate random dates tailored to your application’s needs, making your database seeding process both efficient and flexible.
Generating Random Dates with Laravel Faker
Laravel Faker is a powerful library that enables developers to generate fake data for testing and seeding databases. One of the common requirements is to generate random dates. Below are methods to accomplish this effectively.
Basic Usage of Faker for Dates
To generate random dates using Laravel Faker, you first need to include the Faker library in your project. The Faker library is typically included with Laravel by default.
“`php
use Faker\Factory as Faker;
$faker = Faker::create();
“`
Once you have the Faker instance, you can generate random dates using a variety of methods:
– **Random Date in the Past**:
This generates a date from the past.
“`php
$pastDate = $faker->dateTimeBetween(‘-1 years’, ‘now’);
“`
– **Random Date in the Future**:
This generates a date in the future.
“`php
$futureDate = $faker->dateTimeBetween(‘now’, ‘+1 years’);
“`
– **Random Date within a Specific Range**:
You can specify a custom range for more control over the generated dates.
“`php
$customDate = $faker->dateTimeBetween(‘2020-01-01’, ‘2023-12-31’);
“`
Formatting Generated Dates
Faker allows you to format the generated dates to fit your specific needs. You can format dates using the `format` method:
“`php
$formattedDate = $faker->dateTimeBetween(‘-1 years’, ‘now’)->format(‘Y-m-d H:i:s’);
“`
Common Date Formats
Format | Description |
---|---|
`Y-m-d` | 2023-10-25 |
`d/m/Y` | 25/10/2023 |
`F j, Y` | October 25, 2023 |
`Y-m-d H:i` | 2023-10-25 14:30 |
Seeding with Random Dates
When using Faker in database seeding, you can directly assign random dates to your model attributes. Here’s an example of how to seed a `users` table with random registration dates:
“`php
use Illuminate\Database\Seeder;
use App\Models\User;
class UserSeeder extends Seeder
{
public function run()
{
$faker = Faker::create();
foreach (range(1, 50) as $index) {
User::create([
‘name’ => $faker->name,
’email’ => $faker->unique()->safeEmail,
‘registered_at’ => $faker->dateTimeBetween(‘-2 years’, ‘now’),
]);
}
}
}
“`
Using Custom Date Formats in Seeders
When seeding, you may want to ensure that the date format matches your database requirements. Here’s how you can format the date before saving:
“`php
‘registered_at’ => $faker->dateTimeBetween(‘-2 years’, ‘now’)->format(‘Y-m-d H:i:s’),
“`
This ensures that the date is saved in a consistent format, making it easier to query and manipulate later.
Using Laravel Faker to generate random dates is straightforward and flexible. By leveraging its methods and formatting capabilities, you can easily create realistic date data for testing and seeding purposes.
Expert Insights on Generating Random Dates with Laravel Faker
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “Utilizing Laravel Faker to generate random dates is an essential practice for developers aiming to populate their databases with realistic data. It not only enhances testing but also ensures that applications can handle a variety of date inputs seamlessly.”
James Thornton (Lead Developer, CodeCraft Solutions). “The flexibility of Laravel Faker in generating random dates allows developers to simulate real-world scenarios effectively. By using the ‘dateTimeBetween’ method, one can specify a range, providing a robust way to test date-related functionalities in applications.”
Linda Zhao (Data Scientist, Analytics Hub). “Incorporating random date generation through Laravel Faker is critical for data analysis and machine learning projects. It helps in creating diverse datasets, which can lead to more accurate models and insights when analyzing trends over time.”
Frequently Asked Questions (FAQs)
What is Laravel Faker?
Laravel Faker is a library used to generate fake data for testing and seeding databases in Laravel applications. It provides a variety of methods to create random data, including names, addresses, and dates.
How can I generate a random date using Laravel Faker?
You can generate a random date by using the `dateTime` or `dateTimeBetween` methods provided by the Faker library. For example, `$faker->dateTime()` generates a random date and time, while `$faker->dateTimeBetween($startDate, $endDate)` allows you to specify a range for the random date.
Can I specify a format for the random date generated by Laravel Faker?
Yes, you can format the random date by using the `format` method. For instance, `$faker->dateTime()->format(‘Y-m-d’)` will return the date in the specified ‘YYYY-MM-DD’ format.
Is it possible to generate random dates within a specific year using Laravel Faker?
Yes, you can generate random dates within a specific year by using the `dateTimeBetween` method with the start and end dates set to that year. For example, `$faker->dateTimeBetween(‘2023-01-01’, ‘2023-12-31’)` will yield a random date within the year 2023.
What are the advantages of using Laravel Faker for generating random dates?
Using Laravel Faker for generating random dates ensures consistency and realism in test data. It allows developers to create diverse datasets quickly, which is essential for testing various scenarios in applications.
Can Laravel Faker generate future dates?
Yes, Laravel Faker can generate future dates by specifying a future range in the `dateTimeBetween` method. For example, `$faker->dateTimeBetween(‘now’, ‘+1 year’)` will generate a random date within the next year.
In summary, Laravel Faker is a powerful tool that allows developers to generate random data for testing and seeding databases. One of its useful features is the ability to generate random dates, which can be crucial for creating realistic datasets. By utilizing the Faker library, developers can easily create dates within specific ranges or formats, enhancing the quality of their applications during the development phase.
Key takeaways include the flexibility of Faker in generating various types of date formats and the ease of integration into Laravel projects. Developers can specify parameters such as start and end dates to ensure that the generated random dates meet their specific requirements. This capability not only saves time but also improves the reliability of tests by providing diverse data scenarios.
Moreover, leveraging Laravel Faker for random date generation can significantly improve the efficiency of the development workflow. By automating the creation of test data, developers can focus more on building features and less on manual data entry. Overall, incorporating Faker into Laravel applications is a best practice that enhances both productivity and the robustness of the testing process.
Author Profile
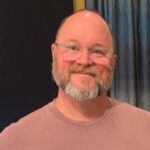
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?