How Can You Update a GitHub File Using Golang?
In the fast-paced world of software development, version control systems like GitHub have become indispensable tools for collaboration and code management. As developers increasingly turn to automation to streamline their workflows, the ability to programmatically update files on GitHub can save time and reduce human error. For those familiar with Go, a powerful and efficient programming language, leveraging its capabilities to interact with GitHub’s API opens up a world of possibilities. Whether you’re looking to automate routine updates, manage documentation, or integrate GitHub actions into your applications, understanding how to update a GitHub file using Golang is a skill worth mastering.
This article will guide you through the essentials of updating files on GitHub using Go. We’ll explore the GitHub API, which provides a robust interface for interacting with repositories, and highlight how Go’s simplicity and performance make it an ideal choice for this task. You’ll learn about the necessary authentication steps, how to construct API requests, and the best practices for error handling—all crucial elements for ensuring your updates are seamless and efficient.
By the end of this article, you’ll have a solid understanding of how to programmatically update files on GitHub using Go, empowering you to enhance your projects and automate your development processes. Whether you’re a seasoned Go developer
Setting Up the GitHub API Client
To update a file on GitHub using Go, the first step is to set up a GitHub API client. This client will allow you to interact with the GitHub repository and perform operations such as fetching the current file content, updating it, and committing changes. You can use the `go-github` package, which is a widely used library for working with the GitHub API in Go.
“`go
import (
“context”
“github.com/google/go-github/v47/github”
“golang.org/x/oauth2”
)
func createClient(token string) *github.Client {
ctx := context.Background()
ts := oauth2.StaticTokenSource(
&oauth2.Token{AccessToken: token},
)
tc := oauth2.NewClient(ctx, ts)
return github.NewClient(tc)
}
“`
Make sure to replace `token` with a GitHub personal access token that has the necessary permissions for repository access.
Retrieving the Current File Content
Before updating a file, it’s essential to retrieve its current content and SHA (the unique identifier for the file version). The SHA is necessary because GitHub requires it to ensure that you are updating the correct version of the file.
Here’s how to fetch the file content:
“`go
func getFileContent(client *github.Client, owner, repo, filePath string) (string, string, error) {
file, _, _, err := client.Repositories.GetContents(context.Background(), owner, repo, filePath, nil)
if err != nil {
return “”, “”, err
}
content, err := file.GetContent()
if err != nil {
return “”, “”, err
}
return content, file.SHA, nil
}
“`
This function returns the file content and its SHA, which you will use for the update.
Updating the File
Once you have the file content and SHA, you can proceed to update the file. The update requires the new content, the SHA of the existing file, and a commit message.
Here’s how to perform the update:
“`go
func updateFile(client *github.Client, owner, repo, filePath, newContent, sha, commitMessage string) error {
options := &github.RepositoryContentFileOptions{
Message: &commitMessage,
Content: []byte(newContent),
SHA: &sha,
}
_, _, err := client.Repositories.UpdateFile(context.Background(), owner, repo, filePath, options)
return err
}
“`
You can call this function after retrieving the current file content and SHA, passing in the new content you wish to save.
Example Usage
Here’s a complete example of how you can combine the above functions to update a file in a GitHub repository:
“`go
func main() {
token := “your_personal_access_token”
owner := “repository_owner”
repo := “repository_name”
filePath := “path/to/your/file.txt”
newContent := “This is the updated content.”
commitMessage := “Update file content”
client := createClient(token)
currentContent, sha, err := getFileContent(client, owner, repo, filePath)
if err != nil {
panic(err)
}
err = updateFile(client, owner, repo, filePath, newContent, sha, commitMessage)
if err != nil {
panic(err)
}
}
“`
Handling Errors
When working with the GitHub API, it is crucial to handle errors gracefully. Common errors include authentication issues, file not found, or permission errors. Always check for errors after API calls and implement appropriate error handling logic.
Error Type | Possible Causes | Suggested Actions |
---|---|---|
Authentication Error | Invalid token or insufficient permissions | Verify token and permissions |
File Not Found | Incorrect file path or repository | Check the file path and repository settings |
Permission Denied | Token lacks write permissions | Update token permissions to include write access |
Prerequisites
To update a file on GitHub using Go, ensure you have the following:
- A GitHub account with repository access
- Go installed on your machine
- GitHub Personal Access Token (PAT) with required scopes (repo, workflow)
- Go modules initialized in your project
Setting Up Your Go Environment
Install the necessary packages to interact with the GitHub API. Use the following command to install the `go-github` library:
“`bash
go get github.com/google/go-github/v37/github
“`
Additionally, ensure you have the `oauth2` package for authentication:
“`bash
go get golang.org/x/oauth2
“`
Authenticating with GitHub
Create a function to authenticate using your Personal Access Token. Here’s an example:
“`go
package main
import (
“context”
“golang.org/x/oauth2”
“github.com/google/go-github/v37/github”
)
func createGitHubClient(token string) *github.Client {
ctx := context.Background()
ts := oauth2.StaticTokenSource(&oauth2.Token{AccessToken: token})
tc := oauth2.NewClient(ctx, ts)
return github.NewClient(tc)
}
“`
Updating a File in a GitHub Repository
To update a file, you need to retrieve the current file contents and then create a new commit. The following steps outline this process:
- Get the current file content:
Retrieve the current content of the file you wish to update.
- Modify the content:
Make the necessary changes to the file content.
- Create a new commit:
Push the updated content back to the repository.
Here is the complete example code:
“`go
package main
import (
“context”
“fmt”
“github.com/google/go-github/v37/github”
“golang.org/x/oauth2”
“log”
)
func main() {
token := “YOUR_PERSONAL_ACCESS_TOKEN”
owner := “YOUR_GITHUB_USERNAME”
repo := “YOUR_REPOSITORY_NAME”
path := “path/to/your/file.txt”
newContent := []byte(“New content to be written”)
client := createGitHubClient(token)
// Get the file’s current content
file, _, _, err := client.Repositories.GetContents(context.Background(), owner, repo, path, nil)
if err != nil {
log.Fatalf(“Error getting file content: %v”, err)
}
// Update the file
options := &github.RepositoryContentFileOptions{
Message: github.String(“Updating file content”),
Content: newContent,
SHA: github.String(*file.SHA),
}
_, _, err = client.Repositories.UpdateFile(context.Background(), owner, repo, path, options)
if err != nil {
log.Fatalf(“Error updating file: %v”, err)
}
fmt.Println(“File updated successfully.”)
}
“`
Handling Errors
Error handling is crucial when interacting with external APIs. Ensure to check for errors at each step. Possible errors include:
- Unauthorized access (Invalid token)
- Not found (Incorrect repository or file path)
- Conflicts (File was modified since retrieval)
Implement appropriate error messages to assist in debugging.
Testing Your Implementation
To test the implementation:
- Ensure your Go environment is set up properly.
- Run the program and verify the file is updated in the specified GitHub repository.
- Check the commit history to confirm the update message reflects your changes.
Expert Insights on Updating GitHub Files with Golang
Jessica Tran (Senior Software Engineer, Open Source Initiative). “Updating a GitHub file using Golang can be streamlined by utilizing the GitHub API. It is essential to authenticate properly and handle the JSON payload effectively to ensure that changes are committed accurately.”
Michael Chen (DevOps Specialist, Tech Innovations). “When working with Golang to update files on GitHub, leveraging libraries such as ‘go-github’ can significantly simplify the process. This library abstracts much of the complexity involved in making API calls and managing responses.”
Linda Patel (Cloud Solutions Architect, Digital Solutions Group). “It is crucial to implement error handling and logging when updating GitHub files with Golang. This practice not only aids in debugging but also ensures that any issues during the update process are captured and addressed promptly.”
Frequently Asked Questions (FAQs)
How can I authenticate with GitHub using Golang?
To authenticate with GitHub in Golang, you can use a personal access token. Create a token in your GitHub account settings, then use it in your HTTP requests by including it in the `Authorization` header as `Bearer
What libraries are recommended for making HTTP requests in Golang?
The standard library `net/http` is commonly used for making HTTP requests in Golang. Additionally, libraries like `go-git` and `github.com/google/go-github` provide higher-level abstractions for interacting with GitHub’s API.
How do I update a file in a GitHub repository using Golang?
To update a file, retrieve the file’s current content and SHA using the GitHub API, modify the content as needed, and then use the `PUT` method to create a new commit with the updated file, specifying the new content and the previous SHA.
What is the GitHub API endpoint for updating a file?
The endpoint for updating a file is `PUT /repos/{owner}/{repo}/contents/{path}`. This endpoint requires the repository owner, repository name, and the path of the file you wish to update.
Are there any rate limits for using the GitHub API?
Yes, GitHub imposes rate limits on API requests. For authenticated requests, the limit is typically 5,000 requests per hour per user. Unauthenticated requests are limited to 60 requests per hour.
How can I handle errors when updating a file in GitHub using Golang?
To handle errors, check the HTTP response status code after making the request. If the code indicates an error (e.g., 404 for not found, 403 for forbidden), log the error message and take appropriate action, such as retrying or alerting the user.
Updating a GitHub file using Golang involves several steps that integrate the GitHub API with Go’s HTTP capabilities. First, it is essential to authenticate with the GitHub API, typically using a personal access token. This token allows the application to perform actions on behalf of the user, ensuring secure and authorized access to the repository.
Once authentication is established, the next step is to retrieve the file that needs to be updated. This can be done by making a GET request to the appropriate endpoint of the GitHub API, which provides the file’s current content and its SHA. The SHA is crucial because it serves as a unique identifier for the file’s version, which GitHub requires when submitting updates to prevent conflicts.
After obtaining the file’s content and SHA, the final step is to send a PUT request to the GitHub API with the modified content. This request must include the new content, the SHA of the existing file, and a commit message. Upon successful execution, the file will be updated in the repository, reflecting the changes made through the Go application.
In summary, updating a GitHub file using Golang is a straightforward process that requires proper authentication, retrieval of the file’s current state, and
Author Profile
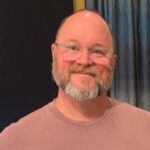
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?