How Can You Effectively Use APIs in Python?
In today’s digital landscape, APIs (Application Programming Interfaces) have become the backbone of modern software development. They enable different applications to communicate with one another, allowing developers to harness the power of existing services and data in innovative ways. Whether you’re building a web application, automating tasks, or integrating third-party services, knowing how to use APIs effectively in Python can elevate your projects to new heights. This article will guide you through the essentials of API usage in Python, empowering you to unlock a world of possibilities.
Using APIs in Python is not only straightforward but also incredibly versatile. With libraries like `requests`, developers can easily send HTTP requests and handle responses, making it simple to interact with various web services. This means you can fetch data from a weather service, post updates to social media, or even retrieve information from a database—all with just a few lines of code. Understanding the fundamental principles of API interaction will provide you with the tools to enhance your applications and streamline processes.
As we delve deeper into the world of APIs, you’ll discover how to authenticate requests, manage data formats like JSON, and handle errors gracefully. Whether you’re a seasoned programmer or just starting your coding journey, mastering API usage in Python will not only expand your skill set but also open doors to countless opportunities in the tech
Understanding API Requests
When working with APIs in Python, it’s crucial to understand the types of requests you can make. The most common HTTP methods used in APIs are GET, POST, PUT, DELETE, and PATCH. Each method serves a specific purpose in the interaction with the API.
- GET: Retrieve data from a specified resource.
- POST: Send data to the API to create a new resource.
- PUT: Update an existing resource with new data.
- DELETE: Remove a specified resource.
- PATCH: Apply partial modifications to a resource.
Using the Requests Library
The `requests` library is a powerful tool for making HTTP requests in Python. It simplifies the process of sending requests and handling responses. To get started, ensure you have the library installed:
“`bash
pip install requests
“`
Here’s how to use the `requests` library for different types of requests:
“`python
import requests
GET request
response = requests.get(‘https://api.example.com/data’)
print(response.json())
POST request
data = {‘key’: ‘value’}
response = requests.post(‘https://api.example.com/data’, json=data)
print(response.json())
“`
Handling Responses
After making a request, it is essential to handle the response effectively. The `response` object contains various attributes and methods for parsing the returned data. The most notable attributes include:
- status_code: Indicates the status of the response (e.g., 200 for success).
- json(): Parses the response body as JSON if applicable.
- text: Returns the raw response body as a string.
Here’s an example of how to check the status code and handle the response:
“`python
response = requests.get(‘https://api.example.com/data’)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f”Error: {response.status_code}”)
“`
Working with JSON Data
APIs often return data in JSON format. Python’s built-in `json` module provides functionality to parse and generate JSON data easily. When you retrieve JSON data from an API, you can convert it into a Python dictionary, making it easier to manipulate.
Here’s a simple example demonstrating how to work with JSON data:
“`python
import json
response = requests.get(‘https://api.example.com/data’)
data = response.json()
Accessing data
for item in data[‘items’]:
print(item[‘name’], item[‘value’])
“`
Table of Common HTTP Status Codes
Understanding HTTP status codes is vital for API interactions. Below is a table of common status codes you may encounter:
Status Code | Description |
---|---|
200 | OK – The request was successful. |
201 | Created – The request was successful and a resource was created. |
204 | No Content – The request was successful, but there is no content to return. |
400 | Bad Request – The request was malformed or invalid. |
401 | Unauthorized – Authentication is required and has failed or has not yet been provided. |
404 | Not Found – The requested resource could not be found. |
500 | Internal Server Error – A generic error occurred on the server. |
By mastering these fundamental concepts and methods, you will be well-equipped to interact with APIs effectively using Python.
Understanding APIs and Their Importance
APIs (Application Programming Interfaces) serve as intermediaries that allow different software applications to communicate with one another. They define the methods and data formats that applications can use to request and exchange information. APIs are crucial in enabling the integration of different services, enhancing functionality, and promoting interoperability among various platforms.
Setting Up Your Environment
To use APIs in Python, ensure you have the necessary environment set up. Follow these steps:
- Install Python: Make sure Python is installed on your machine. You can download it from the official Python website.
- Install Required Libraries: The primary library for making API requests in Python is `requests`. Install it using the following command:
“`bash
pip install requests
“`
Making API Requests
The `requests` library simplifies HTTP requests. Here’s how to make a basic GET request:
“`python
import requests
url = ‘https://api.example.com/data’
response = requests.get(url)
if response.status_code == 200:
data = response.json() Parse JSON response
print(data)
else:
print(f”Error: {response.status_code}”)
“`
This code snippet demonstrates how to:
- Import the library
- Define the API endpoint
- Handle the response and check for errors
Handling Different Request Types
APIs support various request types, including:
- GET: Retrieve data from a server.
- POST: Send data to a server.
- PUT: Update existing data on a server.
- DELETE: Remove data from a server.
Here’s an example of a POST request:
“`python
url = ‘https://api.example.com/data’
payload = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
response = requests.post(url, json=payload)
if response.status_code == 201:
print(“Data created successfully:”, response.json())
else:
print(f”Error: {response.status_code}”)
“`
Working with Headers and Authentication
Many APIs require authentication and custom headers. You can include these in your requests as follows:
“`python
url = ‘https://api.example.com/protected-data’
headers = {
‘Authorization’: ‘Bearer YOUR_ACCESS_TOKEN’,
‘Content-Type’: ‘application/json’
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print(“Protected data:”, response.json())
else:
print(f”Error: {response.status_code}”)
“`
Handling Errors Gracefully
It’s important to handle potential errors gracefully. Use try-except blocks to manage exceptions and respond to different status codes effectively:
“`python
try:
response = requests.get(url)
response.raise_for_status() Raise an error for bad responses
except requests.exceptions.HTTPError as err:
print(f”HTTP error occurred: {err}”)
except Exception as err:
print(f”Other error occurred: {err}”)
else:
print(“Success:”, response.json())
“`
Parsing Response Data
Most APIs return data in JSON format. You can easily parse this data into Python objects using the `json()` method:
“`python
data = response.json()
for item in data[‘items’]:
print(item[‘name’], item[‘value’])
“`
When working with complex data, consider using libraries like `pandas` for better manipulation and analysis:
“`python
import pandas as pd
df = pd.json_normalize(data[‘items’])
print(df.head())
“`
Rate Limiting and Best Practices
When using APIs, adhere to best practices:
- Rate Limiting: Be aware of the API’s rate limits to avoid being blocked. Implement retry logic with exponential backoff.
- Caching: Cache responses when possible to reduce unnecessary API calls.
- Documentation: Always refer to the API documentation for specific endpoints, required parameters, and response formats.
Utilizing these practices will enhance your efficiency and ensure smooth interactions with APIs.
Expert Insights on Utilizing APIs in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When utilizing APIs in Python, it is essential to understand the requests library, as it simplifies the process of making HTTP requests. Properly handling responses and exceptions will ensure robust applications that can gracefully manage errors.”
Michael Chen (Data Scientist, Analytics Hub). “Incorporating APIs into Python projects allows for seamless data integration. I recommend using JSON for data interchange, as it is lightweight and easy to parse with Python’s built-in libraries, enhancing data manipulation capabilities.”
Sarah Patel (Lead Developer, Cloud Solutions Corp.). “Effective API usage in Python requires a solid understanding of authentication methods, such as OAuth. Implementing secure access is crucial for protecting sensitive data and ensuring compliance with industry standards.”
Frequently Asked Questions (FAQs)
How do I install the necessary libraries to use APIs in Python?
To use APIs in Python, you typically need the `requests` library. Install it using pip with the command `pip install requests`. This library simplifies making HTTP requests.
What is the basic syntax for making a GET request in Python?
The basic syntax for a GET request using the `requests` library is as follows:
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
“`
This retrieves data from the specified API endpoint.
How can I send data to an API using Python?
To send data to an API, use a POST request. The syntax is:
“`python
import requests
response = requests.post(‘https://api.example.com/data’, json={‘key’: ‘value’})
“`
This sends JSON data to the specified endpoint.
How do I handle API responses in Python?
You can handle API responses by checking the status code and accessing the response content. For example:
“`python
if response.status_code == 200:
data = response.json()
else:
print(‘Error:’, response.status_code)
“`
This checks if the request was successful and retrieves the JSON data.
What should I do if I encounter an error while using an API?
If you encounter an error, first check the status code of the response. Common codes include 400 for bad requests and 404 for not found. Review the API documentation for specific error handling guidelines.
How can I authenticate with an API in Python?
Authentication methods vary by API. Common methods include API keys, OAuth tokens, or Basic Auth. For example, to use an API key, include it in the headers:
“`python
headers = {‘Authorization’: ‘Bearer YOUR_API_KEY’}
response = requests.get(‘https://api.example.com/data’, headers=headers)
“`
This sends the API key for authentication.
In summary, using APIs in Python is a powerful way to interact with external services and access data programmatically. The process typically involves understanding the API’s documentation, which provides crucial information about endpoints, request methods, and required parameters. By utilizing libraries such as `requests`, developers can easily send HTTP requests to the API and handle responses efficiently. This streamlined approach allows for seamless integration of various functionalities into Python applications.
Key takeaways include the importance of authentication when accessing APIs, as many require API keys or tokens to ensure secure communication. Additionally, handling errors gracefully is vital to maintain robust applications, as APIs may return different status codes based on the success or failure of requests. By implementing error handling and response validation, developers can create more resilient applications that can adapt to changes in API behavior.
Furthermore, understanding the data formats commonly used in APIs, such as JSON or XML, is essential for effective data manipulation. Python’s built-in libraries simplify the process of parsing and converting these formats into usable data structures. Overall, mastering API usage in Python opens up numerous opportunities for developers to enhance their projects with external data and services, making it a valuable skill in today’s programming landscape.
Author Profile
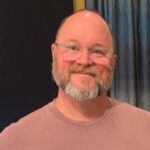
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?