How Can You Resolve the Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded?
In the world of Java programming, few errors strike as much fear into the hearts of developers as the infamous `java.lang.OutOfMemoryError: GC overhead limit exceeded`. This seemingly cryptic message often signals a critical issue lurking beneath the surface of your application, one that can lead to performance bottlenecks and application crashes if not addressed promptly. As Java continues to power a vast array of applications—from web services to enterprise solutions—understanding this error is essential for maintaining robust and efficient software.
The `GC overhead limit exceeded` error occurs when the Java Virtual Machine (JVM) spends an excessive amount of time performing garbage collection while recovering very little memory. This situation can arise from various factors, including memory leaks, inefficient memory usage, or simply an application that has outgrown its allocated resources. When the JVM detects that it is spending more than 98% of its time on garbage collection and recovering less than 2% of the heap, it triggers this error, effectively signaling that the system is unable to keep up with memory demands.
In this article, we will delve into the intricacies of the `OutOfMemoryError`, exploring its causes, implications, and strategies for resolution. Whether you are a seasoned developer or a newcomer to Java, understanding this
Understanding the Causes of OutOfMemoryError
The `Java.Lang.OutOfMemoryError: GC overhead limit exceeded` error occurs when the Java Virtual Machine (JVM) spends too much time performing garbage collection (GC) and recovers very little memory in return. This situation typically signifies that the application has reached a critical state where it is unable to allocate enough memory for new objects. Here are some common causes of this error:
- Memory Leaks: Unintentional retention of object references can prevent the garbage collector from reclaiming memory.
- Insufficient Heap Space: The allocated heap space may not be sufficient for the application’s needs, leading to frequent garbage collection cycles.
- Large Object Creation: Continuously creating large objects can exhaust the available memory quickly.
- High Object Retention: If objects are retained longer than necessary, they contribute to memory pressure.
Identifying Symptoms of the Error
The symptoms of the `GC overhead limit exceeded` error may manifest in several ways. Developers should be vigilant for the following indicators:
- Frequent GC Activity: An unusually high frequency of garbage collection events.
- Long GC Pauses: Significant pauses in application response time during garbage collection.
- Performance Degradation: General sluggishness in application performance, especially during memory-intensive operations.
Monitoring and Diagnosing Memory Usage
To effectively diagnose memory-related issues, developers can utilize various monitoring tools and techniques. These tools help visualize memory consumption and identify potential leaks.
Tool | Description |
---|---|
JVisualVM | A monitoring tool that provides visual representations of memory usage, CPU consumption, and thread activity. |
Java Mission Control | A performance profiling tool that can analyze runtime behavior and memory consumption. |
Heap Dumps | Memory snapshots that can be analyzed to identify retained object references and memory leaks. |
Utilizing these tools enables developers to pinpoint issues leading to `OutOfMemoryError` and take corrective actions.
Solutions to Mitigate the Error
When facing the `GC overhead limit exceeded` error, several strategies can be employed to mitigate the problem:
- Increase Heap Size: Adjust the JVM parameters to allocate more memory, for instance, `-Xmx` to set the maximum heap size.
- Optimize Code: Review the application code to identify and fix memory leaks or inefficient memory usage patterns.
- Profile and Analyze: Use profiling tools to analyze memory allocations and identify large or unnecessary objects.
- Use Efficient Data Structures: Choose appropriate data structures that can handle the expected load without excessive overhead.
By implementing these strategies, developers can significantly reduce the likelihood of encountering memory-related issues in their applications.
Understanding `OutOfMemoryError: GC Overhead Limit Exceeded`
The `OutOfMemoryError: GC Overhead Limit Exceeded` is a specific Java error that indicates the Java Virtual Machine (JVM) is spending too much time performing garbage collection while still failing to free up sufficient memory. This situation typically arises when the application is unable to allocate new objects due to insufficient heap space, leading the garbage collector to work excessively to reclaim memory.
Causes of the Error
Several factors can contribute to the occurrence of this error:
- Memory Leaks: Persistent references to objects that are no longer needed prevent them from being garbage collected.
- Inadequate Heap Size: The default heap size may be insufficient for memory-intensive applications.
- High Object Creation Rate: Rapidly creating objects can overwhelm the garbage collector, especially if they are not being collected efficiently.
- Improper GC Configuration: Ineffective garbage collection tuning can lead to excessive time spent on GC.
Identifying Symptoms
Symptoms that may indicate the presence of this error include:
- Significant application slowdowns or freezes.
- Increased CPU usage due to extensive garbage collection activity.
- Frequent allocation failures leading to exceptions.
Tuning Garbage Collection
To mitigate `GC Overhead Limit Exceeded`, consider the following garbage collection tuning strategies:
Parameter | Description |
---|---|
`-Xmx` | Set the maximum heap size to accommodate application needs. |
`-Xms` | Set the initial heap size, ideally equal to `-Xmx`. |
`-XX:+UseG1GC` | Use the G1 garbage collector, which can offer better performance for large heaps. |
`-XX:GCTimeRatio` | Adjust the ratio of time spent in GC compared to application time. Lower values can reduce GC overhead. |
`-XX:MaxGCPauseMillis` | Set a target for maximum pause time during GC, helping to control latencies. |
Code Optimization Techniques
In addition to adjusting JVM parameters, optimizing the application code can significantly help reduce memory usage:
- Minimize Object Creation: Reuse objects where possible instead of instantiating new ones.
- Use Appropriate Data Structures: Select the right data structures to optimize memory usage.
- Profile Memory Usage: Use tools like VisualVM or Eclipse Memory Analyzer to identify memory leaks and heavy memory consumers.
- Implement Caching Wisely: Ensure that caching mechanisms do not retain unnecessary objects.
Monitoring and Maintenance
Regular monitoring and maintenance can prevent the occurrence of this error:
- Enable GC Logging: Use flags like `-XX:+PrintGCDetails` to log garbage collection events and analyze memory usage patterns.
- Set Alerts: Implement monitoring solutions to alert on high memory usage or frequent garbage collection events.
- Review Application Architecture: Ensure the architecture supports scalability and efficient memory usage.
By addressing the factors contributing to `OutOfMemoryError: GC Overhead Limit Exceeded`, developers can enhance application performance and reliability.
Understanding Java’s OutOfMemoryError: GC Overhead Limit Exceeded
Dr. Emily Carter (Senior Java Performance Engineer, Tech Innovations Inc.). “The ‘GC Overhead Limit Exceeded’ error typically indicates that the garbage collector is spending too much time trying to free up memory without success. This often suggests that the application is either holding onto too much memory or that the heap size is insufficient for the workload.”
Michael Chen (Lead Software Architect, Cloud Solutions Corp.). “To effectively address the ‘Java.Lang.Outofmemoryerror: GC Overhead Limit Exceeded’ issue, developers should analyze memory usage patterns and consider optimizing their code to reduce memory consumption. Additionally, increasing the heap size can provide temporary relief, but it is essential to identify the root cause.”
Sarah Thompson (Java Performance Consultant, OptimizeIT). “Frequent occurrences of this error signal a need for a thorough review of the application’s memory management strategies. Implementing proper caching mechanisms and revisiting object lifecycle management can significantly mitigate the risk of hitting the GC overhead limit.”
Frequently Asked Questions (FAQs)
What does the error “Java.Lang.Outofmemoryerror: Gc Overhead Limit Exceeded” mean?
This error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection and recovering very little memory. It typically occurs when the application is using a significant amount of memory but is unable to free up enough space for new allocations.
What causes the “Gc Overhead Limit Exceeded” error?
The error is often caused by memory leaks, insufficient heap size, or inefficient memory usage within the application. It may also arise when the application processes large datasets or has a high number of objects in memory.
How can I resolve the “Gc Overhead Limit Exceeded” error?
To resolve this error, consider increasing the heap size using JVM options (e.g., `-Xmx` and `-Xms`), optimizing your code to reduce memory consumption, identifying and fixing memory leaks, or using profiling tools to analyze memory usage patterns.
What JVM options can help prevent this error?
You can use options such as `-XX:+UseG1GC` to enable the G1 garbage collector, `-Xmx` to increase the maximum heap size, and `-XX:GCTimeRatio` to adjust the garbage collection time ratio, which can help mitigate this issue.
Are there any tools available to diagnose memory issues in Java applications?
Yes, tools such as VisualVM, Eclipse Memory Analyzer (MAT), and JProfiler can help diagnose memory issues. These tools allow you to analyze heap dumps, monitor memory usage, and identify potential memory leaks.
Is it safe to ignore the “Gc Overhead Limit Exceeded” error?
Ignoring this error is not advisable, as it indicates that the application is struggling to manage memory efficiently. Addressing the underlying issues is crucial to ensure application stability and performance.
The Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded error is a critical issue that developers encounter when the Java Virtual Machine (JVM) spends an excessive amount of time performing garbage collection without reclaiming sufficient memory. This error indicates that the application is running low on heap space, leading to performance degradation and potential application crashes. Understanding the causes and implications of this error is essential for maintaining optimal application performance and ensuring a stable user experience.
Several factors can contribute to the occurrence of this error, including memory leaks, inadequate heap size configuration, and inefficient memory management practices. Developers must conduct thorough analyses of their applications to identify memory usage patterns and optimize resource allocation. Tools such as profilers and memory analyzers can assist in pinpointing memory leaks and areas where memory consumption can be improved, thereby reducing the likelihood of encountering this error.
To mitigate the risk of the GC Overhead Limit Exceeded error, it is crucial to implement best practices in memory management. This includes configuring appropriate heap sizes based on application requirements, regularly monitoring memory usage, and employing efficient coding techniques to minimize object creation and retention. By proactively addressing these aspects, developers can enhance application performance and reduce the frequency of memory-related issues.
Author Profile
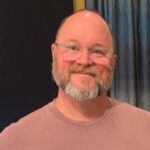
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?