How Can You Effectively Test an SMTP Server Using PowerShell?
In today’s digital landscape, email communication remains a cornerstone of business operations and personal correspondence alike. However, ensuring that your SMTP (Simple Mail Transfer Protocol) server is functioning correctly is crucial to maintaining seamless email delivery. Whether you’re a system administrator troubleshooting email issues or a developer testing application functionalities, knowing how to test an SMTP server effectively can save you time and prevent potential disruptions. This article will guide you through the process of using PowerShell to test your SMTP server, empowering you with the tools needed to diagnose and resolve email-related challenges efficiently.
SMTP servers play a vital role in the transmission of emails, acting as the backbone of email communication. However, like any technology, they can encounter issues that hinder their performance. Testing your SMTP server can help identify problems such as connectivity issues, authentication failures, or configuration errors. By leveraging PowerShell, a powerful scripting language built into Windows, you can perform these tests with ease and precision, streamlining the troubleshooting process.
In this article, we will explore the fundamental concepts behind SMTP servers and the importance of testing them regularly. We will also delve into the capabilities of PowerShell, highlighting its versatility and effectiveness in managing and testing server configurations. By the end, you will be equipped with practical knowledge and techniques to ensure your SMTP
Using PowerShell to Test SMTP Server Connectivity
To effectively test your SMTP server using PowerShell, you can utilize the `Telnet` command or the `Send-MailMessage` cmdlet. However, if your system does not have Telnet enabled, using PowerShell’s built-in capabilities provides a robust alternative.
To verify SMTP server connectivity, follow these steps:
- Open PowerShell: Launch PowerShell with administrative privileges to ensure you have the necessary permissions.
- Define SMTP Server Details: You need the hostname or IP address of the SMTP server and the port number (commonly port 25, 587, or 465).
- Test SMTP Connection: Use the `Test-NetConnection` cmdlet to check if the SMTP server is reachable on the specified port. For example:
“`powershell
Test-NetConnection -ComputerName smtp.yourdomain.com -Port 25
“`
This command will return information about the connection, including whether it was successful.
Sending a Test Email
To further validate the SMTP server’s functionality, you can attempt to send a test email using the `Send-MailMessage` cmdlet. Make sure you have the necessary credentials and server settings. Here’s how to do it:
“`powershell
$SmtpServer = “smtp.yourdomain.com”
$SmtpPort = 587
$Username = “[email protected]”
$Password = ConvertTo-SecureString “your_password” -AsPlainText -Force
$Credential = New-Object System.Management.Automation.PSCredential($Username, $Password)
Send-MailMessage -From “[email protected]” -To “[email protected]” -Subject “Test Email” -Body “This is a test email.” -SmtpServer $SmtpServer -Port $SmtpPort -Credential $Credential -UseSsl
“`
In this script, replace the placeholder values with your actual SMTP server details and credentials. The `-UseSsl` parameter is important if your SMTP server requires a secure connection.
Interpreting Results
When you execute the commands, you will receive outputs that indicate the status of your SMTP server connection and email sending capabilities. Here are the possible outcomes:
Outcome | Description |
---|---|
Success | The connection was successful, and the email was sent without errors. |
Failure | The connection could not be established. Check your SMTP server address, port, and network settings. |
Authentication Error | Your credentials may be incorrect, or the SMTP server might require different authentication methods. |
SSL Error | The server may not support SSL/TLS if you encounter this error. Verify server settings. |
Understanding these results is crucial for diagnosing issues with the SMTP server. If errors occur, ensure that the firewall settings allow traffic on the specified SMTP port and that your credentials are correct.
Using PowerShell to Test an SMTP Server
To test an SMTP server using PowerShell, you can utilize the `Send-MailMessage` cmdlet or establish a TCP connection with the server. Each method has its advantages, depending on the level of detail you require from your tests.
Testing SMTP with Send-MailMessage
The `Send-MailMessage` cmdlet is a straightforward method to send an email directly through the SMTP server. Here’s how to utilize it:
“`powershell
Send-MailMessage -From “[email protected]” -To “[email protected]” -Subject “Test Email” -Body “This is a test email.” -SmtpServer “smtp.example.com” -Credential (Get-Credential) -Port 587 -UseSsl
“`
Parameters Explained:
- -From: The sender’s email address.
- -To: The recipient’s email address.
- -Subject: The subject line of the email.
- -Body: The body content of the email.
- -SmtpServer: The hostname or IP address of the SMTP server.
- -Credential: Prompts for credentials to authenticate with the SMTP server.
- -Port: The port used for SMTP (commonly 25, 587, or 465).
- -UseSsl: Indicates whether to use SSL/TLS for the connection.
Considerations:
- Ensure that the SMTP server is reachable from your PowerShell environment.
- Check the firewall settings if you encounter connectivity issues.
- Review the SMTP server’s logs for any authentication or connectivity errors.
Testing SMTP Connectivity with TCP
For a more technical approach, you can test connectivity to the SMTP server using TCP. This method allows you to verify if the server is accepting connections on the specified port.
“`powershell
$SMTPServer = “smtp.example.com”
$Port = 25
$TCPClient = New-Object System.Net.Sockets.TcpClient
try {
$TCPClient.Connect($SMTPServer, $Port)
if ($TCPClient.Connected) {
Write-Host “Connected to $SMTPServer on port $Port”
}
} catch {
Write-Host “Failed to connect to $SMTPServer on port $Port. Error: $_”
} finally {
$TCPClient.Close()
}
“`
Benefits of TCP Testing:
- Provides immediate feedback on connectivity issues.
- Useful for troubleshooting network and firewall configurations.
Using Telnet for SMTP Testing
If you prefer using Telnet, you can manually send SMTP commands to the server. This method is useful for debugging and checking server responses.
- Open Command Prompt or PowerShell.
- Type the following command:
“`bash
telnet smtp.example.com 25
“`
- After connecting, you can issue commands such as:
- `HELO yourdomain.com`
- `MAIL FROM: [email protected]`
- `RCPT TO: [email protected]`
- `DATA`
- Type your email content followed by a period (`.`) on a new line to end the data section.
Commands Overview:
Command | Description |
---|---|
`HELO` | Introduces the client to the server. |
`MAIL FROM` | Specifies the sender’s email. |
`RCPT TO` | Specifies the recipient’s email. |
`DATA` | Begins the data section for the email. |
Using these methods, you can effectively test and troubleshoot SMTP server connections and functionality in your PowerShell environment.
Expert Insights on Testing SMTP Servers with PowerShell
Dr. Emily Carter (Senior Network Engineer, Tech Solutions Corp). “Testing an SMTP server using PowerShell is a critical skill for network engineers. Utilizing the `Send-MailMessage` cmdlet allows for quick verification of SMTP configurations and connectivity, ensuring that email services are functioning as expected.”
Michael Tran (IT Security Analyst, CyberSafe Networks). “From a security perspective, testing SMTP servers with PowerShell can help identify vulnerabilities. By sending test emails and analyzing responses, IT professionals can ensure that their email servers are not only operational but also secure against common threats.”
Laura Jenkins (Systems Administrator, CloudTech Innovations). “PowerShell provides a versatile environment for SMTP testing. By scripting automated tests, administrators can monitor server performance over time and quickly address any issues that arise, making it an invaluable tool in system maintenance.”
Frequently Asked Questions (FAQs)
How can I test an SMTP server using PowerShell?
You can test an SMTP server by using the `Send-MailMessage` cmdlet in PowerShell. Specify the SMTP server, sender, recipient, subject, and body of the email to verify connectivity and functionality.
What parameters do I need to provide for Send-MailMessage?
You need to provide parameters such as `-SmtpServer` (the SMTP server address), `-From` (the sender’s email), `-To` (the recipient’s email), `-Subject` (the email subject), and `-Body` (the email content). Optionally, you can include `-Credential` for authentication.
Can I test SMTP authentication with PowerShell?
Yes, you can test SMTP authentication by using the `-Credential` parameter with `Send-MailMessage`. This allows you to provide a username and password for servers that require authentication.
What if my SMTP server uses SSL/TLS?
If your SMTP server requires SSL/TLS, use the `-UseSsl` parameter in the `Send-MailMessage` cmdlet. Ensure that your SMTP server supports this option for secure communication.
How do I check if the SMTP server is reachable?
You can check the reachability of the SMTP server by using the `Test-NetConnection` cmdlet in PowerShell. Specify the SMTP server address and port (usually 25, 587, or 465) to determine if the server is accessible.
What should I do if I encounter errors while testing the SMTP server?
If you encounter errors, verify the SMTP server address, check network connectivity, ensure the correct port is used, and confirm that your credentials are valid. Reviewing the error message can provide specific insights into the issue.
Testing an SMTP server using PowerShell is a crucial task for system administrators and IT professionals. The process involves utilizing the built-in cmdlets and .NET classes to establish a connection with the SMTP server, send test emails, and verify the server’s response. This ensures that the server is configured correctly and can handle email communication effectively. By following the appropriate commands and scripts, users can efficiently diagnose connectivity issues and validate SMTP configurations.
One of the key takeaways is the importance of understanding the parameters required for the SMTP connection, such as the server address, port number, and authentication details. Additionally, leveraging PowerShell’s capabilities allows for automation and scripting, making it easier to perform repeated tests or integrate SMTP testing into larger monitoring solutions. This not only saves time but also enhances the reliability of email services by providing timely feedback on server health.
Moreover, it is essential to consider security aspects when testing SMTP servers. Using secure connections (SSL/TLS) and proper authentication methods is vital to protect sensitive information during transmission. PowerShell provides options to implement these security measures, ensuring that email communications remain secure and compliant with organizational policies.
testing an SMTP server using PowerShell is a straightforward yet powerful method to ensure
Author Profile
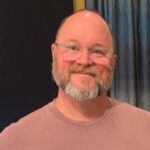
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?