Is JavaScript Case Sensitive? Unraveling the Mystery!
Is JavaScript Case Sensitive?
In the ever-evolving landscape of web development, JavaScript stands out as one of the most popular programming languages, powering everything from simple web pages to complex applications. As developers dive into the intricacies of this versatile language, they often encounter a fundamental question: Is JavaScript case sensitive? Understanding the nuances of case sensitivity is crucial for writing effective code and avoiding common pitfalls that can lead to frustrating bugs.
JavaScript, like many programming languages, treats identifiers—such as variable names, function names, and object properties—as case-sensitive. This means that the language distinguishes between uppercase and lowercase letters, making `myVariable`, `MyVariable`, and `MYVARIABLE` three entirely different entities. This characteristic can significantly impact how developers write and organize their code, as a simple typographical error can lead to unexpected results or errors during execution.
Moreover, the case sensitivity of JavaScript extends beyond just variable names; it also applies to built-in functions and methods. For instance, calling `console.log` correctly is essential, as writing `Console.Log` will result in an error. As we delve deeper into the topic, we’ll explore the implications of case sensitivity in JavaScript, common mistakes developers make, and best practices to ensure your
Understanding Case Sensitivity in JavaScript
JavaScript is a case-sensitive programming language, meaning that it differentiates between uppercase and lowercase letters. This characteristic affects variable names, function names, and other identifiers. For instance, the variables `myVariable`, `MyVariable`, and `MYVARIABLE` are considered three distinct entities.
Implications of Case Sensitivity
The case sensitivity in JavaScript can lead to various implications in coding practices. Understanding these implications is crucial for maintaining code readability and preventing errors. Here are some key points to consider:
- Variable Declaration: When declaring variables, be consistent with naming conventions to avoid confusion. Using a consistent case style, such as camelCase or snake_case, can enhance code clarity.
- Function Names: Similar to variables, function names must also adhere to case sensitivity. Calling a function with the wrong case will result in a reference error.
- Object Properties: Object properties are also case-sensitive. Accessing a property with the wrong case will yield “, leading to potential runtime errors.
Examples of Case Sensitivity
To illustrate the case sensitivity of JavaScript, consider the following examples:
“`javascript
let example = “Hello”;
let Example = “World”;
console.log(example); // Outputs: Hello
console.log(Example); // Outputs: World
“`
In this example, `example` and `Example` are treated as two separate variables due to their differing cases.
Common Mistakes Related to Case Sensitivity
Developers often encounter issues stemming from case sensitivity. Below is a table listing common mistakes and their solutions:
Mistake | Solution |
---|---|
Using inconsistent variable names, e.g., `myVar` vs. `MyVar` | Stick to a naming convention throughout the codebase. |
Calling a function with incorrect case, e.g., `myFunction()` vs. `MyFunction()` | Always verify the function name’s case before invocation. |
Accessing object properties with the wrong case, e.g., `obj.property` vs. `obj.Property` | Check the object definition for accurate property names. |
By being mindful of case sensitivity in JavaScript, developers can write more robust and error-free code.
Understanding Case Sensitivity in JavaScript
JavaScript is indeed case-sensitive. This means that the language differentiates between uppercase and lowercase letters in identifiers such as variable names, function names, and other identifiers.
Implications of Case Sensitivity
The case sensitivity of JavaScript has several important implications for developers:
- Variable Declaration: The following variable names are considered distinct:
- `myVariable`
- `MyVariable`
- `MYVARIABLE`
- Function Names: Function calls must match the case of their definitions:
- If a function is defined as `function myFunction() {}`, calling `myfunction()` will result in an error.
- Object Properties: Accessing object properties is also case-sensitive:
- For an object defined as `const obj = { key: ‘value’ };`, `obj.key` and `obj.Key` will yield different results.
Common Pitfalls
Developers, especially those new to JavaScript, may encounter several common pitfalls due to case sensitivity:
- Typographical Errors:
- Misspelling a variable or function name by altering its case can lead to runtime errors.
- Code Maintainability:
- Inconsistent casing can create confusion and make code harder to read and maintain.
- Library and Framework Integration:
- Many libraries are case-sensitive in their API, which requires careful attention to the casing used in method calls.
Best Practices for Managing Case Sensitivity
To mitigate issues related to case sensitivity, consider the following best practices:
- Consistent Naming Conventions:
- Adopt a naming convention (e.g., camelCase, PascalCase) and apply it consistently throughout your codebase.
- Code Reviews:
- Implement regular code reviews to catch case-related errors early in the development process.
- Use Linters:
- Utilize tools like ESLint to enforce consistent casing and identify potential issues before runtime.
Case Sensitivity in JavaScript vs. Other Languages
Understanding how JavaScript’s case sensitivity compares to other programming languages can provide further insight:
Language | Case Sensitivity |
---|---|
JavaScript | Yes |
Python | Yes |
Java | Yes |
C | Yes |
PHP | No (in function names) |
SQL | No (in identifiers) |
This table illustrates that while many popular programming languages are case-sensitive, some, like PHP and SQL, exhibit more flexibility, particularly concerning function names and identifiers.
Conclusion on Case Sensitivity
Recognizing and understanding the case sensitivity of JavaScript is crucial for effective programming within the language. By adhering to best practices and being mindful of naming conventions, developers can avoid common pitfalls and enhance code clarity and maintainability.
Understanding Case Sensitivity in JavaScript: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “JavaScript is indeed case sensitive, meaning that variable names, function names, and other identifiers must be used consistently in terms of capitalization. For instance, ‘Variable’ and ‘variable’ would be interpreted as two distinct identifiers, which can lead to significant bugs if not handled carefully.”
Michael Chen (Lead Developer, CodeMaster Solutions). “Understanding the case sensitivity of JavaScript is crucial for developers. It affects not only variable declarations but also object properties and method names. A common pitfall for beginners is confusing ‘myVariable’ with ‘MyVariable’, which can result in unexpected behavior in the code.”
Sarah Patel (JavaScript Instructor, Code Academy). “In JavaScript, case sensitivity is a fundamental aspect that differentiates it from some other programming languages. This characteristic emphasizes the importance of consistency in naming conventions, which can enhance code readability and maintainability for teams working on large projects.”
Frequently Asked Questions (FAQs)
Is JavaScript case sensitive?
Yes, JavaScript is case sensitive. This means that variable names, function names, and other identifiers must be used consistently in terms of their capitalization.
What are examples of case sensitivity in JavaScript?
For instance, the variables `myVariable` and `MyVariable` are considered two distinct identifiers in JavaScript due to their different casing.
How does case sensitivity affect variable declarations?
When declaring variables, using different cases can lead to errors or unexpected behavior. For example, if you declare a variable as `myVar`, referencing `MyVar` will result in a reference error.
Are built-in JavaScript functions case sensitive as well?
Yes, built-in JavaScript functions are also case sensitive. For example, `console.log()` is valid, while `Console.Log()` will result in an error since the casing does not match.
Can case sensitivity lead to bugs in JavaScript code?
Absolutely, case sensitivity can lead to bugs if developers do not maintain consistent casing throughout their code. This can result in variables or unexpected function calls.
How can developers avoid issues related to case sensitivity?
Developers can avoid case sensitivity issues by adopting consistent naming conventions, using linting tools, and thoroughly reviewing code to ensure proper casing is maintained across all identifiers.
JavaScript is a case-sensitive programming language, which means that it differentiates between uppercase and lowercase letters in identifiers, keywords, and other elements. This characteristic is fundamental to how JavaScript operates, affecting variable names, function names, and object properties. For example, the variables `myVariable`, `MyVariable`, and `MYVARIABLE` would be treated as distinct entities within the same scope, leading to potential confusion if not properly managed.
The case sensitivity of JavaScript can have significant implications for code readability and maintenance. Developers must be consistent in their naming conventions to avoid errors and enhance code clarity. This is especially crucial in collaborative environments where multiple developers may work on the same codebase. Adopting a clear and consistent naming strategy can help mitigate the risks associated with case sensitivity.
In summary, understanding that JavaScript is case-sensitive is essential for effective programming in this language. It is vital for developers to be aware of this feature to prevent naming conflicts and ensure that their code functions as intended. By adhering to best practices in naming conventions, developers can create more robust and maintainable code, ultimately leading to a more efficient development process.
Author Profile
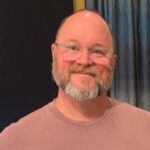
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?