How Can I Use jQuery to Mimic Chat Assistant Responses?
In the ever-evolving landscape of web development, creating interactive and engaging user experiences has become paramount. One of the most compelling ways to achieve this is through the implementation of chat assistants that can mimic human responses. Utilizing jQuery, developers can craft chat interfaces that not only respond intelligently but also enhance user interaction. This article delves into the fascinating world of jQuery-based chat assistants, exploring how they can simulate conversational exchanges, improve user engagement, and streamline communication on websites.
As we navigate through the intricacies of jQuery, we’ll uncover the fundamental techniques that empower developers to build chat assistants capable of mimicking human-like responses. From understanding the core principles of jQuery to implementing dynamic response systems, the journey will highlight the essential tools and methodologies that make these chat interfaces not just functional, but also intuitive and engaging.
Moreover, we will examine the benefits of integrating a jQuery mimic chat assistant into various applications, emphasizing its role in enhancing user satisfaction and driving conversions. Whether you’re a seasoned developer or a curious newcomer, this exploration will equip you with the insights needed to harness the power of jQuery in creating chat assistants that resonate with users and elevate their online experience.
Implementing jQuery for Chat Assistant Responses
To effectively mimic chat assistant responses using jQuery, developers can leverage various techniques that enhance user interaction and provide a more engaging experience. By utilizing jQuery’s capabilities, developers can create dynamic and responsive chat interfaces that simulate real-time conversations.
One common approach involves using jQuery to animate the display of messages, making them appear more lifelike. This can be achieved through functions that control the visibility and timing of message elements.
Creating a Simple Chat Interface
A basic chat interface consists of input fields, a display area for messages, and buttons for sending messages. The following HTML structure can serve as a foundation:
“`html
“`
With this structure, jQuery can be utilized to manage user input and display responses.
jQuery Functions for Interaction
The following jQuery functions can be implemented to enhance the chat experience:
- Sending Messages: Captures user input and appends it to the message area.
- Generating Responses: Mimics a chat assistant’s reply using predefined responses or simple algorithms.
Here’s a code snippet illustrating these functions:
“`javascript
$(document).ready(function() {
$(‘send-button’).click(function() {
var userMessage = $(‘user-input’).val();
$(‘message-area’).append(‘
‘);
$(‘user-input’).val(”);
// Mimic chat assistant response
setTimeout(function() {
$(‘message-area’).append(‘
‘);
}, 1000);
});
});
“`
Styling the Chat Interface
To create a visually appealing chat interface, CSS can be applied to differentiate between user and assistant messages. Below is a simple styling example:
“`css
chat-container {
border: 1px solid ccc;
padding: 10px;
width: 300px;
height: 400px;
overflow-y: scroll;
}
.user-message {
text-align: right;
background-color: e0f7fa;
padding: 5px;
margin: 5px;
border-radius: 10px;
}
.assistant-message {
text-align: left;
background-color: f1f8e9;
padding: 5px;
margin: 5px;
border-radius: 10px;
}
“`
Enhancing User Experience with Delays
To mimic a more realistic chat experience, introducing delays in responses can be effective. This can be done using the `setTimeout` function, which allows developers to control the timing of responses, giving users the impression that the assistant is thinking before replying.
Sample Response Table
The following table provides examples of user queries and corresponding assistant responses:
User Query | Assistant Response |
---|---|
Hello! | Hi there! How can I assist you today? |
What is the weather like? | The weather is sunny with a chance of rain in the evening. |
Tell me a joke. | Why did the scarecrow win an award? Because he was outstanding in his field! |
By following these guidelines, developers can create an engaging and responsive chat assistant that enhances user interaction through jQuery.
Implementing jQuery for Chat Assistant Responses
To create a chat assistant that mimics human responses using jQuery, several key components must be integrated. These include event handling, dynamic content rendering, and response generation logic.
Setting Up the HTML Structure
An effective chat interface begins with a well-defined HTML structure. This includes elements for displaying messages, a text input for user input, and a button to send messages.
“`html
“`
Styling the Chat Interface
CSS can enhance the user experience by making the chat interface visually appealing. Below is a simple CSS styling guide.
“`css
chat-window {
border: 1px solid ccc;
padding: 10px;
width: 300px;
height: 400px;
overflow-y: scroll;
}
messages {
height: 350px;
overflow-y: auto;
}
user-input {
width: 70%;
}
send-btn {
width: 25%;
}
“`
jQuery Script for Interaction
The core functionality of the chat assistant can be developed using jQuery. Below is an example of a script that handles user input and simulates a response from the assistant.
“`javascript
$(document).ready(function() {
$(‘send-btn’).click(function() {
var userMessage = $(‘user-input’).val();
if (userMessage) {
$(‘messages’).append(‘
‘);
$(‘user-input’).val(”);
simulateResponse(userMessage);
}
});
function simulateResponse(userMessage) {
var response = generateResponse(userMessage);
setTimeout(function() {
$(‘messages’).append(‘
‘);
$(‘chat-window’).scrollTop($(‘chat-window’)[0].scrollHeight);
}, 1000);
}
function generateResponse(message) {
// Simple response logic
if (message.includes(“hello”)) {
return “Hello! How can I assist you today?”;
} else if (message.includes(“help”)) {
return “I’m here to help! Please describe your issue.”;
} else {
return “I’m not sure how to respond to that.”;
}
}
});
“`
Enhancing the Chatbot with More Features
To make the chat assistant more interactive and human-like, consider the following enhancements:
- Typing Indicator: Display an indicator while the assistant is “typing.”
- Randomized Responses: Use an array of responses to provide variety in replies.
- User Message Formatting: Differentiate between user and assistant messages visually.
Example of Randomized Responses
The response function can be modified to include an array for varied replies.
“`javascript
function generateResponse(message) {
var responses = {
“hello”: [“Hi there!”, “Hello! How can I assist you?”, “Greetings! What can I do for you?”],
“help”: [“Sure! What do you need help with?”, “I’m here to help you. Please tell me more.”],
“default”: [“I’m not sure about that.”, “Can you please clarify?”]
};
for (var key in responses) {
if (message.includes(key)) {
var randIndex = Math.floor(Math.random() * responses[key].length);
return responses[key][randIndex];
}
}
return responses[“default”][Math.floor(Math.random() * responses[“default”].length)];
}
“`
Testing and Debugging the Chat Assistant
Testing is crucial to ensure the chat assistant functions as intended. Key aspects to test include:
- User input handling
- Response generation accuracy
- Interface usability on various devices
Utilize browser developer tools to debug any issues, ensuring a seamless user experience.
Expert Insights on Jquery Mimic Chat Assistant Responses
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Implementing jQuery to mimic chat assistant responses can significantly enhance user experience by providing dynamic interactions. The key lies in ensuring that the responses are contextually relevant and timely, which can be achieved through effective event handling and AJAX calls.”
Michael Thompson (Lead UX Designer, Interactive Solutions). “Using jQuery to create a chat assistant interface allows for a more fluid and engaging user experience. It is crucial to focus on the design elements and response animations to make the interactions feel more human-like, thereby increasing user satisfaction and retention.”
Sarah Lee (Digital Interaction Specialist, Future Tech Labs). “Mimicking chat assistant responses with jQuery can streamline communication in web applications. However, developers must prioritize accessibility and performance to ensure that all users, regardless of their technical proficiency, can benefit from these interactive features.”
Frequently Asked Questions (FAQs)
What is jQuery Mimic Chat Assistant Responses?
jQuery Mimic Chat Assistant Responses refers to using jQuery, a popular JavaScript library, to create interactive chat interfaces that simulate responses typically provided by virtual assistants.
How can I implement jQuery to mimic chat responses?
You can implement jQuery to mimic chat responses by utilizing AJAX calls to fetch pre-defined responses or by creating a simple array of responses that are randomly selected based on user input.
What are the benefits of using jQuery for chat interfaces?
The benefits of using jQuery for chat interfaces include simplified DOM manipulation, enhanced event handling, and the ability to create dynamic content without reloading the page, which improves user experience.
Are there any specific plugins for creating chat assistants with jQuery?
Yes, there are several jQuery plugins available, such as jQuery Chat, jQuery Simple Chat, and others that provide pre-built functionalities for creating chat interfaces, making development faster and easier.
Can jQuery mimic natural language processing in chat responses?
While jQuery itself does not provide natural language processing capabilities, it can be integrated with external APIs or libraries that specialize in NLP to enhance the chat assistant’s ability to understand and generate human-like responses.
Is it possible to customize the appearance of the chat interface using jQuery?
Yes, jQuery allows for extensive customization of the chat interface’s appearance through CSS manipulation, enabling developers to style the chat window, text bubbles, and overall layout to match their application’s design.
In summary, utilizing jQuery to mimic chat assistant responses can significantly enhance user interaction on websites. By leveraging jQuery’s capabilities, developers can create dynamic and responsive chat interfaces that simulate real-time conversations. This approach not only improves user engagement but also provides a more personalized experience, allowing users to receive instant feedback and assistance.
One of the key takeaways from the discussion is the importance of user experience in the design of chat assistants. A well-implemented jQuery solution can facilitate seamless interactions, making it easier for users to navigate through information and receive support. Moreover, incorporating features such as message animations and typing indicators can further enrich the chat experience, making it feel more human-like and engaging.
Additionally, developers should consider the scalability and maintainability of their jQuery chat solutions. As user demands evolve, it is essential to build a system that can adapt to new requirements without extensive rework. This foresight not only ensures longevity but also enhances the overall effectiveness of the chat assistant in meeting user needs.
Author Profile
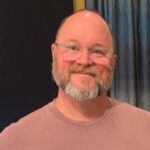
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?