How Can You Effectively Stop a Running Python Code?
Introduction
In the world of programming, particularly with Python, there are moments when code execution needs to come to an abrupt halt. Whether it’s due to an infinite loop, a debugging session gone awry, or simply a need to re-evaluate your approach, knowing how to stop your Python code effectively is an essential skill for every developer. This article will guide you through the various methods and techniques to safely and efficiently terminate your Python scripts, ensuring you maintain control over your coding environment.
When working with Python, you might encounter situations where your script runs longer than expected or gets stuck in a loop. Understanding how to interrupt or terminate your code can save you time and frustration. There are several built-in commands and tools that allow you to stop your code execution gracefully, whether you’re using an integrated development environment (IDE), a command line interface, or even a Jupyter notebook. Each method has its own advantages, and knowing when to use them is key to effective debugging and development.
Moreover, stopping your Python code isn’t just about halting execution; it’s also about understanding the implications of doing so. For instance, terminating a script prematurely can lead to incomplete data processing or corrupted files. As we delve deeper into the various techniques available, we’ll explore not only how to stop your code
Using Keyboard Interrupt
The most straightforward way to stop a running Python script is by using a keyboard interrupt. This is typically done by pressing `Ctrl + C` in the terminal or command prompt where the script is running. This sends a `KeyboardInterrupt` signal to the Python interpreter, which can halt the execution of the code.
Keep in mind that if your code is handling exceptions, you may need to ensure that the `KeyboardInterrupt` is not being caught and ignored. Here’s an example:
python
try:
while True:
pass # Simulate long-running process
except KeyboardInterrupt:
print(“Program stopped by user.”)
This will allow the program to exit gracefully when `Ctrl + C` is pressed.
Using System Exit
For more controlled termination of a Python script, you can use the `sys.exit()` function from the `sys` module. This method is useful when you want to exit a script programmatically, based on certain conditions or events in your code.
python
import sys
def main():
print(“Running main function…”)
# Some conditions
if some_condition:
sys.exit(“Exiting due to some condition.”)
main()
The `sys.exit()` function can take an optional argument, which can be an integer (status code) or a string (message). An integer of `0` generally indicates a successful exit, while any non-zero value indicates an error.
Using the os Module
Another method to stop a Python process is to use the `os` module, particularly `os._exit()`. This function terminates the process immediately without calling cleanup handlers, flushing stdio buffers, etc. It is a more forceful way to exit and should be used judiciously.
python
import os
def terminate():
print(“Terminating process…”)
os._exit(0)
terminate()
This method is particularly useful in scenarios where you want to halt execution without going through the normal shutdown process of a Python script.
Terminating from Another Process
If you need to stop a Python script that is running in another process, you can do so using the `os.kill()` function combined with the process ID (PID) of the running script. This is useful for managing scripts from a controlling application or during development.
python
import os
import signal
# Example PID of the running Python script
pid = 12345
os.kill(pid, signal.SIGTERM) # Sends a termination signal
This method sends a termination signal to the specified process, allowing it to handle cleanup if necessary.
Handling Threads and Asynchronous Code
When dealing with multi-threaded applications or asynchronous code, stopping the execution becomes a bit more complex. In a multi-threaded environment, you may need to set flags that signal threads to stop their execution gracefully.
For asynchronous code, you can use the `asyncio` library’s event loop to cancel tasks. Here’s how to do it:
python
import asyncio
async def main_task():
while True:
print(“Running…”)
await asyncio.sleep(1)
async def stop_task(loop):
await asyncio.sleep(5) # Run for 5 seconds
loop.stop() # Stop the loop
loop = asyncio.get_event_loop()
try:
loop.create_task(main_task())
loop.run_until_complete(stop_task(loop))
finally:
loop.close()
In this example, the main task runs until the event loop is stopped after five seconds.
Method | Description | Use Case |
---|---|---|
Keyboard Interrupt | Stops execution with Ctrl + C | Interactive scripts |
sys.exit() | Programmatic exit with optional status | Conditional exits |
os._exit() | Immediate termination without cleanup | Forceful stops |
os.kill() | Terminate another process | External control |
Asyncio Stop | Graceful cancellation of async tasks | Asynchronous applications |
Methods to Stop Python Code Execution
When working with Python, there are several methods to stop code execution, either gracefully or forcefully. Understanding these methods is essential for effective debugging and control over your Python scripts.
Graceful Termination
Graceful termination allows your program to finish its current tasks and release resources properly before stopping. Here are some common approaches:
- Using the `exit()` function: This function is part of the `sys` module and can be used to terminate a script.
python
import sys
sys.exit()
- Returning from a function: If you are inside a function, you can simply use the `return` statement to exit the function and stop further execution.
- Using exceptions: Raising an exception can also stop execution. This method is useful when you want to indicate an error or specific condition.
python
raise Exception(“Stopping execution”)
Forceful Termination
In some scenarios, you may need to forcibly stop a Python script. Here are methods to achieve this:
- Keyboard Interrupt: Pressing `Ctrl + C` in the terminal sends a KeyboardInterrupt signal to the Python interpreter, causing it to terminate the program immediately.
- Using `os._exit()`: This function terminates the process without calling cleanup handlers, flushing stdio buffers, etc.
python
import os
os._exit(0)
Termination in Different Environments
The method of stopping Python code may vary based on the environment in which the code is executed. Here are some common environments and the recommended termination methods:
Environment | Recommended Method |
---|---|
Terminal/Command Line | `Ctrl + C` or `sys.exit()` |
Jupyter Notebook | Use the “Interrupt Kernel” option |
Integrated Development Environment (IDE) | Stop button or `sys.exit()` |
Web applications | Handle termination via API or user input |
Handling Long-Running Processes
For long-running scripts, it is advisable to implement checks that allow for a controlled shutdown. Some approaches include:
- Signal Handling: You can catch termination signals using the `signal` module.
python
import signal
def signal_handler(signum, frame):
print(“Signal received, stopping execution.”)
sys.exit()
signal.signal(signal.SIGINT, signal_handler)
- Flag Variables: Use a global flag variable to indicate when the program should terminate.
python
should_run = True
def stop_execution():
global should_run
should_run =
while should_run:
# Your code here
if condition_to_stop:
stop_execution()
Utilizing these techniques ensures that you can effectively control the execution flow of your Python scripts, whether you need a soft exit or a hard stop.
Expert Strategies for Halting Python Code Execution
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively stop Python code during execution, developers should utilize the built-in `sys.exit()` function. This method allows for a graceful termination of the program, ensuring that any necessary cleanup can be performed before exiting.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “In scenarios where a script runs indefinitely, implementing signal handling using the `signal` module is crucial. This approach allows the program to respond to external signals, such as keyboard interrupts, providing a controlled way to stop the execution.”
Sarah Patel (Python Instructor, The Coding Academy). “For debugging purposes, using an interactive debugger like `pdb` can be invaluable. It enables developers to pause the execution at any point, inspect the state of the program, and decide whether to continue or halt the process.”
Frequently Asked Questions (FAQs)
How can I stop a running Python script in the terminal?
You can stop a running Python script in the terminal by pressing `Ctrl + C`. This sends a KeyboardInterrupt signal to the Python process, terminating it.
What command can I use to kill a Python process in Linux?
You can use the `kill` command followed by the process ID (PID) of the Python script. First, find the PID using `ps aux | grep python`, then execute `kill
Is there a way to stop a Python script programmatically?
Yes, you can stop a Python script programmatically using `sys.exit()` or `raise SystemExit`. This will terminate the script execution at the point where the command is called.
What should I do if a Python script becomes unresponsive?
If a Python script becomes unresponsive, you can forcefully terminate it using `kill -9
Can I stop a Python script running in an IDE like PyCharm?
Yes, you can stop a Python script running in an IDE like PyCharm by clicking the red stop button in the run console, which sends a termination signal to the script.
How can I handle graceful termination of a Python script?
To handle graceful termination, you can use signal handling with the `signal` module. Define a signal handler function and register it to handle signals like SIGINT or SIGTERM, allowing for cleanup before exiting.
In summary, stopping Python code can be accomplished through various methods, each suited to different scenarios and user needs. The most common approaches include using keyboard interrupts, such as Ctrl+C in the terminal, which sends a signal to terminate the running process. Additionally, programmatically managing execution flow through exception handling or implementing specific exit conditions can provide a more controlled way to halt code execution.
It is also important to consider the context in which the code is running. For instance, in environments like Jupyter notebooks, users can stop code execution using the interrupt button, while in integrated development environments (IDEs), there are often built-in options to terminate running scripts. Understanding these different methods allows developers to choose the most appropriate technique based on their working environment and the nature of the task at hand.
Ultimately, the ability to stop Python code effectively enhances debugging and development efficiency. By mastering these techniques, programmers can ensure that they maintain control over their code execution, allowing for quicker iterations and problem-solving. Recognizing the right method for stopping code can significantly improve workflow and contribute to a more productive programming experience.
Author Profile
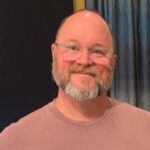
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?