How Can You Write a Python Program from Scratch?
How To Write A Python Program
In the ever-evolving landscape of technology, Python has emerged as a powerhouse programming language, celebrated for its simplicity and versatility. Whether you’re a novice stepping into the world of coding or an experienced developer looking to expand your skill set, understanding how to write a Python program is an essential milestone on your journey. This article will guide you through the fundamental concepts and practical steps necessary to harness the full potential of Python, empowering you to create everything from simple scripts to complex applications.
Writing a Python program begins with grasping the core principles of the language, including its syntax, data types, and control structures. Python’s readability and straightforward syntax make it an ideal choice for beginners, while its robust libraries and frameworks offer seasoned developers the tools needed for advanced projects. This article will explore the foundational elements that contribute to effective programming in Python, ensuring that you not only learn how to write code but also understand the logic behind it.
As we delve deeper, you will discover the importance of planning your program, structuring your code effectively, and debugging common errors. With practical examples and tips, we aim to equip you with the knowledge and confidence to tackle your own Python projects. Whether your goal is to automate tasks, analyze data, or develop web applications
Understanding Variables and Data Types
In Python, variables are used to store data values. A variable is created the moment you assign a value to it. Python is dynamically typed, meaning you do not need to declare the variable type explicitly; the interpreter infers it from the assigned value.
Common data types in Python include:
- Integers: Whole numbers, e.g., `5`, `-3`
- Floats: Decimal numbers, e.g., `3.14`, `-0.001`
- Strings: Sequences of characters, e.g., `”Hello, World!”`
- Booleans: Represents `True` or “
Here is a simple example of variable assignment:
“`python
age = 30
height = 5.9
name = “Alice”
is_student = True
“`
You can check the type of a variable using the `type()` function:
“`python
print(type(age)) Output:
“`
Control Flow Statements
Control flow statements allow you to dictate the order in which code executes. The most common control flow statements are `if`, `elif`, and `else`.
The syntax for these statements is straightforward:
“`python
if condition:
Execute block of code
elif another_condition:
Execute another block of code
else:
Execute this block if all conditions above are
“`
Here’s an example:
“`python
temperature = 25
if temperature > 30:
print(“It’s hot outside.”)
elif temperature < 10:
print("It's cold outside.")
else:
print("The weather is moderate.")
```
Additionally, loops such as `for` and `while` enable repeated execution of code blocks.
- For Loop: Iterates over a sequence (like a list or string)
- While Loop: Continues as long as a condition is true
Example of a `for` loop:
“`python
for i in range(5):
print(i)
“`
Functions in Python
Functions are a block of reusable code that performs a specific task. They help in organizing and modularizing code. You define a function using the `def` keyword followed by the function name and parentheses.
Here is the syntax:
“`python
def function_name(parameters):
Function body
return value
“`
Example of a simple function:
“`python
def greet(name):
return f”Hello, {name}!”
message = greet(“Bob”)
print(message) Output: Hello, Bob!
“`
Functions can also take multiple parameters:
“`python
def add(a, b):
return a + b
“`
The use of default parameters can simplify function calls:
“`python
def multiply(a, b=1):
return a * b
“`
Data Structures: Lists, Tuples, and Dictionaries
Python provides several built-in data structures to handle collections of data. The most commonly used are lists, tuples, and dictionaries.
Data Structure | Definition | Mutability |
---|---|---|
List | An ordered collection of items | Mutable |
Tuple | An ordered collection of items | Immutable |
Dictionary | A collection of key-value pairs | Mutable |
Lists are defined using square brackets:
“`python
fruits = [“apple”, “banana”, “cherry”]
“`
Tuples are defined using parentheses:
“`python
coordinates = (10.0, 20.0)
“`
Dictionaries are defined using curly braces:
“`python
student = {“name”: “John”, “age”: 21}
“`
Accessing elements in these data structures is straightforward, and they offer various methods for manipulation. For instance, you can append to a list or update a dictionary key.
Understanding these fundamental components is crucial for writing effective Python programs.
Understanding the Basics of Python Syntax
Python’s syntax is designed to be readable and straightforward. It uses indentation to define the structure of the code, which enhances its clarity. Key elements of Python syntax include:
- Variables: Used to store data values.
- Data Types: Includes integers, floats, strings, and booleans.
- Operators: Arithmetic, comparison, and logical operators allow manipulation of data.
Example of basic variable assignment and data types:
“`python
age = 30 Integer
height = 5.9 Float
name = “Alice” String
is_student = True Boolean
“`
Writing Your First Python Program
To create a simple Python program, follow these steps:
- Choose an IDE or Text Editor: Options include PyCharm, VSCode, or even simple editors like Notepad.
- Create a New File: Save it with a `.py` extension, for example, `hello.py`.
- Write the Code: Begin with a simple print statement.
“`python
print(“Hello, World!”)
“`
- Run the Program: Use the command line or the IDE’s run feature to execute the file.
Using Control Structures
Control structures dictate the flow of your program. The main types include:
– **Conditional Statements**: `if`, `elif`, and `else` allow branching logic.
– **Loops**: `for` and `while` loops enable repeated execution of code blocks.
Example of a conditional statement:
“`python
if age >= 18:
print(“You are an adult.”)
else:
print(“You are a minor.”)
“`
Example of a loop:
“`python
for i in range(5):
print(i)
“`
Defining Functions
Functions encapsulate reusable code. They improve modularity and maintainability. Define a function using the `def` keyword followed by the function name and parameters.
Example:
“`python
def greet(name):
print(f”Hello, {name}!”)
“`
To call the function:
“`python
greet(“Alice”)
“`
Working with Libraries and Modules
Python has a vast ecosystem of libraries and modules that extend its capabilities. Use the `import` statement to include them in your programs. Commonly used libraries include:
Library | Purpose |
---|---|
NumPy | Numerical computations |
Pandas | Data manipulation and analysis |
Matplotlib | Data visualization |
Example of importing a library:
“`python
import math
print(math.sqrt(16)) Outputs: 4.0
“`
Debugging and Error Handling
Writing robust code requires effective debugging and error handling. Python uses exceptions to manage errors. Use `try` and `except` blocks to catch exceptions.
Example of error handling:
“`python
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero.”)
“`
Utilize debugging tools available in your IDE to step through your code and identify issues.
Best Practices for Writing Python Code
Adhering to best practices enhances code quality and maintainability. Consider the following:
- Follow PEP 8 Guidelines: Ensure consistent coding style.
- Use Meaningful Variable Names: Improve code readability.
- Comment Your Code: Explain complex logic and functions.
- Write Unit Tests: Validate code functionality through testing.
Example of a comment:
“`python
This function returns the square of a number
def square(num):
return num ** 2
“`
By applying these practices, you contribute to cleaner, more efficient code development in Python.
Expert Guidance on Writing Python Programs
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When writing a Python program, it is crucial to start with a clear understanding of the problem you are trying to solve. This involves breaking down the requirements and designing a structured approach before diving into the code.”
James Liu (Python Developer Advocate, CodeCraft). “Utilizing Python’s extensive libraries can significantly enhance the efficiency of your program. Familiarizing yourself with libraries such as NumPy, Pandas, and Flask can save time and improve functionality.”
Sarah Thompson (Lead Instructor, Python Academy). “Writing clean and maintainable code should be a priority for any Python programmer. Adhering to the PEP 8 style guide and incorporating comments will make your code more readable for others and for your future self.”
Frequently Asked Questions (FAQs)
How do I start writing a Python program?
To start writing a Python program, first ensure that Python is installed on your system. Use a text editor or an Integrated Development Environment (IDE) like PyCharm or VSCode to create a new file with a `.py` extension. Write your code in this file and execute it using the command line or the run feature in your IDE.
What are the basic components of a Python program?
The basic components of a Python program include variables, data types, operators, control flow statements (like if-else and loops), functions, and modules. Each of these elements plays a crucial role in structuring and executing a program effectively.
How can I handle errors in my Python program?
You can handle errors in your Python program using try-except blocks. This allows you to catch exceptions and manage them gracefully, preventing your program from crashing. Additionally, you can use finally blocks for cleanup actions that should occur regardless of whether an error occurred.
What libraries should I consider using in Python?
Popular libraries to consider include NumPy for numerical computations, Pandas for data manipulation, Matplotlib for data visualization, and Flask or Django for web development. The choice of library depends on the specific requirements of your project.
How do I debug a Python program?
To debug a Python program, you can use built-in tools like the Python debugger (pdb) or IDE features that allow step-by-step execution. Print statements can also help track variable values and program flow. Additionally, using logging can provide insights into the program’s behavior during execution.
What resources are available for learning Python programming?
Numerous resources are available for learning Python programming, including online courses (such as Coursera and Udemy), official Python documentation, books like “Automate the Boring Stuff with Python,” and community forums such as Stack Overflow and Reddit. These resources cater to various learning styles and levels.
In summary, writing a Python program involves several key steps that guide the developer from concept to execution. Initially, it is essential to define the problem clearly and outline the requirements of the program. This foundational understanding sets the stage for effective coding and helps in identifying the necessary libraries and tools that may be required during the development process.
Once the problem is defined, the next step is to design the program’s architecture. This includes creating algorithms and flowcharts that map out the logic and structure of the code. By visualizing the program’s flow, developers can identify potential challenges and optimize their approach before diving into actual coding. This planning phase is crucial for ensuring that the program is efficient and meets the outlined requirements.
After the design phase, the actual coding begins. Utilizing Python’s syntax and built-in functions, developers can implement the program step by step. It is important to write clean, readable code and to comment on complex sections for future reference. Testing and debugging are also integral parts of this process, as they help identify and resolve issues that may arise during execution.
writing a Python program is a structured process that requires careful planning, clear coding, and thorough testing. By following these steps, developers can
Author Profile
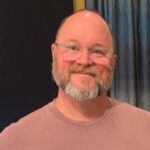
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?