How Can You Effectively Set Up a Docker Reverse Proxy Server?
In today’s digital landscape, where applications are becoming increasingly complex and interconnected, the need for efficient routing and management of web traffic has never been more critical. A reverse proxy server acts as an intermediary for requests from clients seeking resources from other servers, providing a layer of abstraction and control that enhances security, load balancing, and performance. Enter Docker, a powerful platform that simplifies the deployment and management of applications through containerization. By setting up a Docker reverse proxy server, you can streamline your application architecture, optimize resource usage, and ensure seamless access to your services.
Setting up a Docker reverse proxy server not only improves the efficiency of your web applications but also empowers you to manage multiple services with ease. With Docker’s containerization capabilities, you can encapsulate your applications and their dependencies, making deployment and scaling a breeze. A reverse proxy can handle incoming traffic, directing it to the appropriate containerized service based on predefined rules, which enhances both security and performance. This setup is particularly beneficial for microservices architectures, where applications are broken down into smaller, manageable components.
Moreover, implementing a Docker reverse proxy server allows you to take advantage of advanced features such as SSL termination, caching, and load balancing. These functionalities not only improve the user experience but also provide a robust framework for scaling your applications
Choosing a Reverse Proxy Server
When setting up a Docker reverse proxy server, it’s essential to select a suitable software solution that meets your requirements. Common choices include Nginx, Traefik, and HAProxy, each offering unique advantages.
- Nginx: Known for its high performance and low resource consumption, Nginx is widely used for serving static content and acting as a reverse proxy.
- Traefik: Designed for dynamic environments, Traefik integrates seamlessly with Docker, allowing for automatic service discovery and routing.
- HAProxy: A robust option for load balancing, HAProxy excels in managing high traffic loads and offers advanced features for performance optimization.
Setting Up Nginx as a Reverse Proxy
To configure Nginx as a reverse proxy in Docker, follow these steps:
- Create a Docker Network: This allows your containers to communicate.
“`bash
docker network create nginx-network
“`
- Run the Nginx Container: Use a Docker image for Nginx.
“`bash
docker run -d –name nginx-proxy –network nginx-network -p 80:80 -v /path/to/nginx.conf:/etc/nginx/conf.d/default.conf nginx
“`
- Configure Nginx: Create an `nginx.conf` file with the following content:
“`nginx
server {
listen 80;
location / {
proxy_pass http://your_backend_service:port;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
“`
This configuration sets up a basic reverse proxy that forwards traffic to your backend service.
Using Traefik as a Reverse Proxy
Traefik simplifies the reverse proxy setup with built-in support for Docker labels. Here’s how to set it up:
- Run Traefik in Docker:
“`bash
docker run -d –name traefik –network nginx-network -p 80:80 -p 443:443 traefik:v2.5 –providers.docker –entrypoints.web.address=:80
“`
- Label Your Services: When deploying your backend services, add labels to enable Traefik routing:
“`yaml
version: ‘3.7’
services:
myservice:
image: myservice-image
labels:
- “traefik.enable=true”
- “traefik.http.routers.myservice.rule=Host(`yourdomain.com`)”
- “traefik.http.services.myservice.loadbalancer.server.port=80”
“`
This configuration allows Traefik to automatically detect and route traffic to your service based on the specified host.
Configuring SSL with Let’s Encrypt
To secure your reverse proxy with SSL, you can leverage Let’s Encrypt for automatic certificate management. For both Nginx and Traefik, the process involves:
- Nginx:
- Use Certbot to obtain and renew SSL certificates.
- Modify the Nginx configuration to listen on port 443 and include SSL directives.
- Traefik:
- Add the following to your Traefik command:
“`bash
–certificatesresolvers.myresolver.acme.email=your_email@example.com
–certificatesresolvers.myresolver.acme.storage=/acme.json
–certificatesresolvers.myresolver.acme.httpchallenge.entrypoint=web
“`
This setup ensures that your reverse proxy server is secure and can handle HTTPS traffic effectively.
Feature | Nginx | Traefik | HAProxy |
---|---|---|---|
Dynamic Configuration | Manual | Automatic | Manual |
Load Balancing | Yes | Yes | Yes |
SSL Management | Certbot | Automatic with Let’s Encrypt | Manual |
By carefully choosing the appropriate reverse proxy server and correctly configuring SSL, you can enhance the security and performance of your applications running in Docker containers.
Understanding Reverse Proxy
A reverse proxy acts as an intermediary for requests from clients seeking resources from servers. Unlike a traditional proxy, which forwards requests from clients to the internet, a reverse proxy forwards requests from clients to one or more backend servers. This configuration provides several benefits:
- Load Balancing: Distributes incoming traffic across multiple servers.
- SSL Termination: Handles SSL encryption, offloading work from backend servers.
- Caching: Stores frequently accessed content to improve response times.
- Security: Hides the identity and structure of backend servers.
Prerequisites
Before setting up a Docker reverse proxy server, ensure you have the following:
- Docker Installed: Ensure Docker is installed and running on your system.
- Docker Compose: Install Docker Compose for managing multi-container Docker applications.
- Domain Name: A registered domain name pointing to your server’s IP address.
- Basic Knowledge: Familiarity with Docker commands and networking concepts.
Setting Up the Reverse Proxy with Nginx
Nginx is a popular choice for a reverse proxy due to its performance and flexibility. Follow these steps to set it up:
- Create a Docker Network: This allows containers to communicate with each other.
“`bash
docker network create nginx-network
“`
- Create a Docker Compose File: This file defines the services, networks, and volumes.
“`yaml
version: ‘3’
services:
nginx:
image: nginx:latest
container_name: nginx_proxy
restart: always
ports:
- “80:80”
- “443:443”
volumes:
- ./nginx.conf:/etc/nginx/nginx.conf
networks:
- nginx-network
networks:
nginx-network:
external: true
“`
- Configure Nginx: Create an `nginx.conf` file with the following basic configuration:
“`nginx
http {
server {
listen 80;
server_name your-domain.com;
location / {
proxy_pass http://backend_service:port;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
}
“`
- Deploy the Configuration: Run the following command to start the reverse proxy.
“`bash
docker-compose up -d
“`
Adding Backend Services
To route traffic to various backend applications, you can define additional services in the `docker-compose.yml`. For example:
“`yaml
backend_service:
image: your-backend-image
networks:
- nginx-network
“`
Ensure the `proxy_pass` directive in the Nginx configuration points to the correct service name and port.
Testing the Setup
After deploying the reverse proxy and backend services, test the configuration by accessing the domain in your web browser. Use tools like `curl` or Postman to ensure requests are being routed correctly.
SSL Configuration
For secure connections, configure SSL using Let’s Encrypt with Certbot. Update your `docker-compose.yml` to include Certbot service:
“`yaml
certbot:
image: certbot/certbot
volumes:
- ./certs:/etc/letsencrypt
“`
Modify your Nginx configuration to handle HTTPS:
“`nginx
server {
listen 443 ssl;
server_name your-domain.com;
ssl_certificate /etc/letsencrypt/live/your-domain.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/your-domain.com/privkey.pem;
location / {
proxy_pass http://backend_service:port;
…
}
}
“`
Run Certbot to obtain SSL certificates:
“`bash
docker-compose run certbot certonly –webroot –webroot-path=/var/www/certbot -d your-domain.com
“`
Monitoring and Logs
To monitor the reverse proxy and view logs, use:
- Docker Logs: Access logs using `docker logs nginx_proxy`.
- Nginx Access Logs: Typically located in `/var/log/nginx/access.log` inside the container.
Utilizing these tools helps maintain the health and performance of your reverse proxy setup.
Expert Insights on Setting Up a Docker Reverse Proxy Server
Dr. Emily Carter (Cloud Infrastructure Specialist, Tech Innovations Inc.). “Setting up a Docker reverse proxy server is essential for managing multiple applications seamlessly. It allows for better load balancing and can enhance security by hiding the internal architecture from external users.”
James Thompson (DevOps Engineer, Agile Solutions Group). “When configuring a Docker reverse proxy, it is crucial to choose the right tools, such as Nginx or Traefik. These tools not only simplify routing but also provide advanced features like SSL termination and automatic service discovery.”
Linda Nguyen (Software Architect, CloudTech Advisors). “A well-implemented Docker reverse proxy can significantly improve application performance. It serves as a single entry point for requests, enabling efficient request handling and reducing latency for end-users.”
Frequently Asked Questions (FAQs)
What is a Docker reverse proxy server?
A Docker reverse proxy server is an intermediary server that forwards client requests to other servers, allowing for load balancing, SSL termination, and simplified access to multiple services running in Docker containers.
Why would I need a reverse proxy with Docker?
Using a reverse proxy with Docker enhances security, improves performance, and simplifies the management of multiple services by providing a single point of access and enabling features like caching and traffic routing.
How do I set up a reverse proxy using Nginx in Docker?
To set up a reverse proxy using Nginx in Docker, create a Docker container running Nginx, configure the Nginx configuration file to define upstream servers, and map the necessary ports to expose the service.
Can I use Traefik as a reverse proxy for Docker containers?
Yes, Traefik is a popular choice for a reverse proxy in Docker environments, as it automatically discovers services and routes traffic based on defined rules, making it easy to manage microservices.
What are the common issues when configuring a Docker reverse proxy?
Common issues include misconfigured DNS settings, incorrect port mappings, firewall restrictions, and SSL certificate problems, which can lead to service unavailability or improper routing of requests.
Is it necessary to have SSL configured for a Docker reverse proxy?
While not strictly necessary, configuring SSL for a Docker reverse proxy is highly recommended to secure data in transit, protect user privacy, and comply with modern web standards.
Setting up a Docker reverse proxy server is an essential task for managing multiple web applications efficiently. By utilizing a reverse proxy, you can streamline traffic management, enhance security, and simplify the deployment of services. The process typically involves configuring a reverse proxy container, such as Nginx or Traefik, which routes requests to various backend containers based on specified rules. This setup not only improves resource utilization but also provides a centralized point for SSL termination and load balancing.
One of the key takeaways from the discussion is the importance of proper configuration. Ensuring that the reverse proxy is correctly set up to handle domain routing, SSL certificates, and security headers can significantly impact the performance and security of your applications. Additionally, utilizing Docker Compose can simplify the orchestration of your containers, allowing for easy scaling and management of services. Understanding the networking aspects within Docker is also crucial, as it enables seamless communication between the proxy and backend services.
Furthermore, monitoring and logging are vital components of maintaining a reverse proxy server. Implementing tools for tracking performance metrics and access logs can help identify bottlenecks and security threats. Regular updates and maintenance of both the reverse proxy and backend services are essential to ensure optimal functionality and security. Overall, a well-config
Author Profile
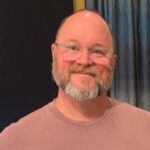
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?