Can You Implement iOS In-App Purchases Within a WebView?
In the ever-evolving landscape of mobile applications, the integration of in-app purchases (IAP) has become a pivotal feature for developers and businesses alike. As iOS continues to dominate the mobile market, understanding how to effectively implement IAP within a webview is crucial for maximizing revenue and enhancing user experience. This article delves into the intricacies of managing in-app purchases in a webview environment, exploring the challenges, best practices, and innovative solutions that can streamline the process. Whether you’re a seasoned developer or just starting your journey in app development, this guide will equip you with the knowledge needed to navigate the complexities of IAP on iOS.
When it comes to in-app purchases, Apple has established strict guidelines that developers must adhere to, especially when dealing with webviews. These guidelines are designed to ensure a seamless user experience while maintaining security and compliance with App Store policies. However, integrating IAP within a webview presents unique challenges, such as handling transactions, managing user authentication, and ensuring that purchases are recognized across different platforms. Understanding these nuances is essential for developers looking to create a successful app that leverages the power of in-app purchases.
Moreover, the rise of hybrid apps and web-based solutions has prompted developers to explore innovative ways to incorporate
Understanding In-App Purchases in iOS WebView
In-app purchases (IAP) enable developers to sell digital goods or services within their applications. On iOS, Apple has specific guidelines regarding IAP transactions that developers must adhere to, particularly when these transactions are conducted within a WebView.
When implementing IAP in a WebView, it’s crucial to understand the limitations imposed by Apple’s App Store Review Guidelines. Apple typically requires that any purchase for digital content or services must go through their in-app purchasing system. This means that developers cannot facilitate purchases directly through a WebView that bypasses Apple’s payment system.
Key Considerations for Implementing IAP in WebView
Developers need to consider several important points when integrating IAP within a WebView:
- Compliance with Apple Guidelines: Ensure that the app complies with Apple’s guidelines regarding IAP, as non-compliance can result in rejection during the App Store review process.
- User Experience: Provide a seamless transition between the WebView and the native app for IAP transactions. Users should be able to make purchases without confusion.
- Security and Privacy: Safeguard users’ payment information and ensure that all transactions are secure.
Implementation Steps
To effectively implement IAP in a WebView environment, follow these steps:
- Set Up In-App Purchases: Register your app for IAP through App Store Connect and define the products you wish to sell.
- Handle the Purchase Flow:
- Launch the WebView for users to browse content.
- Redirect users to a native purchase interface when they choose to make a purchase.
- Use StoreKit for Transactions: Implement StoreKit to handle the purchase process, including payment processing and receipt validation.
Challenges and Solutions
There are several challenges developers may face when integrating IAP in WebView, along with potential solutions:
Challenge | Solution |
---|---|
App Store Rejection | Adhere strictly to Apple’s guidelines. |
User Confusion | Clearly communicate the purchase process. |
Security Risks | Implement SSL and secure payment gateways. |
Best Practices for IAP in WebView
To ensure a successful implementation of IAP in WebView, consider the following best practices:
- Maintain Transparency: Clearly inform users about the need to complete purchases through the app.
- Test Thoroughly: Conduct extensive testing to ensure that the WebView to native app transition is smooth.
- Monitor Feedback: Collect user feedback to improve the purchasing experience continually.
By following these guidelines and best practices, developers can effectively navigate the complexities of implementing in-app purchases within a WebView, ensuring compliance with Apple’s policies and providing a positive user experience.
Understanding In-App Purchases in iOS WebView
In-app purchases (IAPs) in iOS allow developers to sell digital goods and services within their applications. When integrating IAPs into a WebView, developers face unique challenges and considerations that differ from native implementations.
WebView Limitations
Utilizing a WebView for in-app purchases presents several limitations:
- Apple’s Guidelines: Apple mandates that all digital content consumed within an app must use their in-app purchase system. This means that if a WebView is employed to deliver content, it must still adhere to this rule.
- User Experience: Navigating through a WebView can lead to a subpar user experience if not designed properly. Users may find it cumbersome compared to native purchase flows.
- Payment Processing: Payments made through a WebView using non-IAP methods can lead to app rejection during the App Store review process.
Implementation Strategies
To effectively implement IAPs in a WebView, consider the following strategies:
- Native Bridge: Establish a communication bridge between the WebView and the native app. This can allow the WebView to invoke native purchase functions.
- JavaScript Interface: Create a JavaScript interface that can call native functions when a user wants to make a purchase. This will ensure that the native IAP process is invoked.
- Error Handling: Implement robust error handling to manage cases where purchases fail or are interrupted.
Steps to Integrate IAP in WebView
- Set Up IAP in App Store Connect:
- Configure your IAP products in App Store Connect.
- Ensure you have the correct product identifiers.
- Configure the WebView:
- Load your content securely using HTTPS.
- Ensure JavaScript is enabled for interactive elements.
- Create a Native Purchase Flow:
- Use `StoreKit` to manage purchases.
- Implement methods for fetching product information, processing payments, and handling transactions.
- Communicate Between WebView and Native Code:
- Use `WKScriptMessageHandler` to listen for JavaScript messages from the WebView.
- Trigger native purchase processes based on JavaScript calls.
Example Code Snippet
The following snippet illustrates how to set up communication between the WebView and native IAP functions:
“`swift
import WebKit
class ViewController: UIViewController, WKScriptMessageHandler {
var webView: WKWebView!
override func viewDidLoad() {
super.viewDidLoad()
let contentController = WKUserContentController()
contentController.add(self, name: “purchaseProduct”)
let config = WKWebViewConfiguration()
config.userContentController = contentController
webView = WKWebView(frame: self.view.frame, configuration: config)
self.view.addSubview(webView)
if let url = URL(string: “https://yourapp.com”) {
webView.load(URLRequest(url: url))
}
}
func userContentController(_ userContentController: WKUserContentController, didReceive message: WKScriptMessage) {
if message.name == “purchaseProduct”, let productId = message.body as? String {
purchaseProduct(productId: productId)
}
}
func purchaseProduct(productId: String) {
// Implement StoreKit purchase logic here
}
}
“`
Testing and Compliance
Testing in-app purchases requires:
- Sandbox Environment: Use Apple’s sandbox environment to test purchases without real transactions.
- Review Compliance: Ensure that your implementation follows Apple’s guidelines to prevent rejection during the app review process.
- Logging: Implement logging to track purchase attempts and outcomes for better debugging and compliance verification.
By following these guidelines and strategies, developers can effectively integrate in-app purchases into a WebView while adhering to Apple’s requirements and providing a seamless user experience.
Expert Insights on iOS In-App Purchases in WebView
Dr. Emily Chen (Mobile App Development Specialist, Tech Innovators Inc.). “Implementing in-app purchases within a WebView on iOS can present significant challenges, particularly due to Apple’s strict App Store guidelines. Developers must ensure that they comply with the rules surrounding in-app purchases to avoid rejection during the app review process.”
Mark Thompson (Senior iOS Developer, App Solutions Group). “While using WebView for in-app purchases can simplify the integration of web-based content, it is crucial to recognize that Apple mandates the use of their in-app purchase system for digital goods and services. Any workaround could lead to compliance issues and potential removal from the App Store.”
Lisa Patel (Legal Advisor, Digital Commerce Law Firm). “The legal implications of handling in-app purchases through WebView are complex. Developers must be aware of the potential for violating Apple’s policies, which could result in legal disputes or financial penalties. It is advisable to consult with legal experts when navigating these waters.”
Frequently Asked Questions (FAQs)
Can I implement in-app purchases in a WebView on iOS?
No, Apple does not allow in-app purchases to be processed through WebViews. All in-app purchases must be conducted through the native app interface using Apple’s In-App Purchase (IAP) framework.
What are the restrictions for in-app purchases in iOS apps?
iOS apps must use Apple’s IAP system for digital goods and services. Physical goods and services, as well as certain types of content, are exempt from this requirement.
How can I handle subscriptions in my iOS app?
You can handle subscriptions by implementing Apple’s IAP framework directly in your native app. This allows you to manage subscription tiers, billing cycles, and user access effectively.
What happens if I try to bypass Apple’s in-app purchase system?
Attempting to bypass Apple’s IAP system can lead to your app being rejected from the App Store or removed if it is already published. Compliance with Apple’s guidelines is essential for app approval.
Are there alternatives to in-app purchases for web applications on iOS?
Yes, web applications can use external payment methods for physical goods or services. However, for digital content, you must use Apple’s IAP system to remain compliant with App Store policies.
Can I link to a website for purchases within my iOS app?
You can link to external websites for purchases, but you cannot directly promote or facilitate in-app purchases outside of Apple’s IAP system. Doing so may result in App Store rejection.
The integration of in-app purchases (IAP) within a WebView on iOS presents several challenges and considerations for developers. Apple’s App Store guidelines are stringent regarding in-app purchases, primarily requiring that all digital goods and services consumed within an app must utilize Apple’s in-app purchasing system. This requirement complicates the use of WebViews, as developers must ensure compliance with these guidelines to avoid potential app rejections or penalties from Apple.
Moreover, implementing IAP in a WebView involves understanding the technical limitations and user experience implications. WebViews can sometimes lead to a suboptimal user experience compared to native app interfaces, particularly when handling payment processes. Developers must be cautious about maintaining a seamless and secure transaction flow, which is crucial for user trust and retention.
In summary, while it is technically feasible to implement in-app purchases in a WebView on iOS, developers must navigate Apple’s policies and prioritize user experience. Adhering to these guidelines not only ensures compliance but also enhances the overall functionality and acceptance of the app within the App Store ecosystem.
Author Profile
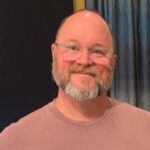
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?