How Can You Use Laravel 11’s dd() Function to Dump All Request Data?
In the world of web development, Laravel has emerged as a powerful framework that streamlines the process of building robust applications. As developers dive into the intricacies of Laravel 11, they often seek efficient ways to debug their applications and manage HTTP requests. One of the most effective tools at their disposal is the `dd()` function, a debugging aid that allows developers to quickly inspect variables and request data. In this article, we will explore how to leverage `dd()` in conjunction with the `$request` object to gain deeper insights into incoming data, ultimately enhancing the development workflow.
Understanding how to utilize `dd()` effectively can transform the way developers interact with incoming requests. The `$request` object in Laravel encapsulates all the data sent by the client, including form inputs, query parameters, and more. By using `dd($request)`, developers can instantly visualize the entire request payload, making it easier to identify issues and ensure that data is being processed correctly. This powerful combination not only aids in debugging but also enhances the overall efficiency of the development process.
As we delve deeper into the nuances of Laravel 11 and its debugging capabilities, we will uncover best practices for using `dd()` with the `$request` object. From understanding the structure of the request to filtering
Understanding the Request Object
The `$request` object in Laravel is a powerful tool that allows developers to handle incoming HTTP requests. It encapsulates all the data associated with a request, including query parameters, form data, and uploaded files. This object provides a straightforward way to access this data and manage user input effectively.
Key features of the `$request` object include:
- Access to Input Data: You can easily retrieve input data via methods such as `input()`, `query()`, and `all()`.
- File Uploads: The `$request` object simplifies the handling of file uploads through the `file()` method.
- Session Management: It provides convenient access to session data through the `session()` method.
Using dd() with the Request Object
The `dd()` function in Laravel stands for “dump and die.” It is a helpful debugging tool that outputs the contents of a variable and stops the script execution. Using `dd()` on the `$request` object can help you inspect all incoming data for debugging purposes.
Example of using `dd()` with `$request`:
“`php
public function store(Request $request)
{
dd($request);
}
“`
When invoked, this will display all properties of the `$request` object, including headers, method, and all input data.
Dumping All Request Data
To get a comprehensive view of all the data contained within the `$request` object, you can utilize the `all()` method, which returns all input data as an array. This can be particularly useful when you want to inspect all incoming data in a readable format.
For example:
“`php
public function store(Request $request)
{
dd($request->all());
}
“`
This will output an array of all input data, making it easier to debug large sets of information.
Table of Common Request Methods
The following table summarizes common methods used with the `$request` object in Laravel:
Method | Description |
---|---|
input($key, $default = null) | Retrieve a value from the request input. |
all() | Get all input data as an associative array. |
query($key = null, $default = null) | Retrieve a query string parameter. |
file($key) | Retrieve an uploaded file from the request. |
has($key) | Determine if the request contains a given input item. |
Utilizing these methods effectively can greatly enhance your debugging process and streamline how you handle incoming requests within your Laravel applications.
Using `dd()` with `$request` to Dump All Data
In Laravel, the `dd()` function is a powerful tool that allows developers to quickly dump and die, providing an immediate look at the contents of variables. When dealing with HTTP requests, you may want to inspect the entirety of the data contained within a `$request` object.
Accessing Request Data
To access all the input data from a request, you can utilize the `all()` method provided by the `$request` object. This method retrieves all input data as an associative array.
“`php
dd($request->all());
“`
This command will output all the data submitted with the request, including:
- Query parameters
- Form data
- Uploaded files
Dumping Specific Input Types
If you need to inspect specific types of input, Laravel provides methods to filter the data accordingly:
– **Query Parameters:**
“`php
dd($request->query());
“`
– **Form Data:**
“`php
dd($request->input());
“`
– **Uploaded Files:**
“`php
dd($request->file());
“`
Utilizing these specific methods can help you narrow down your debugging process.
Handling JSON Requests
When dealing with JSON payloads, the request data can be accessed similarly. Laravel automatically parses JSON data, and you can use the `getContent()` method for raw access:
“`php
dd(json_decode($request->getContent(), true));
“`
This will decode the JSON string into an associative array, allowing you to inspect it easily.
Using `dd()` in Middleware
If you want to inspect requests globally, consider using `dd()` in middleware. You can create a middleware that dumps the request data for debugging purposes:
“`php
public function handle($request, Closure $next)
{
dd($request->all());
return $next($request);
}
“`
This approach allows you to review requests systematically without modifying individual controllers.
Customizing Output with `toArray()`
For more advanced scenarios, you may want to convert the request to an array with additional customization. The `toArray()` method can be helpful:
“`php
dd($request->toArray());
“`
This method provides a clean array representation of the request data, making it easier to read and analyze.
Performance Considerations
While `dd()` is invaluable during development, be cautious about its use in production environments. Frequent dumping of request data can lead to performance issues and exposure of sensitive information.
- Limit usage to development environments.
- Consider logging data instead of dumping it in production.
- Always sanitize output to avoid leaking sensitive data.
Effective debugging in Laravel using `dd()` with the `$request` object can significantly enhance your development process. By leveraging the various methods available for accessing request data, you can streamline your debugging workflow and ensure a smoother development experience.
Expert Insights on Laravel 11’s Request Handling
Jessica Tran (Senior PHP Developer, CodeCraft Solutions). “Utilizing the `dd($request)` function in Laravel 11 is an efficient way to debug incoming requests. It allows developers to quickly inspect the contents of the request object, making it easier to identify issues with data being sent to the application.”
Michael Chen (Lead Software Engineer, Tech Innovations Inc.). “In Laravel 11, leveraging `dd($request)` not only simplifies debugging but also enhances the development workflow. It provides a comprehensive view of the request data, including headers and payload, which is crucial for troubleshooting API interactions.”
Laura Patel (Full-Stack Developer, Digital Solutions Group). “The `dd($request)` function is an invaluable tool in Laravel 11 for inspecting the request lifecycle. It helps developers understand how data flows through the application and ensures that the expected data structure is maintained throughout the process.”
Frequently Asked Questions (FAQs)
What is the purpose of using `dd($request)` in Laravel?
Using `dd($request)` in Laravel allows developers to dump and die the contents of the request object, providing a quick way to inspect incoming request data, including headers, query parameters, and input data.
How do I use `dd()` to display all request data in Laravel 11?
To display all request data, you can simply call `dd($request->all())`, which will output all input data from the request as an array, making it easy to debug.
Can I use `dd($request)` in controller methods?
Yes, `dd($request)` can be used within any controller method to inspect the incoming request data at that point in the application’s lifecycle.
What is the difference between `dd($request)` and `dump($request)`?
`dd($request)` will output the request data and immediately terminate the script, while `dump($request)` will output the data without terminating the script, allowing further code execution.
Is it safe to use `dd()` in production environments?
It is not recommended to use `dd()` in production environments, as it exposes sensitive request data. It is best suited for development and debugging purposes only.
What alternatives exist for debugging requests in Laravel?
Alternatives include using logging functions like `Log::info($request->all())` to log request data to a file, or using Laravel’s built-in debugging tools such as Telescope for a more comprehensive overview of requests and application performance.
utilizing Laravel 11’s debugging capabilities, particularly the `dd()` (dump and die) function, can significantly enhance the development process. By leveraging `$request` to `dd` all incoming request data, developers can gain immediate insights into the structure and content of the request. This practice not only aids in troubleshooting but also allows for a deeper understanding of how data flows through the application.
Moreover, the ability to inspect the entire request object provides a comprehensive view of all parameters, headers, and files associated with the request. This is particularly valuable during the development phase, where understanding user input is crucial for building robust applications. The `dd()` function serves as a powerful tool for rapid debugging, helping developers to identify issues quickly and efficiently.
Key takeaways from the discussion include the importance of effective debugging techniques in Laravel 11 and the role of the `$request` object in managing user input. By incorporating `dd($request->all())` into the development workflow, developers can streamline their debugging process and improve overall application quality. Emphasizing best practices in debugging will ultimately lead to more maintainable and reliable code.
Author Profile
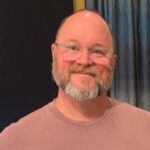
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?