How to Resolve the ‘Jest Modulenamemapper: Encountered an Unexpected Token in Cjs Folder’ Error?
In the realm of JavaScript testing, Jest has emerged as a powerful tool, offering developers a seamless way to ensure their code performs as expected. However, as projects grow in complexity and structure, developers often encounter various challenges that can hinder their testing experience. One such challenge is the error message: “Jest encountered an unexpected token” when working with module name mapping in a CommonJS (CJS) folder. This situation can be frustrating, especially when it disrupts the flow of development and testing. In this article, we will delve into the intricacies of Jest’s module name mapping and explore the underlying causes of this error, equipping you with the knowledge to troubleshoot and resolve it effectively.
Understanding how Jest handles module resolution and mapping is crucial for any developer looking to streamline their testing process. When working with different module formats, such as CommonJS and ES modules, Jest’s configuration can sometimes lead to unexpected behaviors, particularly when it comes to interpreting module paths. The “unexpected token” error often arises from misconfigurations or incompatibilities within the project setup, leaving developers puzzled about the next steps to take.
As we explore this topic, we’ll break down the common pitfalls associated with Jest’s module name mapping and provide insights into best practices for configuring your
Understanding Jest Module Name Mapper
Jest’s module name mapper is a powerful feature that allows developers to customize how modules are resolved in their tests. This can be particularly useful when working with libraries that may not be structured in a way that Jest recognizes by default. The configuration for the module name mapper can be set in the `jest.config.js` file, allowing you to specify how certain paths should be handled.
To use module name mapping effectively, you can define patterns that replace module paths with specific directories or mock implementations. Here are some common use cases:
- Mapping absolute paths to relative paths.
- Redirecting module imports to mock versions during testing.
- Handling third-party libraries that do not have proper module exports.
The configuration typically looks like this:
“`javascript
module.exports = {
moduleNameMapper: {
‘^@components/(.*)$’: ‘
‘^@utils/(.*)$’: ‘
},
};
“`
Common Issues and Error Handling
When utilizing Jest with a module name mapper, you may encounter various issues, particularly with unexpected tokens or file formats. One common error is the message: “Jest encountered an unexpected token” when attempting to import files from a directory such as `cjs`. This usually indicates that Jest is trying to process a file format it doesn’t recognize, typically CommonJS (CJS) modules.
To address this, consider the following strategies:
- Check your Babel Configuration: Ensure that your Babel is properly set up to transpile CJS modules. You may need to include the necessary presets or plugins in your Babel configuration file.
- Transform CJS files: If you are using CommonJS files, make sure Jest is set to transform them. You can specify transform settings in your Jest configuration:
“`javascript
transform: {
‘^.+\\.jsx?$’: ‘babel-jest’,
‘^.+\\.cjs$’: ‘babel-jest’,
},
“`
- File Extensions: Ensure that your file extensions are correctly configured in Jest. If you are using files with specific extensions, such as `.cjs`, make sure those extensions are included in your Jest configuration.
- ESM Support: If your project uses ECMAScript modules (ESM), ensure your Node.js version supports it and that your Jest configuration is set up to handle ESM.
Resolving the Unexpected Token Error
When encountering the “unexpected token” error specifically related to CJS folders, follow these troubleshooting steps:
- Verify File Structure: Ensure that the paths defined in your module name mapper correspond accurately to your project structure. Any misalignment can lead to errors.
- Use the Correct Jest Version: Ensure you are using a version of Jest that supports the features you are trying to use. Check for updates or known issues in the Jest repository.
- Review Dependency Versions: Sometimes, the issue may arise from outdated dependencies. Make sure your related libraries (like Babel and Jest) are updated to their latest versions.
- Testing Configuration: Use a simple test case to isolate the problem. Create a minimal setup to determine if the issue is with the Jest configuration or the specific files being imported.
Issue | Possible Solution |
---|---|
Unexpected token error | Verify Babel configuration and file extensions. |
Module not found | Check module name mapper paths. |
Transform errors | Update Jest and Babel dependencies. |
By systematically addressing these aspects, you can effectively troubleshoot and resolve issues related to Jest’s module name mapper and unexpected tokens in your project.
Understanding Jest ModuleNameMapper
Jest’s `moduleNameMapper` is a configuration option that allows you to define custom module paths and aliases, which can help in resolving modules during testing. This feature is particularly useful when dealing with complex directory structures or when using libraries that require specific path resolutions.
Common Use Cases for ModuleNameMapper:
- Mapping module paths to simplify imports.
- Resolving asset files like stylesheets or images.
- Mocking modules during testing to isolate unit tests.
Example Configuration:
“`json
“jest”: {
“moduleNameMapper”: {
“^@components/(.*)$”: “
“^@assets/(.*)$”: “
}
}
“`
Encountering Unexpected Token Errors
An unexpected token error typically arises when Jest attempts to parse files that are not in a format it can understand. This can occur when a `.cjs` folder or file is encountered, especially if it contains ES module syntax without proper configuration.
Common Causes:
- Using ES modules in a project configured for CommonJS.
- Incorrect file extensions or misconfigured build tools.
- Absence of Babel or other transpilers in the Jest setup.
Debugging Steps:
- Verify the file extension of the module causing the error. Ensure it matches the expected format.
- Check your Jest configuration for the presence of `transform` settings that specify how files should be processed.
- Ensure Babel is correctly set up to transpile both ES and CommonJS code.
Resolving Unexpected Token Issues in .cjs Files
To handle unexpected token errors related to `.cjs` files, consider the following solutions:
Configuration Adjustments:
- Update Jest’s `transform` option to include `.cjs` files:
“`json
“jest”: {
“transform”: {
“^.+\\.(js|jsx|ts|tsx|cjs)$”: “babel-jest”
}
}
“`
- If using TypeScript, ensure that `ts-jest` is set up to handle different module types:
“`json
“jest”: {
“preset”: “ts-jest”,
“globals”: {
“ts-jest”: {
“useESM”: true
}
}
}
“`
Using Babel for Transpilation:
- Ensure that your Babel configuration supports both CommonJS and ES module syntax:
“`json
{
“presets”: [
“@babel/preset-env”
],
“plugins”: [
“@babel/plugin-transform-modules-commonjs”
]
}
“`
Sample Jest Configuration for Mixed Modules:
“`json
“jest”: {
“moduleNameMapper”: {
“^@/(.*)$”: “
},
“transform”: {
“^.+\\.(js|jsx|ts|tsx|cjs)$”: “babel-jest”
},
“extensionsToTreatAsEsm”: [“.ts”, “.tsx”]
}
“`
By carefully configuring Jest and ensuring your file structures align with the expected formats, you can mitigate unexpected token errors effectively.
Expert Insights on Resolving Jest Modulenamemapper Issues
Dr. Emily Carter (Software Testing Specialist, Code Quality Institute). “The error message ‘Jest encountered an unexpected token’ typically indicates that Jest is unable to parse a file due to its module format. When dealing with a CJS (CommonJS) folder, ensure that your Jest configuration is set to handle the module resolution correctly. Utilizing the `moduleNameMapper` option in your Jest config can help redirect module paths effectively.”
Michael Thompson (Senior JavaScript Engineer, DevOps Innovations). “When you encounter issues with Jest and CJS folders, it is crucial to check your Babel or TypeScript configurations. Ensure that the transpilation settings are compatible with Jest’s expectations. A misconfigured setup can lead to unexpected token errors, especially when mixing module types.”
Sarah Kim (Lead QA Engineer, Agile Testing Group). “To troubleshoot the ‘unexpected token’ error in Jest, I recommend examining the file extensions and ensuring that Jest is configured to process the relevant file types. Adding proper transformations in the Jest config can mitigate these issues, particularly when working with mixed module formats in your project.”
Frequently Asked Questions (FAQs)
What is Jest’s moduleNameMapper?
Jest’s moduleNameMapper is a configuration option that allows you to define custom mappings for module paths. This is particularly useful for resolving paths to modules that may not be directly accessible or when using non-standard file extensions.
Why am I encountering an “Unexpected Token” error in a CJS folder?
An “Unexpected Token” error typically indicates that Jest is attempting to parse a file that contains syntax not supported by the current parser. This often occurs when using ES modules in a CommonJS (CJS) environment without proper transpilation.
How can I resolve the “Unexpected Token” error in Jest?
To resolve this error, ensure that your Babel or TypeScript configuration is set up correctly to transpile your code. Additionally, verify that your Jest configuration includes the appropriate transform settings for handling modern JavaScript syntax.
What should I include in my Jest configuration to handle CJS and ESM?
In your Jest configuration, include a transform property that specifies how to process files. For example, use Babel or ts-jest to transpile ES modules and ensure that the moduleNameMapper is set to correctly resolve paths for both CJS and ESM.
Can I use Jest with TypeScript in a CJS environment?
Yes, Jest can be used with TypeScript in a CJS environment. Ensure that you have ts-jest configured as the transformer in your Jest setup, and that your tsconfig.json is configured to output CommonJS modules.
What are the common causes of module resolution issues in Jest?
Common causes of module resolution issues in Jest include incorrect module paths, missing dependencies, misconfigured moduleNameMapper settings, and syntax errors in the files being tested. Always check your configuration and ensure that all necessary modules are installed.
In summary, the issue of encountering an unexpected token error in Jest, particularly when dealing with module name mapping and CJS (CommonJS) folders, often arises due to configuration mismatches or syntax errors in the codebase. Jest, a popular testing framework for JavaScript applications, relies on proper configuration to accurately interpret and execute test files. When the module name mapper is not correctly set up to handle specific paths or file types, it can lead to unexpected behavior, including syntax errors that disrupt the testing process.
One of the key takeaways from this discussion is the importance of ensuring that the Jest configuration aligns with the project’s structure and the types of modules being used. Developers should pay close attention to the `moduleNameMapper` option in the Jest configuration file, as it allows for the mapping of module paths, which can help Jest resolve imports correctly. Additionally, using Babel or TypeScript can introduce specific configurations that need to be accounted for in Jest to avoid syntax issues.
Furthermore, it is essential to regularly check for updates in both Jest and any related dependencies, as improvements and bug fixes can influence how modules are handled. By maintaining an up-to-date environment and thoroughly reviewing configuration settings, developers can mitigate the risk of encountering unexpected token
Author Profile
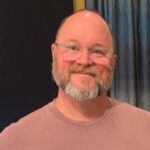
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?