How Can I Resolve the ‘Index Signature for Type ‘string’ Is Missing in Type’ Error in TypeScript?
In the world of TypeScript, the balance between flexibility and type safety can sometimes lead to perplexing errors. One such error that developers frequently encounter is the dreaded message: “Index signature for type ‘string’ is missing in type.” This seemingly cryptic notification can halt your coding progress and leave you scratching your head, wondering what went wrong. Understanding this error is crucial for anyone looking to harness the full power of TypeScript’s type system while maintaining clean and efficient code.
At its core, this error arises from TypeScript’s strict enforcement of types, particularly when dealing with objects and their properties. When you attempt to access or assign values to properties in an object that lack a defined index signature, TypeScript throws a fit, insisting that you clarify your intentions. This can be particularly frustrating for developers accustomed to the more lenient nature of JavaScript, where such constraints are absent. However, this strictness is what makes TypeScript a powerful tool for building robust applications.
As we delve deeper into this topic, we will explore the underlying principles of index signatures, how they function within the TypeScript type system, and practical strategies for resolving the error. Whether you’re a seasoned TypeScript veteran or a newcomer to the language, understanding how to navigate this error will enhance
Understanding the Index Signature Error
When working with TypeScript, you may encounter the error message stating that an “Index signature for type ‘string’ is missing in type.” This error typically arises when attempting to access properties on an object that does not have a defined index signature for the specified type. An index signature is a way to define the types of keys and values for an object, allowing for dynamic property access.
What Causes the Error?
The error usually occurs due to:
- Accessing a property that is not explicitly defined: If you try to access a property on an object that TypeScript does not recognize, it will throw this error.
- Inadequate type definitions: When the type of an object does not include an index signature, TypeScript cannot infer that the object can accept dynamic string keys.
Example Scenario
Consider the following example where the error can arise:
“`typescript
interface User {
name: string;
age: number;
}
const user: User = {
name: “John Doe”,
age: 30,
};
// Error: Index signature for type ‘string’ is missing in type ‘User’.
console.log(user[“address”]);
“`
In this scenario, the interface `User` does not define an index signature, leading to an error when attempting to access the `address` property.
How to Fix the Error
To resolve this issue, you can define an index signature in your interface. This allows for dynamic property access while maintaining type safety.
Adding an Index Signature
Here’s how you can modify the `User` interface to include an index signature:
“`typescript
interface User {
name: string;
age: number;
[key: string]: any; // Index signature
}
“`
With the index signature added, you can now access dynamic properties without encountering an error:
“`typescript
const user: User = {
name: “John Doe”,
age: 30,
address: “123 Main St”
};
console.log(user[“address”]); // Outputs: 123 Main St
“`
Key Takeaways
- Index Signature: It allows an object to have dynamic properties defined as string keys.
- Type Safety: Always specify the type of values for index signatures to maintain type safety.
Example Table of TypeScript Index Signatures
Interface | Index Signature | Usage |
---|---|---|
User | [key: string]: any | Allows dynamic properties like address, phone, etc. |
Product | [key: string]: string | number | Enables adding properties for product details like SKU, price, etc. |
By implementing an index signature in your TypeScript interfaces, you can avoid the “Index signature for type ‘string’ is missing in type” error and facilitate dynamic property access while maintaining robust type definitions.
Understanding the Error
The error message “Index signature for type ‘string’ is missing in type” typically arises in TypeScript when an object does not conform to a defined interface or type that expects certain properties. This issue often occurs when you are trying to access properties of an object using a string index, but the TypeScript type system cannot guarantee that those properties exist.
Common Scenarios Leading to This Error
- Missing Properties: The object lacks properties that are expected based on its type definition.
- Incorrect Type Assignments: Assigning an object of a different type that does not match the expected index signature.
- Using Literal Types: When using literal types instead of a broader index signature, the TypeScript compiler raises an error if the property is not explicitly defined.
Index Signature Explanation
An index signature allows you to define the shape of an object when you do not know all the property names in advance. It can be defined as follows:
“`typescript
interface MyObject {
[key: string]: number; // This means any property key of type string will have a value of type number
}
“`
Benefits of Using Index Signatures
- Flexibility: They enable objects to have dynamic property names.
- Type Safety: They ensure that the values associated with dynamic keys adhere to specified types.
How to Resolve the Error
To fix the “Index signature for type ‘string’ is missing in type” error, consider the following approaches:
Define an Appropriate Index Signature
Ensure that the interface or type includes an index signature that accommodates the properties you intend to use.
“`typescript
interface MyObject {
[key: string]: number; // This includes an index signature
specificKey: number; // This can still have specific keys
}
“`
Use Type Assertions
If you are certain about the type structure but TypeScript is unable to infer it, you can use type assertions to bypass the error:
“`typescript
const obj = {} as MyObject; // Assert the type explicitly
obj[‘dynamicKey’] = 42; // No error, as the type is asserted
“`
Explicitly Define All Properties
If the object should only have specific known properties, define them explicitly instead of using an index signature.
“`typescript
interface MyObject {
specificKey1: number;
specificKey2: number;
}
“`
Example Code Snippet
The following example illustrates a scenario that leads to the error and how to resolve it:
“`typescript
interface UserScores {
[username: string]: number; // Index signature
}
const scores: UserScores = {
alice: 10,
bob: 20,
};
// This will cause an error if ‘charlie’ is not defined in the type
const charlieScore = scores[‘charlie’]; // Error: Index signature for type ‘string’ is missing
“`
To resolve it:
“`typescript
// Ensure that scores can accommodate any username
scores[‘charlie’] = 30; // Now it works without error
“`
Best Practices
To mitigate the occurrence of this error in your TypeScript code, consider the following best practices:
- Utilize Index Signatures Wisely: Only use them when necessary, as they can make your type definitions less strict.
- Keep Type Definitions Updated: Regularly review and update type definitions as your code evolves.
- Use Type Checking Tools: Leverage TypeScript’s compiler options to catch potential errors early during development.
By adhering to these practices, you can enhance the robustness of your TypeScript code and minimize index signature-related errors.
Understanding TypeScript’s Index Signature Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “The error message ‘Index Signature For Type ‘string’ Is Missing In Type’ typically arises when a TypeScript interface lacks a defined index signature. This can lead to runtime errors if properties are accessed dynamically without proper type definitions.”
Michael Chen (TypeScript Specialist, CodeCraft Academy). “To resolve this issue, developers should ensure that their interfaces include an index signature that accommodates the expected types. This not only prevents the error but also enhances code maintainability and type safety.”
Sarah Thompson (Lead Developer, NextGen Apps). “Understanding the implications of index signatures is crucial for TypeScript users. When you encounter this error, consider revisiting your interface definitions and ensuring they align with the dynamic nature of your data structures.”
Frequently Asked Questions (FAQs)
What does the error “Index Signature For Type ‘string’ Is Missing In Type” mean?
This error indicates that a TypeScript object is expected to have an index signature for string keys, but the type definition does not include one. It typically arises when trying to access properties dynamically on an object that lacks the necessary index signature.
How can I resolve the “Index Signature For Type ‘string’ Is Missing In Type” error?
To resolve this error, you can define an index signature in your type declaration. For example, you can add `[key: string]: valueType;` to your interface or type definition to allow for dynamic string keys.
When should I use an index signature in TypeScript?
You should use an index signature when you need to allow an object to have properties with dynamic names that are not known at compile time. This is common in scenarios involving dictionaries or maps where keys are not predetermined.
Can I use an index signature with specific property types?
Yes, you can use an index signature with specific property types. However, all properties accessed via the index signature must conform to the defined type. For example, you can have an index signature that specifies all values must be of type `number` or `string`.
What are the implications of using index signatures on performance?
Using index signatures does not significantly impact performance, but it can lead to less strict type checking. This may allow for more flexibility in your code, but it also increases the risk of runtime errors if not managed carefully.
Are there any alternatives to using index signatures in TypeScript?
Alternatives to using index signatures include defining a more specific type for known properties or using mapped types to create a more structured approach. This can enhance type safety while still allowing for some level of dynamic property access.
The error message “Index Signature For Type ‘string’ Is Missing In Type” typically arises in TypeScript when an object is expected to have an index signature, but the defined type does not accommodate for it. This situation often occurs when developers attempt to access properties of an object using dynamic keys, yet the type definition does not permit such access. Understanding this error is crucial for ensuring type safety and preventing runtime errors in applications.
One of the primary reasons for this error is the lack of an index signature in the type definition. An index signature allows an object to have properties with dynamic keys, enabling the use of strings or numbers as keys. To resolve this issue, developers can define an index signature in their type, thus allowing for the intended flexibility in property access. This adjustment not only resolves the error but also enhances the robustness of the code by clarifying the expected structure of the object.
Another key takeaway is the importance of thorough type definitions in TypeScript. By explicitly defining the shape of objects and their properties, developers can leverage TypeScript’s type-checking capabilities to catch potential errors during development. This practice leads to more maintainable code and reduces the likelihood of encountering similar issues in the future. Overall, addressing the “Index Signature
Author Profile
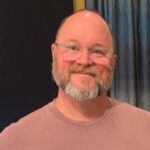
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?