How Can I Create a Javascript Accordion That Allows Only One Section to Be Open at a Time?
In the realm of web development, user experience is paramount, and one of the most effective ways to enhance it is through the use of accordions. These interactive elements allow users to expand and collapse content sections, making information more digestible and organized. However, a common challenge arises when multiple accordion panels are open simultaneously, leading to a cluttered interface that can overwhelm users. This article delves into the elegant solution of creating a JavaScript accordion that ensures only one panel is open at any given time, streamlining the user experience and maintaining a clean layout.
Accordions are not just a trend; they are a functional design choice that can significantly improve how users interact with content-heavy websites. By implementing a JavaScript solution that restricts users to a single open panel, developers can guide users through information in a structured manner. This approach not only enhances usability but also encourages users to engage more deeply with the content presented.
As we explore the mechanics behind this functionality, we will cover the essential JavaScript techniques and best practices that make it possible to create a seamless accordion experience. Whether you’re a seasoned developer or just starting your journey in web design, understanding how to implement this feature will empower you to create more intuitive and user-friendly interfaces. Get ready to transform
Understanding the Accordion Structure
An accordion is a user interface component that allows for the expansion and contraction of sections of content, making it a popular choice for displaying large amounts of information in a compact format. When implementing an accordion where only one section remains open at a time, it is essential to structure the HTML elements appropriately to facilitate this behavior.
Typically, an accordion consists of:
- A container for all accordion items
- Individual items, each containing a header and content
- A mechanism (JavaScript) to control the opening and closing of sections
Below is a simple HTML structure for an accordion:
“`html
Item 1
Item 2
Item 3
“`
JavaScript Implementation
To ensure that only one accordion item is open at a time, you can utilize JavaScript to handle click events on the headers. The following script demonstrates a straightforward approach:
“`javascript
document.querySelectorAll(‘.accordion-header’).forEach(header => {
header.addEventListener(‘click’, function() {
const content = this.nextElementSibling;
const isActive = content.style.display === ‘block’;
// Close all contents
document.querySelectorAll(‘.accordion-content’).forEach(item => {
item.style.display = ‘none’;
});
// Open the clicked one if it was not already open
if (!isActive) {
content.style.display = ‘block’;
}
});
});
“`
This code performs the following functions:
- Listens for clicks on each accordion header.
- Toggles the visibility of the corresponding content.
- Ensures that all other sections are closed when a new section is opened.
Styling the Accordion
To enhance the user experience, CSS plays a vital role in styling the accordion. Below is a sample CSS snippet to improve the visual appeal:
“`css
.accordion {
border: 1px solid ddd;
border-radius: 4px;
}
.accordion-item {
border-bottom: 1px solid ddd;
}
.accordion-header {
background-color: f1f1f1;
padding: 10px;
cursor: pointer;
transition: background-color 0.3s;
}
.accordion-header:hover {
background-color: e0e0e0;
}
.accordion-content {
display: none;
padding: 10px;
}
“`
This CSS code provides:
- A subtle border around the accordion.
- Distinct styling for headers and content.
- A hover effect to indicate interactivity.
Example of Accordion Behavior
The following table summarizes the expected behavior of the accordion when interacting with it:
Action | Accordion State |
---|---|
Click Header 1 | Item 1 opens, others close |
Click Header 2 | Item 2 opens, Item 1 closes |
Click Header 3 | Item 3 opens, Item 2 closes |
Click Header 1 Again | Item 1 closes |
In this setup, the accordion maintains a clean and organized interface while ensuring that users can only focus on one section of content at a time, promoting clarity and usability.
Creating an Accordion with JavaScript
To implement an accordion where only one section is open at a time, you can utilize a combination of HTML, CSS, and JavaScript. This approach ensures a smooth user experience and maintains a clean UI.
HTML Structure
The HTML structure for the accordion involves a series of buttons and content sections. Each button toggles the visibility of its corresponding content.
“`html
Section 1
Section 2
Section 3
“`
CSS Styling
The CSS styles will enhance the visual appearance of the accordion. It is essential to hide the content sections by default and style the headers.
“`css
.accordion {
border: 1px solid ccc;
border-radius: 5px;
}
.accordion-header {
background-color: f1f1f1;
cursor: pointer;
padding: 10px;
border: 1px solid ccc;
margin: 0;
}
.accordion-content {
display: none;
padding: 10px;
border-top: 1px solid ccc;
}
“`
JavaScript Functionality
The JavaScript will manage the toggling of sections. By ensuring that only one section is open at a time, we can enhance usability.
“`javascript
document.querySelectorAll(‘.accordion-header’).forEach(header => {
header.addEventListener(‘click’, function() {
const currentlyActive = document.querySelector(‘.accordion-header.active’);
const content = this.nextElementSibling;
if (currentlyActive && currentlyActive !== this) {
currentlyActive.classList.remove(‘active’);
currentlyActive.nextElementSibling.style.display = ‘none’;
}
this.classList.toggle(‘active’);
content.style.display = content.style.display === ‘block’ ? ‘none’ : ‘block’;
});
});
“`
Accessibility Considerations
When implementing an accordion, it is vital to ensure it is accessible to all users. Here are some best practices:
- Use ARIA roles: Add `role=”button”` to headers and `role=”region”` to content sections.
- Keyboard navigation: Ensure users can navigate using the keyboard.
- Focus management: Set focus to the currently opened section for screen readers.
“`html
Section 1
“`
Testing the Accordion
Testing your accordion is crucial. Verify the following:
- Only one section opens at a time.
- Clicking on the active header closes the section.
- All content is accessible via keyboard navigation.
- Screen readers correctly announce the changes in content visibility.
By following these guidelines, you can create an efficient and user-friendly accordion that maintains a clean interface while providing a seamless interaction experience.
Expert Insights on Implementing a JavaScript Accordion with Single Open Functionality
Jessica Lin (Front-End Developer, CodeCraft Solutions). “Implementing an accordion that allows only one section to be open at a time is essential for maintaining a clean user interface. This can be achieved by ensuring that when a new section is opened, all others are closed programmatically. Utilizing event listeners effectively can streamline this process.”
Michael Chen (UI/UX Designer, Creative Interfaces). “From a user experience perspective, having only one accordion panel open at a time helps prevent information overload. It encourages users to focus on one piece of content at a time, which can significantly enhance their interaction with the interface.”
Sarah Patel (JavaScript Engineer, Tech Innovations Inc.). “To implement a JavaScript accordion that restricts multiple sections from being open simultaneously, developers should leverage the `classList` API for toggling classes. This approach not only simplifies the code but also enhances performance by reducing unnecessary DOM manipulations.”
Frequently Asked Questions (FAQs)
What is a JavaScript accordion?
A JavaScript accordion is a UI component that allows users to expand and collapse sections of content. It typically displays a list of items, where only one section can be opened at a time, enhancing user experience by organizing information efficiently.
How can I implement an accordion that allows only one section to be open at a time?
To implement such an accordion, you can use JavaScript to add event listeners to each section’s header. When a header is clicked, the script should close any currently open sections before opening the clicked one, ensuring only one section remains expanded.
What JavaScript methods are commonly used to create an accordion?
Common methods include `addEventListener` for handling click events, `classList.toggle` to add or remove CSS classes for showing or hiding content, and `querySelector` or `getElementById` for selecting DOM elements.
Can I create a JavaScript accordion using only CSS?
While CSS alone can create a basic accordion using the `:checked` pseudo-class with input elements, it lacks the flexibility and control that JavaScript provides for managing open/close states dynamically.
What are some best practices for creating a JavaScript accordion?
Best practices include ensuring accessibility by using appropriate ARIA roles, providing clear visual indicators for open and closed states, and optimizing performance by minimizing DOM manipulations and event listeners.
How do I style a JavaScript accordion for better user experience?
To enhance user experience, use CSS transitions for smooth opening and closing animations, provide contrasting colors for active and inactive sections, and ensure that the accordion is responsive to different screen sizes.
implementing a JavaScript accordion that allows only one section to be open at a time is a valuable feature for enhancing user experience on web pages. This functionality prevents information overload by ensuring that users can focus on one item at a time. By utilizing event listeners and managing the active states of the accordion panels, developers can create a clean and efficient interface that promotes better navigation and readability.
One of the key takeaways from this discussion is the importance of maintaining a clear structure in the code. By organizing the JavaScript logic to handle the opening and closing of accordion sections systematically, developers can ensure that the functionality remains robust and easy to maintain. Additionally, employing best practices such as using data attributes can simplify the process of targeting specific elements within the DOM.
Furthermore, it is essential to consider accessibility when designing accordions. Implementing keyboard navigation and ensuring that the accordion is usable with screen readers can significantly improve the user experience for individuals with disabilities. By prioritizing accessibility, developers not only comply with legal standards but also create a more inclusive web environment.
Author Profile
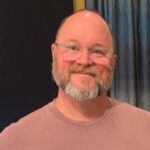
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?