Is JavaScript Case Sensitive? Understanding the Importance of Case in Coding
### Is JavaScript Case Sensitive?
In the vast landscape of programming languages, understanding the nuances of syntax and structure is crucial for both novice and seasoned developers. One such fundamental aspect that often raises questions is case sensitivity. As you delve into the world of JavaScript, a language that powers the dynamic web, you may find yourself pondering: Is JavaScript case sensitive? This seemingly simple question opens the door to a deeper exploration of how JavaScript interprets identifiers, variables, and functions, and how these elements interact within your code.
At its core, case sensitivity refers to the distinction between uppercase and lowercase letters in programming. In JavaScript, this characteristic plays a pivotal role in how the language recognizes and differentiates between various identifiers. Whether you’re declaring variables, defining functions, or invoking methods, the case you choose can significantly impact the functionality and readability of your code. This article will guide you through the implications of case sensitivity in JavaScript, illustrating how it can influence everything from variable declarations to object properties.
As you navigate through this discussion, you’ll discover that case sensitivity is not just a technical detail; it shapes the way you write and understand JavaScript. By grasping this concept, you’ll enhance your coding practices, avoid common pitfalls, and ultimately become a more proficient developer. So
Understanding Case Sensitivity in JavaScript
JavaScript is a case-sensitive language, which means that it distinguishes between uppercase and lowercase letters in identifiers. This characteristic affects various elements of the language, including variable names, function names, and property names. As a result, the following identifiers would be treated as distinct:
- `myVariable`
- `MyVariable`
- `MYVARIABLE`
This case sensitivity ensures that developers can use the same sequence of characters in different contexts without causing conflicts. It is crucial for maintaining clarity and avoiding errors in code.
Impact on Variable Declaration
When declaring variables in JavaScript, the case of the letters used directly impacts their scope and accessibility. For example:
javascript
let score = 10;
let Score = 20;
console.log(score); // Outputs: 10
console.log(Score); // Outputs: 20
In this example, `score` and `Score` are two entirely different variables, which could lead to confusion if not managed properly. Developers are encouraged to adopt consistent naming conventions to enhance code readability.
Common Practices for Naming Conventions
To avoid potential issues stemming from case sensitivity, developers often follow specific naming conventions:
- Camel Case: Commonly used for variable names (e.g., `myVariableName`).
- Pascal Case: Often used for class names (e.g., `MyClassName`).
- Snake Case: Rarely used in JavaScript, but can be seen in constants (e.g., `MY_CONSTANT`).
Convention | Description | Example |
---|---|---|
Camel Case | First letter lowercase, subsequent words capitalized | myVariableName |
Pascal Case | First letter of each word capitalized | MyClassName |
Snake Case | Words separated by underscores, typically uppercase | MY_CONSTANT |
Case Sensitivity in Object Properties
Similar to variables, object properties in JavaScript are also case-sensitive. This can lead to unexpected behavior if the casing is inconsistent. For example:
javascript
let obj = {
name: ‘Alice’,
Name: ‘Bob’
};
console.log(obj.name); // Outputs: Alice
console.log(obj.Name); // Outputs: Bob
In this object, `name` and `Name` are treated as separate properties, which can complicate data management if not handled carefully.
Being aware of case sensitivity in JavaScript is vital for writing efficient, error-free code. By following best practices in naming and being consistent with identifier usage, developers can minimize errors and improve the maintainability of their code.
Understanding Case Sensitivity in JavaScript
JavaScript is a case-sensitive language, which means that it differentiates between uppercase and lowercase letters in identifiers, keywords, and operators. This characteristic is essential for developers to grasp as it impacts variable naming, function definitions, and many other aspects of code.
Implications of Case Sensitivity
In JavaScript, case sensitivity leads to distinct meanings for names that differ only by case. For instance:
- `variable`, `Variable`, and `VARIABLE` are considered three different identifiers.
- Function names are also case-sensitive, e.g., `myFunction()` is different from `MyFunction()`.
This feature can lead to errors if not properly managed. Consider the following examples:
javascript
let myVar = 10;
console.log(myvar); // ReferenceError: myvar is not defined
In the above code, the attempt to access `myvar` fails because it is not the same as `myVar`.
Common Case Sensitivity Mistakes
Developers often encounter issues due to case sensitivity. Here are some common pitfalls:
- Variable Naming: Confusion between similarly named variables.
- Function Calls: Calling functions with incorrect casing, leading to runtime errors.
- Object Properties: Accessing properties using the wrong case can result in undefined values.
Best Practices for Managing Case Sensitivity
To avoid complications arising from case sensitivity, consider the following best practices:
- Consistent Naming Conventions: Adopt a standard naming convention (e.g., camelCase or snake_case) and apply it consistently throughout your code.
- Use Descriptive Names: Choose descriptive and unique names for variables and functions to minimize confusion.
- Code Reviews: Implement regular code reviews to catch case-related issues early in the development process.
- Linting Tools: Utilize linting tools to enforce naming conventions and catch potential errors related to case sensitivity.
Case Sensitivity in JavaScript Keywords
JavaScript keywords, such as `if`, `else`, `return`, and `function`, are also case-sensitive. This means that using an incorrect case will result in syntax errors. For example:
javascript
If (condition) { // SyntaxError: Unexpected identifier
// code
}
The correct usage would be `if (condition)`.
Summary of Case Sensitivity Rules
Aspect | Description |
---|---|
Identifiers | Case-sensitive; `myVar` is different from `myvar`. |
Function Names | Case-sensitive; `myFunction` and `MyFunction` are different. |
Keywords | Must be in the correct case; `if` is not the same as `If`. |
Object Properties | Accessing properties is case-sensitive; `obj.prop` ≠ `obj.Prop`. |
Understanding and adhering to case sensitivity in JavaScript is crucial for writing error-free and maintainable code. By following established practices and leveraging tools, developers can significantly reduce the risk of issues arising from case-related mistakes.
Understanding JavaScript Case Sensitivity from Expert Perspectives
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “JavaScript is indeed case sensitive, which means that variable names, function names, and other identifiers must be used consistently in terms of casing. For instance, ‘Variable’ and ‘variable’ would be treated as distinct identifiers, potentially leading to bugs if not managed properly.”
Michael Chen (Lead Developer, CodeMaster Solutions). “The case sensitivity of JavaScript is a fundamental aspect that every developer must grasp. It is essential for maintaining clarity and avoiding conflicts in larger codebases, where similar names might exist but differ only in case.”
Linda Thompson (JavaScript Specialist, WebDev Academy). “Understanding that JavaScript is case sensitive is crucial for effective coding practices. This characteristic not only helps in preventing errors but also ensures that code is more readable and maintainable, as developers can create meaningful variable names without worrying about accidental overlaps.”
Frequently Asked Questions (FAQs)
Is JavaScript case sensitive?
Yes, JavaScript is a case-sensitive programming language. This means that variables, function names, and other identifiers must be used consistently in terms of capitalization. For example, `myVariable` and `MyVariable` would be treated as two distinct identifiers.
What are the implications of case sensitivity in JavaScript?
The case sensitivity in JavaScript can lead to errors if identifiers are not used consistently. Developers must be careful to match the exact case when referencing variables or functions to avoid runtime errors or unexpected behavior.
Are other programming languages also case sensitive?
Many programming languages, such as Java, C++, and Python, are case-sensitive as well. However, some languages, like SQL and HTML, are not case-sensitive, which can lead to different coding practices and conventions.
How can I avoid case sensitivity issues in JavaScript?
To avoid case sensitivity issues, establish a consistent naming convention for variables and functions, such as camelCase or snake_case. Additionally, using code linters can help identify potential case-related errors before runtime.
Can I use the same name with different cases in JavaScript?
Yes, you can use the same name with different cases in JavaScript, but it is not recommended. Doing so can lead to confusion and bugs, as the language will treat them as separate entities.
What should I do if I encounter a case sensitivity error?
If you encounter a case sensitivity error, check your code for inconsistencies in capitalization. Ensure that all instances of the variable or function name match in case, and refactor your code to maintain a consistent naming convention.
JavaScript is indeed a case-sensitive programming language, which means that it distinguishes between uppercase and lowercase letters in identifiers, variables, functions, and other elements. This characteristic is crucial for developers to understand, as it affects how code is written and executed. For instance, the variable names “myVariable” and “myvariable” would be treated as two distinct entities, leading to potential errors if a developer inadvertently uses the wrong case.
Understanding the case sensitivity of JavaScript is essential for maintaining code integrity and ensuring that functions and variables are referenced correctly throughout a program. This sensitivity extends to built-in objects and methods as well, where using the incorrect case can result in a failure to execute the desired functionality. Therefore, developers must adhere to consistent naming conventions and be vigilant about the case used in their code.
In summary, the case sensitivity of JavaScript plays a significant role in the language’s functionality and usability. Developers must be aware of this feature to avoid common pitfalls and ensure that their code runs as intended. By adopting best practices in naming conventions and staying attentive to case usage, programmers can enhance the reliability and readability of their JavaScript applications.
Author Profile
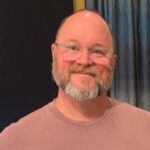
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?