How Can You Save a DataFrame as a CSV in Python?
In the world of data science and analytics, the ability to manipulate and store data efficiently is paramount. One of the most common tasks that data professionals encounter is saving data in a format that is both accessible and easy to share. Enter the CSV (Comma-Separated Values) format—a simple yet powerful way to store tabular data. If you’ve been working with dataframes in Python, you may find yourself wondering how to seamlessly export your data for future use or collaboration. This article will guide you through the process of saving a dataframe as a CSV file in Python, ensuring that your valuable data is preserved and ready for analysis.
Understanding how to save a dataframe as a CSV file is not just a technical skill; it’s a fundamental part of data management. With libraries like Pandas, Python makes it incredibly straightforward to convert complex data structures into a widely recognized format. This capability allows data scientists and analysts to share their findings with colleagues or integrate their work into larger data processing workflows. Whether you’re working on a small personal project or a large-scale enterprise application, mastering this skill will enhance your data handling capabilities and streamline your workflow.
As we delve into the specifics of saving dataframes as CSV files, you’ll discover the various options available, including how to customize your output to suit your needs. From
Saving a DataFrame to CSV
To save a DataFrame as a CSV file in Python, the `pandas` library provides a straightforward method called `to_csv()`. This function allows for a variety of options to customize the output, making it a versatile tool for data handling.
The basic syntax of the `to_csv()` method is as follows:
“`python
DataFrame.to_csv(filepath_or_buffer, sep=’,’, na_rep=”, float_format=None,
header=True, index=True, index_label=None, mode=’w’,
encoding=None, compression=’infer’, quoting=None,
line_terminator=None, chunksize=None,
date_format=None, doublequote=True,
escapechar=None, decimal=’.’,
errors=’strict’)
“`
Key Parameters
- filepath_or_buffer: The destination file path or a file-like object where the CSV will be saved.
- sep: The string used to separate values. By default, this is a comma (`,`).
- na_rep: String representation of missing data. Defaults to an empty string.
- header: Whether to write out the column names. Default is `True`.
- index: Whether to write row names (index). Default is `True`.
- encoding: Defines the encoding for the output file (e.g., ‘utf-8’).
- compression: Defines the compression mode (e.g., ‘gzip’).
Example Usage
Here is a simple example illustrating how to save a DataFrame as a CSV file:
“`python
import pandas as pd
Sample DataFrame
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
Save DataFrame to CSV
df.to_csv(‘output.csv’, index=)
“`
In this example, the DataFrame is saved to a file called `output.csv`, and the index is excluded from the output.
Customizing CSV Output
When saving a DataFrame, you might want to customize the output according to specific requirements. Below are some common customizations:
- Changing the delimiter: To use a semicolon instead of a comma, set `sep=’;’`.
- Handling missing values: To represent missing data as ‘N/A’, use `na_rep=’N/A’`.
- Specifying encoding: For UTF-8 encoding, use `encoding=’utf-8’`.
Example of Customization
“`python
df.to_csv(‘custom_output.csv’, sep=’;’, na_rep=’N/A’, encoding=’utf-8′, index=)
“`
Handling Different Data Types
When saving data, it is important to ensure that data types are preserved correctly. Below is a table outlining how different data types are handled during the CSV export:
Data Type | CSV Representation |
---|---|
Integer | Numeric value |
Float | Numeric value, decimal point included |
String | Text value, surrounded by quotes if necessary |
DateTime | ISO format by default (YYYY-MM-DD HH:MM:SS) |
Using the `to_csv()` method effectively allows for the seamless export of DataFrames to CSV files, accommodating various data types and customization options to fit your needs.
Saving a DataFrame as CSV Using Pandas
To save a DataFrame as a CSV file in Python, the Pandas library provides a straightforward method called `to_csv()`. This method allows you to export your DataFrame to a CSV file, specifying various parameters to customize the output.
Basic Syntax
The basic syntax for saving a DataFrame to a CSV file is as follows:
“`python
DataFrame.to_csv(‘filename.csv’, sep=’,’, index=True, header=True)
“`
- ‘filename.csv’: The name of the output file, including the .csv extension.
- sep: The delimiter to use (default is a comma).
- index: Boolean indicating whether to write row names (indices). Default is `True`.
- header: Boolean indicating whether to write column names. Default is `True`.
Example of Saving a DataFrame
Here is an example that demonstrates how to save a DataFrame to a CSV file:
“`python
import pandas as pd
Create a sample DataFrame
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
Save the DataFrame to a CSV file
df.to_csv(‘output.csv’, index=)
“`
In this example, the DataFrame is saved as ‘output.csv’ without the index.
Common Parameters
When using `to_csv()`, several parameters can be particularly useful:
Parameter | Description |
---|---|
`sep` | Specify a different delimiter (e.g., `sep=’\t’` for tab-separated values). |
`index` | Write row names (default is `True`). Set to “ to omit row indices. |
`header` | Write column names (default is `True`). Set to “ to omit headers. |
`columns` | Specify a subset of columns to write. |
`mode` | File mode (default is `’w’` for write). Use `’a’` to append to an existing file. |
`encoding` | Specify the encoding (e.g., `encoding=’utf-8’`). |
`compression` | Specify compression format (e.g., `compression=’gzip’`). |
Handling Special Cases
- Handling NaN Values: You can specify how to represent missing values in the CSV file with the `na_rep` parameter.
“`python
df.to_csv(‘output.csv’, na_rep=’N/A’)
“`
- Writing in Append Mode: If you need to add data to an existing CSV file without overwriting it, set the `mode` parameter to `’a’`.
“`python
df.to_csv(‘output.csv’, mode=’a’, header=)
“`
- Exporting Only Specific Columns: To save only selected columns from the DataFrame, use the `columns` parameter.
“`python
df.to_csv(‘output.csv’, columns=[‘Name’, ‘City’])
“`
Using the `to_csv()` method in Pandas allows for efficient data export with numerous customization options to suit various needs. By understanding the parameters available, you can create CSV files that meet your specific requirements.
Expert Insights on Saving DataFrames as CSV in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When saving a DataFrame as a CSV in Python, it is crucial to utilize the `pandas` library effectively. The `to_csv()` function allows for various parameters that can optimize the output, such as specifying the delimiter and handling missing data. This flexibility makes it an essential tool for data manipulation.”
Michael Chen (Senior Software Engineer, Data Solutions Group). “To ensure the integrity of your data when saving a DataFrame as a CSV, always check the encoding parameter in the `to_csv()` method. Using UTF-8 is generally recommended to avoid issues with special characters, especially when dealing with international datasets.”
Lisa Patel (Machine Learning Engineer, AI Analytics Corp.). “It is advisable to consider the size of your DataFrame when saving it as a CSV. For larger datasets, using the `chunksize` parameter in the `to_csv()` function can help manage memory usage effectively, allowing for smoother processing without overwhelming system resources.”
Frequently Asked Questions (FAQs)
How can I save a DataFrame as a CSV file in Python?
To save a DataFrame as a CSV file in Python, use the `to_csv()` method provided by the pandas library. For example, `df.to_csv(‘filename.csv’, index=)` will save the DataFrame `df` to a file named `filename.csv` without including the index.
What parameters can I use with the to_csv() method?
The `to_csv()` method accepts several parameters, including `sep` for specifying the delimiter, `header` to include or exclude column names, `index` to include or exclude row indices, and `mode` to define the file opening mode (e.g., ‘w’ for write, ‘a’ for append).
Can I specify a different encoding when saving a CSV file?
Yes, you can specify a different encoding by using the `encoding` parameter in the `to_csv()` method. For example, `df.to_csv(‘filename.csv’, encoding=’utf-8′)` saves the file with UTF-8 encoding.
Is it possible to save a DataFrame to a CSV file with a specific date format?
Yes, you can save a DataFrame with a specific date format by converting the date columns to strings with the desired format before using the `to_csv()` method. Use `pd.to_datetime()` and `dt.strftime()` for formatting.
How can I handle missing values when saving a DataFrame as a CSV?
You can handle missing values by using the `na_rep` parameter in the `to_csv()` method. For instance, `df.to_csv(‘filename.csv’, na_rep=’N/A’)` will replace NaN values with ‘N/A’ in the saved CSV file.
Can I append data to an existing CSV file using the to_csv() method?
Yes, you can append data to an existing CSV file by setting the `mode` parameter to ‘a’ in the `to_csv()` method. For example, `df.to_csv(‘filename.csv’, mode=’a’, header=)` appends the DataFrame to the specified file without writing the header again.
In summary, saving a DataFrame as a CSV file in Python is a straightforward process that primarily utilizes the Pandas library. This library provides a method called `to_csv()`, which facilitates the conversion of DataFrames into CSV format with a variety of customizable options. Users can specify parameters such as file path, delimiter, header inclusion, and index handling, allowing for flexibility in how the data is exported.
One of the key insights is the importance of understanding the various parameters available in the `to_csv()` method. For instance, the `index` parameter can be set to “ if the user does not want to include the DataFrame’s index in the output file. Additionally, the `sep` parameter allows users to define a custom delimiter, which can be particularly useful when dealing with data that contains commas. These options enhance the usability of the exported CSV file in different contexts.
Furthermore, it is essential to handle file paths correctly to avoid common pitfalls, such as overwriting existing files unintentionally. Users should also be mindful of the encoding format, especially when dealing with non-ASCII characters, to ensure that the data is accurately represented in the CSV file. Overall, mastering the process of saving DataFrames as CSV files
Author Profile
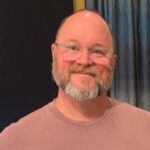
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?