How Can You Effectively Test Your JavaScript Code?
In the dynamic world of web development, JavaScript stands as a cornerstone, powering everything from interactive web pages to complex applications. However, as with any programming language, writing code is only half the battle; ensuring that code functions correctly and efficiently is where the real challenge lies. Testing JavaScript code is not just a best practice—it’s a necessity for developers who aim to deliver seamless user experiences and maintain high-quality standards. In this article, we will explore the essential techniques and tools that can elevate your JavaScript testing game, ensuring your code is robust, reliable, and ready for the real world.
When it comes to testing JavaScript, understanding the various methodologies is crucial. From unit testing, which focuses on individual components, to integration and end-to-end testing that assess the interactions between different parts of an application, each approach serves a unique purpose in the development lifecycle. By employing these strategies, developers can catch bugs early, improve code quality, and ultimately save time and resources in the long run.
Moreover, the landscape of JavaScript testing is enriched by a plethora of frameworks and libraries designed to streamline the process. Tools like Jest, Mocha, and Cypress provide powerful features that simplify writing and executing tests, making it easier for developers to integrate testing into their
Unit Testing
Unit testing is a fundamental practice in software development that involves testing individual components or functions of the code to ensure they work as intended. In JavaScript, there are several frameworks available for unit testing, such as Jest, Mocha, and Jasmine. These frameworks provide tools to write and execute tests, making it easier to catch errors early.
When implementing unit tests, consider the following best practices:
- Write tests for every function.
- Keep tests isolated; each test should only verify one behavior.
- Use descriptive names for your test cases to clarify what they are testing.
- Run tests frequently to catch issues early in the development process.
Here is an example of a simple unit test using Jest:
“`javascript
function add(a, b) {
return a + b;
}
test(‘adds 1 + 2 to equal 3’, () => {
expect(add(1, 2)).toBe(3);
});
“`
Integration Testing
Integration testing focuses on verifying the interactions between different modules or services in your application. This type of testing ensures that combined components work together as expected. Tools like Cypress and TestCafe can be utilized for this purpose.
Integration tests often involve:
- Testing data flow between modules.
- Verifying that the system behaves correctly under various conditions.
- Ensuring that APIs function as intended when called from different parts of the application.
An example of an integration test could involve testing a function that interacts with an API. Here is a basic structure using Mocha and Chai:
“`javascript
const chai = require(‘chai’);
const expect = chai.expect;
const axios = require(‘axios’);
describe(‘API Integration’, () => {
it(‘should fetch data from the API’, async () => {
const response = await axios.get(‘https://api.example.com/data’);
expect(response.status).to.equal(200);
expect(response.data).to.have.property(‘id’);
});
});
“`
End-to-End Testing
End-to-end (E2E) testing simulates real user scenarios and validates the system as a whole. This type of testing verifies that the application behaves correctly from the user’s perspective. Tools such as Selenium, Cypress, and Puppeteer are commonly used for E2E testing.
Key aspects of E2E testing include:
- Testing complete workflows and user interactions.
- Validating that all components work together seamlessly.
- Checking the application’s performance under load.
A simple example of an E2E test using Cypress might look like this:
“`javascript
describe(‘My First Test’, () => {
it(‘Visits the Kitchen Sink’, () => {
cy.visit(‘https://example.cypress.io’);
cy.contains(‘type’).click();
cy.url().should(‘include’, ‘/commands/actions’);
cy.get(‘.action-email’).type(‘[email protected]’).should(‘have.value’, ‘[email protected]’);
});
});
“`
Test-Driven Development (TDD)
Test-Driven Development (TDD) is a software development approach where tests are written before the actual code. This methodology encourages developers to think through the design and requirements of their code ahead of time, leading to better architecture and fewer defects.
The TDD process typically follows these steps:
- Write a failing test for the desired functionality.
- Write the minimum code necessary to pass the test.
- Refactor the code while ensuring all tests still pass.
Here is a summary table illustrating the TDD cycle:
Step | Description |
---|---|
Write Test | Define a test for a new feature or functionality. |
Run Test | Execute the test to ensure it fails (as expected). |
Implement Code | Write the minimum code required to pass the test. |
Run Test Again | Verify that the test passes with the new code. |
Refactor | Improve the code while ensuring all tests remain passing. |
Understanding Testing Frameworks
Various testing frameworks exist for JavaScript, each with unique features and purposes. Selecting the right framework is crucial for effective testing. Below are some of the most popular frameworks:
- Jest
- Created by Facebook, Jest is widely used for testing React applications.
- Provides a zero-config setup and built-in mocking capabilities.
- Mocha
- A flexible framework that allows for asynchronous testing.
- Supports a variety of assertion libraries like Chai.
- Jasmine
- A behavior-driven development framework that does not require a DOM.
- Comes with built-in assertions and spies.
- Cypress
- A front-end testing tool specifically designed for end-to-end testing.
- Provides a complete testing environment with time-travel debugging.
Writing Tests
Writing effective tests requires a clear understanding of the code being tested. Below are key principles:
- Unit Tests: Focus on individual components or functions.
- Use mocks and stubs to isolate code.
- Integration Tests: Assess the interaction between multiple components.
- Ensure that components work together as expected.
- End-to-End Tests: Simulate user interactions with the application.
- Test the application as a whole to verify overall functionality.
Best Practices for Testing
Implementing best practices can enhance the quality and maintainability of your tests. Consider the following guidelines:
- Keep Tests Isolated: Each test should be independent to avoid cascading failures.
- Use Descriptive Naming: Test names should clearly indicate their purpose.
- Automate Testing: Integrate tests into the CI/CD pipeline for continuous feedback.
- Regularly Refactor Tests: Update and optimize tests alongside application changes.
- Use Coverage Tools: Tools like Istanbul can help identify untested areas of your code.
Running Tests
Running tests can typically be done using command-line interfaces. Here’s how to execute tests in various frameworks:
Framework | Command to Run Tests |
---|---|
Jest | `npm test` or `jest` |
Mocha | `mocha` |
Jasmine | `jasmine` |
Cypress | `cypress open` or `cypress run` |
Debugging Tests
Debugging tests is essential for identifying issues. Here are some methods to effectively debug JavaScript tests:
- Console Logging: Insert `console.log()` statements to trace variable values and execution flow.
- Debugging Tools: Use IDE debugging features or browser developer tools to step through test execution.
- Use Assertions: Employ assertions to validate expected outcomes; failing assertions can help pinpoint issues.
Continuous Testing and Integration
Integrating testing into the development workflow ensures that code is continuously verified. Key components include:
- Continuous Integration (CI): Tools like Travis CI, CircleCI, or GitHub Actions automate the testing process.
- Test-Driven Development (TDD): Write tests before implementing functionality to drive development.
- Regular Commit Hooks: Implement pre-commit hooks to run tests before code is pushed.
Implementing a robust testing strategy enhances code quality and application reliability. By understanding frameworks, writing effective tests, and integrating testing into your workflow, you can ensure your JavaScript applications perform as intended.
Expert Insights on Testing JavaScript Code
Dr. Emily Carter (Lead Software Engineer, CodeQuality Labs). “Effective testing of JavaScript code begins with a solid understanding of both unit and integration testing frameworks. Utilizing tools like Jest and Mocha can significantly enhance the reliability of your code by ensuring that individual components function correctly before they are integrated.”
Mark Thompson (Senior Developer Advocate, Tech Innovations Inc.). “Incorporating automated testing into your JavaScript development workflow is crucial. Continuous integration tools can help run tests automatically on code changes, allowing developers to catch errors early and maintain a high standard of code quality.”
Lisa Chen (JavaScript Specialist, WebDev Solutions). “When testing JavaScript code, it is essential to cover edge cases and potential user interactions. Tools like Cypress for end-to-end testing provide valuable insights into how your application behaves in real-world scenarios, ensuring a robust user experience.”
Frequently Asked Questions (FAQs)
How can I test JavaScript code in a browser?
You can test JavaScript code in a browser by using the Developer Tools. Open the tools (usually by pressing F12 or right-clicking and selecting “Inspect”), navigate to the “Console” tab, and enter your JavaScript code directly there. Press Enter to execute it.
What are unit tests in JavaScript?
Unit tests are automated tests that verify the functionality of individual components or functions in your JavaScript code. They ensure that each part of the code behaves as expected, facilitating easier debugging and maintenance.
Which frameworks are commonly used for testing JavaScript?
Common frameworks for testing JavaScript include Jest, Mocha, Jasmine, and QUnit. These frameworks provide various utilities for writing and running tests efficiently.
How do I set up Jest for testing my JavaScript code?
To set up Jest, install it via npm by running `npm install –save-dev jest`. Create a test file with a `.test.js` extension, write your test cases, and run the tests using the command `npx jest`.
What is the purpose of integration testing in JavaScript?
Integration testing verifies the interaction between different modules or components in a JavaScript application. It ensures that combined parts of the application work together as intended, identifying issues that may not be apparent in unit tests.
Can I test asynchronous JavaScript code?
Yes, you can test asynchronous JavaScript code using Promises or async/await syntax. Most testing frameworks, including Jest and Mocha, provide support for handling asynchronous tests, allowing you to ensure that your code behaves correctly even with asynchronous operations.
Testing JavaScript code is a critical aspect of the development process, ensuring that applications function as intended and maintain high quality. Various methodologies exist for testing, including unit testing, integration testing, and end-to-end testing. Each of these approaches serves a specific purpose, allowing developers to isolate and verify individual components, assess the interaction between modules, and evaluate the overall user experience, respectively. By employing a combination of these testing strategies, developers can effectively identify and rectify issues early in the development cycle.
Additionally, leveraging testing frameworks and libraries such as Jest, Mocha, and Cypress can significantly streamline the testing process. These tools provide robust environments for writing and executing tests, offering features such as assertions, mocking, and test runners. The choice of framework often depends on the specific requirements of the project, including the complexity of the application and the team’s familiarity with the tools. Integrating automated testing into the development workflow further enhances efficiency, allowing for continuous integration and delivery practices.
Ultimately, the key takeaway from the discussion on testing JavaScript code is the importance of adopting a structured approach to ensure code reliability and performance. By prioritizing testing throughout the development lifecycle, teams can reduce the likelihood of bugs, improve code maintainability, and enhance user satisfaction
Author Profile
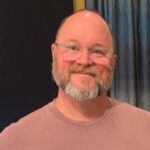
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?