How Can You Effectively Save Data in Python?
How To Save In Python: A Comprehensive Guide
In the ever-evolving world of programming, the ability to effectively manage and save data is a skill that every Python developer must master. Whether you’re building a simple script or a complex application, knowing how to save information efficiently can significantly enhance your project’s functionality and user experience. Python, with its rich ecosystem of libraries and straightforward syntax, provides a plethora of options for data storage, making it accessible for both beginners and seasoned developers alike.
As we delve into the intricacies of saving data in Python, we’ll explore various methods and best practices that cater to different needs. From saving data to files in formats like CSV or JSON to utilizing databases for more structured storage, each approach has its unique advantages and use cases. Understanding when and how to implement these techniques will empower you to make informed decisions that optimize your applications.
Moreover, we’ll touch on the importance of data persistence and how it plays a crucial role in application development. By learning how to save data effectively, you’ll not only enhance your coding skills but also ensure that your applications can handle real-world scenarios with ease. Get ready to unlock the full potential of Python’s data handling capabilities and elevate your programming projects to new heights!
Saving Data to Files
In Python, saving data to files is a common task that can be accomplished using built-in functions. The most common methods include writing to text files and binary files, and the choice between these often depends on the type of data being saved.
To save data to a text file, you can use the `open()` function in conjunction with the `write()` method. Here’s a simple example:
“`python
data = “Hello, World!”
with open(“example.txt”, “w”) as file:
file.write(data)
“`
This code snippet creates a new text file named `example.txt` and writes the string “Hello, World!” into it. The `with` statement ensures that the file is properly closed after its suite finishes, even if an error occurs.
For more complex data structures like lists or dictionaries, the `json` module can be used to serialize the data into a JSON format, which is both human-readable and easily parsed:
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
with open(“data.json”, “w”) as file:
json.dump(data, file)
“`
Saving Data in Different Formats
Python supports various formats for saving data. Below are some of the most commonly used formats and their respective libraries:
- CSV (Comma-Separated Values): Ideal for tabular data.
- JSON (JavaScript Object Notation): Suitable for structured data, especially for APIs.
- Pickle: A binary format for Python objects, useful for saving complex data structures.
Here’s a brief table summarizing these formats:
Format | Use Case | Library |
---|---|---|
CSV | Tabular data | csv |
JSON | Structured data | json |
Pickle | Python object serialization | pickle |
To save data in CSV format, you can use the `csv` module:
“`python
import csv
data = [[‘Name’, ‘Age’, ‘City’], [‘Alice’, 30, ‘New York’], [‘Bob’, 25, ‘Los Angeles’]]
with open(“data.csv”, “w”, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
Using Databases for Persistent Storage
For larger applications or when you need to manage relationships between different sets of data, utilizing a database is often the best choice. Python offers several libraries for database interaction, including `sqlite3` for SQLite databases, and `SQLAlchemy` for working with various SQL databases.
Here’s an example of how to save data to an SQLite database:
“`python
import sqlite3
connection = sqlite3.connect(‘example.db’)
cursor = connection.cursor()
cursor.execute(‘CREATE TABLE IF NOT EXISTS users (name TEXT, age INTEGER)’)
cursor.execute(‘INSERT INTO users (name, age) VALUES (?, ?)’, (‘Alice’, 30))
connection.commit()
connection.close()
“`
This code connects to an SQLite database, creates a table if it doesn’t exist, and inserts a record.
Effective data saving techniques in Python allow for efficient data management and retrieval, catering to various application needs. By leveraging file formats and database systems, developers can ensure that their applications efficiently handle data persistence.
File Saving in Python
Python provides various methods to save data to files, catering to different data types and formats. The most common approaches include using the built-in file handling capabilities and utilizing libraries like `pickle`, `json`, and `csv`.
Saving Text Files
To save data in a plain text file, Python’s built-in `open()` function is used, combined with methods like `write()` or `writelines()`. Here’s an example:
“`python
data = “Hello, World!”
with open(‘output.txt’, ‘w’) as file:
file.write(data)
“`
Key points to remember:
- Use `’w’` to write (overwrites the file if it exists).
- Use `’a’` to append (adds to the end of the file).
- Always close the file using `file.close()` or utilize `with` statement for automatic closure.
Saving JSON Data
JSON is a popular format for data exchange. Python provides the `json` module to facilitate saving dictionaries and lists as JSON files.
Example:
“`python
import json
data = {“name”: “Alice”, “age”: 30}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
For loading JSON back into Python, use `json.load()`.
Saving CSV Files
CSV (Comma-Separated Values) is widely used for tabular data. The `csv` module in Python allows easy reading and writing of CSV files.
Example:
“`python
import csv
data = [[“Name”, “Age”], [“Alice”, 30], [“Bob”, 25]]
with open(‘data.csv’, ‘w’, newline=”) as csv_file:
writer = csv.writer(csv_file)
writer.writerows(data)
“`
When dealing with CSVs, be mindful of:
- Specifying `newline=”` to prevent extra blank rows on Windows.
- Handling various delimiters as needed.
Using Pickle for Object Serialization
The `pickle` module allows for saving Python objects to files, enabling complex data structures to be stored and retrieved easily.
Example:
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.pkl’, ‘wb’) as pickle_file:
pickle.dump(data, pickle_file)
“`
To load the data back, use:
“`python
with open(‘data.pkl’, ‘rb’) as pickle_file:
data = pickle.load(pickle_file)
“`
Important considerations:
- Pickle is Python-specific; files are not human-readable.
- Be cautious when unpickling data from untrusted sources due to security risks.
Saving DataFrames with Pandas
For those using the Pandas library, saving DataFrames is straightforward and supports multiple formats.
Example for saving to CSV:
“`python
import pandas as pd
df = pd.DataFrame({‘Name’: [‘Alice’, ‘Bob’], ‘Age’: [30, 25]})
df.to_csv(‘dataframe.csv’, index=)
“`
Other formats supported include:
- Excel (`.xlsx`)
- HDF5
- JSON
Each method provides parameters to customize the output format, such as specifying separators or including/excluding the index.
When saving data in Python, the choice of method depends on the data structure and required format. Each method has its advantages and appropriate use cases. Understanding these nuances will enhance your data handling capabilities in Python.
Expert Insights on Efficient Data Saving in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “To effectively save data in Python, it is crucial to understand the different formats available, such as CSV, JSON, and binary. Each format serves unique purposes, and selecting the right one can significantly enhance data retrieval speed and storage efficiency.”
Michael Thompson (Senior Software Engineer, CodeCrafters Ltd.). “Utilizing libraries like Pandas and Pickle can streamline the data saving process in Python. Pandas provides robust methods for saving DataFrames, while Pickle allows for the serialization of Python objects, making it easier to save complex data structures.”
Sarah Patel (Python Developer, Open Source Solutions). “Implementing proper error handling during data saving operations is essential. This ensures that your application can gracefully handle issues like file permissions or disk space limitations, thus preventing data loss and maintaining integrity.”
Frequently Asked Questions (FAQs)
How can I save data to a file in Python?
You can save data to a file in Python using the built-in `open()` function along with the `write()` method. For example, to save text data, open a file in write mode (`’w’`), write the data, and then close the file.
What is the difference between ‘w’ and ‘a’ modes when saving files in Python?
The ‘w’ mode opens a file for writing and truncates the file to zero length if it exists, effectively overwriting it. The ‘a’ mode opens a file for appending, allowing you to add data to the end of the file without deleting existing content.
How can I save Python objects using the pickle module?
You can use the `pickle` module to serialize and save Python objects. Import the module, open a file in binary write mode (`’wb’`), and use `pickle.dump()` to write the object to the file. To read it back, use `pickle.load()`.
Is there a way to save data in JSON format in Python?
Yes, you can save data in JSON format using the `json` module. Use `json.dump()` to write a Python dictionary or list to a file after opening it in write mode. This allows for easy data interchange with other programming languages.
How do I save a DataFrame to a CSV file in Python using pandas?
To save a DataFrame to a CSV file, use the `to_csv()` method provided by the pandas library. Specify the filename and any additional parameters for formatting, such as `index=` to exclude row indices.
Can I save multiple data types in a single file in Python?
Yes, you can save multiple data types in a single file by using formats like JSON or by utilizing libraries such as `pickle` or `h5py`. These formats allow you to store complex data structures, including lists, dictionaries, and arrays, in a single file.
In summary, saving data in Python can be accomplished through various methods, each suited to different types of data and use cases. The most common approaches include using built-in file handling techniques for plain text or binary files, employing libraries such as `pickle` for object serialization, and utilizing structured formats like JSON and CSV for data interchange. Each method has its advantages, depending on the complexity and requirements of the data being saved.
Moreover, it is essential to consider the context in which the data will be used. For instance, if interoperability with other systems is a priority, formats like JSON or CSV may be preferable. Conversely, if the goal is to preserve Python objects and their states, the `pickle` module provides a straightforward solution. Understanding these nuances will enable developers to choose the most effective method for their specific needs.
Additionally, best practices should be followed when saving data, such as implementing error handling to manage file access issues, ensuring data integrity, and considering security implications when dealing with sensitive information. By adhering to these practices, developers can enhance the reliability and safety of their data-saving processes in Python.
Author Profile
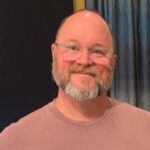
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?