How Can jQuery Be Used to Mimic AI Chat Responses?
In the digital age, the way we interact with technology is evolving at an unprecedented pace. One of the most exciting developments in this realm is the rise of AI chatbots, which are transforming customer service, personal assistance, and even entertainment. But what if you could harness the power of jQuery to create your own AI chat responses that mimic human-like interactions? This article delves into the fascinating world of jQuery and its potential to enhance user experiences through intelligent chat interfaces. Whether you’re a seasoned developer or a curious beginner, the possibilities are boundless, and the journey is just beginning.
As we explore the intersection of jQuery and AI chat responses, we will uncover how this popular JavaScript library can be utilized to create dynamic and responsive chat interfaces. By leveraging jQuery’s capabilities, developers can build chatbots that not only respond to user queries but also engage users in a more conversational manner. This approach not only improves user satisfaction but also fosters a deeper connection between users and digital platforms.
Moreover, the integration of AI technologies into jQuery-based chat systems opens up a world of opportunities for customization and personalization. Imagine chatbots that learn from interactions, adapt their responses, and provide tailored experiences based on user preferences. This article will guide you through the essential concepts
Understanding the Basics of jQuery
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. By utilizing jQuery, developers can write less code to achieve complex functionalities, which is particularly useful for mimicking AI chat responses.
Key features of jQuery include:
- DOM Manipulation: Easily modify HTML elements and attributes.
- Event Handling: Simplify the process of capturing user interactions.
- Animations: Create engaging user experiences with simple methods.
- AJAX: Facilitate asynchronous data retrieval, enhancing responsiveness.
Setting Up jQuery for Chat Responses
To mimic AI chat responses using jQuery, the first step is to include the jQuery library in your HTML file. You can either download it or link to a CDN. For example:
“`html
“`
Once jQuery is included, you can begin to manipulate the DOM to create chat functionalities. The structure of a basic chat application typically includes:
- An input field for user messages.
- A display area for chat responses.
- A button to send messages.
Here’s a simple HTML structure:
“`html
“`
Creating Chat Responses with jQuery
Implementing chat responses involves capturing user input and displaying simulated AI responses. Below is a jQuery code snippet that demonstrates this functionality:
“`javascript
$(document).ready(function() {
$(‘send-button’).click(function() {
var userMessage = $(‘user-input’).val();
$(‘chat-display’).append(‘
‘);
$(‘user-input’).val(”);
// Simulating AI response
setTimeout(function() {
var aiResponse = generateAIResponse(userMessage);
$(‘chat-display’).append(‘
‘);
}, 1000);
});
});
function generateAIResponse(message) {
// Basic example of AI response logic
if (message.includes(“hello”)) {
return “Hi there! How can I help you today?”;
} else {
return “I’m sorry, I didn’t understand that.”;
}
}
“`
Styling the Chat Interface
A visually appealing chat interface enhances user experience. Consider the following CSS to style the chat container:
“`css
chat-container {
width: 300px;
border: 1px solid ccc;
padding: 10px;
border-radius: 5px;
}
chat-display {
height: 200px;
overflow-y: scroll;
margin-bottom: 10px;
}
.user-message {
text-align: right;
color: blue;
}
.ai-response {
text-align: left;
color: green;
}
“`
Enhancing User Experience
To further improve the chat experience, consider implementing the following features:
- Typing Indicators: Show a “typing…” message while the AI is generating a response.
- Message History: Store chat history to allow users to scroll back through previous messages.
- Keyboard Shortcuts: Enable users to send messages with the Enter key.
The table below summarizes additional functionalities that can enhance chat interfaces:
Feature | Description |
---|---|
Typing Indicators | Displays an indicator when the AI is processing a response. |
Message History | Allows users to scroll back and view past messages. |
Keyboard Shortcuts | Enable sending messages using the Enter key. |
Understanding jQuery for AI Chat Responses
jQuery is a powerful JavaScript library that simplifies HTML document traversing, event handling, and animation, making it an excellent choice for developing dynamic web applications, including AI chat interfaces. Utilizing jQuery can enhance user experience by providing smooth interactions and quick responses.
Key Features of jQuery
- DOM Manipulation: Simplifies the process of selecting and manipulating HTML elements.
- Event Handling: Streamlines event management, allowing for responsive chat features.
- AJAX Support: Facilitates asynchronous requests to servers, enabling real-time chat interactions without full page reloads.
Setting Up jQuery in Your Project
To integrate jQuery into your web application, you can either download it or link to a CDN. Here’s how to set it up via CDN:
“`html
“`
Creating a Simple Chat Interface
A basic chat interface can be constructed using HTML and jQuery. Below is an example layout:
“`html
“`
Implementing AI Chat Responses
To mimic AI chat responses, you can set up a simple script that simulates a delay, providing a more human-like interaction. Here’s a jQuery example:
“`javascript
$(document).ready(function() {
$(‘sendButton’).click(function() {
const userMessage = $(‘userInput’).val();
$(‘messages’).append(`
`);
$(‘userInput’).val(”);
setTimeout(function() {
const aiResponse = getAIResponse(userMessage);
$(‘messages’).append(`
`);
}, 1000); // Mimic delay of 1 second
});
function getAIResponse(message) {
// Simple AI logic for demo purposes
if (message.includes(“hello”)) {
return “Hi there! How can I help you today?”;
}
return “I’m not sure how to respond to that.”;
}
});
“`
Enhancing User Experience
To make the chat more interactive and engaging, consider implementing the following features:
- Typing Indicators: Show an indicator when the AI is “typing” to enhance realism.
- Message History: Store previous messages for continuity.
- User Feedback: Allow users to rate responses for future improvements.
Example of a Typing Indicator
You can enhance the chat experience with a typing indicator using jQuery:
“`javascript
function showTypingIndicator() {
$(‘messages’).append(“
“);
setTimeout(function() {
$(‘.ai-typing’).remove();
}, 1000);
}
“`
Conclusion on jQuery Integration
By leveraging jQuery for AI chat applications, developers can create responsive, user-friendly interfaces that enhance interaction quality. Integrating features such as AJAX calls for real-time communication and user input handling makes the chat experience both efficient and effective.
Expert Insights on Jquery Mimic AI Chat Responses
Dr. Emily Carter (Lead AI Researcher, Tech Innovations Lab). “Jquery can significantly enhance the user experience in chat applications by mimicking AI responses. By integrating Jquery, developers can create dynamic interfaces that provide real-time feedback, making interactions feel more natural and engaging.”
Michael Chen (Senior Software Engineer, Interactive Solutions Inc.). “Using Jquery to mimic AI chat responses allows for a more fluid interaction model. It enables developers to simulate conversational patterns and responses, which can be particularly useful in training AI systems to understand human-like dialogue.”
Lisa Patel (UX/UI Designer, Future Tech Designs). “Incorporating Jquery into AI chat interfaces not only enhances responsiveness but also allows for the implementation of animations and transitions that can make the chat experience more intuitive. This approach can lead to increased user satisfaction and retention.”
Frequently Asked Questions (FAQs)
What is jQuery Mimic AI Chat Responses?
jQuery Mimic AI Chat Responses refers to the use of jQuery, a JavaScript library, to create chat interfaces that simulate artificial intelligence responses, providing users with an interactive experience similar to chatting with a real AI.
How can I implement jQuery to mimic AI chat responses?
To implement jQuery for mimicking AI chat responses, you can use jQuery to handle user input, manage chat logs, and generate pre-defined responses or random replies based on user queries, enhancing interactivity.
Are there any libraries or plugins that assist with jQuery AI chat simulations?
Yes, several libraries and plugins, such as jQuery Chatbot and BotUI, can simplify the development of chat interfaces by providing built-in functionalities for creating chatbots that mimic AI responses.
What are the benefits of using jQuery for chat interfaces?
Using jQuery for chat interfaces offers advantages such as simplified DOM manipulation, event handling, and AJAX support, which can enhance user experience and streamline the development process.
Can jQuery mimic complex AI responses, such as natural language processing?
While jQuery can simulate basic AI responses, it does not inherently support complex natural language processing. Integrating external APIs or libraries specialized in NLP would be necessary for more advanced capabilities.
Is it possible to integrate jQuery chat simulations with backend AI services?
Yes, jQuery chat simulations can be integrated with backend AI services through AJAX calls or WebSocket connections, allowing real-time communication and dynamic response generation based on user interactions.
In summary, the concept of using jQuery to mimic AI chat responses revolves around leveraging the capabilities of jQuery to create interactive and dynamic web applications. By utilizing jQuery’s powerful DOM manipulation and event handling features, developers can simulate the behavior of AI chatbots, providing users with an engaging and responsive experience. This approach allows for the integration of pre-defined responses, creating a semblance of intelligent conversation without the complexities of actual AI implementation.
Furthermore, the implementation of jQuery in this context highlights the importance of user experience in web design. By mimicking conversational patterns, developers can enhance user engagement and satisfaction. The ability to provide instant feedback and simulate a dialogue can make web applications feel more intuitive and accessible, thereby encouraging users to interact more frequently and effectively with the platform.
Ultimately, the use of jQuery to replicate AI chat responses serves as a valuable tool for developers seeking to enhance interactivity on their websites. It emphasizes the significance of creating user-friendly interfaces that can respond to user inputs in a timely manner. As technology continues to evolve, the methods of integrating such features will likely become more sophisticated, paving the way for richer user experiences in the digital landscape.
Author Profile
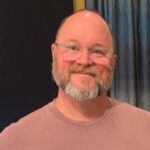
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?