Why Is My Jest Config Custom Environment Not Working: Troubleshooting ‘Cannot Be Found’ Issues?
In the ever-evolving landscape of JavaScript testing, Jest has emerged as a powerful tool that developers rely on to ensure their code runs smoothly and efficiently. However, as with any robust framework, users often encounter challenges that can hinder their testing experience. One such issue is the struggle with configuring custom environments, particularly when the test environment fails to work as expected or cannot be found. This article delves into the intricacies of Jest’s configuration, offering insights and solutions to help you navigate these common pitfalls and optimize your testing setup.
When working with Jest, the ability to customize your testing environment is crucial for simulating real-world scenarios and ensuring comprehensive test coverage. However, misconfigurations can lead to frustrating errors, such as the inability to locate the specified test environment. Understanding the nuances of Jest’s configuration files, including `jest.config.js` and the various environment options available, is essential for overcoming these obstacles.
In this exploration, we will highlight common reasons why your custom test environment might not be functioning correctly, as well as practical strategies to troubleshoot and resolve these issues. Whether you’re a seasoned developer or just starting your journey with Jest, this guide will equip you with the knowledge needed to enhance your testing workflow and achieve seamless integration of custom environments.
Understanding Jest Configuration for Custom Environments
When using Jest, customizing the testing environment can be essential for certain projects, particularly those that utilize specific frameworks or libraries. However, issues may arise if the configuration is not set up correctly. Here, we will delve into common pitfalls and their solutions.
Common Configuration Issues
A frequent error encountered is the failure to recognize the custom environment specified in the Jest configuration. Below are several reasons why this might occur:
- Path Issues: The custom environment file’s path may not be correctly specified. Ensure that the relative or absolute path to the environment file is accurate.
- File Naming: The custom environment file must be named correctly and should have the appropriate file extension (e.g., `.js`, `.ts` for TypeScript).
- Exporting the Environment: The custom environment must be properly exported. It should extend Jest’s `NodeEnvironment` or `JsDomEnvironment` correctly.
- Dependencies: Ensure that all dependencies required by the custom environment are installed and correctly set up.
Setting Up a Custom Environment
To set up a custom environment in Jest, follow these steps:
- Create the Custom Environment File: Write your custom environment logic in a JavaScript or TypeScript file. For instance, create a file named `MyCustomEnvironment.js`.
- Configure Jest to Use the Custom Environment: Modify the `jest.config.js` or `package.json` to include your custom environment.
Example configuration in `jest.config.js`:
“`javascript
module.exports = {
testEnvironment: “
};
“`
- Export Your Environment: Ensure your custom environment is exported correctly:
“`javascript
const NodeEnvironment = require(‘jest-environment-node’);
class MyCustomEnvironment extends NodeEnvironment {
async setup() {
await super.setup();
// Your setup code here
}
async teardown() {
// Your teardown code here
await super.teardown();
}
}
module.exports = MyCustomEnvironment;
“`
Troubleshooting Steps
If your custom environment is still not being recognized, consider these troubleshooting steps:
- Verify Configuration: Double-check the Jest configuration file to ensure the `testEnvironment` property is set correctly.
- Check Console Logs: Run Jest with the `–debug` flag to see detailed logs, which can help identify where the failure occurs.
- Update Jest: Ensure you are using the latest version of Jest, as improvements and bug fixes are continually released.
- Clear Cache: Sometimes, Jest may have cached previous configurations. Clear the cache using `jest –clearCache`.
Example of Jest Configuration
Below is a sample Jest configuration file with a custom environment:
“`javascript
module.exports = {
preset: ‘ts-jest’,
testEnvironment: “
transform: {
‘^.+\\.(ts|tsx)$’: ‘ts-jest’,
},
testRegex: ‘(/__tests__/.*|(\\.|/)(test|spec))\\.(jsx?|tsx?)$’,
moduleFileExtensions: [‘ts’, ‘tsx’, ‘js’, ‘jsx’, ‘json’, ‘node’],
};
“`
This configuration file indicates that the custom environment is located at the specified path and includes other common Jest settings.
Property | Description |
---|---|
preset | Specifies the preset configuration for Jest, often used with TypeScript. |
testEnvironment | Path to the custom environment file. |
transform | Defines how files should be transformed before testing. |
testRegex | Regex pattern to find test files. |
moduleFileExtensions | Extensions of the files that Jest will look for. |
Understanding Jest Custom Environments
Jest allows users to define custom test environments to facilitate various testing scenarios. A custom environment can be particularly useful when the default settings do not meet specific requirements. However, issues may arise when the custom environment is not recognized or cannot be found by Jest.
Common Reasons for Environment Not Found Errors
Several factors can lead to the “custom environment not found” error:
- Incorrect Path: The path specified in the Jest configuration might be incorrect.
- Missing Environment Module: The custom environment module may not be defined or exported correctly.
- Configuration Issues: Jest might not be properly configured to recognize the custom environment.
- Version Compatibility: Ensure that the Jest version supports custom environments.
Configuring a Custom Test Environment
To set up a custom test environment in Jest, follow these steps:
- Create the Custom Environment: Define your environment by extending `NodeEnvironment` or another base class.
“`javascript
const NodeEnvironment = require(‘jest-environment-node’);
class CustomEnvironment extends NodeEnvironment {
async setup() {
await super.setup();
// Custom setup logic here
}
async teardown() {
// Custom teardown logic here
await super.teardown();
}
}
module.exports = CustomEnvironment;
“`
- Update Jest Configuration: Modify your `jest.config.js` to include the custom environment.
“`javascript
module.exports = {
testEnvironment: ‘
};
“`
- Verify the Configuration: Ensure the path is correct and the module is being loaded.
Debugging Steps for Configuration Issues
If you encounter issues with the custom environment, consider the following debugging steps:
- Check the Path: Confirm the path specified in the configuration matches the actual file location.
- Console Logging: Add `console.log()` statements in your environment file to trace execution.
- Run Jest with Verbose Flag: Use the `–verbose` flag to get more detailed error messages.
“`bash
jest –verbose
“`
- Review Package.json: Ensure that Jest is listed correctly in your `package.json` dependencies.
Example of a Complete Jest Configuration
Here’s an example of a complete Jest configuration that utilizes a custom environment:
“`javascript
module.exports = {
testEnvironment: ‘
setupFilesAfterEnv: [‘
testPathIgnorePatterns: [‘/node_modules/’, ‘/dist/’],
transform: {
‘^.+\\.jsx?$’: ‘babel-jest’,
},
moduleFileExtensions: [‘js’, ‘jsx’, ‘json’, ‘node’],
};
“`
Ensure all paths and settings align with your project structure to avoid errors.
Additional Resources and Tools
Consider utilizing these tools and resources for better configuration management:
- Documentation: Refer to the [Jest Documentation](https://jestjs.io/docs/configuration) for detailed configuration options.
- Community Forums: Engage with community forums such as Stack Overflow for troubleshooting.
- Linting Tools: Use ESLint to catch syntax and path errors early in the development process.
By following these guidelines, you should be able to configure and troubleshoot custom environments in Jest effectively.
Resolving Jest Config Custom Environment Issues
Jessica Tran (Senior Software Engineer, Testing Innovations Inc.). “When encountering issues with Jest configuration for custom environments, it is crucial to ensure that the environment is correctly specified in the Jest configuration file. Often, developers overlook the need for the environment to be properly imported or installed, which can lead to Jest not recognizing it.”
Michael Chen (Lead Developer Advocate, Open Source Testing). “A common pitfall when configuring custom environments in Jest is the path resolution. Ensure that the paths defined in your Jest config accurately reflect the directory structure of your project. If Jest cannot locate the specified environment, it will throw an error indicating that it cannot be found.”
Sarah Patel (Quality Assurance Specialist, CodeGuardians). “In my experience, issues with Jest custom environments often stem from version mismatches. Always verify that the versions of Jest and any associated plugins or environments are compatible. Running `npm outdated` can help identify any discrepancies that may be causing the configuration to fail.”
Frequently Asked Questions (FAQs)
What is a custom environment in Jest?
A custom environment in Jest allows developers to define specific settings and behaviors for their test environment, facilitating tailored testing scenarios that differ from the default environment.
How do I configure a custom environment in Jest?
To configure a custom environment in Jest, specify the environment in your Jest configuration file (e.g., `jest.config.js`) using the `testEnvironment` property, pointing it to your custom environment module.
Why is my custom environment not being recognized by Jest?
If your custom environment is not recognized, ensure that the path to the environment module is correct and that the module exports a valid Jest environment class. Additionally, check for any typos in your configuration file.
What should I do if Jest cannot find my custom test environment?
If Jest cannot find your custom test environment, verify that the module is installed correctly, the path is accurate, and that the module exports the necessary Jest environment class. Running Jest with the `–showConfig` option can help diagnose configuration issues.
Are there any common mistakes when setting up a custom environment in Jest?
Common mistakes include incorrect paths to the environment module, failing to export the environment class properly, and misconfiguring the Jest configuration file. Always double-check these elements for accuracy.
How can I debug issues related to custom environments in Jest?
To debug issues, utilize Jest’s verbose logging options, check the console for error messages, and ensure that your environment module is functioning correctly by testing it independently. Additionally, reviewing Jest’s documentation can provide insights into proper configuration.
In summary, the issue of a custom test environment not being recognized in Jest configurations can stem from several factors. Users often encounter difficulties when attempting to set up a custom environment, particularly when the environment is not properly defined or when Jest is unable to locate the specified environment module. This can lead to errors during the test execution phase, resulting in a failure to run tests as intended.
Key takeaways from this discussion include the importance of ensuring that the custom environment is correctly referenced in the Jest configuration file. This involves verifying the path to the environment module and ensuring that it is correctly exported. Additionally, users should check that their Jest version supports the features they are attempting to implement, as compatibility issues can also contribute to the problem.
Moreover, it is advisable to consult the Jest documentation for any updates or changes regarding custom environments. Engaging with the community through forums or GitHub discussions can also provide insights and solutions from other developers who may have faced similar challenges. By following these best practices, users can enhance their Jest testing experience and effectively utilize custom environments in their testing workflows.
Author Profile
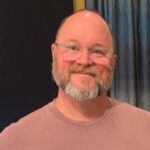
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?