Why Does the ‘Java Package Does Not Exist’ Error Keep Haunting My Code?
In the world of Java programming, encountering errors can be a common yet frustrating experience. One such error that developers often face is the dreaded message: “Java Package Does Not Exist.” This seemingly innocuous notification can halt your coding progress and leave you scratching your head, wondering what went wrong. Understanding the underlying causes of this issue is crucial for any Java developer, whether you’re a seasoned pro or just starting your programming journey. In this article, we will delve into the intricacies of package management in Java, exploring the reasons behind this error and offering insights into how to resolve it effectively.
Overview
At its core, the “Java Package Does Not Exist” error typically arises from issues related to package naming, directory structure, or classpath configurations. When Java cannot locate a specified package, it can disrupt the entire compilation process, leading to confusion and wasted time. This error may stem from simple typos in your code or more complex problems related to project setup and dependencies.
Moreover, understanding Java’s package system is essential for maintaining organized and efficient code. Packages serve as a way to group related classes and interfaces, promoting modularity and reusability. However, when these packages are misconfigured or improperly referenced, it can lead to frustrating roadblocks. By
Understanding the ‘Java Package Does Not Exist’ Error
The ‘Java Package Does Not Exist’ error typically arises when the Java compiler cannot find a package that is referenced in the code. This issue can stem from various factors, including incorrect package declarations, missing libraries, or misconfigured project settings.
Common causes of this error include:
- Incorrect Package Name: The package name in the Java file does not match the directory structure.
- Missing Libraries: Required libraries are not included in the project’s build path.
- IDE Misconfiguration: The Integrated Development Environment (IDE) may not be properly configured to recognize the package.
- Classpath Issues: The classpath may not include the necessary directories or JAR files.
To resolve this error, it is essential to systematically check each potential cause.
Steps to Resolve the Error
- Verify Package Declaration: Ensure that the package declaration at the top of your Java file matches the directory structure of your project.
Example:
“`java
package com.example.myapp;
“`
- Check Build Path: If you are using an IDE like Eclipse or IntelliJ IDEA, check the project’s build path settings to confirm that all necessary libraries are included.
- Adjust Classpath: If compiling from the command line, ensure the classpath is correctly set to include all required directories and JAR files. Use the `-cp` option with the `javac` command to specify the classpath.
Example command:
“`bash
javac -cp “.:lib/*” MyApp.java
“`
- Rebuild the Project: Sometimes, simply cleaning and rebuilding the project can resolve configuration issues.
- Check for Typos: Look for any typographical errors in the package name or file names.
Example of Directory Structure
Understanding the expected directory structure can clarify how packages should be organized. Below is an example of a correct directory layout:
“`
src/
└── com/
└── example/
└── myapp/
├── Main.java
└── Utils.java
“`
In this example, the `Main.java` and `Utils.java` files should both declare the same package:
“`java
package com.example.myapp;
“`
Package Management Tools
Utilizing tools for package management can also help in avoiding such errors. Below are some popular tools and their benefits:
Tool | Benefits |
---|---|
Maven | Dependency management, project structure, and build automation. |
Gradle | Flexible build automation and dependency resolution. |
Ant | Customizable build processes and dependency management. |
Using these tools can streamline the process of managing packages and libraries, reducing the likelihood of encountering the ‘Java Package Does Not Exist’ error. Proper configuration and understanding of your project’s dependencies are crucial in maintaining a smooth development workflow.
Understanding the “Java Package Does Not Exist” Error
The “Java Package Does Not Exist” error typically indicates that the Java compiler or runtime cannot locate a specified package. This may stem from various issues related to classpath configuration, missing libraries, or incorrect package declarations.
Common Causes of the Error
Several factors can lead to this error. Understanding these causes is crucial for effective troubleshooting:
- Incorrect Package Declaration: Ensure that the package declaration at the top of your Java file matches the directory structure of your project. For example, if your package is declared as `package com.example;`, the corresponding directory should be `/com/example/`.
- Classpath Issues: The Java compiler relies on the classpath to locate packages and classes. If the required libraries or directories are not included in the classpath, the error will occur. Check your IDE or build tool configuration to ensure that all necessary paths are included.
- Missing JAR Files: If your project depends on external libraries packaged as JAR files, make sure these files are available in your project’s build path. If a JAR is missing, the compiler will not find the classes it needs.
- Incorrect Build Configuration: If you’re using a build tool like Maven or Gradle, verify that your `pom.xml` or `build.gradle` files are correctly configured and that all dependencies are resolved.
- IDE Configuration Issues: Integrated Development Environments (IDEs) like Eclipse or IntelliJ IDEA might have their own project settings that require adjustment. Ensure that the project settings are correctly pointing to your source folders and libraries.
Troubleshooting Steps
To resolve the “Java Package Does Not Exist” error, follow these steps systematically:
- Check Package Name:
- Confirm that the package name in your Java file matches the directory structure.
- Ensure that there are no typos in the package declaration.
- Verify Classpath Settings:
- In your IDE, check the project properties or preferences to see the classpath configuration.
- For command-line compilation, ensure you use the `-cp` flag to specify the correct classpath.
- Inspect Dependency Management:
- For Maven, run `mvn clean install` to refresh dependencies.
- For Gradle, use `gradle build` to check for dependency issues.
- Add Missing JARs:
- If using an IDE, add the required JAR files to the build path.
- For Maven, add the necessary dependencies to your `pom.xml`.
- Clean and Rebuild:
- Perform a clean build in your IDE to remove any residual files.
- Use commands like `mvn clean` or `gradle clean` as appropriate.
Example Configuration
Below is an example of a basic Maven project structure and its `pom.xml` configuration:
“`plaintext
my-project/
— src/ | ||
---|---|---|
— main/ | ||
— java/ | ||
`– com/ | ||
`– example/ | ||
`– MyClass.java | ||
— pom.xml |
“`
Example `pom.xml`:
“`xml
Correctly structuring your project and configuring your build tools will help you avoid the “Java Package Does Not Exist” error efficiently.
Understanding the ‘Java Package Does Not Exist’ Error
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “The ‘Java Package Does Not Exist’ error typically arises when the Java compiler cannot locate the specified package in the classpath. This issue often stems from incorrect package declarations or missing dependencies in the project setup.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “To resolve the ‘Java Package Does Not Exist’ error, developers should ensure that the package structure in the file system matches the package declaration in the source code. Additionally, verifying that all necessary libraries are included in the build path is crucial.”
Sarah Patel (Java Architect, FutureTech Labs). “This error can also occur during the migration of projects to new environments. It is essential to check the configuration files and ensure that the environment variables are correctly set to include the necessary paths for the Java packages.”
Frequently Asked Questions (FAQs)
What does the error “Java Package Does Not Exist” mean?
This error indicates that the Java compiler cannot find the specified package in the classpath. It typically occurs when the package is not included in the project or the directory structure does not match the package declaration.
How can I resolve the “Java Package Does Not Exist” error?
To resolve this error, ensure that the package is correctly defined in your source files and that the directory structure matches the package name. Additionally, verify that the classpath includes the directory or JAR file containing the package.
What should I check if I have imported the package but still see the error?
If the package is imported but the error persists, check for typos in the package name, confirm that the package is compiled, and ensure that the build path includes the necessary libraries or source folders.
Can this error occur due to IDE configuration issues?
Yes, IDE configuration issues can lead to this error. Ensure that the project settings are correctly configured to include all necessary source folders and libraries in the build path.
Is it possible to encounter this error with third-party libraries?
Yes, this error can occur with third-party libraries if the library is not correctly added to the project dependencies or if the package structure within the library does not match the expected structure.
What tools can help diagnose the “Java Package Does Not Exist” issue?
Tools such as IDEs with built-in error checking, build tools like Maven or Gradle, and command-line utilities can help diagnose the issue by providing detailed error messages and insights into the classpath configuration.
In the context of Java programming, the error message “Java Package Does Not Exist” typically indicates that the Java compiler cannot locate a specified package during the compilation or execution of a program. This issue often arises from several common causes, including incorrect package naming, missing dependencies, or improper configuration of the classpath. Developers must ensure that the package name is spelled correctly and matches the directory structure of the project, as Java is case-sensitive and requires precise alignment between the code and file organization.
Additionally, the absence of required libraries or external packages can lead to this error. It is crucial for developers to verify that all necessary libraries are included in the project’s build path. This can be managed through build tools like Maven or Gradle, which facilitate dependency management and can automatically resolve missing packages. Furthermore, ensuring that the IDE is properly configured to recognize the project structure and dependencies can prevent such errors from occurring.
Another critical aspect to consider is the environment setup. Developers should confirm that their Java Development Kit (JDK) is correctly installed and configured, as well as ensure that the JAVA_HOME environment variable points to the correct JDK installation. Misconfigurations in these areas can lead to the compiler being unable to find the necessary packages. Regular
Author Profile
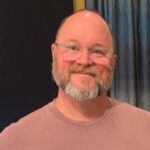
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?