Why is ‘$ Is Not Defined’ Error Occurring in JavaScript and How Can You Fix It?
In the world of web development, JavaScript stands as a cornerstone language, enabling developers to create dynamic and interactive user experiences. However, even seasoned programmers can encounter frustrating errors that disrupt their workflow. One such common issue is the dreaded message: `$ is not defined`. This seemingly innocuous error can halt your coding progress and leave you scratching your head, wondering what went wrong. Understanding the root causes of this error is crucial for anyone looking to build robust applications using JavaScript and popular libraries like jQuery.
When you see the `$ is not defined` error, it typically indicates that the JavaScript interpreter cannot find the `$` symbol, which is often associated with jQuery. This can happen for several reasons, such as the jQuery library not being properly linked in your HTML file, or it being loaded after your script attempts to use it. Additionally, this error can arise if there are conflicts with other libraries or if you’re working in a scope where `$` is not recognized.
Navigating through these potential pitfalls is essential for smooth development. By understanding the nuances of JavaScript and how libraries interact within your code, you can quickly diagnose and resolve the `$ is not defined` error. In the following sections, we will delve deeper into the causes, solutions,
Understanding the Error
The error `$ is not defined` typically occurs in JavaScript when the code tries to use the jQuery library without it being properly loaded. The dollar sign `$` is a shorthand reference to jQuery, and if jQuery is not available, any attempt to use `$` will result in this error. This situation can arise from various scenarios, such as forgetting to include the jQuery script, including it incorrectly, or attempting to use jQuery before it has been fully loaded.
Common Causes of the Error
There are several common reasons why this error might occur:
- jQuery Not Included: The jQuery library might not be linked in your HTML file.
- Incorrect Path: The path to the jQuery file may be incorrect or the file may not be present at the specified location.
- Order of Scripts: Scripts that depend on jQuery may be executed before jQuery is loaded.
- Conflict with Other Libraries: If another JavaScript library that uses the `$` symbol is included, it may cause a conflict.
How to Fix the Error
To resolve the `$ is not defined` error, follow these steps:
- Ensure jQuery is Included: Make sure that the jQuery script is included in your HTML file. You can do this by adding the following script tag in the `` or at the end of the ``:
“`html
“`
- Check the Script Order: If you have multiple scripts, ensure that jQuery is loaded before any scripts that use it. For example:
“`html
“`
- Use jQuery in No Conflict Mode: If there are conflicts with other libraries, use jQuery’s noConflict mode to avoid using the `$` shorthand:
“`javascript
jQuery.noConflict();
jQuery(document).ready(function($) {
// Your code using jQuery
});
“`
Debugging Tips
If you continue to experience issues after following the above steps, consider the following debugging tips:
- Check the Console: Use the browser’s developer console to check for additional errors that may provide more context.
- Network Tab: In the developer tools, check the Network tab to ensure that the jQuery file is loading correctly without 404 errors.
- Use a Local Copy: If you’re using a CDN, try downloading jQuery and linking to it locally to rule out any CDN-related issues.
Example of Correct Implementation
Here’s an example of a simple HTML document correctly implementing jQuery:
“`html
“`
This code ensures jQuery is loaded before executing any jQuery-dependent scripts, preventing the `$ is not defined` error.
Understanding the Error
The error message `$ is not defined` typically occurs in JavaScript when the code attempts to use the jQuery library (or similar libraries) without it being loaded properly. This can arise from several issues, including:
- The jQuery library is not included in the HTML file.
- The jQuery script tag is incorrectly placed.
- The jQuery file is not accessible due to a broken link.
Common Causes
Identifying the root cause of this error involves checking several key areas:
- Missing jQuery Library: Ensure that you have included the jQuery library in your HTML file.
- Script Load Order: JavaScript files should be loaded in the correct sequence. If your script that uses `$` is loaded before jQuery, the error will occur.
- Incorrect jQuery Version: Using a version of jQuery that is incompatible with your code can also lead to this issue.
- No Conflict Mode: If jQuery is in no conflict mode, the `$` alias will not work unless explicitly defined.
How to Fix the Error
Here are steps to troubleshoot and fix the `$ is not defined` error:
- Include jQuery Correctly: Ensure that jQuery is included in your HTML file. You can do this via a CDN or by downloading the library.
“`html
“`
- Check Script Placement: Place your jQuery-dependent scripts after the jQuery library inclusion. Ideally, place them at the end of the `` tag.
“`html
“`
- Verify File Paths: If using a local jQuery file, check the path to ensure it is correct and accessible.
- Use No Conflict Mode: If there are conflicts with other libraries, you can use jQuery’s no conflict mode:
“`javascript
jQuery.noConflict();
jQuery(document).ready(function($) {
// Your jQuery code using $ as an alias
});
“`
Debugging Techniques
To effectively debug the issue, consider the following techniques:
- Check Console for Errors: Use the browser’s developer tools (F12) to check for any loading errors or syntax issues in the console.
- Network Tab Inspection: In the developer tools, inspect the network tab to verify that the jQuery library is loading successfully.
- Try Alternative Aliases: If using no conflict mode, test using `jQuery` instead of `$` to see if the code works.
Example Scenario
Here’s a simple example that demonstrates the correct usage of jQuery:
“`html
“`
This example correctly includes jQuery and initializes a click event without encountering the `$ is not defined` error.
Understanding the `$ Is Not Defined` Error in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “The `$ is not defined` error typically arises when the jQuery library is not properly loaded before your script attempts to use it. Ensuring that the jQuery script tag is included in your HTML before any custom scripts can resolve this issue.”
Michael Chen (Lead Front-End Engineer, Digital Solutions Co.). “This error can also occur if you are using a different version of jQuery or if the `$` alias has been overridden. It is important to check for any conflicts with other libraries or frameworks that might change the behavior of the `$` symbol.”
Sarah Thompson (JavaScript Educator, Code Academy). “For beginners, encountering the `$ is not defined` error can be frustrating. I always advise my students to familiarize themselves with the concept of scope in JavaScript, as this can help in understanding why certain variables or functions may not be accessible at runtime.”
Frequently Asked Questions (FAQs)
What does the error “$ is not defined” mean in JavaScript?
The error “$ is not defined” indicates that the JavaScript interpreter cannot find the definition for the variable or function named “$”. This typically occurs when jQuery is not loaded or is loaded after your script that uses it.
How can I resolve the “$ is not defined” error?
To resolve this error, ensure that jQuery is correctly included in your HTML file before any script that uses the “$” symbol. You can include jQuery from a CDN or a local file, ensuring it is placed in the `
What are common reasons for the “$ is not defined” error?
Common reasons include: jQuery not being included in the HTML, a typo in the jQuery script tag, or loading your script before jQuery. Additionally, conflicts with other libraries that use the “$” symbol can also cause this issue.
Can I use a different symbol instead of “$” for jQuery?
Yes, you can use the jQuery noConflict mode to assign jQuery to a different variable. For example, you can use `jQuery.noConflict();` and then use `jQuery` instead of “$” to avoid conflicts with other libraries.
Is it possible to use jQuery without the “$” symbol?
Yes, you can use jQuery without the “$” symbol by referencing it directly as `jQuery`. This is particularly useful in scenarios where the “$” symbol is being used by another library or framework.
What should I check if the error persists after including jQuery?
If the error persists, check for multiple jQuery versions being loaded, ensure there are no JavaScript errors in the console that may prevent execution, and verify that your script runs after the DOM is fully loaded, typically using `$(document).ready()`.
The error message “$ is not defined” in JavaScript typically indicates that the jQuery library, or any other library that uses the dollar sign as a shorthand, has not been properly loaded or initialized in the environment. This can occur for various reasons, such as incorrect script tag placement, missing library files, or issues with asynchronous loading. Understanding the context in which this error occurs is crucial for effective debugging and resolution.
One common cause of this error is placing script tags incorrectly in the HTML document. To avoid this, ensure that jQuery is included before any script that attempts to use the $ symbol. Additionally, if the script is being loaded asynchronously, it is important to ensure that the jQuery library is fully loaded before executing any dependent code. Utilizing the jQuery ready function can help manage this timing issue effectively.
Another key takeaway is the importance of checking for library conflicts. If multiple libraries use the $ symbol, it can lead to conflicts. In such cases, using jQuery’s noConflict mode allows developers to avoid these issues by releasing the $ variable and using jQuery instead. This practice promotes better compatibility and prevents errors related to variables.
In summary, the “$ is not defined” error is a common
Author Profile
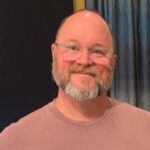
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?