Why Am I Seeing ‘Initializing’: Cannot Convert From ‘Bool’ Error and How Can I Fix It?
In the world of programming, encountering errors is an inevitable part of the development process. One such error that can leave developers scratching their heads is the infamous message: “Initializing: Cannot Convert From ‘Bool’.” This seemingly cryptic notification often arises in languages like Cor Java when there’s a mismatch in expected data types. For both novice and seasoned programmers, understanding the nuances behind this error can not only save time but also enhance coding practices. In this article, we will delve into the intricacies of this error message, exploring its common causes, implications, and strategies for resolution.
Overview
At its core, the “Cannot Convert From ‘Bool'” error signifies a type mismatch, where a boolean value is mistakenly assigned or passed to a variable or method that expects a different data type. This can happen in various scenarios, such as when initializing variables, passing parameters to functions, or returning values from methods. The implications of this error can range from minor bugs to significant disruptions in application logic, making it crucial for developers to grasp the underlying principles of type safety and conversion.
Moreover, the error serves as a reminder of the importance of understanding data types within programming languages. Each language has its own set of rules and conventions regarding data types, and failing
Common Causes of the Error
The error message “Initializing: Cannot Convert From ‘Bool'” typically arises in programming environments when a boolean value is being incorrectly assigned or passed to a function or variable that expects a different data type. The key scenarios leading to this error include:
- Type Mismatch: The most frequent cause is attempting to assign a boolean (`true` or “) where a different type, such as an integer or string, is expected.
- Function Parameters: If a function is defined to accept a specific parameter type, passing a boolean where another type is required will trigger this error.
- Implicit Conversion Failures: Some programming languages do not automatically convert between types. For instance, languages like Cand Java are strongly typed and require explicit conversion.
Examples of the Error
To better understand the error, consider the following examples in different programming contexts:
- CExample:
“`csharp
bool isAvailable = true;
int availableStatus = isAvailable; // Error: Cannot convert from ‘bool’ to ‘int’
“`
- Java Example:
“`java
boolean isReady = ;
String status = isReady; // Error: incompatible types: boolean cannot be converted to String
“`
These examples illustrate how the type system in strongly typed languages enforces rules that prevent implicit conversions.
Resolving the Error
To resolve this error, you can implement the following strategies:
- Explicit Conversion: Convert the boolean value to the expected type explicitly. For instance, in C, you can use:
“`csharp
int availableStatus = isAvailable ? 1 : 0; // Convert bool to int
“`
- Change Variable Types: Ensure that the variable receiving the value matches the type of the value being assigned. For example:
“`java
boolean isReady = ;
boolean status = isReady; // Corrected to the same type
“`
- Check Function Signatures: Review the function signature and ensure that the arguments being passed match the expected types.
Best Practices to Avoid Errors
To prevent encountering the “Cannot Convert From ‘Bool'” error in the future, consider the following best practices:
- Use Strong Typing: Choose languages that enforce strong typing, which helps catch these errors at compile time.
- Consistent Data Types: Use consistent data types across your application to minimize type-related errors.
- Code Reviews: Regularly review code with peers to catch type mismatches early in the development process.
Error Handling Strategies
Implementing robust error handling can also help manage and debug such issues effectively. Below are some strategies:
- Try-Catch Blocks: Use try-catch statements to handle exceptions gracefully.
- Logging: Log errors with detailed messages to help identify the source of type mismatches.
- Unit Testing: Write unit tests that cover various scenarios, including edge cases, to ensure type safety.
Error Type | Description | Solution |
---|---|---|
Type Mismatch | Assigning a boolean to an incompatible type | Use explicit conversion or change variable type |
Function Parameter Error | Passing incorrect type to a function | Check and match function parameter types |
Implicit Conversion Error | Failing to convert types automatically | Manually convert types as needed |
Error Context and Explanation
The error message `Initializing’: Cannot Convert From ‘Bool` typically arises in programming environments, particularly in languages like Cor C++. This issue often occurs when a boolean value is expected, but the initialization context does not support it. Understanding the root causes can help developers rectify the error efficiently.
- Common Scenarios:
- Assigning a boolean to a non-boolean type.
- Misusing conditional expressions in initializations.
- Incorrectly structured code that leads to type mismatches.
Debugging Steps
To resolve the `Cannot Convert From ‘Bool` error, follow these debugging steps:
- Review Variable Declarations:
- Ensure that the variable being initialized is of the correct type.
- Check for any implicit conversions that may lead to type conflicts.
- Check Function Returns:
- If the error is associated with a function return, verify that the function explicitly returns a boolean type if that is required.
- Confirm that the function call matches the expected return type.
- Examine Conditional Statements:
- Look at if-else statements or ternary operations for proper boolean evaluations.
- Ensure that the conditions are evaluating to a boolean rather than a different type.
Code Examples
The following examples illustrate typical scenarios that lead to this error:
Example 1: Incorrect Initialization
“`csharp
int isActive = true; // Error: Cannot convert from ‘bool’ to ‘int’
“`
Correction
“`csharp
bool isActive = true; // Correct: ‘isActive’ is of type bool
“`
Example 2: Function Return Issue
“`csharp
public int CheckStatus()
{
return true; // Error: Cannot convert from ‘bool’ to ‘int’
}
“`
Correction
“`csharp
public bool CheckStatus()
{
return true; // Correct: Function now returns bool
}
“`
Best Practices to Avoid Errors
Implementing certain best practices can help mitigate the occurrence of this error:
- Type Consistency: Always declare variables with the correct data types and ensure functions return the expected types.
- Code Reviews: Regular code reviews can help catch type mismatches early in the development process.
- Unit Testing: Implement unit tests that specifically check for type-related issues during the initialization phase.
Tools and Resources
Utilizing various tools and resources can further assist in identifying and resolving type conversion issues:
Tool/Resource | Description |
---|---|
IDE Debugger | Use the debugger in your Integrated Development Environment to step through the code. |
Static Code Analyzers | Tools like ReSharper for .NET or SonarQube can catch type issues early. |
Online Documentation | Refer to language-specific documentation for type conversion guidelines and examples. |
By adhering to these guidelines and leveraging the right tools, developers can effectively address and prevent the `Cannot Convert From ‘Bool` error in their coding practices.
Understanding the ‘Cannot Convert From Bool’ Error in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Cannot Convert From Bool’ error typically arises when there is a mismatch between expected data types in programming languages. It is crucial to ensure that boolean values are being used in contexts where they are appropriate, such as conditional statements or logical operations.”
Mark Thompson (Lead Developer, CodeMaster Solutions). “This error often indicates that a boolean value is being incorrectly assigned to a variable that expects a different data type. Developers should carefully check their variable declarations and ensure that type conversions are handled properly to prevent such issues.”
Lisa Nguyen (Programming Instructor, Code Academy). “Understanding type safety is essential in modern programming. The ‘Cannot Convert From Bool’ error serves as a reminder to programmers to be vigilant about type compatibility, particularly in languages that enforce strict type checking.”
Frequently Asked Questions (FAQs)
What does the error ‘Cannot Convert From ‘Bool’ mean?
This error typically occurs in programming languages like Cwhen there is an attempt to assign a boolean value to a variable or parameter that expects a different data type, such as an integer or string.
How can I resolve the ‘Cannot Convert From ‘Bool’ error?
To resolve this error, ensure that the variable or parameter you are assigning the boolean value to is of the correct data type. If necessary, convert the boolean to the appropriate type using methods like `Convert.ToInt32()` for integers.
In which scenarios is this error commonly encountered?
This error is commonly encountered during conditional statements, method calls, or when manipulating data structures that expect specific types, particularly when using frameworks or libraries that enforce strict type checking.
Can this error occur in any programming language?
While the specific error message may vary, similar type conversion errors can occur in many programming languages that enforce strong typing, including C, Java, and Swift.
Is there a way to prevent the ‘Cannot Convert From ‘Bool’ error?
To prevent this error, always ensure that the data types of variables and parameters match the expected types in your code. Implementing type checks or using explicit conversions can also help mitigate this issue.
What tools can assist in identifying type conversion errors?
Integrated Development Environments (IDEs) like Visual Studio or JetBrains Rider provide real-time error detection and suggestions, which can help identify and resolve type conversion errors effectively. Additionally, static code analysis tools can assist in catching these issues during development.
The error message “Initializing’: Cannot Convert From ‘Bool” typically arises in programming environments, particularly in languages like Cor similar, where type safety is enforced. This error indicates that there is an attempt to assign a boolean value to a variable or parameter that expects a different data type. Understanding the context in which this error occurs is crucial for effective debugging and code correction.
One of the main points to consider is the importance of data type compatibility in programming. When initializing variables or passing parameters to functions, developers must ensure that the data types match the expected types. This requires a careful review of the variable declarations and function signatures to avoid such type conversion errors. Utilizing strong typing can help catch these issues at compile time, preventing runtime errors.
Another key takeaway is the significance of clear error messages in guiding developers towards resolving issues. The error message serves as a prompt to review the code and identify mismatches in data types. Additionally, leveraging integrated development environments (IDEs) that provide real-time feedback and suggestions can significantly reduce the occurrence of such errors. By adhering to best practices in type management, programmers can enhance the reliability and maintainability of their code.
Author Profile
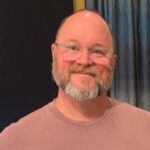
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?