How Can You Effectively Suppress Warnings in Python?
### Introduction
In the world of programming, warnings can often feel like unwelcome guests at a party—sometimes helpful, but often just adding noise to the experience. Python, with its robust error handling and user-friendly syntax, is no stranger to these notifications. While warnings serve a crucial purpose in alerting developers to potential issues in their code, there are times when you may want to suppress them to maintain focus or streamline your output. Whether you’re debugging a complex application or simply trying to keep your console clean, understanding how to manage these warnings is essential for any Python programmer.
Suppressing warnings in Python is not just about silencing notifications; it’s about creating an environment conducive to productivity and clarity. As you delve deeper into this topic, you’ll discover various methods to control the visibility of these messages, allowing you to tailor your coding experience to your needs. From utilizing built-in libraries to implementing custom solutions, the tools at your disposal can help you navigate through the noise and focus on what truly matters: writing efficient, effective code.
In this article, we will explore the different techniques available for suppressing warnings in Python. By the end, you’ll be equipped with the knowledge to manage these alerts effectively, ensuring that your development process remains as smooth and efficient as possible. So, let’s
Understanding Python Warnings
Python provides a built-in warnings module that allows developers to issue warning messages. These messages can alert the user to potential issues in the code without raising an exception. Warnings are generally used to inform developers about deprecated features, potential bugs, or other important notices that don’t stop program execution. However, in certain scenarios, you may wish to suppress these warnings to maintain clean output or to focus on more critical issues.
Methods to Suppress Warnings
There are several effective methods to suppress warnings in Python. Each method can be applied depending on the specific context or requirement.
Using the Warnings Module
The `warnings` module provides a straightforward way to suppress warnings globally or locally. The `filterwarnings()` function allows you to filter out specific warnings.
- Suppressing All Warnings Globally:
python
import warnings
warnings.filterwarnings(“ignore”)
- Suppressing Specific Warning Types:
python
import warnings
warnings.filterwarnings(“ignore”, category=UserWarning)
- Suppressing Warnings in a Specific Context:
You can use a context manager to suppress warnings only in a certain block of code:
python
import warnings
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
# Code that triggers warnings here
Using the `-W` Command Line Option
When running a Python script from the command line, you can use the `-W` option to control warning behavior directly. For instance, to ignore all warnings, run:
bash
python -W ignore your_script.py
Alternatively, to ignore specific warnings:
bash
python -W “ignore::UserWarning” your_script.py
Suppressing Warnings in Jupyter Notebooks
If you are working in a Jupyter notebook, you might want to suppress warnings using IPython commands:
python
import warnings
warnings.filterwarnings(“ignore”)
You can also use the following magic command:
python
%config Application.verbose_crash=
Comparison of Suppression Methods
The following table summarizes the different methods to suppress warnings in Python:
Method | Scope | Usage |
---|---|---|
Global Suppression | Entire script | Simple and effective for all warnings |
Specific Warning Type | Entire script | Good for targeting specific warnings |
Context Manager | Block of code | Ideal for localized suppression |
Command Line Option | Entire script | Useful for command-line execution |
Jupyter Notebook Suppression | Notebook cell | Best for interactive environments |
By using these methods, you can effectively manage warnings in your Python applications, allowing for cleaner and more focused output.
Understanding Warnings in Python
Warnings in Python are generated by the `warnings` module, which alerts developers to potential issues in their code that may not necessarily raise exceptions but could lead to problems down the line. Common scenarios that trigger warnings include deprecated features, usage of outdated libraries, or issues with data types.
Methods to Suppress Warnings
There are several effective methods to suppress warnings in Python. Each method can be used depending on the context and specific requirements of your project.
Using the `warnings` Module
The `warnings` module provides straightforward functions to control the display of warnings. You can suppress warnings globally or locally in your code.
- Suppressing all warnings globally:
python
import warnings
warnings.filterwarnings(“ignore”)
- Suppressing specific warnings:
python
warnings.filterwarnings(“ignore”, category=DeprecationWarning)
- Suppressing warnings in a specific context:
python
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
# Code that triggers warnings
Suppressing Warnings via Command Line
You can also suppress warnings when running Python scripts from the command line by using the `-W` option:
bash
python -W ignore your_script.py
This command ignores all warnings for the duration of the script.
Using Context Managers
For finer control, context managers can be utilized to suppress warnings only in specific blocks of code. This is particularly useful when you want to ensure warnings are only ignored in certain parts of your application.
python
import warnings
def some_function():
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
# Code that may generate warnings
Selective Suppression with Filters
You may want to suppress warnings based on specific conditions or categories. Here are the main types of filters available:
Filter Type | Description |
---|---|
`ignore` | Ignores the specified warning. |
`always` | Always shows the specified warning. |
`error` | Treats the specified warning as an error. |
`default` | Displays the warning once per location. |
`module` | Displays the warning once per module. |
`once` | Displays the warning once, regardless of location. |
Example of selective suppression:
python
warnings.filterwarnings(“ignore”, category=UserWarning, module=’your_module_name’)
Best Practices for Suppressing Warnings
When suppressing warnings, consider the following best practices:
- Suppress selectively: Avoid suppressing all warnings globally. Instead, target specific categories or modules to maintain awareness of potential issues in your code.
- Document suppression: Clearly comment on why specific warnings are being suppressed to aid future developers in understanding the rationale behind the decision.
- Regularly review code: Periodically revisit the warnings you are suppressing, as the underlying reasons may change with updates to libraries or Python itself.
- Use warnings as guidance: Treat warnings as informative messages that could help improve code quality rather than merely obstacles to be ignored.
By following these guidelines, you can effectively manage warnings in your Python applications while maintaining code quality and readability.
Expert Insights on Suppressing Warnings in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Suppressing warnings in Python can be essential for maintaining clean output, especially in production environments. Utilizing the `warnings` module effectively allows developers to control the visibility of warnings, ensuring that only critical issues are highlighted.”
Michael Chen (Lead Software Engineer, CodeCrafters). “It is important to approach warning suppression with caution. While the `warnings.filterwarnings()` function can be used to ignore specific warnings, developers should ensure they do not overlook significant issues that could arise from ignoring them.”
Sarah Patel (Python Educator and Author). “In educational settings, I encourage students to understand the nature of warnings rather than suppressing them outright. However, for seasoned developers, using context managers like `with warnings.catch_warnings()` provides a flexible way to suppress warnings temporarily without affecting the global state.”
Frequently Asked Questions (FAQs)
How can I suppress warnings in Python?
You can suppress warnings in Python using the `warnings` module. By calling `warnings.filterwarnings(“ignore”)`, you can prevent warnings from being displayed.
What is the purpose of suppressing warnings?
Suppressing warnings can help maintain clean output, especially in production environments where warnings may not be relevant to end users or may clutter logs.
Can I suppress specific warnings instead of all warnings?
Yes, you can suppress specific warnings by using `warnings.filterwarnings(“ignore”, category=YourWarningType)`, where `YourWarningType` is the specific warning class you want to ignore.
Is it possible to temporarily suppress warnings in a specific section of code?
Yes, you can use the `warnings.catch_warnings()` context manager to temporarily suppress warnings within a specific block of code, ensuring that warnings are only ignored during that context.
What are the potential risks of suppressing warnings?
Suppressing warnings can lead to overlooking important issues in the code, such as deprecated features or potential bugs, which may result in unexpected behavior or performance problems.
How do I enable warnings again after suppressing them?
To re-enable warnings after suppressing them, you can call `warnings.filterwarnings(“default”)` or simply exit the context manager if you used `warnings.catch_warnings()`.
Suppressing warnings in Python is an essential practice for developers who wish to maintain clean and readable output, especially in production environments. Python provides several mechanisms to manage warnings, primarily through the `warnings` module. This module allows users to filter warnings based on specific conditions, enabling them to ignore, display, or even convert warnings into errors if necessary. Understanding how to effectively utilize this module can enhance code clarity and prevent unnecessary distractions during execution.
Key takeaways include the importance of using the `warnings.filterwarnings()` function to control the visibility of warnings. This function allows developers to specify the type of warnings they want to suppress, whether they are specific to a module, category, or message. Additionally, using context managers like `warnings.catch_warnings()` can provide localized control over warning suppression, allowing developers to suppress warnings only in specific sections of code without affecting the entire program.
Moreover, it is crucial to strike a balance between suppressing warnings and addressing the underlying issues that cause them. While it may be tempting to silence warnings, developers should consider reviewing and resolving the root causes to ensure code quality and maintainability. By doing so, they can prevent potential issues that may arise from ignoring important warnings, ultimately leading to more robust and reliable
Author Profile
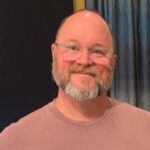
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?