How Can You Effectively Stop Code Execution in Python?
In the world of programming, particularly in Python, the ability to control the flow of your code is paramount. Whether you’re debugging a complex application or simply experimenting with scripts, knowing how to stop the execution of your code can save you time and frustration. Python offers several methods to halt your programs, each suited for different scenarios and needs. Understanding these techniques not only enhances your coding efficiency but also empowers you to manage errors and unexpected behavior gracefully.
When working with Python, there are various situations where you might want to stop your code from running. It could be due to encountering an error, reaching a specific condition, or even during the development phase when you want to test certain functionalities without running the entire program. Each of these scenarios requires a different approach, from using built-in functions to implementing exception handling. By mastering these techniques, you can gain better control over your code and ensure that your programs behave as expected.
Moreover, knowing how to effectively stop your code can lead to improved debugging practices. Instead of letting your program run into infinite loops or unintended consequences, you can strategically halt execution, allowing you to analyze the current state of your application. This skill not only enhances your problem-solving abilities but also contributes to writing cleaner, more efficient code. As we delve deeper into
Using Keyboard Interrupts
One of the most straightforward methods to stop a running Python program is by using a keyboard interrupt. This is achieved by pressing `Ctrl+C` in the terminal or command prompt where the script is executing. This sends a signal to the Python interpreter to terminate the program immediately.
When you issue a keyboard interrupt, Python raises a `KeyboardInterrupt` exception, which can be handled gracefully if necessary. For example, you can implement a try-except block to manage cleanup operations before the program exits:
“`python
try:
while True:
pass Your main code here
except KeyboardInterrupt:
print(“Program terminated by user.”)
“`
Utilizing the sys.exit() Function
The `sys.exit()` function from the `sys` module is another way to programmatically terminate a Python script. This function can be called at any point in your code to stop execution. It can also accept an optional argument to indicate an exit status:
“`python
import sys
def main():
print(“Program is running.”)
sys.exit(“Exiting the program.”)
main()
“`
By convention, an exit status of `0` indicates a successful termination, while any non-zero value indicates an error.
Exiting with os._exit()
For scenarios where you need to stop a program immediately, `os._exit()` can be utilized. This function exits the process without calling cleanup handlers, flushing stdio buffers, etc. It is particularly useful in child processes created using the `os` module.
“`python
import os
def terminate_process():
print(“Terminating process immediately.”)
os._exit(0)
terminate_process()
“`
Handling Signals for Graceful Shutdown
Python allows you to handle various operating system signals, which can be useful for graceful shutdowns of your applications. The `signal` module can be used to define custom behavior when receiving specific signals, such as `SIGINT` (interrupt signal).
“`python
import signal
import time
def signal_handler(sig, frame):
print(“Signal received, shutting down gracefully.”)
exit(0)
signal.signal(signal.SIGINT, signal_handler)
while True:
print(“Running…”)
time.sleep(1)
“`
This example sets up a signal handler that will execute the `signal_handler` function when a `SIGINT` signal is received, allowing for cleanup operations before exiting.
Comparison of Termination Methods
The following table summarizes the various methods to stop code execution in Python, highlighting their characteristics and use cases:
Method | Characteristics | Use Case |
---|---|---|
Keyboard Interrupt (Ctrl+C) | Immediate stop; raises KeyboardInterrupt | Interactive scripts where user input is expected |
sys.exit() | Programmatically exits; can provide exit status | Regular termination from within code |
os._exit() | Immediate exit; skips cleanup | Child processes needing abrupt termination |
Signal Handling | Custom exit behavior on receiving signals | Long-running processes that need graceful shutdown |
Each method has its own advantages and scenarios where it is most applicable, allowing developers to choose the best approach based on their specific requirements.
Using Keyboard Interrupts
One of the simplest methods to stop a running Python program is by using keyboard interrupts. This can be achieved by pressing `Ctrl + C` in the terminal or command prompt where the script is running. This sends a `KeyboardInterrupt` exception to the program, which can be caught or ignored.
Handling Keyboard Interrupts in Code
To gracefully handle a keyboard interrupt, you can use a try-except block:
“`python
try:
while True:
print(“Running…”)
except KeyboardInterrupt:
print(“Program stopped.”)
“`
This code will allow your program to handle the interrupt smoothly, performing any necessary cleanup before exiting.
Exiting with sys.exit()
The `sys` module provides a method called `exit()` that can be used to terminate a program. This is particularly useful when you want to exit from within a function or based on a specific condition.
Example of Using sys.exit()
“`python
import sys
def main():
print(“This will run.”)
sys.exit(“Exiting the program.”)
main()
“`
In this example, the program will print a message and then terminate with the specified exit message.
Using os._exit() for Immediate Exit
For situations where you need to exit the program immediately without cleanup, `os._exit()` can be used. This method does not raise any exceptions and skips the normal termination procedures.
Example of Using os._exit()
“`python
import os
print(“This will run.”)
os._exit(0)
print(“This will not run.”)
“`
In this case, the last print statement will not execute because `os._exit()` terminates the interpreter immediately.
Terminating Threads
If your Python program uses threads, stopping it can be more complex. Python does not provide a direct way to kill threads; instead, you should implement a flag that the thread checks regularly to determine if it should stop.
Example of Stopping a Thread
“`python
import threading
import time
stop_event = threading.Event()
def run():
while not stop_event.is_set():
print(“Thread is running…”)
time.sleep(1)
thread = threading.Thread(target=run)
thread.start()
Stop the thread after 5 seconds
time.sleep(5)
stop_event.set()
thread.join()
print(“Thread has been stopped.”)
“`
In this code, the `stop_event` is used to signal the thread to stop gracefully.
Using Signals to Stop a Program
In Unix-like systems, you can use the `signal` module to handle asynchronous events. This allows you to define handlers for specific signals like `SIGINT` (which is sent by `Ctrl + C`).
Example of Using Signals
“`python
import signal
import time
def handler(signum, frame):
print(“Signal handler called with signal:”, signum)
exit(0)
signal.signal(signal.SIGINT, handler)
while True:
print(“Running…”)
time.sleep(1)
“`
This example sets up a signal handler that will print a message and exit when a `SIGINT` signal is received.
Using a Timeout in Functions
You can also implement a timeout mechanism in your functions to ensure that they do not run indefinitely. The `signal` module can be used to raise an exception after a specified timeout.
Example of Timeout with Signals
“`python
import signal
def handler(signum, frame):
raise TimeoutError(“Function timed out.”)
signal.signal(signal.SIGALRM, handler)
def long_running_function():
signal.alarm(5) Set a timeout of 5 seconds
try:
while True:
print(“Working…”)
finally:
signal.alarm(0) Disable the alarm
try:
long_running_function()
except TimeoutError as e:
print(e)
“`
This method allows you to enforce a maximum duration for function execution and respond accordingly.
Expert Insights on Stopping Code Execution in Python
Dr. Emily Carter (Senior Software Engineer, CodeSafe Technologies). “In Python, you can stop the execution of a program using the `sys.exit()` function. This method allows for a clean exit, ensuring that any necessary cleanup can be performed before the program terminates.”
Michael Thompson (Lead Python Developer, Tech Innovations Inc.). “Utilizing exceptions is another effective way to halt code execution in Python. By raising an exception, you can control the flow of your program and exit at specific points based on certain conditions.”
Sarah Lee (Python Instructor, Code Academy). “For interactive environments, such as Jupyter notebooks, you can use the `KeyboardInterrupt` exception, which allows you to stop the execution of a running cell gracefully by pressing `Ctrl+C`.”
Frequently Asked Questions (FAQs)
How can I stop a running Python script in the terminal?
To stop a running Python script in the terminal, you can typically use the keyboard shortcut `Ctrl + C`. This sends a keyboard interrupt signal to the Python process, terminating it.
What is the purpose of the `sys.exit()` function in Python?
The `sys.exit()` function is used to exit from Python. It raises a `SystemExit` exception, which can be caught in outer levels of the program, allowing for cleanup actions before termination.
Can I stop a Python script that is running in an infinite loop?
Yes, you can stop a Python script running in an infinite loop by using `Ctrl + C` in the terminal. Alternatively, you can terminate the process using task management tools like `Task Manager` on Windows or `kill` command on Unix-based systems.
Is there a way to programmatically stop a Python script?
Yes, you can programmatically stop a Python script by using the `exit()` or `sys.exit()` functions. You can also raise an exception or return from the main function to terminate the script.
What should I do if a Python script is unresponsive and won’t stop?
If a Python script becomes unresponsive, you can forcefully terminate it using system tools. On Windows, use `Task Manager` to end the process. On Unix-based systems, you can use the `kill` command followed by the process ID (PID).
How can I gracefully stop a Python script using a signal?
To gracefully stop a Python script using a signal, you can use the `signal` module to handle specific signals like `SIGINT` or `SIGTERM`. Implement a signal handler function that performs cleanup actions before exiting the script.
In Python, stopping code execution can be achieved through various methods depending on the context and requirements. The most common techniques include using the `break` statement to exit loops, the `return` statement to exit functions, and the `sys.exit()` function to terminate the entire program. Each of these methods serves a specific purpose and can be employed effectively to manage the flow of a Python program.
Additionally, handling exceptions is crucial for gracefully stopping code execution when unexpected errors occur. The `try` and `except` blocks allow developers to catch exceptions and take appropriate actions, such as logging the error or performing cleanup tasks before halting the program. This not only enhances the robustness of the code but also improves user experience by preventing abrupt terminations.
Moreover, understanding how to stop code execution is essential for debugging and optimizing performance. By strategically placing exit points in the code, developers can streamline processes and avoid unnecessary computations. This practice contributes to more efficient and maintainable code, ultimately leading to better software development outcomes.
Author Profile
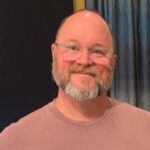
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?