How Can You Resolve the ‘Java Virtual Machine Launcher: A Java Exception Has Occurred’ Error?
In the world of Java programming, encountering errors is an inevitable part of the development journey. One of the more perplexing and frustrating issues developers face is the dreaded “Java Virtual Machine Launcher: A Java Exception Has Occurred” message. This seemingly cryptic notification can halt your projects in their tracks, leaving you grappling with the underlying causes and potential solutions. Whether you’re a seasoned developer or a newcomer to the Java ecosystem, understanding this error is crucial for maintaining productivity and ensuring smooth application performance.
The Java Virtual Machine (JVM) is the backbone of Java applications, enabling them to run on any platform that supports Java. However, when the JVM encounters an exception, it can trigger a cascade of problems that disrupt your workflow. This error might arise from various factors, including configuration issues, incompatible libraries, or even environmental problems. As such, recognizing the symptoms and understanding the context of this error is essential for diagnosing and resolving the issue effectively.
In this article, we will delve into the common causes of the “Java Exception Has Occurred” error and explore practical troubleshooting techniques to help you get back on track. By equipping yourself with the knowledge to tackle this error head-on, you can enhance your Java development experience and minimize downtime, allowing you to focus on
Common Causes of the Error
The “Java Exception Has Occurred” error can stem from various issues, often related to the Java Runtime Environment (JRE) or Java Development Kit (JDK). Understanding these causes can help in troubleshooting the problem effectively. Some common causes include:
- Corrupted Installation: A damaged or incomplete installation of Java can lead to various runtime errors.
- Insufficient Memory: If the system runs out of memory while executing a Java application, an exception may be triggered.
- Incompatible Java Version: Running applications built for a different version of Java can result in compatibility issues.
- Java Environment Variables: Incorrect configurations of environment variables such as `JAVA_HOME` or `PATH` can lead to execution failures.
Troubleshooting Steps
To resolve the “Java Exception Has Occurred” error, you can follow these troubleshooting steps:
- Reinstall Java: Uninstall the current version of Java and download the latest version from the official website. Ensure a clean installation by removing residual files.
- Check Memory Allocation: Monitor system memory usage to ensure sufficient resources are available. You can increase the memory allocation for Java applications by modifying the JVM options.
- Update Environment Variables: Verify that the `JAVA_HOME` and `PATH` variables are correctly set to the directory of the Java installation.
- Run in Compatibility Mode: If using an older application, try running it in compatibility mode for an earlier version of Windows.
- Check for Updates: Ensure that your operating system and all related software are updated to the latest versions.
Java Exception Handling
Java provides a robust exception handling mechanism that allows developers to manage errors gracefully. The key components of this mechanism include:
- Try-Catch Block: Encloses code that may throw exceptions, allowing the program to continue execution even when an error occurs.
- Finally Block: Executes code after try and catch blocks, regardless of whether an exception occurred, making it useful for cleanup activities.
Here is a simple example of a try-catch block in Java:
“`java
try {
// Code that may throw an exception
int result = 10 / 0; // This will throw ArithmeticException
} catch (ArithmeticException e) {
System.out.println(“An error occurred: ” + e.getMessage());
} finally {
System.out.println(“Execution completed.”);
}
“`
Key Takeaways
To summarize the main points regarding the “Java Exception Has Occurred” error, the following table highlights the causes and solutions:
Cause | Solution |
---|---|
Corrupted Installation | Reinstall Java |
Insufficient Memory | Check memory allocation |
Incompatible Java Version | Run compatible versions |
Incorrect Environment Variables | Update JAVA_HOME and PATH |
By addressing these common issues and following best practices for exception handling, developers can minimize the occurrence of errors and improve the stability of their Java applications.
Understanding the Error Message
The error message “A Java Exception Has Occurred” during the Java Virtual Machine (JVM) launch indicates that the JVM encountered a problem while trying to execute a Java application. This could stem from various issues ranging from configuration errors to incompatible Java versions.
Common causes of this error include:
- Corrupted Java installation: Files may be missing or damaged.
- Incompatible Java version: The application may require a specific version of Java.
- Insufficient memory: The system may not have enough memory allocated to run the Java application.
- Environment variable issues: Incorrectly set JAVA_HOME or PATH variables can lead to this error.
Troubleshooting Steps
To resolve the “A Java Exception Has Occurred” error, follow these troubleshooting steps:
- Verify Java Installation:
- Check if Java is correctly installed by running `java -version` in the command prompt.
- If Java is not installed or is the wrong version, download and install the latest version from the [official Java website](https://www.java.com).
- Check Environment Variables:
- Ensure that the JAVA_HOME variable points to the correct Java installation directory.
- Update the PATH variable to include the Java `bin` directory.
- Example settings:
- JAVA_HOME: `C:\Program Files\Java\jdk-xx.x.x`
- PATH: `C:\Program Files\Java\jdk-xx.x.x\bin`
- Allocate More Memory:
- If the application requires more memory, adjust the JVM memory settings. This can often be done using command line options:
- `-Xms` (initial heap size)
- `-Xmx` (maximum heap size)
- Example command: `java -Xms512m -Xmx1024m -jar YourApplication.jar`
- Reinstall Java:
- If other steps fail, consider uninstalling and reinstalling Java to ensure all components are intact.
Common Java Exception Types
Various exceptions may trigger the JVM error. Understanding these can help in diagnosing the underlying issue:
Exception Type | Description |
---|---|
`NullPointerException` | Attempting to use an object reference that has not been initialized. |
`ClassNotFoundException` | The JVM cannot find the specified class during execution. |
`OutOfMemoryError` | The Java application has exceeded the allocated memory limit. |
`IOException` | An error occurs during input or output operations. |
Logging and Debugging
To gain further insight into the error, enable logging and debugging features:
- Use the `-verbose:class` option to log class loading information.
- Redirect error output to a file using:
“`shell
java -jar YourApplication.jar > error.log 2>&1
“`
- Review the log file for specific exceptions or stack traces that can help identify the problem.
Consulting Documentation and Forums
If the issue persists, consult the following resources:
- Official Java Documentation: Provides comprehensive guidance on JVM errors and configurations.
- Stack Overflow: A vast community where users share solutions for similar issues.
- Java User Groups: Local or online groups where developers can seek assistance from peers.
By systematically following these steps, users can effectively troubleshoot and resolve the “A Java Exception Has Occurred” error, allowing for successful execution of Java applications.
Expert Insights on Java Virtual Machine Launcher Exceptions
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘A Java Exception Has Occurred’ typically indicates an issue with the Java Virtual Machine (JVM) configuration or the application itself. It is essential to check the JVM arguments and ensure that all required libraries are correctly referenced in the classpath.”
Mark Thompson (Java Development Specialist, CodeCraft Solutions). “When encountering this exception, developers should first examine the stack trace for specific error messages. Often, the root cause can be traced back to incompatible versions of Java or missing dependencies that the application relies on.”
Linda Zhao (Java Architect, FutureTech Systems). “In my experience, many Java exceptions stem from memory management issues within the JVM. It is advisable to monitor heap usage and consider adjusting the JVM’s memory settings to prevent such exceptions from occurring during runtime.”
Frequently Asked Questions (FAQs)
What does the error “A Java Exception Has Occurred” mean?
This error indicates that the Java Virtual Machine (JVM) encountered an unexpected condition that prevented it from executing properly. It typically signifies a problem with the Java application or its environment.
What are common causes of the “A Java Exception Has Occurred” error?
Common causes include incompatible Java versions, corrupted Java installation, insufficient system resources, or issues within the Java application itself, such as bugs or misconfigurations.
How can I troubleshoot the “A Java Exception Has Occurred” error?
To troubleshoot, ensure that you are using the correct Java version for your application, reinstall Java, check for updates, verify system resource availability, and review application logs for specific error messages.
Can I fix the error by reinstalling Java?
Yes, reinstalling Java can resolve the error if it is caused by a corrupted installation. Ensure you completely uninstall the previous version before installing the latest version.
Is there a way to get more details about the exception?
Yes, you can enable detailed logging in the Java application or check the console output for stack traces and error messages that provide more context about the exception.
What should I do if the error persists after troubleshooting?
If the error persists, consider reaching out to the application’s support team or forums for assistance, as there may be specific issues related to the application that require expert intervention.
The Java Virtual Machine (JVM) Launcher is a crucial component in the execution of Java applications. When users encounter the error message “A Java Exception Has Occurred,” it typically indicates that an issue has arisen during the initialization or execution of a Java program. This error can stem from various sources, including incorrect configurations, incompatible Java versions, or missing dependencies. Understanding the context of this error is essential for effective troubleshooting and resolution.
Key insights into resolving the “A Java Exception Has Occurred” error include verifying the Java installation and ensuring that the correct version is being used for the application. Users should also check for any updates or patches that may address known issues. Additionally, reviewing the application’s configuration settings and dependencies can help identify potential conflicts or missing components that could lead to this exception.
In summary, the occurrence of a Java exception during the JVM launch process highlights the importance of maintaining a properly configured Java environment. By systematically addressing potential causes and ensuring compatibility between the Java Runtime Environment and the application, users can minimize disruptions and enhance the stability of their Java applications. Effective troubleshooting not only resolves immediate issues but also contributes to a better understanding of Java application management.
Author Profile
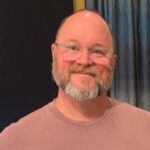
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?