How Can I View All the Python Libraries Installed on My System?
In the world of Python programming, libraries are the unsung heroes that empower developers to build robust applications with ease. Whether you’re a seasoned coder or just starting out, understanding how to manage and view the libraries installed in your Python environment is crucial for optimizing your workflow and ensuring your projects run smoothly. With thousands of libraries available, from data analysis tools like Pandas to web frameworks like Flask, knowing which ones you have at your disposal can significantly enhance your coding experience. In this article, we will explore the various methods to see all Python libraries installed in your system, helping you unlock the full potential of your programming toolkit.
When diving into Python development, it’s common to accumulate a variety of libraries over time. Each library serves a unique purpose, providing functions and features that can save you countless hours of coding. However, as your library collection grows, it can become challenging to keep track of what you have installed. Fortunately, Python offers several straightforward methods to list all the libraries currently in your environment, allowing you to manage dependencies effectively and avoid potential conflicts.
Understanding how to view your installed libraries is not just about organization; it’s also about ensuring compatibility and optimizing your projects. With the right tools at your fingertips, you can easily check for updates, identify unused libraries
Using pip to List Installed Libraries
One of the most straightforward methods to see all Python libraries installed in your environment is by using the `pip` command. `pip` is the package installer for Python and comes pre-installed with most Python distributions. To list all installed libraries, you can run the following command in your terminal or command prompt:
“`
pip list
“`
This command provides a table of installed packages along with their respective versions. The output will look similar to this:
Package | Version |
---|---|
numpy | 1.21.0 |
pandas | 1.3.0 |
requests | 2.25.1 |
Additionally, if you want to see the output in a more detailed format, you can use:
“`
pip freeze
“`
This command outputs a list of installed packages in a format that can be used in a `requirements.txt` file, which is useful for replicating the environment.
Using Python Code to List Installed Libraries
If you prefer to view installed libraries programmatically, you can utilize the `pkg_resources` module that comes with the `setuptools` package. Here’s how you can do it:
“`python
import pkg_resources
installed_packages = pkg_resources.working_set
installed_packages_list = sorted([“%s==%s” % (i.key, i.version) for i in installed_packages])
for package in installed_packages_list:
print(package)
“`
This code snippet retrieves and prints all installed libraries along with their versions in a sorted list.
Virtual Environments and Installed Libraries
When working with virtual environments, it is essential to activate the environment before checking installed libraries. This ensures that you are viewing the packages specific to that environment rather than the global Python installation. To activate a virtual environment, use the following command:
- On Windows:
“`
.\venv\Scripts\activate
“`
- On macOS/Linux:
“`
source venv/bin/activate
“`
Once activated, you can use `pip list` or `pip freeze` as previously described to see the libraries installed in that specific virtual environment.
Using Anaconda to List Installed Libraries
If you are using Anaconda, you can list installed packages in your conda environment with the following command:
“`
conda list
“`
This command will display a comprehensive list of all packages installed in the active conda environment, including their versions, build strings, and channels. The output will look like this:
Package | Version | Build | Channel |
---|---|---|---|
numpy | 1.21.0 | py38he8f5b81_1 | defaults |
pandas | 1.3.0 | py38he8f5b81_0 | defaults |
You can also specify a particular environment by adding the `-n` flag followed by the environment name:
“`
conda list -n myenv
“`
This command will list the packages installed in the specified conda environment, allowing for greater flexibility in managing dependencies.
Using the Command Line to List Installed Libraries
To view all Python libraries installed in your environment, the command line provides a straightforward approach. Depending on your operating system and Python version, you can use different commands.
- For pip (the package installer for Python):
- Open your terminal or command prompt.
- Execute the following command:
“`
pip list
“`
- This command will display a list of installed packages along with their versions.
- For pip with a specific format:
- To get a more detailed output, you can use:
“`
pip freeze
“`
- This will output installed packages in a format suitable for requirements files.
Using Python Code to List Installed Libraries
You can also retrieve the list of installed libraries programmatically by utilizing the `pkg_resources` module from the `setuptools` package.
“`python
import pkg_resources
installed_packages = pkg_resources.working_set
installed_packages_list = sorted([“%s==%s” % (i.key, i.version) for i in installed_packages])
for package in installed_packages_list:
print(package)
“`
This script lists all installed packages in a sorted order, displaying the package name alongside its version.
Using Anaconda to Manage Libraries
If you are utilizing Anaconda for package management, the `conda` command can be employed to list installed libraries.
- Open Anaconda Prompt and execute:
“`
conda list
“`
- This command will provide a comprehensive list of installed packages in the active conda environment, including their versions and build information.
Viewing Installed Libraries in Jupyter Notebooks
For users working within Jupyter Notebooks, you can run shell commands directly from a notebook cell:
“`python
!pip list
“`
This command will output the installed libraries directly within the notebook interface, making it convenient for quick checks.
Managing Virtual Environments
When using virtual environments, it’s essential to activate the desired environment before listing installed libraries. The activation commands may differ based on the environment management tool:
- For virtualenv:
- Activate the environment:
“`
source /path/to/venv/bin/activate On macOS/Linux
.\path\to\venv\Scripts\activate On Windows
“`
- Then use `pip list` to see the packages.
- For conda:
- Activate the environment:
“`
conda activate your_environment_name
“`
- Subsequently, run `conda list`.
Using Package Management Tools
Various package management tools can also be used to view installed libraries. The following tools provide graphical interfaces or enhanced command-line utilities:
Tool | Description |
---|---|
`pipenv` | Manages virtual environments and dependencies. Use `pipenv graph` to see installed packages. |
`poetry` | A dependency manager that provides `poetry show` to list installed packages. |
`pipdeptree` | Displays installed packages in a tree format. Install it using `pip install pipdeptree` and run `pipdeptree`. |
These tools facilitate better management and visualization of your Python libraries.
Expert Insights on Viewing Installed Python Libraries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To effectively see all Python libraries installed in your environment, utilizing the command ‘pip list’ in your terminal is the most straightforward approach. This command provides a comprehensive list of all packages along with their respective versions, which is essential for maintaining project dependencies.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “For those managing multiple environments, employing ‘pip freeze’ is invaluable. This command not only lists installed libraries but also formats the output for easy use in requirements files, facilitating smoother deployment and collaboration across teams.”
Sarah Kim (Data Scientist, AI Research Labs). “In addition to command-line tools, using Python scripts to programmatically access installed libraries through the ‘pkg_resources’ module can provide more flexibility. This method allows for custom filtering and integration into larger applications, enhancing the visibility of dependencies.”
Frequently Asked Questions (FAQs)
How can I see all Python libraries installed in my environment?
You can see all installed Python libraries by using the command `pip list` in your command line or terminal. This command will display a list of all packages along with their versions.
Is there a way to see installed libraries in a specific virtual environment?
Yes, activate the virtual environment first using the command `source
Can I see installed libraries with their details?
You can use the command `pip show
What command can I use to export a list of installed libraries?
You can export the list of installed libraries to a requirements file using the command `pip freeze > requirements.txt`. This file can be used later to recreate the environment.
Are there any graphical tools to view installed Python libraries?
Yes, tools like Anaconda Navigator and PyCharm provide graphical interfaces to manage and view installed libraries in your Python environments.
How do I check installed libraries if I am using Jupyter Notebook?
In a Jupyter Notebook, you can run the command `!pip list` in a code cell to display all installed libraries directly within the notebook interface.
In summary, identifying all the Python libraries installed in your environment is a straightforward process that can be accomplished using several methods. The most common approaches include utilizing the command-line interface with commands such as `pip list` or `pip freeze`, which provide a comprehensive list of installed packages along with their respective versions. Additionally, leveraging Python scripts to programmatically access the list of installed libraries through the `pkg_resources` module or the `importlib.metadata` library can also be effective for more advanced users.
Understanding how to view installed libraries is crucial for managing dependencies in Python projects. It allows developers to ensure that they are using the correct versions of libraries, facilitating compatibility and reducing the likelihood of encountering runtime errors. Furthermore, being aware of the installed packages can assist in identifying any unused or outdated libraries that may need to be updated or removed to optimize the development environment.
whether you are a beginner or an experienced developer, knowing how to see all Python libraries installed is an essential skill. It not only aids in maintaining a clean and efficient workspace but also enhances your overall productivity by ensuring that you have the necessary tools at your disposal. Regularly checking your installed libraries can lead to better project management and a smoother development experience.
Author Profile
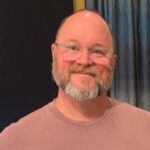
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?