How Can You Effectively Undo a Git Pull?
In the fast-paced world of software development, version control systems like Git are indispensable tools that empower teams to collaborate seamlessly on projects. However, even the most experienced developers can find themselves in a bind when an unexpected change arises after executing a `git pull`. Whether it’s a merge conflict, unwanted changes, or simply a mistake, knowing how to effectively undo a Git pull can save you time and frustration. In this article, we’ll explore the various methods to revert your repository to a previous state, ensuring that you can maintain control over your codebase and keep your projects on track.
When you perform a `git pull`, you’re not just fetching updates from a remote repository; you’re also merging those changes into your local branch. This process can sometimes lead to complications, especially if the incoming changes conflict with your local modifications. Understanding how to undo a Git pull is crucial for maintaining the integrity of your work and avoiding the pitfalls of unintended merges. Fortunately, Git offers several tools and commands that can help you navigate these challenges with ease.
In the following sections, we will delve into the various scenarios that might necessitate undoing a pull, from simple rollbacks to more complex resolutions. Whether you’re dealing with a straightforward mistake or a more intricate situation,
Understanding the Git Pull Command
When you execute the `git pull` command, it effectively combines two actions: fetching changes from the remote repository and merging them into your local branch. This operation can sometimes lead to unintended consequences, especially if there are conflicts or if the pulled changes are not desired. Understanding how to revert these changes is crucial for maintaining a clean project history.
Identifying the Changes Made by Git Pull
Before undoing a `git pull`, it’s essential to review what changes were introduced. You can do this using the `git log` command to inspect the commit history.
- To view recent commits, use:
“`
git log –oneline
“`
- This command will provide a concise view of recent commits, allowing you to identify which commit was added by the pull.
Undoing a Git Pull with Git Reset
If you decide that the changes brought in by the `git pull` are not suitable, you can use `git reset` to revert to the previous state. This method is effective if you have not yet pushed the changes to the remote repository.
- To reset your branch to the state it was in before the last pull, execute:
“`
git reset –hard HEAD~1
“`
- This command will remove the last commit entirely.
Important Notes:
- Using `–hard` will discard all local changes. Use it with caution.
- If you only want to keep the changes but not the commit itself, consider using `–soft`.
Using Git Revert for a Safer Alternative
If you have already pushed the changes to the remote repository and cannot use `git reset`, consider using `git revert`. This command creates a new commit that undoes the changes made by the problematic commit.
- To revert the last commit, run:
“`
git revert HEAD
“`
- This will prompt you to write a commit message for the revert, which is a good practice to maintain clarity in your commit history.
Command | Description |
---|---|
git reset –hard HEAD~1 | Removes the last commit and all changes (not pushed). |
git revert HEAD | Creates a new commit that undoes the last commit (safe for shared branches). |
Handling Merge Conflicts Post-Pull
If your `git pull` resulted in merge conflicts, you will need to resolve these before you can proceed with any resets or reverts. Here’s how to address conflicts:
- Identify conflicting files using:
“`
git status
“`
- Open each conflicting file and look for conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`).
- Resolve the conflicts manually, then stage the resolved files:
“`
git add
“`
- Finally, complete the merge:
“`
git commit
“`
By understanding these methods, you can effectively manage your repository and maintain a clean history, even after unintended pulls.
Understanding Git Pull
A `git pull` command is essentially a combination of two commands: `git fetch` followed by `git merge`. When you execute a pull, the latest changes from a remote repository are fetched and merged into your current branch. If you need to undo this operation, the method you choose depends on whether you want to discard the changes or revert to a previous commit.
Undoing a Git Pull with Reset
If you want to completely remove the changes introduced by the `git pull`, you can use `git reset`. This is particularly useful when you want to discard the changes entirely.
- Soft Reset: If you want to keep your changes in the working directory but reset the commit history.
“`bash
git reset –soft HEAD~1
“`
- Mixed Reset: This will keep your changes in the working directory but unstage them.
“`bash
git reset HEAD~1
“`
- Hard Reset: This will discard all changes and reset your branch to the previous commit.
“`bash
git reset –hard HEAD~1
“`
Reset Type | Effect on Commit History | Effect on Working Directory |
---|---|---|
Soft | Moves HEAD back | Keeps changes |
Mixed | Moves HEAD back | Keeps changes unstaged |
Hard | Moves HEAD back | Discards all changes |
Undoing a Git Pull with Revert
If you want to undo the changes introduced by the `git pull` while maintaining a clean history, the `git revert` command is appropriate. This command creates a new commit that undoes the changes made by the previous commit.
To revert the last commit created by the pull:
“`bash
git revert HEAD
“`
This approach is beneficial when collaborating with others, as it preserves the history while effectively negating the changes made.
Undoing a Git Pull with Checkout
In cases where you want to restore specific files to their state before the pull, you can use the `git checkout` command.
- Identify the commit before the pull using:
“`bash
git log
“`
- Checkout the specific files:
“`bash
git checkout
“`
This command allows you to selectively revert changes for specific files without affecting the entire commit history.
Common Scenarios and Solutions
Scenario | Recommended Action |
---|---|
Discard all changes from pull | Use `git reset –hard HEAD~1` |
Keep changes but unstage them | Use `git reset HEAD~1` |
Create a new commit to negate pull | Use `git revert HEAD` |
Restore specific files | Use `git checkout |
Best Practices
- Always ensure you have backups of important changes before performing resets or hard operations.
- Communicate with your team if working collaboratively to avoid conflicts and confusion.
- Familiarize yourself with the `git log` command to identify commits accurately before reverting or resetting.
Expert Insights on Reverting a Git Pull
Emily Chen (Senior Software Engineer, CodeCraft Solutions). “When you need to undo a Git pull, the most straightforward method is to use the command `git reset –hard HEAD~1`. This will reset your branch to the state it was in before the last pull, effectively discarding any changes that were brought in.”
Michael Thompson (DevOps Consultant, Agile Innovations). “If you have already pushed the changes after the pull, consider using `git revert` to create a new commit that undoes the changes introduced by the pull. This is a safer approach as it maintains the commit history.”
Sarah Patel (Git Version Control Specialist, TechWise). “In scenarios where you are unsure about the changes made by the pull, utilizing `git reflog` can be incredibly useful. It allows you to view the history of your actions and revert to a specific commit before the pull was executed.”
Frequently Asked Questions (FAQs)
How can I undo a Git pull if I haven’t made any commits after it?
You can undo a Git pull by using the command `git reset –hard HEAD@{1}`. This command resets your branch to the state it was in before the last pull.
What should I do if I have made commits after the Git pull?
If you have made commits after the pull, you can use `git reset –hard HEAD~n`, where `n` is the number of commits you want to undo. Be cautious, as this will permanently delete those commits.
Is there a way to revert a Git pull without losing changes?
Yes, you can use `git revert` to create new commits that undo the changes introduced by the pull. This method preserves your commit history while effectively undoing the changes.
How can I see the changes made by the last Git pull?
You can view the changes made by the last pull using `git log -p HEAD@{1}`. This command shows the commit history along with the differences introduced by the pull.
What if I want to keep the changes from the pull but revert to a previous state?
You can create a new branch from the previous state using `git checkout -b new-branch-name HEAD@{1}`. This allows you to keep the changes from the pull while working on a separate branch.
Can I use Git stash before undoing a pull?
Yes, you can use `git stash` to save your current changes before undoing the pull. After stashing, you can safely reset or revert the pull and then apply your stashed changes with `git stash pop`.
In summary, undoing a Git pull is a crucial skill for developers who may need to revert changes after integrating updates from a remote repository. The process can vary depending on whether the pull resulted in a fast-forward merge or a merge commit. Understanding the difference is essential for selecting the appropriate method to revert changes effectively.
Key methods to undo a Git pull include using the `git reset` command to revert to a previous commit or utilizing `git reflog` to identify and restore the state of the repository before the pull. For those who prefer a more cautious approach, creating a new branch before performing a pull can provide a safety net, allowing for easy recovery if needed.
Furthermore, it is important to note that communication with team members is vital when undoing a pull, especially in collaborative environments. Ensuring that everyone is informed about the changes helps maintain project integrity and avoids potential conflicts. By mastering these techniques, developers can navigate Git more confidently and maintain control over their codebase.
Author Profile
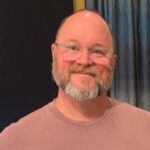
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?