How Can You Effectively Uncomment Code in Python?
How To Uncomment In Python: A Guide for Beginners
In the world of programming, the ability to manipulate code efficiently is crucial, and one of the simplest yet most essential skills is knowing how to uncomment lines in Python. Whether you’re a novice coder just embarking on your programming journey or a seasoned developer revisiting your work, understanding how to manage comments can significantly enhance your coding experience. Comments serve as valuable notes and explanations within your code, but there are times when you may need to bring certain lines back into action. This article will guide you through the process of uncommenting in Python, ensuring you can easily toggle between active code and annotations.
Uncommenting in Python is a straightforward task, yet it plays a vital role in code readability and functionality. Comments are typically used to explain code segments, making it easier for others (or yourself) to understand the logic behind your work. However, during the debugging process or when revising your code, you might find that some previously commented-out lines need to be reactivated. Knowing how to efficiently uncomment these lines can streamline your workflow and enhance your productivity.
In this article, we will explore the various methods for uncommenting in Python, from single-line to multi-line comments. We’ll also discuss best practices for using comments effectively, ensuring
Uncommenting Single Line Comments
In Python, comments are created using the “ symbol. To uncomment a single line comment, simply remove the “ symbol at the beginning of the line. This will make the line executable code. For example:
“`python
print(“This line is commented out.”)
“`
To uncomment it, modify it to:
“`python
print(“This line is now active.”)
“`
This straightforward action allows you to easily toggle between commented and active lines of code.
Uncommenting Multiple Lines
When dealing with multiple lines of comments, the process can vary depending on the development environment (IDE) or text editor you are using. In many IDEs, you can uncomment multiple lines at once, which can significantly enhance your coding efficiency.
- In Visual Studio Code:
- Select the lines you wish to uncomment.
- Press `Ctrl` + `/` (Windows/Linux) or `Cmd` + `/` (Mac). This will toggle the comment status of the selected lines.
- In PyCharm:
- Select the commented lines.
- Use `Ctrl` + `/` (Windows/Linux) or `Cmd` + `/` (Mac) for toggling the comment.
For editors without this functionality, you will need to manually remove the “ from each line.
Using Multi-line Strings for Commenting
Python also supports multi-line strings, which can sometimes be used as comments by enclosing text within triple quotes (`”’` or `”””`). However, these are technically not comments; they are string literals that are not assigned to a variable. To uncomment a block that uses multi-line strings, remove the triple quotes surrounding the text.
Example:
“`python
”’
print(“This is a multi-line comment.”)
print(“It will not execute.”)
”’
“`
To uncomment, you would change it to:
“`python
print(“This line is now active.”)
print(“So is this one.”)
“`
Comparison of Commenting Methods
Here is a summary table comparing the different commenting methods in Python:
Method | Syntax | Use Case |
---|---|---|
Single Line Comment | Quick comments on individual lines | |
Multi-Line Comment (using ) | (each line) | Commenting multiple lines |
Multi-Line String | ”’ or “”” | Block comments (not recommended for actual comments) |
Choosing the appropriate method for commenting and uncommenting in Python depends on your specific needs and the context of your code. Understanding these methods can streamline your coding process and improve code readability.
Understanding Commenting in Python
In Python, comments are a crucial aspect of writing clear and maintainable code. They allow developers to annotate their code, providing explanations or notes that are not executed during runtime. Comments can be single-line or multi-line.
- Single-line comments are initiated with the “ symbol. Everything following this symbol on the same line will be ignored by the Python interpreter.
Example:
“`python
This is a single-line comment
print(“Hello, World!”) This comment is after a statement
“`
- Multi-line comments can be created using triple quotes (`”’` or `”””`). While technically this is a string literal, it is often used for commenting out blocks of code.
Example:
“`python
”’
This is a multi-line comment.
It spans several lines.
”’
print(“Hello, World!”)
“`
How to Uncomment Code in Python
Uncommenting code in Python is a straightforward process, allowing developers to quickly enable or disable sections of their code during development and debugging. Here are the methods to uncomment code:
- Single-line comments:
- To uncomment a single line that has been commented out, simply remove the “ symbol at the beginning of the line.
Example:
“`python
print(“This line is commented out”)
print(“This line is active”)
“`
Uncommenting:
“`python
print(“This line is commented out”)
print(“This line is active”)
“`
- Multi-line comments:
- To uncomment a block of code that has been wrapped in triple quotes, you can remove the triple quotes at the start and end of the block.
Example:
“`python
”’
print(“This is a multi-line comment”)
print(“This line is also commented out”)
”’
print(“This line is active”)
“`
Uncommenting:
“`python
print(“This is a multi-line comment”)
print(“This line is also commented out”)
print(“This line is active”)
“`
Using IDE Features for Uncommenting
Most Integrated Development Environments (IDEs) and code editors provide built-in functionality to uncomment code quickly. Here are some common tools and their methods:
IDE/Editor | Method to Uncomment |
---|---|
PyCharm | Select the commented lines, then use `Ctrl + /` (Windows/Linux) or `Cmd + /` (Mac). |
Visual Studio Code | Highlight the lines, then use `Ctrl + /` (Windows/Linux) or `Cmd + /` (Mac). |
Jupyter Notebook | Highlight the cells and press `Ctrl + /` to toggle comments. |
Atom | Select the code and press `Ctrl + /` or `Cmd + /` to uncomment. |
By utilizing these features, developers can efficiently manage comments and uncommenting without manual editing.
Expert Insights on How to Uncomment in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “Uncommenting in Python is a straightforward process that enhances code readability. By simply removing the ” symbol at the beginning of a line, developers can reactivate previously commented code, which is essential for debugging and iterative development.”
Michael Chen (Lead Instructor, Code Academy). “Understanding how to uncomment lines in Python is crucial for beginners. It not only allows for the testing of code snippets but also teaches the importance of code organization and clarity. Using shortcuts like ‘Ctrl + /’ in many IDEs can significantly speed up this process.”
Sarah Johnson (Technical Writer, Python Programming Journal). “Effective commenting and uncommenting practices are vital for maintaining clean code. In Python, the ability to uncomment lines easily can facilitate collaboration among team members, ensuring that everyone is on the same page when it comes to code functionality.”
Frequently Asked Questions (FAQs)
How do I uncomment a single line in Python?
To uncomment a single line in Python, simply remove the “ symbol at the beginning of the line. This will enable the line of code to execute.
Can I uncomment multiple lines at once in an IDE?
Yes, most Integrated Development Environments (IDEs) allow you to uncomment multiple lines simultaneously. You can usually select the lines and use a specific shortcut, such as `Ctrl + /` in many editors, to toggle comments.
What is the purpose of commenting and uncommenting code?
Commenting code helps to document and explain the code’s functionality, making it easier for others (and yourself) to understand. Uncommenting allows you to re-enable previously disabled code for testing or execution.
Is there a shortcut for uncommenting in Jupyter Notebook?
In Jupyter Notebook, you can uncomment a line by selecting it and pressing `Ctrl + /`. This will toggle the comment status of the selected line.
Can I uncomment a block of code in Python?
Yes, to uncomment a block of code, select the lines you wish to uncomment and remove the “ symbols from the beginning of each line. Alternatively, use the commenting shortcut in your code editor.
What happens if I forget to uncomment a line of code?
If you forget to uncomment a line of code, that line will not execute, which may lead to errors or unexpected behavior in your program. It is essential to ensure that necessary code is uncommented for proper functionality.
In Python, commenting is a crucial practice that aids in code readability and maintenance. To uncomment lines of code, one must remove the comment indicators, which are typically the hash symbol () for single-line comments. For multi-line comments, triple quotes can be utilized, and to uncomment, simply remove the triple quotes surrounding the block of text. Understanding how to effectively uncomment code is essential for debugging and enhancing code functionality.
Key takeaways include the importance of comments in programming, as they provide context and explanations for complex code sections. Moreover, the ability to uncomment lines efficiently allows developers to test and modify their code without permanently altering the original logic. This practice not only fosters better collaboration among team members but also aids in the learning process for those new to programming.
In summary, mastering the process of uncommenting in Python is a fundamental skill for any programmer. It ensures that code remains clean and understandable while allowing for flexibility during development. By adhering to best practices in commenting and uncommenting, developers can enhance their coding efficiency and contribute to more robust software solutions.
Author Profile
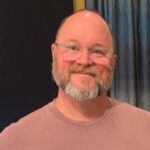
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?