Is a Tuple Mutable in Python? Unpacking the Truth Behind Python’s Data Types
### Introduction
In the vibrant world of Python programming, data structures play a pivotal role in how we organize, manipulate, and interact with information. Among these structures, tuples stand out for their unique characteristics and functionality. As a developer, understanding the nature of tuples is essential, especially when it comes to their mutability. This seemingly simple question—”Is a tuple mutable in Python?”—opens the door to a deeper exploration of how tuples operate, their advantages, and the scenarios in which they shine compared to other data structures like lists.
Tuples, often described as immutable sequences, are a fundamental part of Python’s data handling capabilities. They allow you to store collections of items, yet they come with the intriguing limitation that once created, their contents cannot be altered. This immutability raises important questions about their use cases and the implications for performance and data integrity. As we delve into this topic, we will uncover the reasons behind the design choice of immutability, how it affects your programming practices, and the scenarios in which tuples can be particularly advantageous.
By the end of this exploration, you will not only grasp the concept of tuple mutability but also appreciate the broader context of how this characteristic influences the way Python developers approach data management. Whether you are a novice
Understanding Tuple Mutability
In Python, a tuple is a collection that is ordered and immutable. This means once a tuple is created, its contents cannot be altered, which distinguishes it from other collection types like lists that are mutable. The immutability of tuples provides certain benefits, including:
- Data Integrity: Since tuples cannot be modified, they ensure that the data remains constant throughout its lifecycle.
- Hashability: Tuples can be used as keys in dictionaries because they are hashable, whereas lists cannot be used for this purpose.
- Performance: Tuples can be slightly more memory-efficient than lists, leading to better performance in scenarios where a constant set of values is needed.
Characteristics of Tuples
To further illustrate the properties of tuples, consider the following characteristics:
Feature | Tuple | List |
---|---|---|
Mutability | Immutable | Mutable |
Syntax | `()` (parentheses) | `[]` (brackets) |
Hashable | Yes | No |
Use Case | Fixed collections | Dynamic collections |
These characteristics highlight the fundamental differences between tuples and lists. While both are used to store collections of items, the choice between them depends on the specific needs of the application.
Examples of Tuple Usage
Creating a tuple is straightforward. Here’s how you can define one:
python
my_tuple = (1, 2, 3)
Once defined, attempting to change an element in a tuple will result in a `TypeError`:
python
my_tuple[0] = 4 # This will raise an error
However, tuples can contain mutable objects like lists. For instance:
python
nested_tuple = (1, 2, [3, 4])
nested_tuple[2][0] = 5 # This is valid
In this case, while the tuple itself remains unchanged, the list within it can be modified.
Common Use Cases for Tuples
Tuples are often used in the following scenarios:
- Returning Multiple Values: Functions can return multiple values as tuples, making it easier to manage outputs.
- Unpacking Values: Tuples are commonly unpacked into individual variables, enhancing code readability.
Example of returning multiple values:
python
def coordinates():
return (10, 20)
x, y = coordinates()
In this code snippet, the tuple returned by the `coordinates` function is unpacked into the variables `x` and `y`.
In summary, the immutability of tuples in Python serves specific purposes that align with the needs of various programming scenarios, providing a robust mechanism for managing fixed collections of data.
Understanding Tuple Mutability in Python
In Python, tuples are a fundamental data structure that is often compared to lists. However, their mutability characteristics set them apart significantly.
Definition of Mutability
Mutability refers to the ability of an object to be changed after its creation. Objects that can be altered are termed mutable, while those that cannot be changed are termed immutable.
Characteristics of Tuples
- Immutable: Once a tuple is created, its elements cannot be modified, added, or removed.
- Ordered: Tuples maintain the order of elements, which means the position of elements is preserved.
- Heterogeneous: Tuples can contain elements of different data types, including other tuples.
Comparison: Tuples vs. Lists
Feature | Tuple | List |
---|---|---|
Mutability | Immutable | Mutable |
Syntax | Uses parentheses `()` | Uses square brackets `[]` |
Performance | Generally faster | Slower due to mutability |
Methods | Limited methods available | Extensive methods available |
Examples of Tuple Immutability
Attempting to modify a tuple will result in a `TypeError`. Below are examples illustrating this property:
python
# Creating a tuple
my_tuple = (1, 2, 3)
# Attempting to change an element
try:
my_tuple[0] = 10
except TypeError as e:
print(e) # Output: ‘tuple’ object does not support item assignment
Despite tuples being immutable, they can still contain mutable objects. For instance, a tuple can store a list, which can be modified:
python
# Tuple containing a list
my_tuple = (1, 2, [3, 4])
# Modifying the list inside the tuple
my_tuple[2][0] = 10
print(my_tuple) # Output: (1, 2, [10, 4])
Use Cases for Tuples
Tuples are often used in scenarios where a fixed collection of items is required. Some common use cases include:
- Returning Multiple Values: Functions can return tuples to convey multiple results succinctly.
- Dictionary Keys: Tuples can serve as keys in dictionaries because they are hashable.
- Data Integrity: Using tuples can prevent accidental changes to the data, ensuring consistency.
Tuple Mutability
In Python, tuples are immutable, meaning their contents cannot be altered once defined. This property distinguishes them from lists and makes them suitable for specific programming scenarios where data integrity and fixed collections are desired. Understanding tuple behavior is critical for effective Python programming.
Understanding Tuple Mutability in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, tuples are immutable, meaning once they are created, their elements cannot be changed, added, or removed. This characteristic makes tuples ideal for storing fixed collections of items, ensuring data integrity throughout the program.”
James Liu (Python Developer, CodeCraft Solutions). “The immutability of tuples is a fundamental aspect of their design in Python. This feature not only enhances performance but also allows tuples to be used as keys in dictionaries, unlike mutable types such as lists.”
Sarah Thompson (Computer Science Professor, University of Technology). “Understanding that tuples are immutable is crucial for Python developers. It influences how data structures are chosen in applications, particularly when a stable and unchangeable collection is needed.”
Frequently Asked Questions (FAQs)
Is a tuple mutable in Python?
No, a tuple is immutable in Python. Once a tuple is created, its elements cannot be changed, added, or removed.
What does it mean for a data structure to be immutable?
Immutability means that the data structure cannot be altered after its creation. Any modification attempts will result in the creation of a new object rather than changing the original.
Can I change the elements of a tuple?
No, you cannot change the elements of a tuple directly. If you need a modified version, you must create a new tuple with the desired changes.
Are there any ways to work around tuple immutability?
While you cannot modify a tuple directly, you can convert it to a list, make the necessary changes, and then convert it back to a tuple.
What are the advantages of using tuples over lists?
Tuples are generally faster than lists due to their immutability. They also provide a level of data integrity since their contents cannot be altered, making them suitable for use as dictionary keys.
Can a tuple contain mutable elements?
Yes, a tuple can contain mutable elements such as lists or dictionaries. However, while the mutable elements can be changed, the tuple itself remains immutable.
In Python, tuples are immutable data structures, meaning that once a tuple is created, its elements cannot be modified, added, or removed. This characteristic distinguishes tuples from lists, which are mutable and allow for such modifications. The immutability of tuples makes them particularly useful for situations where a constant set of values is required, ensuring that the data remains unchanged throughout the program’s execution.
Despite their immutable nature, tuples can still contain mutable objects, such as lists or dictionaries. This means that while the tuple itself cannot be altered, the mutable objects within it can be modified. This feature allows for flexibility in certain scenarios, but it is essential to understand the implications of mixing mutable and immutable types when designing data structures in Python.
Key takeaways include the importance of choosing the appropriate data structure based on the requirements of the application. Tuples are ideal for fixed collections of items, providing performance benefits due to their immutability. In contrast, lists should be used when there is a need for dynamic data manipulation. Understanding these distinctions can help developers make informed decisions and write more efficient and effective Python code.
Author Profile
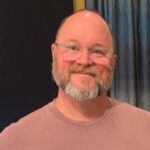
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?