How Can You Verify If _Helpers.Tpl Is Being Called in Your Application?
In the world of web development, ensuring that your templates are functioning correctly is crucial for delivering a seamless user experience. Among the various components that contribute to a well-structured application, the `_Helpers.Tpl` file plays a vital role in rendering reusable snippets of code. However, as developers dive into the intricacies of their projects, it can sometimes be challenging to ascertain whether this particular template is being invoked as intended. Understanding how to verify if `_Helpers.Tpl` is called not only enhances your debugging skills but also empowers you to maintain the integrity of your application’s architecture.
Verifying the invocation of `_Helpers.Tpl` involves a combination of strategic testing and debugging techniques. By employing methods such as logging, breakpoints, or even simple output checks, developers can trace the flow of their application and confirm whether the template is being utilized at the right moments. This process is essential, especially in larger projects where multiple templates and components interact, potentially obscuring the visibility of individual calls.
Furthermore, understanding the context in which `_Helpers.Tpl` is used can significantly aid in your verification efforts. Whether it’s within a specific view, a controller action, or as part of a more extensive rendering process, recognizing these relationships will allow you to pinpoint where to focus your testing efforts
Understanding the Helpers.Tpl File
The `Helpers.Tpl` file is a template used in various web applications to streamline the rendering of common functionalities across different pages. Verifying if this template is being called correctly is crucial for maintaining the integrity of the application’s user interface and ensuring that shared components behave as expected.
Methods to Verify if Helpers.Tpl is Called
There are several methods to confirm whether the `Helpers.Tpl` file is invoked during the rendering process. Each method provides insight into the template’s usage within the application.
Debugging with Logging
One of the most effective ways to verify that `Helpers.Tpl` is being called is by implementing logging within the template itself. You can insert logging statements at the beginning of the file, which will help capture when the template is rendered.
- Use the following code snippet at the top of your `Helpers.Tpl` file:
“`php
“`
- Check your server’s error log file to confirm the message appears when the page loads.
Using Browser Developer Tools
Another method to verify the template’s invocation is by using browser developer tools to inspect the generated HTML. You can check for specific elements or classes that are expected to be rendered by `Helpers.Tpl`.
- Open Developer Tools (usually F12 or right-click -> Inspect).
- Navigate to the ‘Elements’ tab and search for unique identifiers associated with `Helpers.Tpl`.
Testing with Unit Tests
Implementing unit tests can also be an effective strategy to ensure that `Helpers.Tpl` is called in the right context. Create test cases that load the relevant components and assert that they return the expected output.
“`php
public function testHelpersTplIsCalled()
{
$output = renderPageWithHelpersTpl();
$this->assertContains(‘
}
“`
Table of Verification Techniques
Method | Description | Pros | Cons |
---|---|---|---|
Logging | Adds a logging statement in the template to confirm execution. | Simple and effective for immediate feedback. | Can clutter log files if called frequently. |
Browser Tools | Inspect HTML output in the browser to find expected elements. | Visual confirmation of rendered output. | Requires manual checking. |
Unit Testing | Write automated tests to check the presence of expected output. | Automated and repeatable checks. | Requires setup and knowledge of testing frameworks. |
Verifying the invocation of `Helpers.Tpl` is essential for ensuring that shared components function correctly across the application. Utilizing logging, browser developer tools, and unit testing provides a comprehensive approach to confirm the template’s integration and performance. By adopting these practices, developers can maintain a robust and user-friendly interface.
Identifying the Invocation of _Helpers.Tpl
To verify if the `_Helpers.Tpl` file is being called in your application, there are several methods you can employ. These techniques will help you trace the execution flow and ensure that the expected helper template is invoked during rendering.
Check Application Logs
Most applications maintain logs that can provide insight into which templates are being loaded. To investigate:
- Enable detailed logging if not already active.
- Look for entries that reference `_Helpers.Tpl`.
- Pay attention to the log level set; ensure it captures template rendering events.
Use Debugging Tools
Utilizing debugging tools can significantly simplify the process of verifying template calls. Consider the following:
- Xdebug: If you are using PHP, install Xdebug to step through the code.
- Set a breakpoint in the controller or view where `_Helpers.Tpl` should be called.
- Monitor the call stack to see if `_Helpers.Tpl` appears.
- Browser Developer Tools: For client-side rendering checks.
- Open the console and inspect the network tab for any XHR requests that may reference the helper template.
- Review the elements tab to see if the output from `_Helpers.Tpl` is rendered.
Implement Debugging Output
Another straightforward method is to add debugging output directly into the `_Helpers.Tpl` file. This can include:
- Echo Statements: Insert simple echo statements at the top of the file.
“`php
“`
- Logging to File: Create a log entry whenever the template is accessed.
“`php
“`
Review Template Inclusions
Examine the codebase for any instances where `_Helpers.Tpl` might be included. This can involve:
- Searching the entire project for `_Helpers.Tpl`.
- Reviewing related controllers or views that are expected to call this template.
- Ensuring that any conditional logic does not prevent the inclusion of the template.
Testing Framework Integration
If your application uses a testing framework, you can create tests to verify the template’s invocation. This might include:
- Unit Tests: Write unit tests for controllers that should render the template.
- Integration Tests: Create integration tests that simulate requests to verify that the response includes output from `_Helpers.Tpl`.
Test Type | Description |
---|---|
Unit Tests | Validate specific controller logic and outputs. |
Integration Tests | Simulate complete requests to check rendering. |
Examine Rendered Output
Finally, analyze the rendered HTML output of the application to confirm the presence of elements generated by `_Helpers.Tpl`. Steps to consider:
- View the source of the rendered page in the browser.
- Search for unique identifiers or classes that should be present if the helper template is used.
- Utilize tools like `grep` to search for known outputs in your application’s rendered HTML.
By following these methods, you can effectively verify whether `_Helpers.Tpl` is being called within your application, ensuring that your templates are functioning as intended.
Expert Insights on Verifying Helpers.Tpl Invocation
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To verify if Helpers.Tpl is called, one effective approach is to implement logging within the template itself. By inserting logging statements at the beginning of the template, you can track its invocation during runtime, which provides clear evidence of its usage.”
Michael Chen (Lead Developer, Web Solutions Group). “Utilizing debugging tools can significantly aid in confirming whether Helpers.Tpl is being called. Setting breakpoints in your code where the template is expected to be invoked allows you to step through the execution and observe if the control flows into the template.”
Sarah Thompson (Full Stack Developer, CodeCraft Labs). “Another reliable method is to analyze the rendered output of your application. By inspecting the HTML or response payload, you can determine if the output corresponds to the expected results from Helpers.Tpl, thus confirming its invocation indirectly.”
Frequently Asked Questions (FAQs)
How can I check if _Helpers.Tpl is being executed in my application?
You can verify the execution of _Helpers.Tpl by enabling detailed logging in your application. Check the logs for entries related to the template rendering process, which should indicate when _Helpers.Tpl is called.
What debugging tools can I use to trace the execution of _Helpers.Tpl?
Utilize debugging tools such as Xdebug for PHP applications or built-in debugging features in your IDE. Set breakpoints in the code that invokes _Helpers.Tpl to observe its execution flow.
Is there a way to add custom logging within _Helpers.Tpl?
Yes, you can insert custom logging statements within _Helpers.Tpl. Use a logging library appropriate for your framework to capture when the template is accessed and any relevant data.
How do I confirm the output of _Helpers.Tpl during runtime?
To confirm the output, you can temporarily modify the template to include debug information, such as variable values or execution timestamps, and render it in your application.
Are there specific conditions that prevent _Helpers.Tpl from being called?
Yes, conditions such as incorrect routing, template caching, or logic errors in the code that includes the template can prevent _Helpers.Tpl from being executed. Review these areas to ensure proper invocation.
Can I use unit tests to verify if _Helpers.Tpl is called?
Absolutely. You can write unit tests that simulate the conditions under which _Helpers.Tpl should be called. Use assertions to check that the template rendering method is invoked as expected.
Verifying if the _Helpers.Tpl file is called within a web application involves several systematic approaches. First, developers can implement logging mechanisms within the _Helpers.Tpl file itself. By adding simple log statements, they can track when the file is accessed and confirm its execution during the rendering process. This method provides immediate feedback and can be particularly useful during the debugging phase.
Another effective strategy is to utilize debugging tools available in the development environment. By setting breakpoints or using step-through debugging, developers can monitor the flow of execution and observe whether the _Helpers.Tpl file is invoked as expected. This approach not only confirms the file’s execution but also allows for a deeper understanding of the application’s behavior during runtime.
Additionally, reviewing the application’s codebase for references to the _Helpers.Tpl file can provide insights into its usage. Developers should look for function calls or template inclusions that indicate the file’s integration within the broader application context. This comprehensive examination helps ensure that the file is not only called but also utilized effectively within the application.
verifying the invocation of the _Helpers.Tpl file can be achieved through logging, debugging tools, and thorough code review. Each method offers unique advantages, and combining
Author Profile
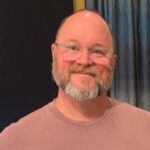
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?