How Can You Resolve Java Net ConnectException: Connection Timed Out Issues in Your Java Applications?
In the world of Java programming, connectivity issues can often feel like a black hole, sucking away precious time and resources. One of the most frustrating errors developers encounter is the `ConnectException: Connection timed out`. This seemingly cryptic message can halt your application’s progress and leave you scratching your head, wondering what went wrong. Understanding the intricacies of this error is crucial for any Java developer, whether you’re building a simple application or managing complex systems that rely on network communication.
At its core, a `ConnectException` indicates that your Java application is struggling to establish a connection to a specified host or service. This can happen for a variety of reasons, from network misconfigurations and firewall restrictions to server unavailability and incorrect connection parameters. As you dive deeper into the nuances of this error, you’ll discover that troubleshooting it requires a methodical approach, combining both technical knowledge and practical problem-solving skills.
In the sections that follow, we will explore the common causes of the `ConnectException: Connection timed out` error, discuss effective strategies for diagnosing the problem, and provide actionable solutions to help you restore connectivity. Whether you’re a seasoned developer or just starting out, understanding how to navigate these challenges will empower you to build more resilient Java applications and enhance your overall programming expertise.
Understanding Connection Timeout
A `ConnectException` indicating a “Connection Timed Out” in Java typically arises when a network connection cannot be established within a specified time frame. This can occur for various reasons, including server unavailability, network issues, or misconfiguration of the connection parameters.
When a client attempts to connect to a server, it sends a request over the network. If the server does not respond within a predetermined timeout period, the client will throw a `ConnectException`. This behavior is essential for preventing applications from hanging indefinitely due to unresponsive servers.
Key factors that influence connection timeouts include:
- Network Latency: Delays in network communications can lead to increased timeout occurrences.
- Server Load: High traffic on the server can result in slow response times or unavailability.
- Firewall Settings: Firewalls may block certain ports or protocols, impeding connections.
- DNS Resolution: Issues with DNS can delay or prevent the connection from being established.
Configuring Timeout Settings
In Java, you can configure connection timeout settings using the `URLConnection` or `HttpURLConnection` classes. Properly setting these values can help manage how long your application waits before throwing a `ConnectException`.
Here is an example of how to set timeout values:
“`java
import java.net.HttpURLConnection;
import java.net.URL;
public class TimeoutExample {
public static void main(String[] args) {
try {
URL url = new URL(“http://example.com”);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(5000); // Set connection timeout to 5 seconds
connection.setReadTimeout(5000); // Set read timeout to 5 seconds
connection.connect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Setting | Description | Default Value |
---|---|---|
setConnectTimeout(int timeout) | Sets the timeout for establishing a connection to the server. | 0 (infinite) |
setReadTimeout(int timeout) | Sets the timeout for reading from the input stream after a connection has been established. | 0 (infinite) |
Common Solutions for Connection Timeout Issues
When encountering a `ConnectException` due to a timeout, consider the following troubleshooting steps:
- Check Server Status: Ensure that the server is running and accessible.
- Review Network Configuration: Verify that the network settings allow for connections to the server.
- Increase Timeout Values: In cases of high latency, increasing the timeout values may prevent premature exceptions.
- Test Connectivity: Use tools like `ping` or `telnet` to check if the server is reachable from your machine.
- Inspect Firewall Rules: Make sure firewalls are not blocking the connection to the target server.
By addressing these areas, you can effectively mitigate connection timeout issues in your Java applications, leading to a more robust and user-friendly experience.
Understanding Java Net ConnectException
The `ConnectException` in Java occurs when an application attempts to connect to a server and the connection is refused or times out. This exception is part of the `java.net` package and is a subclass of `SocketException`, indicating a lower-level socket error during the connection attempt.
Common Causes of Connection Timeout
Several factors can lead to a `ConnectException` indicating a connection timeout:
- Server Unavailability: The server might be down or not reachable due to network issues.
- Firewall Restrictions: Firewalls may block the connection based on security policies.
- Incorrect Hostname or Port: The specified hostname or port number could be incorrect.
- Network Configuration: Issues with DNS, routing, or network settings can hinder connectivity.
- Overloaded Server: The server may be too busy to handle new requests, leading to timeouts.
Handling ConnectException
To effectively handle `ConnectException`, consider implementing the following strategies:
- Try-Catch Blocks: Wrap connection logic within try-catch blocks to gracefully manage exceptions.
“`java
try {
Socket socket = new Socket(“hostname”, port);
} catch (ConnectException e) {
System.out.println(“Connection timed out: ” + e.getMessage());
}
“`
- Retry Logic: Implement retry mechanisms with exponential backoff to handle transient issues.
- Logging: Use logging frameworks to capture the details of the exception for later analysis.
Configuration Settings to Avoid Timeouts
To minimize the occurrence of connection timeouts, consider adjusting the following settings:
Setting | Description |
---|---|
Connection Timeout | Set a reasonable timeout value to avoid long waits. Use `setSoTimeout(int timeout)` on `Socket`. |
Socket Options | Adjust TCP settings like `setKeepAlive(boolean)` to maintain connections. |
Proxy Settings | Ensure proxy configurations are correctly set if applicable. |
Debugging Connection Issues
When facing connection issues, debugging can be crucial. Follow these steps:
- Ping the Server: Verify if the server is reachable.
- Check Port Availability: Use tools like `telnet` or `nc` to check if the port is open.
- Inspect Firewall Rules: Review firewall settings to ensure that the application is allowed to connect.
- Review Application Logs: Check application logs for additional error details that may indicate the root cause.
Best Practices for Networking in Java
To enhance the reliability of network connections in Java applications, consider the following best practices:
- Use Connection Pooling: Implement connection pooling for efficiency.
- Close Resources: Always close sockets and streams to prevent resource leaks.
- Handle Timeouts Gracefully: Allow users to retry or provide fallback mechanisms when timeouts occur.
- Monitor Network Performance: Regularly assess and monitor the network for performance bottlenecks.
By applying these techniques and practices, developers can effectively manage and troubleshoot `ConnectException` issues, ensuring robust network communication in their Java applications.
Expert Insights on Java Net ConnectException: Connection Timed Out Issues
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The Java Net ConnectException: Connection Timed Out error typically arises when a client fails to establish a connection to the server within a specified timeout period. This can be due to network issues, incorrect server addresses, or server overload. It is crucial to analyze the network configuration and ensure that the server is reachable.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “In my experience, handling the ConnectException effectively requires implementing robust error handling and retry mechanisms in your Java application. Additionally, tuning the timeout settings based on the expected network latency can significantly reduce the occurrence of this error.”
Sarah Thompson (Network Architect, CloudNet Systems). “When dealing with Connection Timed Out errors in Java, it is essential to consider both client-side and server-side configurations. Firewalls, proxy settings, and network policies can all impact connectivity. A thorough examination of these elements often reveals the root cause of the problem.”
Frequently Asked Questions (FAQs)
What is a Java Net ConnectException?
A Java Net ConnectException is an exception that occurs when a connection attempt to a remote server fails. This typically indicates that the application cannot reach the server due to network issues or incorrect server configurations.
What does “Connection Timed Out” mean in Java?
“Connection Timed Out” in Java signifies that the application was unable to establish a connection to the specified server within the allotted time frame. This can occur due to server unavailability, network congestion, or firewall restrictions.
What are common causes of Connection Timed Out errors in Java?
Common causes include incorrect server addresses, server downtime, firewall settings blocking the connection, network issues, or incorrect port numbers specified in the connection settings.
How can I troubleshoot a ConnectException in Java?
To troubleshoot a ConnectException, verify the server address and port, check network connectivity, review firewall settings, and ensure that the server is running and accessible. Additionally, using tools like ping or telnet can help diagnose connectivity issues.
What steps can I take to prevent Connection Timed Out errors in my Java application?
To prevent Connection Timed Out errors, consider increasing the timeout settings in your connection code, implementing retry logic for transient failures, and ensuring robust error handling to manage connectivity issues gracefully.
Are there any specific libraries or tools that can help manage network connections in Java?
Yes, libraries such as Apache HttpClient, OkHttp, and Netty provide advanced features for managing network connections, including connection pooling, timeouts, and error handling, which can enhance the reliability of your Java applications.
In Java networking, a `ConnectException` with the message “Connection timed out” typically indicates that a connection attempt to a remote server has failed due to the server not responding within a specified timeout period. This situation can arise from various factors, such as network issues, server unavailability, incorrect host or port configurations, or firewall restrictions. Understanding the underlying causes is crucial for troubleshooting and resolving the issue effectively.
One of the primary takeaways is the importance of verifying the network configuration and ensuring that the target server is reachable. This includes checking the server’s IP address, port number, and ensuring that the server is running and capable of accepting connections. Additionally, developers should implement proper exception handling to manage `ConnectException` instances gracefully, allowing for better user experience and system stability.
Another significant insight is the role of timeout settings in connection attempts. Developers can adjust the timeout duration based on the application’s requirements and the expected network conditions. Setting a reasonable timeout can help in balancing responsiveness with the need to avoid prolonged waiting periods during connection attempts. Furthermore, utilizing tools such as network analyzers can assist in diagnosing connectivity issues, providing valuable information for troubleshooting.
Author Profile
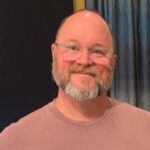
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?