Why Am I Seeing ‘Invalid Use Of Non-Static Member Function’ in My Code?
In the realm of object-oriented programming, the distinction between static and non-static member functions is crucial for effective code design and functionality. Yet, developers often encounter the perplexing error message: “Invalid Use Of Non-Static Member Function.” This seemingly cryptic phrase can halt progress and lead to frustration, especially for those new to programming or transitioning between languages. Understanding the nuances behind this error not only enhances coding proficiency but also deepens one’s grasp of class structures and object behavior.
At its core, the “Invalid Use Of Non-Static Member Function” error arises when a non-static member function is invoked in a context that does not recognize the specific instance of the class it belongs to. Non-static functions, by their very nature, are designed to operate on instances of a class, relying on the state and data encapsulated within those instances. When developers mistakenly attempt to call these functions without an associated object, the compiler raises an alarm, indicating a fundamental misunderstanding of how object-oriented principles work.
This article will delve into the underlying reasons for this error, exploring common scenarios where it occurs and providing clarity on the distinctions between static and non-static functions. By the end, readers will not only be equipped to troubleshoot this error but will also gain a more profound appreciation for the
Understanding Non-Static Member Functions
Non-static member functions are integral to object-oriented programming, allowing for the manipulation of class instances. These functions operate on instance data and require an object of the class to be invoked. When a non-static member function is called, it has access to the instance’s attributes and can modify them.
The key characteristics of non-static member functions include:
- Instance Context: They operate on specific instances, which means they can access instance variables.
- This Pointer: Inside non-static member functions, the `this` pointer is used to refer to the calling object.
- Encapsulation: They promote encapsulation by allowing data to be accessed and modified through methods.
Common Causes of the Error
The error “Invalid Use of Non-Static Member Function” typically arises when developers attempt to call a non-static member function without an instance of the class. Below are some common scenarios leading to this error:
- Direct Call Without Instance: Trying to invoke a non-static member function directly using the class name.
- Static Context: Calling a non-static member function from a static context or static member function without an object reference.
- Misunderstanding Object Scope: Not recognizing that a non-static method requires an object to be invoked.
Example of the Error
Consider the following code snippet that illustrates the error:
“`cpp
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass::display(); // Error: Invalid Use of Non-Static Member Function
return 0;
}
```
In this example, the `display()` function is non-static, but it is being called using the class name `MyClass`, which leads to the error.
Correcting the Error
To resolve this error, ensure that non-static member functions are called on an instance of the class. Here’s the corrected version of the code:
“`cpp
int main() {
MyClass obj; // Create an instance of MyClass
obj.display(); // Correctly call the non-static member function
return 0;
}
“`
In this case, `obj` is an instance of `MyClass`, allowing the `display()` function to be called properly.
Best Practices
To avoid encountering the “Invalid Use of Non-Static Member Function” error, consider the following best practices:
- Always instantiate the class before calling non-static member functions.
- Use appropriate access specifiers to control visibility and encapsulation.
- Understand the distinction between static and non-static member functions to prevent confusion.
Aspect | Static Member Function | Non-Static Member Function |
---|---|---|
Access to Instance Variables | No | Yes |
Called Without Instance | Yes | No |
Usage of ‘this’ Pointer | No | Yes |
Context | Class Level | Instance Level |
Understanding the Error
The “Invalid Use Of Non-Static Member Function” error typically arises in object-oriented programming languages such as C++ when an attempt is made to call a non-static member function without an object of the class. This error can lead to confusion, particularly for those new to object-oriented concepts.
Key characteristics of non-static member functions include:
- Instance Context: They require an instance of the class to be called.
- Access to Instance Variables: They can access and modify instance variables of the class.
- Binding: They are bound to a specific object at runtime.
This error commonly occurs when developers mistakenly try to invoke non-static methods directly through the class name rather than through an instance of the class.
Common Scenarios Leading to the Error
Several typical scenarios can lead to encountering this error:
– **Calling Non-Static Methods from Static Context**: Attempting to call a non-static method within a static method or static block.
“`cpp
class Example {
public:
void nonStaticMethod() { /* code */ }
static void staticMethod() {
nonStaticMethod(); // Error: Invalid use of non-static member function
}
};
“`
– **Using Class Name Instead of Instance**: Invoking a non-static method using the class name.
“`cpp
class Example {
public:
void nonStaticMethod() { /* code */ }
};
Example::nonStaticMethod(); // Error: Invalid use of non-static member function
“`
– **Incorrect Object Reference**: Calling a method on a pointer or reference that is not properly initialized.
“`cpp
class Example {
public:
void nonStaticMethod() { /* code */ }
};
Example* examplePtr; // Not initialized
examplePtr->nonStaticMethod(); // Error: Invalid use of non-static member function
“`
How to Resolve the Error
To resolve the “Invalid Use Of Non-Static Member Function” error, consider the following approaches:
– **Ensure Proper Object Instantiation**: Create an instance of the class before calling the non-static method.
“`cpp
Example example;
example.nonStaticMethod(); // Correct usage
“`
– **Calling from Static Context**: If a non-static method must be called from a static method, either make the method static or instantiate the class.
“`cpp
class Example {
public:
void nonStaticMethod() { /* code */ }
static void staticMethod() {
Example example;
example.nonStaticMethod(); // Correct usage
}
};
“`
– **Check Object Initialization**: Ensure that any pointers or references to objects are properly initialized before invoking methods.
“`cpp
Example* examplePtr = new Example();
examplePtr->nonStaticMethod(); // Correct usage
delete examplePtr; // Clean up memory
“`
Best Practices to Avoid the Error
To minimize the chances of encountering this error in the future, adhere to the following best practices:
- Consistent Object Usage: Always use class instances to call non-static methods.
- Clarity in Code Structure: Keep static and non-static methods clearly separated to avoid confusion.
- Initialize Pointers: Always initialize pointers before usage to prevent dereferencing null or uninitialized pointers.
- Code Review and Testing: Regularly review code and test for edge cases to catch potential errors early.
Implementing these practices can significantly enhance code quality and reduce the occurrence of this type of error.
Understanding the Implications of Invalid Use of Non-Static Member Functions
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “The invalid use of non-static member functions often arises from a misunderstanding of object-oriented principles. It is crucial for developers to recognize that non-static functions require an instance of the class to be invoked, which can lead to runtime errors if mismanaged.”
James Liu (Senior Developer, Code Quality Solutions). “In many cases, the invalid invocation of non-static member functions can indicate poor design choices. Ensuring that functions are appropriately categorized as static or non-static is essential for maintaining code clarity and preventing unexpected behavior.”
Sarah Thompson (Lead Architect, Future Tech Systems). “From an architectural standpoint, the misuse of non-static member functions can complicate the debugging process. It is imperative to establish clear guidelines and best practices to mitigate these issues and enhance overall code reliability.”
Frequently Asked Questions (FAQs)
What does “Invalid Use Of Non-Static Member Function” mean?
This error indicates that a non-static member function is being called in a context where a static context is expected, typically when trying to access the function without an instance of the class.
How can I resolve the “Invalid Use Of Non-Static Member Function” error?
To resolve this error, ensure that you are calling the non-static member function on an instance of the class rather than attempting to call it directly through the class name.
What is the difference between static and non-static member functions?
Static member functions belong to the class itself and can be called without an instance, while non-static member functions require an instance of the class to be invoked, as they operate on instance-specific data.
Can a non-static member function access static members of the class?
Yes, a non-static member function can access static members of the class directly, as static members are shared across all instances of the class.
What happens if I try to call a non-static member function from a static context?
Attempting to call a non-static member function from a static context will result in a compilation error, as there is no instance context available for the non-static function to operate on.
Are there any best practices to avoid this error?
To avoid this error, always ensure that non-static member functions are called on an instance of the class. Additionally, consider using static member functions when instance-specific behavior is not required.
The invalid use of non-static member functions typically arises in object-oriented programming when developers attempt to call a non-static method without an instance of the class. Non-static member functions are designed to operate on instances of a class, meaning they require an object to be invoked. Attempting to call such functions in a static context, where no instance exists, leads to compilation errors and highlights a fundamental misunderstanding of class design principles.
One of the key takeaways from this discussion is the importance of understanding the distinction between static and non-static members in a class. Static members belong to the class itself and can be accessed without creating an instance, while non-static members are tied to specific instances. This distinction is crucial for maintaining proper encapsulation and ensuring that object-oriented principles are upheld in software design.
Furthermore, developers should always ensure that they are invoking non-static member functions on valid instances of their classes. This practice not only prevents errors but also enhances code readability and maintainability. By adhering to these principles, programmers can create more robust and error-free applications, ultimately leading to better software quality and performance.
Author Profile
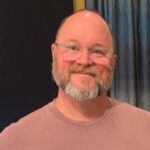
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?