How Can You Write Effective Python Scripts: A Step-by-Step Guide?
How To Write Python Scripts: A Beginner’s Guide to Unlocking the Power of Automation
In a world increasingly driven by technology, the ability to write scripts in Python can be a game-changer. Whether you’re a budding programmer, a data analyst, or simply someone looking to automate mundane tasks, mastering Python scripting opens up a realm of possibilities. With its intuitive syntax and versatile applications, Python has become one of the most popular programming languages today. This article will guide you through the essentials of writing Python scripts, empowering you to harness the full potential of this powerful tool.
Writing Python scripts is not just about coding; it’s about solving problems and streamlining processes. From automating repetitive tasks to analyzing data and creating web applications, Python scripts can significantly enhance productivity and efficiency. As you embark on this journey, you’ll discover the fundamental concepts of scripting, including variables, control structures, and functions, which serve as the building blocks of your programming toolkit.
Moreover, Python’s rich ecosystem of libraries and frameworks provides endless opportunities for expansion and creativity. As you learn to write scripts, you’ll also explore how to leverage these resources to tackle real-world challenges. Whether you’re interested in web scraping, data manipulation, or even game development, understanding how to write Python scripts will equip you with the
Understanding Python Syntax
Python syntax is crucial for writing effective scripts. It is known for its readability and simplicity, which allows programmers to express concepts in fewer lines of code than in many other languages. Here are some key elements of Python syntax:
– **Indentation**: Python uses indentation to define the structure of the code. Proper indentation is essential for defining blocks of code, such as loops and functions.
– **Comments**: Use the “ symbol to add comments in your scripts. Comments are ignored by the interpreter and are useful for explaining code.
– **Variables**: Variables in Python do not require an explicit declaration to reserve memory space. The declaration happens automatically when a value is assigned to a variable.
Example of a simple script demonstrating basic syntax:
“`python
This is a comment
x = 5 Assigning value to a variable
if x > 0:
print(“Positive number”)
“`
Data Types and Variables
Python supports various data types, making it versatile for different programming tasks. Understanding these data types is essential for effective script writing.
- Numeric Types: Integers (`int`), floating-point numbers (`float`), and complex numbers (`complex`).
- Sequence Types: Strings (`str`), lists (`list`), and tuples (`tuple`).
- Mapping Type: Dictionaries (`dict`).
- Set Types: Sets (`set`).
- Boolean Type: Boolean values (`True` or “).
Here is a summary table of common data types in Python:
Data Type | Description |
---|---|
int | Integer values (e.g., 1, 2, -3) |
float | Floating-point numbers (e.g., 1.0, -2.5) |
str | Strings, or text (e.g., “Hello, World!”) |
list | Ordered collection of items (e.g., [1, 2, 3]) |
dict | Key-value pairs (e.g., {“key”: “value”}) |
Control Flow in Python
Control flow statements allow you to dictate the execution order of your scripts. The primary control flow statements in Python are:
– **Conditional Statements**: Use `if`, `elif`, and `else` to execute code blocks based on conditions.
– **Loops**: The `for` loop iterates over a sequence, while the `while` loop continues as long as a condition is true.
Example of control flow:
“`python
number = 10
if number > 0:
print(“Positive”)
elif number < 0:
print("Negative")
else:
print("Zero")
for i in range(5):
print(i)
```
Functions and Modules
Functions are reusable pieces of code that perform a specific task. They help in structuring code and avoiding repetition. In Python, you define a function using the `def` keyword.
- Defining a Function: Use the `def` keyword followed by the function name and parentheses.
- Modules: Python supports modular programming. You can create modules (files containing Python code) and import them into your scripts to use predefined functions and classes.
Example of a function and module usage:
“`python
def greet(name):
return f”Hello, {name}!”
print(greet(“Alice”))
“`
To use a module, save it as `mymodule.py`:
“`python
mymodule.py
def add(a, b):
return a + b
“`
Then, you can import and use it in another script:
“`python
import mymodule
result = mymodule.add(3, 5)
print(result)
“`
This structure not only enhances code readability but also promotes code reuse across different projects.
Setting Up Your Environment
To write Python scripts effectively, it is essential to set up your development environment. This includes installing Python, selecting a code editor, and configuring necessary libraries.
- Install Python
- Download the latest version from the [official Python website](https://www.python.org/downloads/).
- Follow the installation instructions specific to your operating system (Windows, macOS, Linux).
- Ensure to check the box that adds Python to your system PATH during installation.
- Choose a Code Editor
Select a code editor or Integrated Development Environment (IDE) that suits your workflow. Popular choices include:
- Visual Studio Code: Lightweight, customizable, and supports extensions.
- PyCharm: Feature-rich IDE with advanced functionalities for professional developers.
- Jupyter Notebook: Ideal for data analysis and visualization tasks.
- Install Necessary Libraries
Use `pip`, Python’s package manager, to install libraries that enhance functionality. Common libraries include:
- `numpy`: For numerical operations.
- `pandas`: For data manipulation and analysis.
- `requests`: For making HTTP requests.
Writing Your First Script
Once your environment is set up, you can create your first Python script. Follow these steps:
- Create a New File
- Open your chosen code editor.
- Create a new file with a `.py` extension, for example, `hello.py`.
- Write Your Code
Input the following code to print “Hello, World!” to the console:
“`python
print(“Hello, World!”)
“`
- Run Your Script
- Open a terminal or command prompt.
- Navigate to the directory where your script is saved.
- Execute the script with the command:
“`bash
python hello.py
“`
Understanding Python Syntax
Familiarity with Python syntax is crucial for effective scripting. Here are some fundamental elements:
– **Variables**: Store data values. Example:
“`python
name = “Alice”
age = 30
“`
– **Data Types**: Common types include:
Type | Description | Example |
---|---|---|
String | Text data | `”Hello”` |
Integer | Whole numbers | `42` |
Float | Decimal numbers | `3.14` |
Boolean | True or values | `True` |
– **Control Structures**: Direct the flow of the program. Examples include:
– **If Statements**:
“`python
if age >= 18:
print(“Adult”)
else:
print(“Minor”)
“`
- For Loops:
“`python
for i in range(5):
print(i)
“`
Debugging and Error Handling
Debugging is a vital part of scripting. Use the following techniques to identify and fix issues:
- Print Statements: Insert `print()` functions to track variable values and program flow.
- Exceptions: Handle errors gracefully using try-except blocks:
“`python
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero.”)
“`
- Debugging Tools: Utilize built-in debuggers in IDEs like PyCharm or Visual Studio Code to step through code.
Best Practices for Writing Scripts
Implementing best practices ensures your code is clean, efficient, and maintainable. Consider the following guidelines:
- Use Meaningful Variable Names: Choose descriptive names that convey purpose, e.g., `total_price` instead of `tp`.
- Comment Your Code: Provide explanations for complex logic using comments. Example:
“`python
Calculate total price including tax
total_price = price + (price * tax_rate)
“`
- Organize Code with Functions: Break down code into reusable functions for better structure:
“`python
def calculate_tax(price, tax_rate):
return price * tax_rate
“`
By adhering to these principles, you will enhance the readability and usability of your Python scripts.
Expert Insights on Writing Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovators Inc.). “Writing Python scripts requires a clear understanding of both the syntax and the problem you are trying to solve. It is essential to start with a well-defined plan and break down the tasks into manageable functions to enhance code readability and maintainability.”
Michael Chen (Lead Python Developer, Data Solutions Group). “To write effective Python scripts, one must leverage the extensive libraries available. Utilizing libraries such as Pandas for data manipulation or Requests for API interactions can significantly streamline your coding process and improve efficiency.”
Sarah Lopez (Python Programming Instructor, Code Academy). “I always emphasize the importance of testing when writing Python scripts. Implementing unit tests from the outset not only helps identify bugs early but also ensures that your code remains functional as you add new features or refactor existing ones.”
Frequently Asked Questions (FAQs)
What are the basic steps to write a Python script?
To write a Python script, start by installing Python on your computer. Next, choose a text editor or an Integrated Development Environment (IDE) such as PyCharm or VSCode. Create a new file with a `.py` extension, then write your Python code. Finally, save the file and run the script using the command line or the IDE’s run feature.
How do I execute a Python script from the command line?
To execute a Python script from the command line, navigate to the directory containing your script using the `cd` command. Then, type `python script_name.py` or `python3 script_name.py` depending on your Python installation, and press Enter to run the script.
What is the difference between a Python script and a Python module?
A Python script is a file containing Python code that is executed as a standalone program. In contrast, a Python module is a file containing Python definitions and statements that can be imported and reused in other Python scripts or modules.
How can I handle errors in my Python scripts?
You can handle errors in Python scripts using try-except blocks. Place the code that may raise an error inside the try block, and define the actions to take in case of an error in the except block. This approach allows for graceful error handling without crashing the program.
What libraries should I consider for enhancing my Python scripts?
Consider using libraries such as NumPy for numerical computations, Pandas for data manipulation, Matplotlib for data visualization, and Requests for making HTTP requests. These libraries can significantly enhance the functionality of your Python scripts.
How can I make my Python scripts more efficient?
To make your Python scripts more efficient, focus on optimizing algorithms, minimizing the use of global variables, utilizing built-in functions, and employing list comprehensions. Additionally, consider profiling your code to identify bottlenecks and refactoring as necessary.
In summary, writing Python scripts involves understanding the fundamental principles of programming, leveraging the syntax and structure unique to Python, and applying best practices for code organization and readability. Beginners should start with basic concepts such as variables, data types, loops, and functions, gradually progressing to more complex topics like object-oriented programming and libraries. This foundational knowledge is crucial for creating effective and efficient scripts.
Furthermore, utilizing Python’s extensive libraries and frameworks can significantly enhance the capabilities of your scripts. Libraries such as NumPy for numerical computations, pandas for data manipulation, and Flask for web applications can streamline development processes and expand the functionality of your projects. Familiarity with these tools can save time and effort, allowing developers to focus on solving specific problems rather than reinventing the wheel.
Additionally, adopting best practices such as writing clear and concise code, using comments effectively, and adhering to the PEP 8 style guide can improve the maintainability and readability of scripts. Version control systems, like Git, also play a vital role in managing changes and collaborating on projects. By integrating these practices into your workflow, you can enhance both the quality of your scripts and your overall programming proficiency.
Author Profile
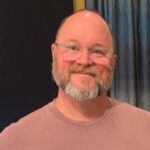
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?