How Can Java Leverage SAML Protocol to Access Salesforce APIs?
In today’s interconnected digital landscape, the need for secure and efficient authentication methods has never been more critical. As organizations increasingly turn to cloud-based services, the Security Assertion Markup Language (SAML) protocol has emerged as a robust solution for facilitating single sign-on (SSO) across various applications. For developers looking to integrate Salesforce APIs into their Java applications, understanding how to leverage SAML for authentication is essential. This article delves into the intricacies of using SAML with Java to streamline access to Salesforce’s powerful suite of APIs, enabling developers to enhance user experiences while maintaining stringent security standards.
When integrating Salesforce APIs, developers often face the challenge of ensuring that their applications authenticate users seamlessly and securely. SAML provides a framework that allows for federated authentication, enabling users to log in once and gain access to multiple services without repeated credential prompts. By utilizing SAML in Java applications, developers can harness this capability to create a smooth user experience while adhering to enterprise security policies.
This overview will guide you through the fundamental concepts of SAML and its implementation in Java, particularly in the context of Salesforce API consumption. As we explore the various components and workflows involved, you’ll gain insights into how SAML not only simplifies authentication but also enhances the overall security posture of your
Configuring SAML in Java for Salesforce API Access
To integrate SAML authentication for accessing Salesforce APIs via Java, you must configure both your Salesforce environment and your Java application properly. This involves setting up the Salesforce connected app for SAML and configuring the Java application to send SAML assertions.
Salesforce Connected App Configuration
- Log in to your Salesforce account and navigate to Setup.
- In the Quick Find box, type Apps and select App Manager.
- Click New Connected App and fill in the required fields:
- Connected App Name: A unique name for your app.
- API Name: Auto-generated, but can be customized.
- Contact Email: Your email for app-related notifications.
- Under API (Enable OAuth Settings), check Enable OAuth Settings and fill in:
- Callback URL: The URL where Salesforce sends the response.
- Selected OAuth Scopes: Choose appropriate scopes such as `Access and manage your data (API)`.
- Under SAML Settings, check Enable SAML and fill in:
- Issuer: The entity ID for your application.
- Entity ID: A unique identifier for the Salesforce service provider.
- ACS URL: The Assertion Consumer Service URL of your Java application.
- Save the connected app and note the Consumer Key and Consumer Secret for later use.
Java Application Configuration
In your Java application, you will need to handle SAML assertions. This typically involves using libraries like OpenSAML or Spring Security SAML. Below is an outline of the key steps:
- Generate SAML Assertion: Create an assertion that includes user identity and attributes.
- Sign the Assertion: Use your private key to sign the SAML assertion.
- Send SAML Assertion to Salesforce: Redirect the user to Salesforce with the signed assertion.
A basic code snippet might look like this:
“`java
// Example of generating a SAML assertion
SAMLAssertion assertion = new SAMLAssertion();
assertion.setIssuer(“your-issuer”);
assertion.setSubject(“[email protected]”);
// Additional attributes can be added here
“`
Handling the SAML Response
Once the user is redirected back to your application with the SAML response, you will need to parse and validate it. This involves:
- Verifying the signature of the SAML response.
- Ensuring the response is not expired.
- Extracting user details from the assertion.
You can create a method to handle the SAML response as follows:
“`java
public void handleSAMLResponse(String samlResponse) {
// Decode and validate the response
// Extract user information
}
“`
Common Issues and Troubleshooting
When implementing SAML with Salesforce APIs in Java, you may encounter several issues:
- Invalid Signature: Ensure that your certificate is correctly configured in both Salesforce and your Java application.
- Expired Assertions: Verify the timestamps in your assertions.
- Misconfigured ACS URL: Double-check the Assertion Consumer Service URL in Salesforce settings.
Issue | Possible Cause | Solution |
---|---|---|
Invalid Signature | Certificate mismatch | Check certificate settings in both systems |
Expired Assertions | Time synchronization issues | Ensure both servers are synchronized |
Misconfigured ACS URL | Incorrect URL in Salesforce | Update the URL in the connected app settings |
By carefully following these configurations and troubleshooting steps, you can successfully implement SAML authentication in your Java application to consume Salesforce APIs.
Understanding SAML Protocol for Salesforce API Access
The Security Assertion Markup Language (SAML) protocol is essential for enabling single sign-on (SSO) capabilities, allowing secure communication between identity providers (IdP) and service providers (SP), such as Salesforce. To utilize SAML for accessing Salesforce APIs, it’s vital to grasp the underlying concepts and configurations.
Configuring Salesforce for SAML
Before implementing SAML in Java, configure Salesforce to act as a service provider. The following steps outline the necessary setup:
- Enable SAML in Salesforce:
- Navigate to Setup in Salesforce.
- Search for Single Sign-On Settings.
- Click New and select SAML.
- Fill in SAML settings:
- Name: Provide a name for the SSO configuration.
- Issuer: Set this to your IdP’s Entity ID.
- Entity Id: Enter the Salesforce instance URL.
- Assertion Consumer Service URL: Use the Salesforce URL for SAML assertions.
- X.509 Certificate: Upload the public certificate from your IdP.
- Save the configuration and note the SAML endpoint URL for later use in your Java application.
Implementing SAML in Java
To use SAML for authentication in Java, consider the following components:
- Libraries:
- Use libraries such as OpenSAML or Spring Security SAML to handle SAML assertions and responses.
- Process Overview:
- Redirect the user to the IdP for authentication.
- The IdP authenticates the user and sends a SAML response back to Salesforce.
- Salesforce processes the SAML assertion, allowing access to its APIs.
Sample Java Code for SAML Authentication
The following code snippet demonstrates a basic implementation of SAML authentication in Java:
“`java
import org.opensaml.saml2.core.Response;
import org.opensaml.security.credential.Credential;
import org.opensaml.security.credential.BasicCredential;
// Initialize SAML response handling
Response samlResponse = // obtain SAML response from IdP
String assertionConsumerServiceURL = “https://your-salesforce-instance.com”;
// Validate the SAML response
if (validateSAMLResponse(samlResponse)) {
// Process user session and API calls
String accessToken = getAccessToken(samlResponse);
// Use access token to call Salesforce APIs
} else {
throw new SecurityException(“Invalid SAML Response”);
}
“`
Calling Salesforce APIs with Access Token
Once authenticated, you can access Salesforce APIs using the obtained access token. The following example demonstrates how to make a simple API call:
- Endpoint: `https://your-salesforce-instance.com/services/data/vXX.X/sobjects/`
- HTTP Method: `GET`
- Headers:
- `Authorization: Bearer {access_token}`
- `Content-Type: application/json`
“`java
import java.net.HttpURLConnection;
import java.net.URL;
URL url = new URL(“https://your-salesforce-instance.com/services/data/vXX.X/sobjects/”);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod(“GET”);
connection.setRequestProperty(“Authorization”, “Bearer ” + accessToken);
connection.setRequestProperty(“Content-Type”, “application/json”);
int responseCode = connection.getResponseCode();
// Handle the response…
“`
Best Practices for SAML Integration
When integrating SAML for accessing Salesforce APIs, consider these best practices:
- Security: Regularly update certificates and use secure communication protocols (TLS).
- Testing: Thoroughly test your SAML assertions and responses in a sandbox environment.
- Logging: Implement logging to monitor authentication flows and any errors.
- Documentation: Maintain clear documentation for your SAML configurations and Java implementations.
By following these guidelines, you can successfully implement SAML authentication for Salesforce APIs using Java.
Leveraging SAML Protocol in Java for Salesforce API Integration
Dr. Emily Carter (Senior Software Architect, Cloud Solutions Inc.). “Utilizing the SAML protocol in Java applications to authenticate with Salesforce APIs enhances security and streamlines user management. It allows for single sign-on capabilities, which are essential for enterprises looking to simplify access while maintaining robust security measures.”
James Liu (Lead Developer, API Integration Group). “Implementing SAML in Java for Salesforce API consumption not only improves authentication processes but also aligns with modern security standards. By leveraging frameworks like Spring Security, developers can efficiently manage SAML assertions and user sessions, ensuring a seamless integration experience.”
Linda Patel (Cybersecurity Consultant, SecureTech Advisors). “When integrating Salesforce APIs using Java and SAML, it is crucial to prioritize the configuration of trust relationships. Properly setting up identity providers and service providers ensures that the authentication flow is secure, mitigating risks associated with unauthorized access.”
Frequently Asked Questions (FAQs)
What is SAML and how is it used with Salesforce APIs?
SAML (Security Assertion Markup Language) is an open standard for exchanging authentication and authorization data between parties. In the context of Salesforce APIs, SAML is used to enable Single Sign-On (SSO), allowing users to authenticate once and gain access to various applications without needing to log in multiple times.
How can I implement SAML in a Java application to access Salesforce APIs?
To implement SAML in a Java application, you can use libraries such as OpenSAML or Spring Security SAML. Configure your application as a SAML Service Provider (SP) and set up the necessary metadata to communicate with Salesforce, which acts as the Identity Provider (IdP).
What are the prerequisites for using SAML with Salesforce APIs in Java?
Prerequisites include having a Salesforce account with API access, a Java development environment, and libraries for SAML processing. Additionally, you need to configure a connected app in Salesforce to handle SAML assertions and set up the appropriate permissions.
What are the common challenges when using SAML with Salesforce APIs?
Common challenges include correctly configuring SAML assertions, ensuring the clock skew between the IdP and SP is minimal, and managing user provisioning and de-provisioning. Debugging SAML responses can also be complex due to the XML format and potential security settings.
How do I troubleshoot SAML authentication issues with Salesforce APIs?
To troubleshoot SAML authentication issues, check the SAML assertion for correct attributes and values, verify the certificate used for signing, and ensure the endpoint URLs are correctly configured. Utilize Salesforce’s debug logs and SAML trace tools to identify errors in the authentication flow.
Is it possible to use SAML with other programming languages besides Java for Salesforce APIs?
Yes, SAML can be implemented in various programming languages such as Python, C, and JavaScript. Each language has its own libraries and frameworks to facilitate SAML integration, but the fundamental principles of SAML authentication remain the same across platforms.
In summary, utilizing the SAML protocol in Java applications to consume Salesforce APIs offers a robust solution for secure authentication and authorization. By implementing SAML, developers can leverage single sign-on (SSO) capabilities, allowing users to access Salesforce resources seamlessly while maintaining a high level of security. This integration is particularly beneficial for organizations that require a unified authentication mechanism across multiple applications, enhancing user experience and reducing the management overhead associated with multiple credentials.
Key insights from the discussion highlight the importance of understanding both SAML and Salesforce’s API authentication mechanisms. Developers must familiarize themselves with the configuration of SAML assertions, the role of identity providers, and how to properly set up Salesforce as a service provider. Additionally, the use of libraries such as Spring Security SAML can simplify the implementation process, providing pre-built functionalities that adhere to SAML standards.
Furthermore, it is crucial to ensure that the security aspects of the implementation are thoroughly tested. This includes validating SAML assertions, managing session lifecycles, and handling potential security vulnerabilities. By adhering to best practices in security and utilizing established libraries, developers can create a secure and efficient integration between their Java applications and Salesforce APIs, ultimately leading to improved operational efficiency and user satisfaction.
Author Profile
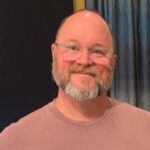
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?