Why Am I Seeing InsecureRequestWarning: Unverified HTTPS Requests to Hosts?
In today’s digital landscape, where security breaches and data privacy concerns are at an all-time high, the integrity of online communications has never been more critical. As developers and users alike navigate the complexities of web interactions, a common warning has emerged: the `InsecureRequestWarning`. This alert serves as a crucial reminder that unverified HTTPS requests can expose sensitive information and compromise system security. Understanding the implications of this warning is essential for anyone involved in web development or cybersecurity, as it highlights the importance of secure connections in an increasingly interconnected world.
At its core, the `InsecureRequestWarning` is a notification that arises when an application attempts to make an HTTPS request without proper verification of the SSL certificate. This situation can occur for various reasons, such as self-signed certificates or misconfigured servers. While it may seem like a minor inconvenience, ignoring this warning can lead to significant vulnerabilities, leaving systems open to man-in-the-middle attacks and data interception. As the reliance on web applications grows, so does the need for developers to prioritize secure coding practices and adhere to best practices for handling HTTPS requests.
In this article, we will delve into the nuances of the `InsecureRequestWarning`, exploring its causes, implications, and how to effectively address it. By equipping yourself with the
Understanding InsecureRequestWarning
The `InsecureRequestWarning` is a warning generated by the `requests` library in Python when an HTTPS request is made to a host that does not have a valid SSL certificate. This warning serves as a critical reminder for developers to ensure that their applications communicate securely over the internet. Ignoring these warnings could expose applications to various security risks, including man-in-the-middle attacks.
Causes of InsecureRequestWarning
Several factors can lead to the generation of an `InsecureRequestWarning`:
- Self-signed SSL certificates: These certificates are not signed by a trusted certificate authority (CA), making them unverified.
- Expired SSL certificates: Certificates that have reached their expiration date no longer provide a valid assurance of security.
- Domain mismatch: The SSL certificate does not match the domain name of the website being accessed, indicating a potential security threat.
- Untrusted certificate authorities: Some SSL certificates are issued by CAs that are not recognized by the system, leading to warnings.
How to Handle InsecureRequestWarning
To address the `InsecureRequestWarning`, developers have a few options:
- Verify SSL Certificates: Ensure that the SSL certificates of the servers you are connecting to are valid and signed by a trusted CA.
- Suppress the Warning: If necessary, you can suppress the warning, although this is not recommended for production environments. This can be done by using:
“`python
import requests
from requests.exceptions import InsecureRequestWarning
requests.packages.urllib3.disable_warnings(InsecureRequestWarning)
“`
- Use a Valid Certificate: If you control the server, consider obtaining a valid SSL certificate from a trusted CA.
- Environment Configuration: Use environment variables to configure SSL verification settings to manage how SSL certificates are handled in your application.
Table of SSL Certificate Validation Steps
Step | Description |
---|---|
1 | Check the certificate’s validity period to ensure it is not expired. |
2 | Verify that the certificate is signed by a trusted certificate authority. |
3 | Ensure the domain name matches the certificate. |
4 | Review the certificate chain for any broken links or issues. |
Best Practices for Secure HTTPS Requests
To ensure secure communication in your applications, consider the following best practices:
- Always use HTTPS for communication between clients and servers.
- Regularly update your SSL certificates and verify their validity.
- Educate your team on the importance of SSL certificate management.
- Implement error handling for SSL verification failures in your applications.
- Utilize security tools that can monitor and alert on SSL certificate issues.
By adhering to these practices, you can minimize the risk of encountering `InsecureRequestWarning` and enhance the overall security of your applications.
Understanding InsecureRequestWarning
InsecureRequestWarning is an alert generated by the Python `requests` library when an HTTPS request is made to a server that cannot be verified. This warning indicates potential security risks, as it suggests that the SSL certificate of the host is either self-signed, expired, or not recognized by the client’s trusted certificate authorities.
Common Causes of InsecureRequestWarning
The warning can arise from several scenarios, including:
- Self-signed SSL certificates: The server uses a certificate not issued by a trusted certificate authority (CA).
- Expired certificates: The SSL certificate has surpassed its validity period.
- Certificate misconfiguration: The server’s SSL certificate does not match the host name.
- Untrusted CAs: The certificate is issued by a CA that is not recognized by the client’s environment.
Impact on Applications
Ignoring InsecureRequestWarning can lead to serious security vulnerabilities, such as:
- Data interception: Attackers may intercept sensitive data transmitted over an insecure connection.
- Man-in-the-middle attacks: Unscrupulous entities could potentially manipulate data exchanges.
- Loss of user trust: Users may lose confidence in applications that compromise security.
Handling InsecureRequestWarning
To manage this warning effectively, developers can choose from several approaches:
- Verify SSL Certificates: Ensure that your server uses a valid certificate from a trusted CA.
- Disable Warnings: Temporarily suppress the warning by using the following code snippet, though this is not recommended for production environments:
“`python
import requests
from requests.packages.urllib3.exceptions import InsecureRequestWarning
requests.packages.urllib3.disable_warnings(InsecureRequestWarning)
“`
- Use `verify` Parameter: Specify the path to a CA_BUNDLE file or directory with certificates of trusted CAs.
“`python
response = requests.get(‘https://example.com’, verify=’/path/to/certfile’)
“`
Best Practices for Secure Requests
To avoid InsecureRequestWarning and enhance the security of your applications, consider implementing these best practices:
- Always use HTTPS: Ensure all communications are conducted over secure protocols.
- Regularly Update Certificates: Monitor and update SSL certificates before they expire.
- Employ Certificate Pinning: Limit connections to a specific server’s certificate to combat man-in-the-middle attacks.
- Utilize Security Scanning Tools: Regularly scan applications for vulnerabilities related to SSL/TLS configurations.
By understanding the implications of InsecureRequestWarning and taking proactive measures, developers can significantly bolster the security posture of their applications, ensuring safe data transmission and maintaining user trust.
Understanding Insecure Request Warnings in HTTPS Communication
Dr. Emily Carter (Cybersecurity Analyst, SecureNet Solutions). “InsecureRequestWarning is a crucial alert for developers, indicating that an unverified HTTPS request is being made. This warning serves as a reminder to ensure that all communications are secure, as failing to do so can expose sensitive data to potential interception.”
Michael Tran (Lead Software Engineer, TechGuard Innovations). “Ignoring the InsecureRequestWarning can lead to significant security vulnerabilities. Developers should always validate SSL certificates and implement proper error handling to mitigate risks associated with unverified requests.”
Sarah Patel (Information Security Consultant, CyberSafe Advisors). “The InsecureRequestWarning highlights the importance of trust in digital communications. Organizations must prioritize secure coding practices and educate their teams about the implications of making unverified HTTPS requests.”
Frequently Asked Questions (FAQs)
What does the warning “InsecureRequestWarning: Unverified HTTPS Request Is Being Made To Host” mean?
This warning indicates that a request is being made to a server over HTTPS without verifying the server’s SSL certificate. This can expose the connection to potential security risks, such as man-in-the-middle attacks.
Why am I receiving this warning in my application?
You are likely receiving this warning because the code is attempting to make an HTTPS request without validating the SSL certificate of the server. This can occur if the server’s certificate is self-signed or if the certificate authority is not recognized.
How can I resolve the “InsecureRequestWarning” in my code?
To resolve this warning, you can either verify the server’s SSL certificate by providing the correct CA bundle or disable the warning by setting `verify=` in your request. However, disabling verification is not recommended for production environments.
Is it safe to ignore the “InsecureRequestWarning”?
Ignoring this warning is not advisable, as it indicates a potential security vulnerability. It is crucial to ensure that SSL certificates are properly validated to maintain secure communications.
What are the implications of making unverified HTTPS requests?
Making unverified HTTPS requests can lead to data interception, unauthorized access, and exposure to various cyber threats. It undermines the security guarantees that HTTPS is designed to provide.
Can I suppress the “InsecureRequestWarning” in my application?
Yes, you can suppress the warning by using the `urllib3` library’s `disable_warnings` function. However, this should be done with caution, as it does not address the underlying security issue.
The warning message “InsecureRequestWarning: Unverified HTTPS Request Is Being Made To Host” typically arises when making HTTPS requests in Python using libraries like `requests` without verifying the SSL certificates. This warning serves as a critical alert to developers that the connection to the server is not secure, potentially exposing sensitive data to interception or manipulation. Ignoring this warning can lead to significant security vulnerabilities, especially when handling user credentials or personal information.
To mitigate this warning, developers have a few options. They can choose to verify SSL certificates by ensuring that the server’s certificate is valid and trusted. This can be done by setting the `verify` parameter to `True` in the request or by providing a path to a CA_BUNDLE file or directory. Alternatively, if the situation allows for it, developers can suppress the warning by setting the `verify` parameter to “, although this is not recommended for production environments due to the associated security risks.
addressing the “InsecureRequestWarning” is crucial for maintaining the integrity and security of applications that rely on HTTPS connections. Developers should prioritize secure practices by verifying SSL certificates and understanding the implications of making unverified requests. By doing so, they can protect both their applications and their
Author Profile
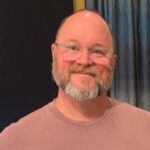
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?